delphi开发的dll接口协议,为方便H5调用将dll转换为webstock传输模式。
转换服务
前端调用效果
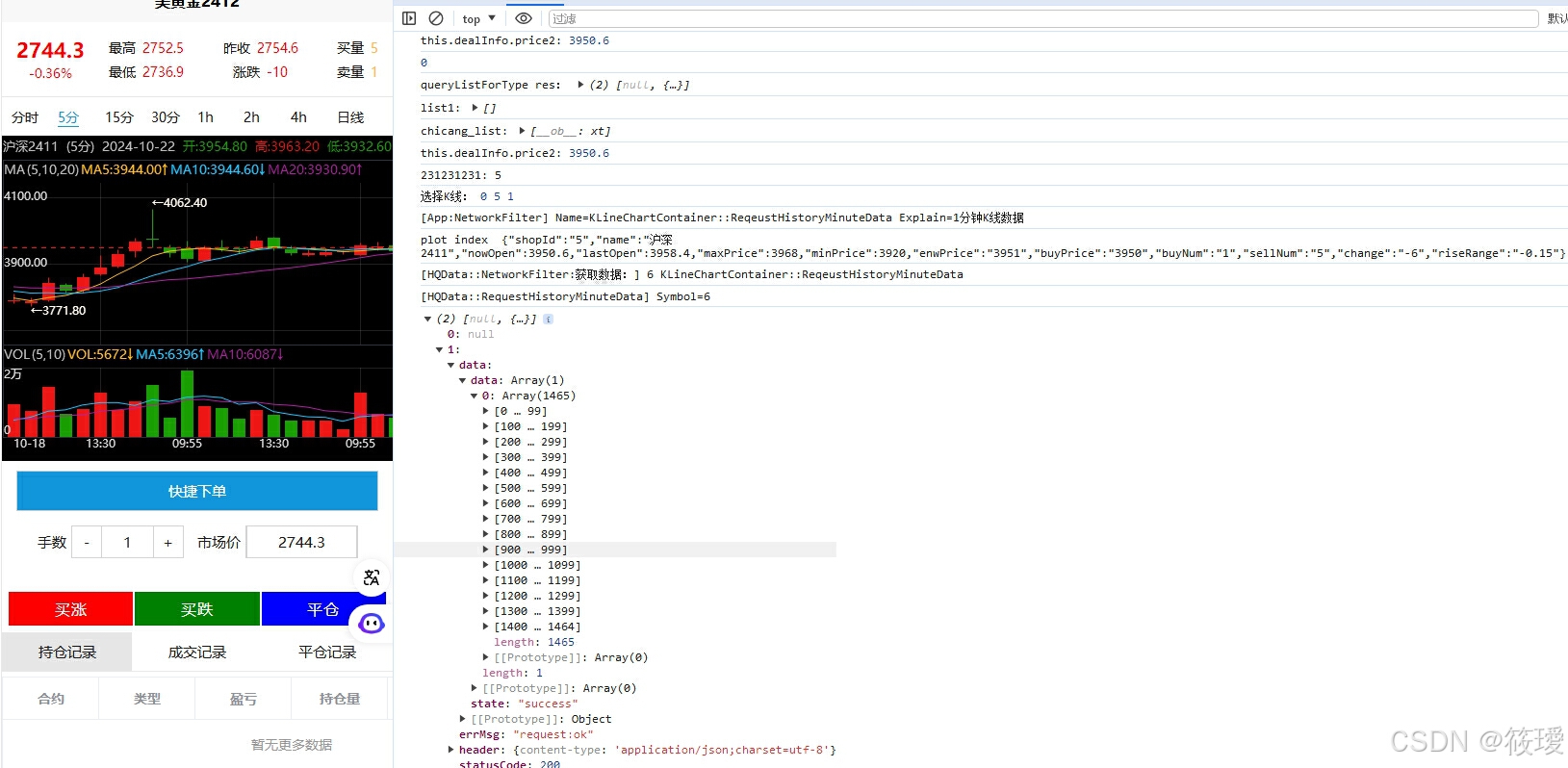
C#代码
cs
#define SHIPAN_IP "IP"
typedef wchar_t* (__stdcall* DLL_GetLoginBytes)(
wchar_t* a_strUserID, wchar_t* a_strPassword, wchar_t* a_strIP, wchar_t* a_strAppVer, unsigned char **bufferOut, int* bufferSize);
typedef void (__stdcall* DLL_Decrypt)(
unsigned char *bufferIn,int bufferInLen, unsigned char** bufferOutSuccess, int* bufferOutSizeSuccess, unsigned char** bufferOutFail
, int* bufferOutSizeFail, bool* blnSuccess);
typedef wchar_t* (__stdcall* DLL_GetCmdBytes)(
wchar_t* a_strCmd, unsigned char** bufferOut, int* bufferSize);
DLL_GetLoginBytes GetLoginBytes;
DLL_Decrypt Decrypt;
DLL_GetCmdBytes GetCmdBytes;
int loadFunc()
{
char filepath[MAX_PATH] = { 0 };
HMODULE hModule = GetModuleHandleA("Trident.exe");
GetModuleFileNameA(hModule, filepath, _MAX_PATH);
std::string dll_path = filepath;
dll_path = dll_path.erase(dll_path.find_last_of('\\') + 1, strlen("Trident.exe"));
SetDllDirectoryA(dll_path.data());
std::string tmp_dll_path = dll_path + "TridentClientLink.dll";
HANDLE hHandle = LoadLibraryA(tmp_dll_path.data());
if (!hHandle)
{
return -1;
}
SetDllDirectoryA("");
GetLoginBytes = (DLL_GetLoginBytes)GetProcAddress((HMODULE)hHandle, "GetLoginBytes");
Decrypt = (DLL_Decrypt)GetProcAddress((HMODULE)hHandle, "Decrypt");
GetCmdBytes = (DLL_GetCmdBytes)GetProcAddress((HMODULE)hHandle, "GetCmdBytes");
if (!GetLoginBytes || !Decrypt || !GetCmdBytes)
{
FreeLibrary(hModule);
return -1;
}
return 0;
}
#define BUF_MAX 1024*200
struct evhttp* http_server = NULL;
event* term = NULL;
event_base* base = NULL;
struct service_cfg
{
char ip[32];
int port;
};
//解析post请求数据
void get_post_message(char** buf, struct evhttp_request* req)
{
size_t post_size = 0;
post_size = evbuffer_get_length(req->input_buffer);//获取数据长度
printf("====line:%d,post len:%d\n", __LINE__, post_size);
if (post_size <= 0)
{
printf("====line:%d,post msg is empty!\n", __LINE__);
return;
}
else
{
printf("====line:%d,post len:%d\n", __LINE__, post_size);
*buf = (char*)calloc(sizeof(char), post_size + 1);
memcpy(*buf, evbuffer_pullup(req->input_buffer, -1), post_size);
printf("====line:%d,post msg:%s\n", __LINE__, *buf);
}
}
SOCKET g_mnptrSocket = 0;
std::wstring g_wip;
int ConnectGmSocket(char* srvIp, int srvPort)
{
int lnRV = Func_SocketInitialize();
if (Func_SocketInitialize() != 0)
{
return lnRV;
}
lnRV = Func_SocketCreateConn(srvIp, srvPort, &g_mnptrSocket);
if (lnRV != 0)
{
return lnRV;
}
return 0;
}
