登录注册窗口(一)
前言
前两集我们完成了历史消息记录的功能,那么我们这一集开始就要把我们的登录注册窗口完成了。
那么我们废话不多说直接来看需求。
需求分析
我们在启动这个程序的时候需要先到登录的窗口,只有当我们完成登录,我们才可以进入到我们的程序的主窗口。我们来思考一下这个窗口需要有什么组件?
在思考组件之前,我们也很快能发现,我们一定要有两种或以上的登录方式,不仅限于用户名登录以及手机发送验证码登录,我们还可以使用二维码进行登录,当然这个后续是否会做再议,我们暂时只做两种方式。
组件一定是要有一个大标题显示我们正在做什么,以及使用用户名登录的时候,我们需要有输入用户名、输入密码、输入验证码的三个输入框,一个显示验证码的图片,还要有两个切换成手机号登录以及切换到注册的两个功能按钮,当然还有一个提交按钮!效果如下面一组图。
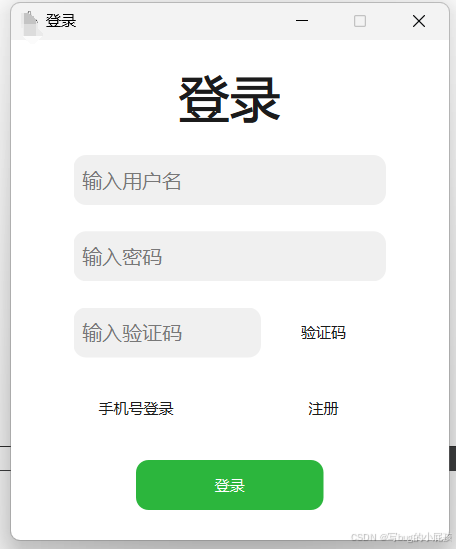
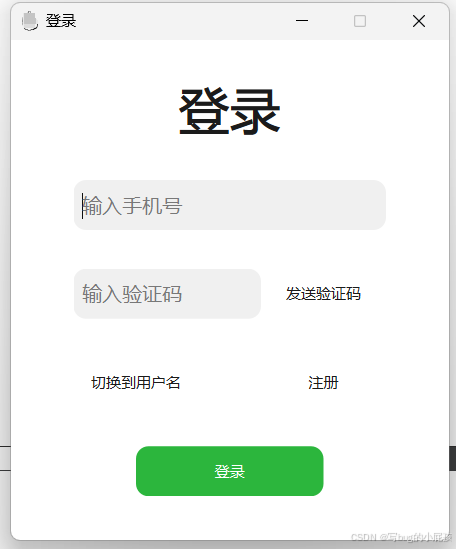
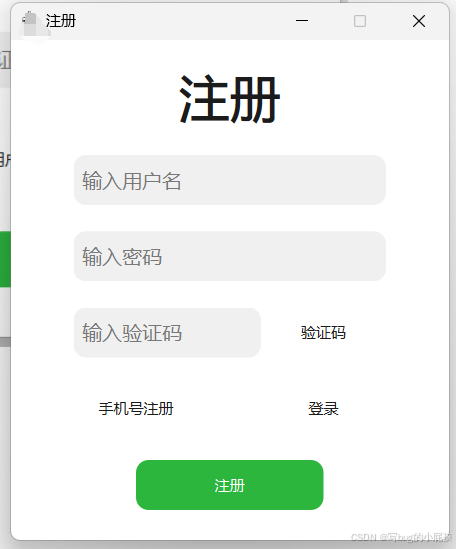
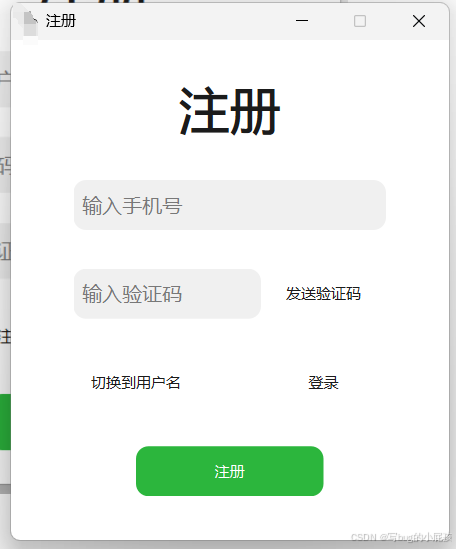
我们可以发现登录和注册的时候是长得一模一样的,只有在切换成手机号或者用户名的时候长的不太一样,那么我们就会分为两个类来完成这一整个登录注册的窗口。
Main函数调整
为了能够我们想随时切换是否跳过登录,我们创建了一个新的宏变量。
cpp
#if TEST_SKIP_LOGIN
MainWidget* w = MainWidget::getInstance();
w->show();
#else
LoginWidget* loginWidget = new LoginWidget(nullptr);
loginWidget->show();
#endif
哦对,记得这里的窗口一定要放置到堆里面去,不要放到栈区里面,说简单点就是要new出来!
