to
查找最佳的轮廓模式
bash
import cv2 as cv
import numpy as np
from matplotlib import pyplot as plt
img = cv.imread('data/test02.png',0)
ret,thresh1 = cv.threshold(img,127,255,cv.THRESH_BINARY)
ret,thresh2 = cv.threshold(img,127,255,cv.THRESH_BINARY_INV)
ret,thresh3 = cv.threshold(img,127,255,cv.THRESH_TRUNC)
ret,thresh4 = cv.threshold(img,127,255,cv.THRESH_TOZERO)
ret,thresh5 = cv.threshold(img,127,255,cv.THRESH_TOZERO_INV)
titles = ['Original Image','BINARY','BINARY_INV','TRUNC','TOZERO','TOZERO_INV']
images = [img, thresh1, thresh2, thresh3, thresh4, thresh5]
for i in range(6):
plt.subplot(2,3,i+1),plt.imshow(images[i],'gray')
plt.title(titles[i])
plt.xticks([]),plt.yticks([])
plt.show()
bash
import cv2 as cv
import numpy as np
from matplotlib import pyplot as plt
img = cv.imread('data/test01.png',0)
img = cv.medianBlur(img,5)
ret,th1 = cv.threshold(img,127,255,cv.THRESH_BINARY)
th2 = cv.adaptiveThreshold(img,255,cv.ADAPTIVE_THRESH_MEAN_C, cv.THRESH_BINARY,11,2)
th3 = cv.adaptiveThreshold(img,255,cv.ADAPTIVE_THRESH_GAUSSIAN_C, cv.THRESH_BINARY,11,2)
titles = ['Original Image', 'Global Thresholding (v = 127)',
'Adaptive Mean Thresholding', 'Adaptive Gaussian Thresholding']
images = [img, th1, th2, th3]
for i in range(4):
plt.subplot(2,2,i+1),plt.imshow(images[i],'gray')
plt.title(titles[i])
plt.xticks([]),plt.yticks([])
plt.show()
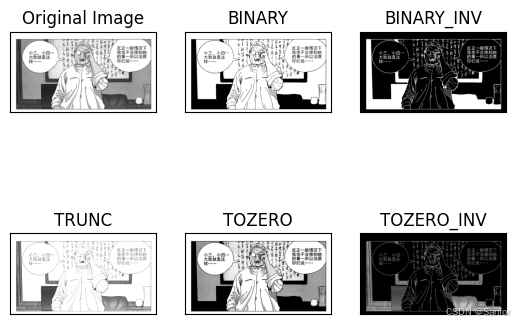
轮廓检测
bash
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取图像
image = cv2.imread('data/test01.png')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用二值化处理
_, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV)
# 进行形态学操作以去除噪声
kernel = np.ones((5, 5), np.uint8)
morph = cv2.morphologyEx(binary, cv2.MORPH_CLOSE, kernel)
# 查找轮廓
contours, _ = cv2.findContours(morph, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 创建一个用于保存分割文本框的列表
text_boxes = []
# 遍历所有轮廓
for contour in contours:
# 计算轮廓的边界框
x, y, w, h = cv2.boundingRect(contour)
aspect_ratio = w / float(h)
print(f'x={x}, y={y}, w={w}, h={h}')
# 过滤掉小的轮廓
if aspect_ratio > 0.1:
# 提取文本框区域
text_box = image[y:y+h, x:x+w]
text_boxes.append(text_box)
# 在原图上绘制边界框
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 保存分割的文本框
text_boxes.reverse()
for i, box in enumerate(text_boxes):
# cv2.imwrite(f'text_box_{i}.png', box)
plt.subplot(len(text_boxes),2,i+1),plt.imshow(box,'gray')
plt.title(f'text_box_{i}.png')
plt.xticks([]),plt.yticks([])
plt.show()