int SendGmSocket(char* pchReqData, int nReqDataLen, char* pchRespData, int* pnRespDataLen)
{
int lnRV = 0;
if (!g_mnptrSocket)
{
return -1;
}
lnRV = Func_SocketSendMsg(g_mnptrSocket, (unsigned char*)pchReqData, (long)nReqDataLen);
if (lnRV != 0)
{
return lnRV;
}
lnRV = Func_SocketRecvMsg(g_mnptrSocket, (unsigned char*)pchRespData, (unsigned long*)pnRespDataLen);
if (lnRV != 0)
{
return lnRV;
}
return 0;
}
int CloseGmSocket()
{
if (!g_mnptrSocket)
{
return 0;
}
Func_SocketFinalize(g_mnptrSocket);
g_mnptrSocket = 0;
return 0;
}
std::string dealStringBuffer(unsigned char * bufferOut, int bufferOutLen,int &ret)
{
char respData[60000] = { 0 };
int respDataLen = 60000;
std::string outdata;
ret = -1;
if (!SendGmSocket((char*)bufferOut, bufferOutLen, respData, &respDataLen) && respDataLen > 13)
{
bool flag = true;
for (int i = 0; i < 10; i++)
{
if (respData[i] != 'a')
{
flag = false;
}
}
if (flag)
{
char strLen[4] = { 0 };
memcpy(strLen, respData + 10, 3);
int len = atoi(strLen);
if (len > 0)
{
//unsigned char* encData = new unsigned char(len + 1);
//memset(encData, 0, len + 1);
unsigned char encData[60000] = { 0 };
memcpy(encData, respData + 13, len);
unsigned char* sucessData = NULL;
unsigned char* failData = NULL;
int sucessDataLen = 0;
int failDataLen = 0;
bool isSucess = false;
Decrypt((unsigned char*)respData, respDataLen, &sucessData, &sucessDataLen, &failData, &failDataLen, &isSucess);
if (isSucess && sucessDataLen > 0)
{
outdata = ascii2utf8((char*)sucessData);
ret = 0;
}
else
{
outdata = "";
ret = -1;
}
}
}
}
return outdata;
}
std::string LongGmDealStringBuffer(char* srvIp, int srvPort, unsigned char* bufferOut, int bufferOutLen,int &isSucess)
{
if (!bufferOut)
{
return "";
}
std::string outdata;
if (!g_mnptrSocket)
{
if (!ConnectGmSocket((char*)SHIPAN_IP, srvPort))
{
outdata = dealStringBuffer(bufferOut, bufferOutLen, isSucess);
}
}
else
outdata = dealStringBuffer(bufferOut, bufferOutLen, isSucess);
return outdata;
}
char* get_message(struct evhttp_request* req)
{
char* buf = NULL;
if (req == NULL)
{
printf("====line:%d,%s\n", __LINE__, "input param req is null.");
return NULL;
}
evhttp_add_header(req->output_headers, "Access-Control-Allow-Origin", "*");
evhttp_add_header(req->output_headers, "Content-Type", "application/json;charset=utf8");
evhttp_add_header(req->output_headers, "Access-Control-Allow-Methods", "POST,GET,OPTIONS,DELETE");
evhttp_add_header(req->output_headers, "Access-Control-Max-Age", "3600");
evhttp_add_header(req->output_headers, "Access-Control-Allow-Headers", "x-requested-with,content-type,X-Token,Authorization");
