博客主页: 南来_北往
系列专栏:Spring Boot实战
前言
文件上传的功能实现是我们做Web应用时候最为常见的应用场景,比如:实现头像的上传,Excel文件数据的导入等功能,都需要我们先实现文件的上传,然后再做图片的裁剪,excel数据的解析入库等后续操作。
今天通过这篇文章,我们就来一起学习一下如何在Spring Boot中实现文件的上传。
实战
1、首先,在项目的pom.xml文件中添加必要的依赖项。对于文件上传功能,通常需要使用spring-boot-starter-web
和commons-fileupload
依赖。
XML
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.3</version>
</dependency>
</dependencies>
2、在application.properties或application.yml文件中配置文件上传的相关属性,例如最大文件大小限制、临时文件夹路径等。
XML
spring:
servlet:
multipart:
max-file-size: 10MB
max-request-size: 10MB
3、创建一个用于处理文件上传请求的控制器类。在该类中,定义一个处理POST请求的方法,并使用@RequestParam
注解来接收上传的文件。
java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return "Please select a file to upload.";
}
try {
// Save the uploaded file to a specific location
String fileName = file.getOriginalFilename();
File dest = new File("path/to/save/" + fileName);
file.transferTo(dest);
return "File uploaded successfully: " + fileName;
} catch (IOException e) {
e.printStackTrace();
return "Failed to upload file: " + e.getMessage();
}
}
}
4、启动Spring Boot应用程序后,可以使用工具(如Postman)或编写客户端代码来发送包含文件的POST请求到/upload
端点。确保请求的内容类型为multipart/form-data
,并在请求体中包含要上传的文件。
测试验证
第一步 :启动Spring Boot应用,访问 http://localhost:8080
,可以看到如下的文件上传页面。
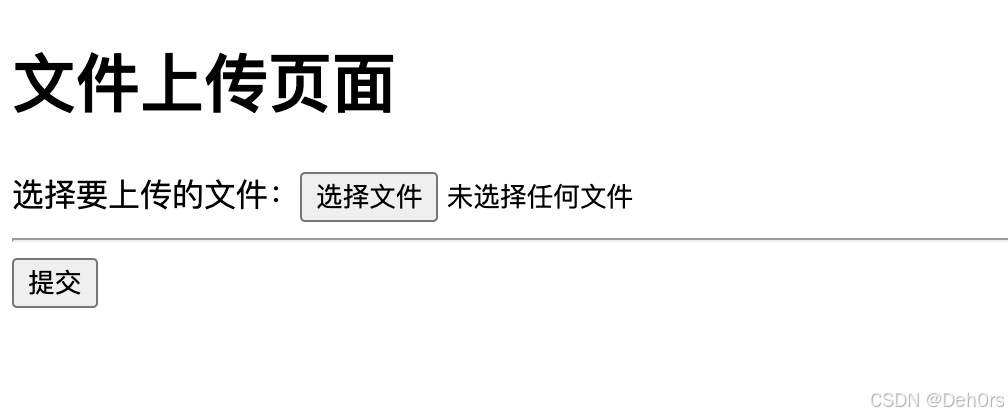
第二步:选择一个不大于2MB的文件,点击"提交"按钮,完成上传。
如果上传成功,将显示类似下面的页面:
你可以根据打印的文件路径去查看文件是否真的上传了。
结论
以上是一个简单的Spring Boot 2.x基础教程,演示了如何实现文件上传功能。你可以根据实际需求进行更多的定制和扩展。