为什么这么说?因为以下代码是清除堆区的内容,你放置在栈区,就是违法操作了。
cpp
this->setAttribute(Qt::WA_DeleteOnClose);
我之前就遇到这个问题,请看下图
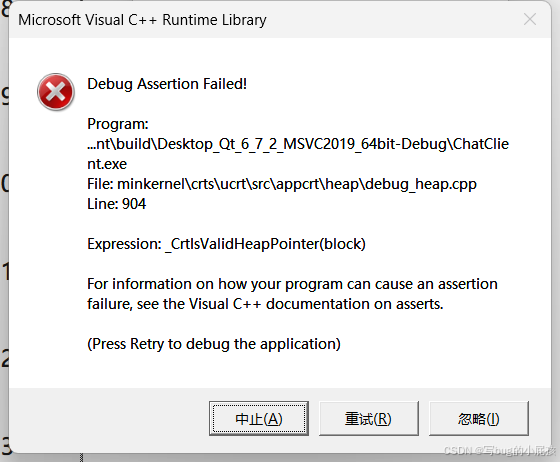
在我点击关闭窗口的时候,就弹出了这个东西。
两个布局
今天我们就不把槽函数给完成了,槽函数放到下一集我们再完成。
LoginWidget
cpp
LoginWidget::LoginWidget(QWidget *parent)
: QWidget{parent}
{
//基本属性
this->setFixedSize(350,400);
this->setWindowTitle("登录");
this->setWindowIcon(QIcon(":/resource/image/logo.png"));
this->setStyleSheet("QWidget { background-color: rgb(255,255,255); }");
this->setAttribute(Qt::WA_DeleteOnClose);
//布局管理器
QGridLayout* layout = new QGridLayout();
layout->setSpacing(0);
layout->setContentsMargins(50,0,50,0);
this->setLayout(layout);
//创建标题
QLabel* titleLabel = new QLabel();
titleLabel->setText("登录");
titleLabel->setAlignment(Qt::AlignCenter);
titleLabel->setFixedHeight(50);
titleLabel->setStyleSheet("QLabel { font-size: 40px; font-weight: 600; }");
QString editStyle = "QLineEdit { border: none; border-radius: 10px; font-size: 16px; background-color: rgb(240,240,240); padding-left: 5px; }";
//用户名输入框
QLineEdit* usernameEdit = new QLineEdit();
usernameEdit->setFixedHeight(40);
usernameEdit->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
usernameEdit->setPlaceholderText("输入用户名");
usernameEdit->setStyleSheet(editStyle);
//密码输入框
QLineEdit* passwordEdit = new QLineEdit();
passwordEdit->setFixedHeight(40);
passwordEdit->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
passwordEdit->setPlaceholderText("输入密码");
passwordEdit->setStyleSheet(editStyle);
passwordEdit->setEchoMode(QLineEdit::Password);
//验证码输入框
QLineEdit* verifyCodeEdit = new QLineEdit();
verifyCodeEdit->setFixedHeight(40);
verifyCodeEdit->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
verifyCodeEdit->setPlaceholderText("输入验证码");
verifyCodeEdit->setStyleSheet(editStyle);
//验证码图片 TODO:
QPushButton* veriCodeWidget = new QPushButton();
veriCodeWidget->setText("验证码");
veriCodeWidget->setStyleSheet("QPushButton { border: none; }");
QString btnGreenStyle = "QPushButton { border: none; border-radius: 10px; background-color: rgb(44,182,61); color: rgb(255,255,255); }";
btnGreenStyle+="QPushButton:pressed { background-color: rgb(240,240,240); }";
QString btnWhiteStyle = "QPushButton { border: none; border-radius: 10px; background-color: transparent; }";
btnWhiteStyle+="QPushButton:pressed { background-color: rgb(240,240,240); }";
//登录按钮
QPushButton* submitBtn = new QPushButton();
submitBtn->setText("登录");
submitBtn->setFixedHeight(40);
submitBtn->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
submitBtn->setStyleSheet(btnGreenStyle);
