题意:为什么 OpenAI 图像生成 API 在 Unity 中返回 400 Bad Request 错误?
问题背景:
I'm testing out dynamically generating images using OpenAI API in Unity. Amusingly, I actually generated most of this code from chatGPT.
我正在测试在 Unity 中使用 OpenAI API 动态生成图像。有趣的是,我实际上是通过 ChatGPT 生成了大部分代码。
The Error response is: "Your request contained invalid JSON: Expecting value: line 1 column 1 (char 0)". But I cant see anything wrong with the json formatting of my requestbody...
错误响应是:'你的请求包含无效的 JSON:期待值:第 1 行第 1 列 (字符 0)'。但我看不出我的请求体的 JSON 格式有什么问题......
I also found this other question which is probably failing for the same reason, whatever it is:
我还发现了另一个问题,可能因为同样的原因而失败,无论是什么原因:Why does Post request to OpenAI in Unity result in error 400?
Here is my code: 以下是我的代码:
public class PlayerScript : MonoBehaviour
{
// Replace API_KEY with your actual API key
private string API_KEY = "<api_key>";
private string API_URL = "https://api.openai.com/v1/images/generations";
void Start()
{
StartCoroutine(GetImage());
}
IEnumerator GetImage()
{
// Create a request body with the prompt "Player"
string requestBody = "{\"prompt\": \"Player\",\"n\": 1,\"size\": \"128x128\"}";
// Create a UnityWebRequest and set the request method to POST
UnityWebRequest www = UnityWebRequest.Post(API_URL, requestBody);
// Set the authorization header with the API key
www.SetRequestHeader("Authorization", "Bearer " + API_KEY);
// Set the content type header
www.SetRequestHeader("Content-Type", "application/json");
// Send the request
yield return www.SendWebRequest();
// Check for errors
if (www.isNetworkError || www.isHttpError)
{
Debug.LogError(www.error);
}
else
{
// do stuff
}
}
}
Any idea what's going wrong? Apologies if it's something obvious, never made web requests in Unity before.
知道哪里出了问题吗?如果问题显而易见请见谅,我以前从未在 Unity 中发过网络请求。
问题解决:
UnityWebRequests url-encodes content before submitting the request. This messes up the formatting. see:
UnityWebRequests 在提交请求之前会对内容进行 URL 编码,这会导致格式混乱。参见:prevent UnityWebRequest.Post() from Url-Encoding the data ? - Questions & Answers - Unity Discussions
using httpClient worked for me.
"使用 httpClient 对我来说是有效的。
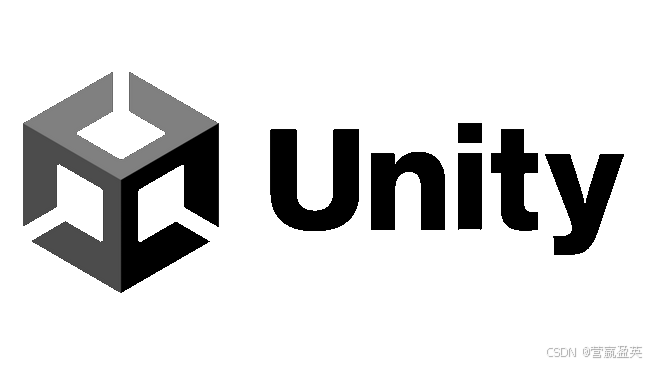