背景:在写串口时候发现需要显示时候显示日志,并且还能支持滚动,于是自己动手封装了一个LogTextView组件
下面直接上代码:
java
public class LogTextView extends ScrollView {
private TextView textView;
private Handler mainHandler; // 用于在主线程中更新UI
public LogTextView(Context context) {
super(context);
this.init(context);
}
public LogTextView(Context context, AttributeSet attrs) {
super(context, attrs);
this.init(context);
}
private void init(Context context) {
this.textView = new TextView(context);
this.textView.setTextColor(Color.WHITE);
this.setOverScrollMode(View.OVER_SCROLL_ALWAYS);
this.textView.setTextSize(14);
this.textView.setPadding(16, 0, 16, 0);
this.textView.setLineSpacing(1.5f, 1.5f); // 设置行间距
this.textView.setSingleLine(false); // 允许多行显示
this.textView.setMaxLines(Integer.MAX_VALUE); // 最大行数为无限
this.textView.setEllipsize(null); // 不使用省略号
this.textView.setHorizontallyScrolling(false);
this.addView(this.textView);
this.mainHandler = new Handler(Looper.getMainLooper());
}
public void appendLog(String log) {
this.mainHandler.post(() -> {
String currentText = this.textView.getText().toString();
if (!currentText.isEmpty()) {
this.textView.setText(currentText + "\n" + log);
} else {
this.textView.setText(log); // 如果为空,则直接设置
}
this.fullScroll(View.FOCUS_DOWN);
this.invalidate(); // 强制刷新视图
});
}
public void clearLogs() {
this.textView.setText("");
}
public String getCurrentLogs() {
return this.textView.getText().toString();
}
public void copyToClipboard(Context context) {
String logContent = this.getCurrentLogs();
ClipboardManager clipboard = (ClipboardManager) context.getSystemService(Context.CLIPBOARD_SERVICE);
ClipData clip = ClipData.newPlainText("Logs", logContent);
clipboard.setPrimaryClip(clip);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
return super.onTouchEvent(event);
}
}
用法:
xml布局里面
XML
<com.example.serialportupgrade.view.LogTextView
android:id="@+id/tv_log"
android:layout_gravity="top"
android:layout_width="match_parent"
android:layout_height="240dp" />
Activity或者Fragment里面调用
java
public class MainActivity extends BaseActivity {
private LogTextView tv_log;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.setContentView(R.layout.activity_main);
this.tv_log = this.findViewById(R.id.tv_log);
this.tv_log.setPadding(0, 0, 0, 0);
this.tv_log.appendLog("欢迎使用\n");
}
}
效果图:
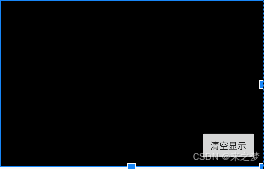