evhttp_add_header(req->output_headers, "Access-Control-Allow-Credentials", "true");
get_post_message(&buf, req);//获取请求数据,一般是json格式的数据
if (buf == NULL)
{
printf("====line:%d,%s\n", __LINE__, "get_post_message return null.");
}
else
{
//可以使用json库解析需要的数据
printf("====line:%d,request data:%s", __LINE__, buf);
}
return buf;
}
//处理post请求
void http_handler_post_logon(struct evhttp_request* req, void* arg)
{
printf("====line:%d,%s\n", __LINE__, "into logon");
char* buf = get_message(req);
if (!buf)
{
return;
}
//回响应
struct evbuffer* retbuff = evbuffer_new();
if (retbuff == NULL)
{
printf("====line:%d,%s\n", __LINE__, "retbuff is null.");
return;
}
std::string outdata("");
std::string userID, passWord, ip, ver;
int count = 0;
if (buf)
{
cJSON* json = cJSON_Parse((char*)buf);
if (json && json->type == cJSON_Object)
{
if (cJSON_GetObjectItem(json, "userID"))
{
userID = cJSON_GetObjectItem(json, "userID")->valuestring;
}
if (cJSON_GetObjectItem(json, "passWord"))
{
passWord = cJSON_GetObjectItem(json, "passWord")->valuestring;
}
if (cJSON_GetObjectItem(json, "ip"))
{
ip = cJSON_GetObjectItem(json, "ip")->valuestring;
}
if (userID.empty())
{
outdata = ascii2utf8("用户名不能为空");
}
if (passWord.empty())
{
outdata = ascii2utf8("密码不能为空");
}
if (ip.empty())
{
outdata = ascii2utf8("ip不能为空");
}
if (!userID.empty() && !passWord.empty() && !ip.empty())
{
std::wstring userid(userID.begin(), userID.end()), pass(passWord.begin(), passWord.end()), wip(ip.begin(), ip.end());
g_wip = wip;
unsigned char* bufferOut = NULL;
int bufferOutLen = 0;
wchar_t* ret = GetLoginBytes((wchar_t*)userid.data(), (wchar_t*)pass.data(), (wchar_t*)wip.data(), (wchar_t*)L"v1.2.6",
&bufferOut, &bufferOutLen);
if (ret && !wcscmp(ret, L"ok"))
{
int isSuccess = 0;
outdata = LongGmDealStringBuffer((char*)SHIPAN_IP, 9002, bufferOut, bufferOutLen, isSuccess);
CloseGmSocket();
}
else if (ret)
{
std::wstring tmpwout = ret;
std::string tmpout(tmpwout.begin(), tmpwout.end());
outdata = tmpout;
}
}
}
free(buf);
}
if (outdata.empty())
{
outdata = "{error}";
}
evbuffer_add_printf(retbuff, outdata.data());
evhttp_send_reply(req, HTTP_OK, "OK", retbuff);
evbuffer_free(retbuff);
}
#include <string>
#include <codecvt>
#include <locale>
std::wstring string_to_wstring(const std::string& str)
{
std::wstring_convert<std::codecvt_utf8_utf16<wchar_t>> converter;
return converter.from_bytes(str);
}
#include <openssl/md5.h>
int md5(char* inbuf, char* outbuf)
{
MD5_CTX ctx;
unsigned char md[16];
int i;
MD5_Init(&ctx);
