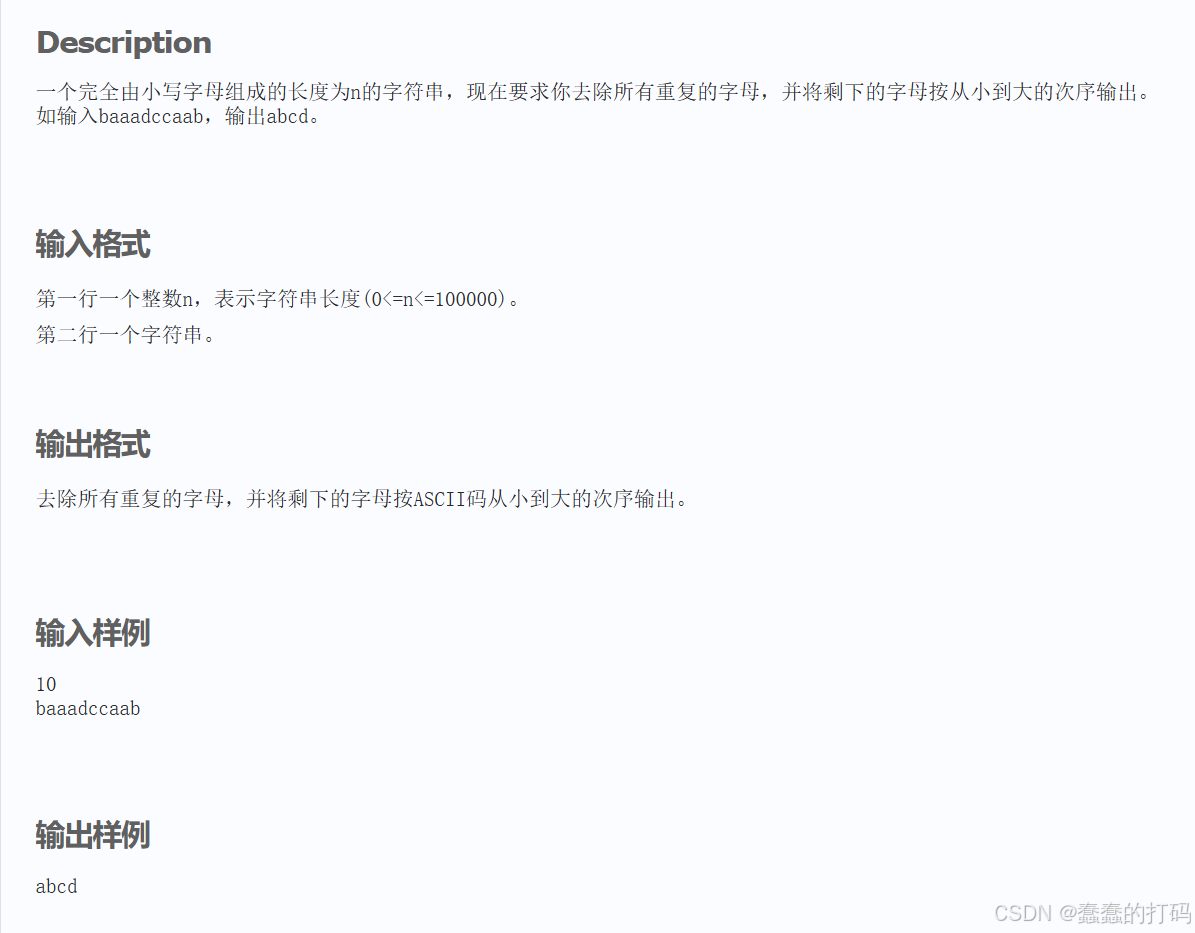
思路
-
**读取输入**:读取字符串的长度和字符串本身。
-
**使用集合去重**:利用集合(`set`)去除字符串中的重复字母。
-
**排序**:将集合中的字母按ASCII码从小到大排序。
-
**输出结果**:将排序后的字母拼接成字符串并输出。
伪代码
```
function remove_duplicates_and_sort(n, s):
if n == 0:
return ""
create a set unique_chars
for each char in s:
add char to unique_chars
convert unique_chars to a list and sort it
join the sorted list into a string
return the resulting string
```
C++代码
cpp
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
using namespace std;
string remove_duplicates_and_sort(int n, const string& s) {
if (n == 0) {
return "";
}
set<char> unique_chars(s.begin(), s.end());
vector<char> sorted_chars(unique_chars.begin(), unique_chars.end());
sort(sorted_chars.begin(), sorted_chars.end());
return string(sorted_chars.begin(), sorted_chars.end());
}
int main() {
int n;
string s;
cin >> n >> s;
string result = remove_duplicates_and_sort(n, s);
cout << result << endl;
return 0;
}
总结
-
**问题建模**:将字符串中的字母去重并排序。
-
**算法选择**:使用集合去重,使用排序算法对集合中的字母进行排序。
-
**实现细节**:利用集合和向量来存储和处理字母,最后将结果拼接成字符串输出。
-
**边界条件**:处理字符串长度为0的情况,直接返回空字符串。