前言
在C语言中,getchar
是一个非常实用的函数,用于从标准输入流(通常是键盘)读取单个字符。这对于处理文本输入非常有用,尤其是在需要逐个字符处理的情况下。本文将深入探讨 getchar
函数的用法和特点,并提供一些示例代码。
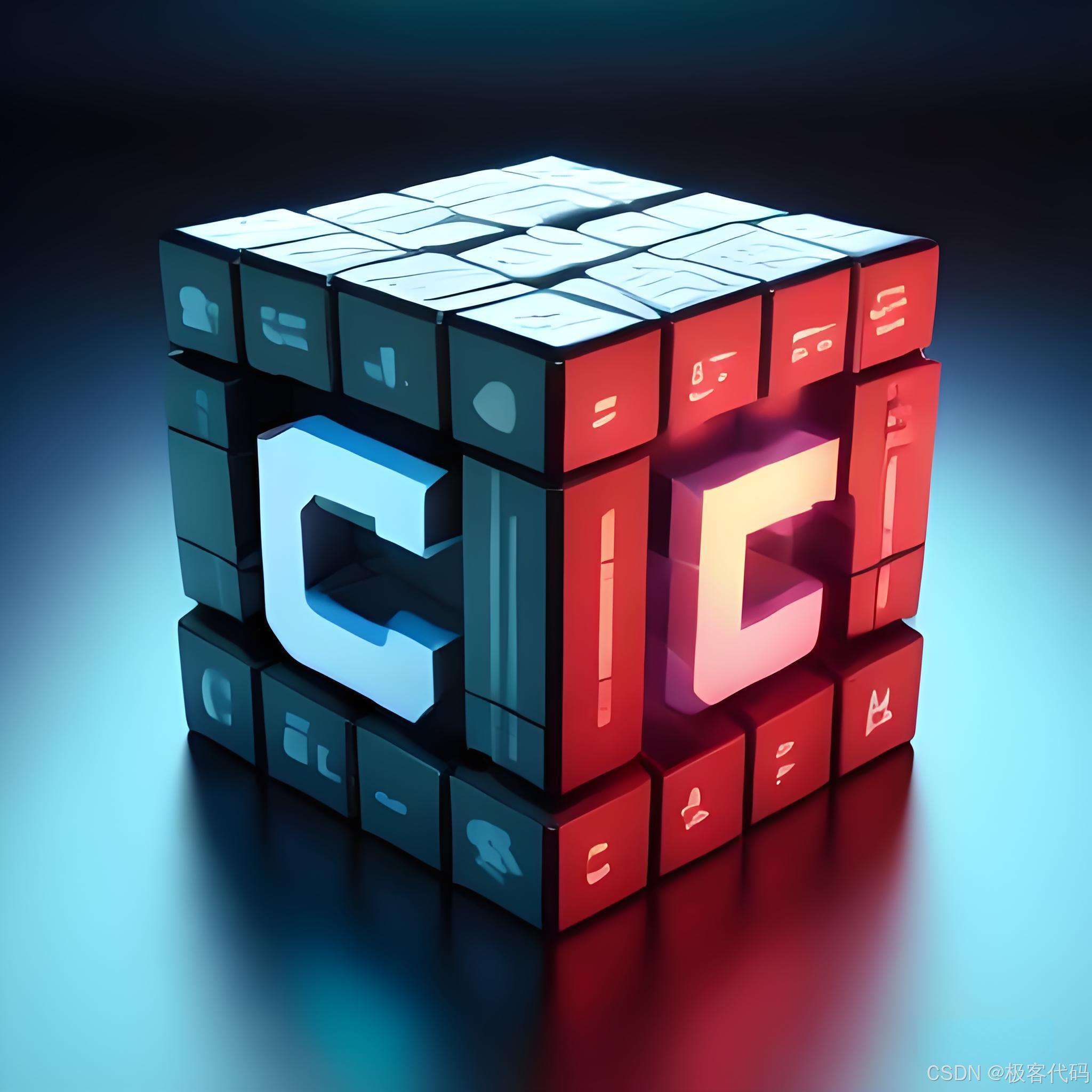
- getchar 函数简介
getchar
函数是从标准输入流读取单个字符的标准库函数。它属于 <stdio.h>
头文件的一部分。
基本语法:
int getchar(void);
getchar
函数返回一个 int
类型的值,这是因为 ASCII 字符可以用 unsigned char
表示,而 unsigned char
可以隐式转换为 int
。如果到达文件结束符(EOF),则返回 -1
。
- getchar 函数的基本使用
让我们看一个简单的例子,演示如何使用 getchar
函数读取用户输入的一个字符。
1#include <stdio.h>
2
3int main() {
4 int ch;
5
6 printf("Enter a character: ");
7 ch = getchar(); // 读取一个字符
8 printf("You entered: %c\n", ch);
9
10 return 0;
11}
输出:
1Enter a character: A
2You entered: A
解释:
int ch;
定义一个整数变量ch
用于存储输入的字符。ch = getchar();
读取用户输入的一个字符。printf("You entered: %c\n", ch);
打印输入的字符。
- getchar 与缓冲区
getchar
函数默认会等待用户输入一个完整的行,然后才读取第一个字符。这意味着如果你按下回车键后才调用 getchar
,它会读取你之前输入的第一个字符。为了避免这种情况,你可以使用 scanf
或者 getc
函数来清空缓冲区。
1#include <stdio.h>
2
3int main() {
4 int ch;
5
6 printf("Press any key and then enter: ");
7 getchar(); // 清空缓冲区
8 ch = getchar(); // 读取一个字符
9 printf("You entered: %c\n", ch);
10
11 return 0;
12}
输出:
1Press any key and then enter: A
2You entered: A
解释:
getchar();
在读取下一个字符之前清空缓冲区。
- 使用 getchar 处理多行输入
getchar
可以连续读取多个字符,直到遇到文件结束符(EOF)。下面的示例演示了如何使用 getchar
读取多行文本。
1#include <stdio.h>
2
3int main() {
4 int ch;
5
6 printf("Enter some text (press Ctrl+D to finish):\n");