MD5_Update(&ctx, (unsigned char*)inbuf, strlen(inbuf));
MD5_Final(md, &ctx);
memcpy(outbuf, md, 16);
return 0;
}
//处理post请求
void http_handler_post_cmd(struct evhttp_request* req, void* arg)
{
char* buf = get_message(req);
if (!buf)
{
return;
}
//回响应
struct evbuffer* retbuff = evbuffer_new();
if (retbuff == NULL)
{
printf("====line:%d,%s\n", __LINE__, "retbuff is null.");
return;
}
std::string outdata("");
std::string cmd, userID, passWord,ip;
std::string pName, kType;
std::string jcType, commodityID, commodityCount, commodityPrice, bsAttr;
int count = 0;
if (buf)
{
cJSON* json = cJSON_Parse((char*)buf);
if (json && json->type == cJSON_Object)
{
if (cJSON_GetObjectItem(json, "cmd"))
{
if (cJSON_GetObjectItem(json, "cmd")->type == cJSON_Number)
{
cmd = std::to_string(cJSON_GetObjectItem(json, "cmd")->valueint);
}
else
{
cmd = cJSON_GetObjectItem(json, "cmd")->valuestring;
}
}
if (cJSON_GetObjectItem(json, "userID"))
{
userID = cJSON_GetObjectItem(json, "userID")->valuestring;
}
if (cJSON_GetObjectItem(json, "passWord"))
{
passWord = cJSON_GetObjectItem(json, "passWord")->valuestring;
}
if (cJSON_GetObjectItem(json, "ip"))
{
ip = cJSON_GetObjectItem(json, "ip")->valuestring;
}
if (cJSON_GetObjectItem(json, "pName"))
{
pName = cJSON_GetObjectItem(json, "pName")->valuestring;
}
if (cJSON_GetObjectItem(json, "kType"))
{
kType = cJSON_GetObjectItem(json, "kType")->valuestring;
}
if (cJSON_GetObjectItem(json, "jcType"))
{
jcType = cJSON_GetObjectItem(json, "jcType")->valuestring;
}
if (cJSON_GetObjectItem(json, "commodityID"))
{
commodityID = cJSON_GetObjectItem(json, "commodityID")->valuestring;
}
if (cJSON_GetObjectItem(json, "commodityCount"))
{
commodityCount = cJSON_GetObjectItem(json, "commodityCount")->valuestring;
}
if (cJSON_GetObjectItem(json, "commodityPrice"))
{
commodityPrice = cJSON_GetObjectItem(json, "commodityPrice")->valuestring;
}
if (cJSON_GetObjectItem(json, "bsAttr"))
{
bsAttr = cJSON_GetObjectItem(json, "bsAttr")->valuestring;
}
if (userID.empty())
{
outdata = ascii2utf8("用户名不能为空");
}
if (passWord.empty())
{
outdata = ascii2utf8("密码不能为空");
}
if (cmd.empty())
{
outdata = ascii2utf8("命令不能为空");
}
if (!cmd.empty() && !userID.empty()&& !passWord.empty())
{
std::wstring userid(userID.begin(), userID.end()), pass(passWord.begin(), passWord.end()), wcmd(cmd.begin(), cmd.end());
std::wstring wip(ip.begin(), ip.end());
int isSuccess = 0;
std::wstring incmd = wcmd;
incmd.append(L";");
/* incmd.append(userid);
incmd.append(L";");
incmd.append(WGetMD5(passWord));
