69@[toc]
1.启停活动页面
1.Activity启动和结束
- 从当前页面跳到新页面
java
startActivity(new Intent(this, ActFinishActivity.class));
- 从当前页面返回上一个页面,相当于关闭当前页面
java
finish();
2.Activity生命周期
- onCreate:创建活动。把页面布局加载进内存,进入了初始状态。
- onStart:开始活动。把活动页面显示在屏幕上,进入了就绪状态。
- onResume:恢复活动。活动页面进入了活跃状态,能够与用户正常交互,例如允许响应用户的点击动作、允许输入文字等。
- onPause:暂停活动。页面进入暂停状态,无法与用户正常交互。
- onStop:停止活动。页面将不再屏幕上显示。
- onDestory:销毁活动。回收活动占用的系统资源,把页面从内存中清楚。
- onNewIntent:重用已有的活动实例。
3. Activity启动模式
- 默认启动模式 standard
- 栈顶复用模式 singleTop
- 栈内复用模式 singleTask
- 全局唯一模式 singleInstance
动态设置启动模式
- Intent.FLAG_ACTIVITY_NEW_TASK:开辟一个新的任务栈
- Intent.FLAG_ACTIVITY_SINGLE_TOP:当栈顶为待跳转的活动实例之时,则重用栈顶的实例
- Intent.FLAG_ACTIVITY_CLEAR_TOP:当栈中存在待跳转的活动实例时,则重新创建一个新的实例并清除原实例上方的所有实例
- Intent.FLAG_ACTIVITY_NO_HISTORY:栈中不保存新启动的活动实例
- Intent.FLAG_ACTIVITY_CLEAR_TASK:跳转到新页面时,栈中的原有实例被清空
java
Intent intent = new Intent(this, LoginSucessActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK | Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
2.在活动之间传递信息
1.显示Intent和隐式Intent
Intent是各组件之间信息沟通的桥梁,它用于Android各组件之间的通信,主要完成下列工作:
- 表明本次通信请求从哪里来,到哪里去,要怎么走
- 发起方携带本次通信需要的数据内容,接收方从收到的意图中解析数据
- 发起方想判断接收方的处理结果,意图就要负责让接收方传回应答的数据内容
元素名称 | 设置方法 | 说明与用途 |
---|---|---|
Component | setConponent | 组件,它指定意图的来源与目标 |
Action | setAction | 动作,它指定意图的动作行为 |
Data | setData | 即Uri,它指定动作要操控的数据路径 |
Category | addCategory | 类别,它指定意图的操作类别 |
type | setType | 数据类型,它指定消息的数据类型 |
Extras | putExtras | 扩展信息,它指定装载的包裹信息 |
Flags | setFlages | 标志位,它指定活动的启动标志 |
显示Intent
1.在Intent构造函数中指定
java
Intent intent = new Intent(this, ActFinishActivity.class);
2.调用意图对象的setClass方法指定
java
Intent intent = new Intent();
intent.setClass(this, ActFinishActivity.class);
3.调用意图对象setComponent方法
java
Intent intent = new Intent();
intent.setComponent(new ComponentName(this, ActFinishActivity.class));
隐式Intent
没有明确指定要跳转的目标活动,只给出一个动作字符串让系统自动匹配,属于模糊匹配。
Intent类系统动作常量名 | 系统动作的常量值 | 说明 |
---|---|---|
ACTION_MAIN | android.intent.action.MAIN | App启动时的入口 |
ACTION_VIEW | android.intent.action.VIEW | 向用户显示数据 |
ACTION_SEND | android.intent.action.SEND | 分享内容 |
ACTION_CALL | android.intent.action.CALL | 直接拨号 |
ACTION_DIAL | android.intent.action.DIAL | 准备拨号 |
ACTION_SENDTO | android.intent.action.SENDTO | 发送短信 |
ACTION_ANSWER | android.intent.action.ANSWER | 接听电话 |
2.向下一个Activity发送数据
Bundle
- 在代码中发送消息包裹,调用意图对象的putExtras方法,即可存入消息包裹
- 在代码中接收消息包裹,调用意图对象的getExtras方法,即可取出消息包裹
java
Intent intent = new Intent(this, ActReceiveActivity.class);
Bundle bundle = new Bundle();
bundle.putString("request_time",new Date().toString());
bundle.putString("request_content",tv_send.getText().toString());
intent.putExtras(bundle);
startActivity(intent);
3.向上一个Activity返回数据
处理下一个页面的应答数据,详细步骤如下:
- 上一个页面打包好请求数据,调用startActivityForResult方法执行跳转方法
- 下一个页面接收并解析请求数据,进行相应处理
- 下一个页面在返回上一个页面时,打包应答数据并调用setResult方法返回数据包裹