7
8 while ((ch = getchar()) != EOF) {
9 printf("%c", ch); // 输出读取的字符
10 }
11
12 printf("\n");
13
14 return 0;
15}
输出:
1Enter some text (press Ctrl+D to finish):
2Hello
3World
4Hello World
解释:
while ((ch = getchar()) != EOF)
循环读取字符直到遇到文件结束符。printf("%c", ch);
打印读取的字符。
- getchar 与条件判断
getchar
可以与条件判断结合使用,以实现特定的逻辑流程。
1#include <stdio.h>
2
3int main() {
4 int ch;
5
6 printf("Enter a character: ");
7 ch = getchar();
8
9 if (ch >= 'a' && ch <= 'z') {
10 printf("You entered a lowercase letter.\n");
11 } else if (ch >= 'A' && ch <= 'Z') {
12 printf("You entered an uppercase letter.\n");
13 } else {
14 printf("You entered something else.\n");
15 }
16
17 return 0;
18}
输出:
1Enter a character: a
2You entered a lowercase letter.
解释:
- 使用
if
语句根据输入字符的范围做出判断。
- getchar 与文件结束符
当 getchar
遇到文件结束符(EOF)时,它会返回 -1
。你可以利用这一点来检测输入是否结束。
1#include <stdio.h>
2
3int main() {
4 int ch;
5
6 printf("Enter some text (press Ctrl+D to finish):\n");
7
8 while ((ch = getchar()) != EOF) {
9 printf("%c", ch); // 输出读取的字符
10 }
11
12 printf("\nEnd of input reached.\n");
13
14 return 0;
15}
输出:
1Enter some text (press Ctrl+D to finish):
2Hello
3World
4Hello World
5End of input reached.
解释:
- 当
getchar
返回-1
时,循环结束。
结论
getchar
函数是C语言中处理字符输入的一个重要工具。通过上述示例,你应该已经了解了如何使用 getchar
函数以及它的一些高级用法。这种能力对于处理文本输入和编写交互式的程序非常有帮助。