incmd.append(L";");*/
if (wcmd == L"2" || wcmd == L"02")
{
unsigned char* bufferOut = NULL;
int bufferOutLen = 0;
std::string str;
GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
for (size_t i = 0; i < 1; i++)
{
outdata += LongGmDealStringBuffer((char*)SHIPAN_IP, 9011, bufferOut, bufferOutLen, isSuccess);
}
}
else if (wcmd == L"200")
{
std::string tempstr;
md5(const_cast<char*>(passWord.c_str()), const_cast<char*>(tempstr.c_str()));
std::cout << tempstr.c_str() << std::endl;
printf("====line:%s\n", tempstr.c_str());
std::wstring wpName(pName.begin(), pName.end()), wkType(kType.begin(), kType.end()),wpass(tempstr.begin(), tempstr.end());
unsigned char* bufferOut = NULL;
int bufferOutLen = 0;
std::string str;
incmd = L"200;";
incmd += userid;
incmd += L";";
incmd += wpass;// WGetMD5
incmd += L";";
incmd += wpName;
incmd += L";";
incmd += wkType;
incmd += L";";
GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
outdata = LongGmDealStringBuffer((char*)SHIPAN_IP/*"139.224.128.7"*/, 9527, bufferOut, bufferOutLen, isSuccess);
}
else
{
unsigned char* bufferOut = NULL;
int bufferOutLen = 0;
wchar_t* ret = GetLoginBytes((wchar_t*)userid.data(), (wchar_t*)pass.data(), (wchar_t*)L"1.2.3.5", (wchar_t*)L"v1.2.6",
&bufferOut, &bufferOutLen);
if (ret && !wcscmp(ret, L"ok"))
{
std::string str = LongGmDealStringBuffer((char*)SHIPAN_IP, 9002, bufferOut, bufferOutLen, isSuccess);
}
{
wchar_t* ret = NULL;
unsigned char* bufferOut = NULL;
int bufferOutLen = 0;
if (wcmd == L"15")
{
std::wstring wjcType(jcType.begin(), jcType.end()),
wcommodityID(commodityID.begin(), commodityID.end()),
wcommodityCount(commodityCount.begin(), commodityCount.end()),
wcommodityPrice(commodityPrice.begin(), commodityPrice.end()),
wbsAttr(bsAttr.begin(), bsAttr.end());
incmd = L"15;";
incmd += wjcType;
incmd += L";";
incmd += wcommodityID;
incmd += L";";
incmd += wcommodityCount;
incmd += L";";
incmd += wcommodityPrice;
incmd += L";";
incmd += wbsAttr;
incmd += L";";
incmd += wip;
incmd += L";";
ret = GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
}
else if (wcmd == L"17")
{
std::string pcType;
if (cJSON_GetObjectItem(json, "pcType"))
{
pcType = cJSON_GetObjectItem(json, "pcType")->valuestring;
}
std::wstring wpcType(pcType.begin(), pcType.end()),
wcommodityID(commodityID.begin(), commodityID.end()),
wcommodityCount(commodityCount.begin(), commodityCount.end()),
wcommodityPrice(commodityPrice.begin(), commodityPrice.end()),
wbsAttr(bsAttr.begin(), bsAttr.end());
incmd = L"17;";
incmd += wpcType;
incmd += L";";
