如何得到一个圆角对话框?
步骤:
1、继承自QDiaglog
2、去掉系统自带的边框
3、设置背景透明,不设置4个角会有多余的部分出现颜色
4、对话框内部添加1个QWidget,给这个widget设置圆角,并添加到布局中让他充满对话框
5、后续对话框的所有内容都添加在这个widget里面
6、模拟QMessageBox的静态方法,提供一个静态方法,调用这个静态方法可以直接显示一个圆角对话框
举例:
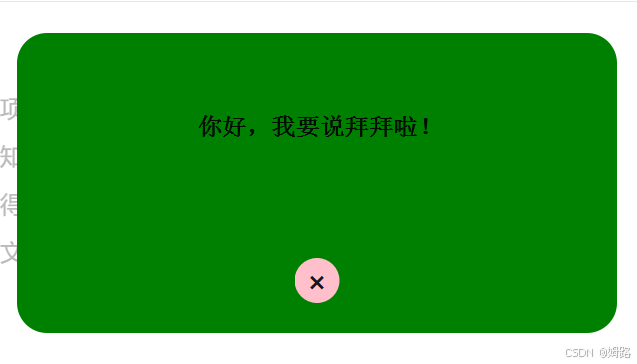
cpp
#ifndef ROUNDEDDIALOG_H
#define ROUNDEDDIALOG_H
#include <QDialog>
#include<QHBoxLayout>
#include<QLabel>
#include<QPushButton>
class RoundedDialog : public QDialog
{
Q_OBJECT
public:
//模拟QMessageBox的静态方法,调用这个静态方法可以直接显示一个圆角对话框
static int roundedDialog()
{
RoundedDialog d;
return d.exec();
}
RoundedDialog(QWidget *parent = nullptr) : QDialog(parent)
{
resize(400,200);
//1.去掉系统自带的边框
setWindowFlag(Qt::FramelessWindowHint);
//2.设置背景透明,不设置4个角会有颜色
setAttribute(Qt::WA_TranslucentBackground);
//内部添加1个QWidget,给这个widget设置圆角,并添加到布局中让他充满对话框
QHBoxLayout* h_box=new QHBoxLayout(this);
h_box->setSpacing(0);
h_box->setContentsMargins(0,0,0,0);
QWidget* w=new QWidget(this);
w->setStyleSheet(".QWidget{border-radius:20px;background-color:green}");
h_box->addWidget(w);
//后续对话框的所有内容都添加在这个widget里面
QLabel* label=new QLabel("你好,我要说拜拜啦!",w);
label->setAlignment(Qt::AlignCenter);
label->setStyleSheet(R"(font: 900 12pt "Arial Black";)");
label->move(120,50);
QPushButton* btn_close=new QPushButton("×",this);
btn_close->setStyleSheet("border-radius:15px;font-size:18px;font-weight:bold;background-color:pink");
btn_close->setGeometry(185,150,30,30);
connect(btn_close,&QPushButton::clicked,this,&QDialog::accept);
}
~RoundedDialog()=default;
};
#endif // ROUNDEDDIALOG_H