- 上一个页面重写方法onActivityResult,解析获得下一页面的返回数据
3.为活动补充附加信息
1.利用资源文件配置字符串
xml
<resources>
<string name="weather_str">晴天</string>
</resources>
java
// 从strings.xml中获取名叫weather_str的文本内容
tv_resource.setText(getString(R.string.weather_str));
2.利用元数据传递配置信息
在Java代码中获取元数据信息的步骤分为三步:
- 调用getPackageManager方法获得当前应用的包管理器
- 调用包管理器的getActivityInfo方法获得当前活动的信息对象
- 活动信息对象的metaData是Bundle包裹类型,调用包裹对象的getString即可获得指定名称的参数值
java
// 通过getPackageManager()获取包管理器
PackageManager packageManager = getPackageManager();
try {
// 使用getActivityInfo方法获取当前活动的信息,同时请求元数据
ActivityInfo activityInfo = packageManager.getActivityInfo(getComponentName(), PackageManager.GET_META_DATA);
String weather = activityInfo.metaData.getString("weather");
tv_meta.setText(weather);
} catch (PackageManager.NameNotFoundException e) {
throw new RuntimeException(e);
}
3.给应用页面注册快捷方式
元数据不仅能传递简单的字符串参数,还能传送更复杂的资源数据,比如支付宝的快捷式菜单。
元数据的meta-data标签除了前面说到的name属性和value属性,还拥有resource属性,该属性可指定一个XML文件,表示元数据想要的复杂信息保存于XML数据之中。
利用元数据配置快捷菜单的步骤如下:
- 在res/values/strings.xml添加各个菜单项名称的字符串配置
- 创建res/xml/shortcuts.xml,在该文件中填入各组菜单项的快捷方式定义(每个菜单对应哪个活动页面)
- 给activity节点注册元数据的快捷菜单配置
xml
<activity
android:name=".ActStartActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data android:name="android.app.shortcuts"
android:resource="@xml/shortcuts" />
</activity>
xml
<?xml version="1.0" encoding="utf-8"?>
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut android:shortcutId="first"
android:enabled="true"
android:icon="@drawable/ic_launcher_foreground"
android:shortcutShortLabel="@string/first_short"
android:shortcutLongLabel="@string/first_long">
<intent android:action="android.intent.action.VIEW"
android:targetPackage="com.zzzjian.demo2"
android:targetClass="com.zzzjian.demo2.ActStartActivity"/>
<categories android:name="android.shortcut.conversation"/>
</shortcut>
<shortcut android:shortcutId="second"
android:enabled="true"
android:icon="@drawable/ic_launcher_foreground"
android:shortcutShortLabel="@string/second_short"
android:shortcutLongLabel="@string/second_long">
<intent android:action="android.intent.action.VIEW"
android:targetPackage="com.zzzjian.demo2"
android:targetClass="com.zzzjian.demo2.JumpFirstActivity"/>
<categories android:name="android.shortcut.conversation"/>
</shortcut>
<shortcut android:shortcutId="third"
android:enabled="true"
android:icon="@drawable/ic_launcher_foreground"
android:shortcutShortLabel="@string/third_short"
android:shortcutLongLabel="@string/third_long">
<intent android:action="android.intent.action.VIEW"
android:targetPackage="com.zzzjian.demo2"
android:targetClass="com.zzzjian.demo2.LoginInputActivity"/>
<categories android:name="android.shortcut.conversation"/>
</shortcut>
</shortcuts>
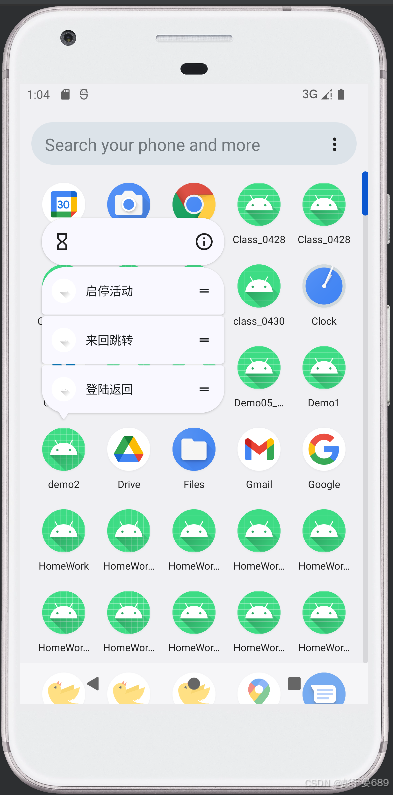