incmd += wcommodityID;
incmd += L";";
incmd += wcommodityCount;
incmd += L";";
incmd += wcommodityPrice;
incmd += L";";
incmd += wbsAttr;
incmd += L";";
incmd += wip;
incmd += L";";
ret = GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
}
else if (wcmd == L"34")
{
std::string zydw,zsdw,zyje,zsje;
if (cJSON_GetObjectItem(json, "zydw"))
{
zydw = cJSON_GetObjectItem(json, "zydw")->valuestring;
}
if (cJSON_GetObjectItem(json, "zsdw"))
{
zsdw = cJSON_GetObjectItem(json, "zsdw")->valuestring;
}
if (cJSON_GetObjectItem(json, "zyje"))
{
zyje = cJSON_GetObjectItem(json, "zyje")->valuestring;
}
if (cJSON_GetObjectItem(json, "zsje"))
{
zsje = cJSON_GetObjectItem(json, "zsje")->valuestring;
}
std::wstring wzydw(zydw.begin(), zydw.end()),
wzsdw(zsdw.begin(), zsdw.end()),
wzyje(zyje.begin(), zyje.end()),
wzsje(zsje.begin(), zsje.end()),
wcommodityID(commodityID.begin(), commodityID.end()),
wcommodityCount(commodityCount.begin(), commodityCount.end()),
wbsAttr(bsAttr.begin(), bsAttr.end());
incmd = L"34;";
incmd += wcommodityID;
incmd += L";";
incmd += wbsAttr;
incmd += L";";
incmd += wcommodityCount;
incmd += L";";
incmd += wzydw;
incmd += L";";
incmd += wzsdw;
incmd += L";";
incmd += wzyje;
incmd += L";";
incmd += wzsje;
incmd += L";";
incmd += wip;
incmd += L";";
ret = GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
}
else
{
ret = GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
}
if (ret && !wcscmp(ret, L"ok"))
{
int port;
std::string str;
str = LongGmDealStringBuffer((char*)SHIPAN_IP, 9527, bufferOut, bufferOutLen, isSuccess);
outdata += str;
if (wcmd == L"20" || wcmd == L"23" || wcmd == L"24" || wcmd == L"25" || wcmd == L"35")
{
auto vec_str = _cut_string((char*)str.data(), str.length(), ';');
if (vec_str.size() == 3)
{
int loop = atoi(vec_str[2].data());
for (int i = 0; i < loop - 1; i++)
{
GetCmdBytes((wchar_t*)incmd.data(), &bufferOut, &bufferOutLen);
outdata += LongGmDealStringBuffer((char*)SHIPAN_IP, 9527, bufferOut, bufferOutLen, isSuccess);
}
}
}
}
else if (ret)
{
std::wstring tmpwout = ret;
std::string tmpout(tmpwout.begin(), tmpwout.end());
outdata = tmpout;
}
}
}
}
}
free(buf);
}
CloseGmSocket();
if (outdata.empty())
{
outdata = "{error}";
}
evbuffer_add_printf(retbuff, outdata.data());
evhttp_send_reply(req, HTTP_OK, "OK", retbuff);
evbuffer_free(retbuff);
}
#ifdef _WIN32
int init_win_socket()
{
WSADATA wsaData;
if (WSAStartup(MAKEWORD(2, 2), &wsaData) != 0)
{
return -1;
}
return 0;
}
void uninit_win_socket()
{
WSACleanup();
}
#endif
static int
