四、文件的顺序读写
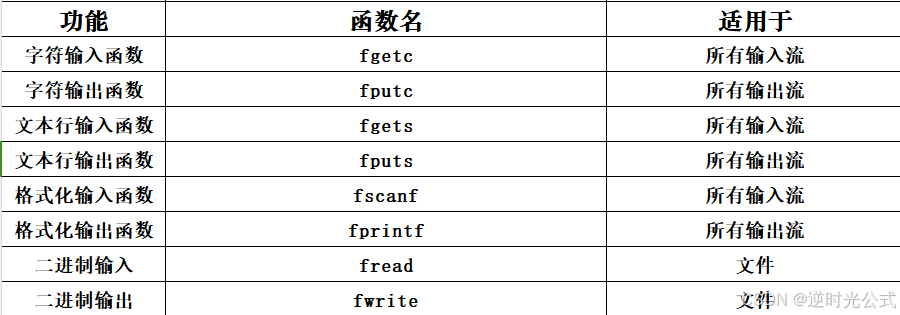
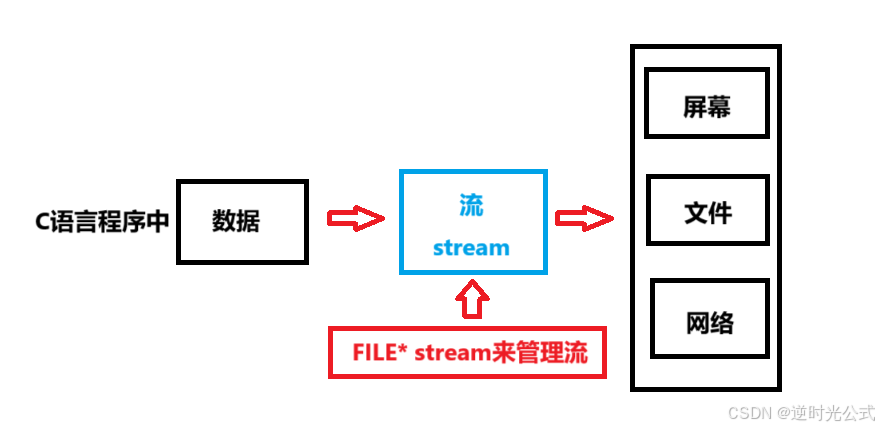
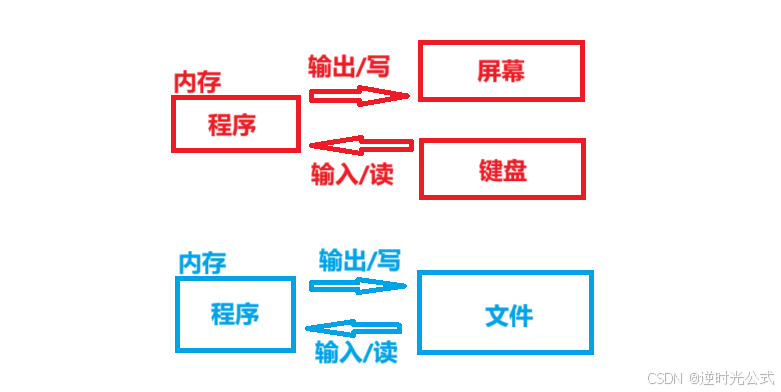
1、fputc (字符输出函数/写)

将一个字符写入文件中
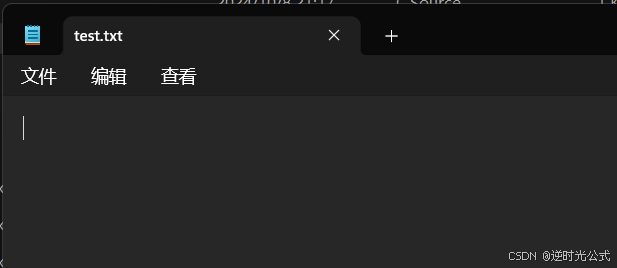
cs
#include <stdio.h>
int main()
{
FILE* pf = fopen("test.txt", "w");
//如果打开失败,返回空指针NULL
if (pf == NULL)
{
perror("fopen");
return 1;
}
//写操作
fputc('a', pf);
fputc('b', pf);
fputc('c', pf);
fputc('d', pf);
fputc('e', pf);
//关闭文件
fclose(pf);
pf = NULL;
return 0;
}
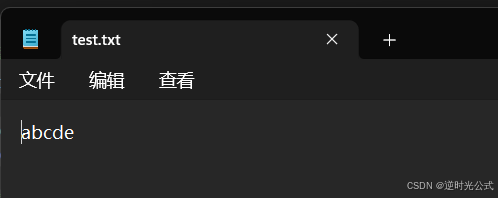
顺序输出一个字符一个字符写,写完一个字符指针指向下一个
2、fgetc(字符输入函数/读)

从指针指向的文件中读字符,返回读到字符的ASCII码值, 顺序输入一个字符一个字符读,读完一个字符指针指向下一个
cs
#include <stdio.h>
int main()
{
FILE* pf = fopen("test.txt", "r");
//如果打开失败,返回空指针NULL
if (pf == NULL)
{
perror("fopen");
return 1;
}
//读操作
char ch = fgetc(pf);
printf("%c", ch);//a
ch = fgetc(pf);
printf("%c", ch);//b
ch = fgetc(pf);
printf("%c", ch);//c
//关闭文件
fclose(pf);
pf = NULL;
return 0;
}
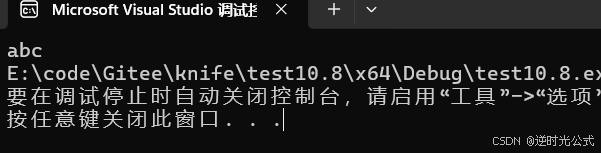