display_listen_sock(struct evhttp_bound_socket* handle)
{
struct sockaddr_storage ss;
evutil_socket_t fd;
ev_socklen_t socklen = sizeof(ss);
char addrbuf[128];
void* inaddr;
const char* addr;
int got_port = -1;
fd = evhttp_bound_socket_get_fd(handle);
memset(&ss, 0, sizeof(ss));
if (getsockname(fd, (struct sockaddr*)&ss, &socklen)) {
perror("getsockname() failed");
return 1;
}
if (ss.ss_family == AF_INET) {
got_port = ntohs(((struct sockaddr_in*)&ss)->sin_port);
inaddr = &((struct sockaddr_in*)&ss)->sin_addr;
}
else if (ss.ss_family == AF_INET6) {
got_port = ntohs(((struct sockaddr_in6*)&ss)->sin6_port);
inaddr = &((struct sockaddr_in6*)&ss)->sin6_addr;
}
else {
fprintf(stderr, "Weird address family %d\n",
ss.ss_family);
return 1;
}
addr = evutil_inet_ntop(ss.ss_family, inaddr, addrbuf,
sizeof(addrbuf));
if (!addr) {
fprintf(stderr, "evutil_inet_ntop failed\n");
return 1;
}
return 0;
}
static void
do_term(int sig, short events, void* args)
{
event_base_loopbreak(base);
fprintf(stderr, "Got %i, Terminating\n", sig);
}
int start_service_libevent(const char* http_addr, int http_port)
{
#ifdef WIN32
init_win_socket();
#endif
if (http_server)
{
printf("====line:%d,%s\n", __LINE__, "http server is started.");
return 0;
}
printf("start port %d", http_port);
//初始化
base = event_base_new();
if (!base)
{
printf("====line:%d,%s\n", __LINE__, "Couldn't create an event_base: exiting.");
return -1;
}
//启动http服务端
http_server = evhttp_new(base);
if (http_server == NULL)
{
printf("====line:%d,%s\n", __LINE__, "http server start failed.");
return -1;
}
//设置请求超时时间(s)
evhttp_set_timeout(http_server, 30);
evhttp_set_cb(http_server, "/logon", http_handler_post_logon, NULL);
evhttp_set_cb(http_server, "/cmd", http_handler_post_cmd, NULL);
evhttp_set_cb(http_server, "", http_handler_post_logon, NULL);
evhttp_bound_socket* handle = evhttp_bind_socket_with_handle(http_server, http_addr, http_port);
if (display_listen_sock(handle)) {
return -1;
}
term = evsignal_new(base, 2, do_term, NULL);
if (!term)
return -1;
if (event_add(term, NULL))
return -1;
event_base_dispatch(base);
return 0;
}
void stop_service()
{
if (http_server)
{
evhttp_free(http_server);
http_server = NULL;
}
if (term)
{
event_free(term);
term = NULL;
}
if (base)
{
event_base_free(base);
base = NULL;
}
#ifdef WIN32
uninit_win_socket();
#endif
}
int main()
{
if (loadFunc())
{
printf("调用动态库失败!");
system("pause");
return -1;
}
start_service_libevent("0.0.0.0", 8099);
//start_service_libevent("0.0.0.0", 7543);
std::cout << "Hello World!\n";
return 0;
}