//手机号登录
QPushButton* phoneModeBtn = new QPushButton();
phoneModeBtn->setFixedSize(100,40);
phoneModeBtn->setText("手机号登录");
phoneModeBtn->setStyleSheet(btnWhiteStyle);
//注册
QPushButton* switchModeBtn = new QPushButton();
switchModeBtn->setFixedSize(100,40);
switchModeBtn->setText("注册");
switchModeBtn->setStyleSheet(btnWhiteStyle);
//添加布局管理器
layout->addWidget(titleLabel,0,0,1,7);
layout->addWidget(usernameEdit,1,0,1,7);
layout->addWidget(passwordEdit,2,0,1,7);
layout->addWidget(verifyCodeEdit,3,0,1,5);
layout->addWidget(veriCodeWidget,3,5,1,2);
layout->addWidget(submitBtn,6,1,1,5);
layout->addWidget(phoneModeBtn,4,0,1,2);
layout->addWidget(switchModeBtn,4,5,1,2);
}
PhoneLoginWidget
cpp
PhoneLoginWidget::PhoneLoginWidget(QWidget *parent)
: QWidget{parent}
{
//基本属性
this->setFixedSize(350,400);
this->setWindowTitle("登录");
this->setWindowIcon(QIcon(":/resource/image/logo.png"));
this->setStyleSheet("QWidget { background-color: rgb(255,255,255); }");
this->setAttribute(Qt::WA_DeleteOnClose);
//布局管理器
QGridLayout* layout = new QGridLayout();
layout->setSpacing(0);
layout->setContentsMargins(50,0,50,0);
this->setLayout(layout);
//标题
QLabel* titleLabel = new QLabel();
titleLabel->setText("登录");
titleLabel->setAlignment(Qt::AlignCenter);
titleLabel->setFixedHeight(50);
titleLabel->setStyleSheet("QLabel { font-size: 40px; font-weight: 600; }");
QString editStyle = "QLineEdit { border: none; border-radius: 10px; font-size: 16px; background-color: rgb(240,240,240); padding-left: 5px; }";
//电话号码输入框
QLineEdit* phoneEdit = new QLineEdit();
phoneEdit->setPlaceholderText("输入手机号");
phoneEdit->setFixedHeight(40);
phoneEdit->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
phoneEdit->setStyleSheet(editStyle);
//验证码输入框
QLineEdit* verifyCodeEdit = new QLineEdit();
verifyCodeEdit->setFixedHeight(40);
verifyCodeEdit->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
verifyCodeEdit->setPlaceholderText("输入验证码");
verifyCodeEdit->setStyleSheet(editStyle);
QString btnWhiteStyle = "QPushButton { border: none; border-radius: 10px; background-color: transparent; }";
btnWhiteStyle+="QPushButton:pressed { background-color: rgb(240,240,240); }";
QString btnGreenStyle = "QPushButton { border: none; border-radius: 10px; background-color: rgb(44,182,61); color: rgb(255,255,255); }";
btnGreenStyle+="QPushButton:pressed { background-color: rgb(240,240,240); }";
//发送验证码按钮
QPushButton* sendVerifyCodeBtn = new QPushButton();
sendVerifyCodeBtn->setFixedSize(100,40);
sendVerifyCodeBtn->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
sendVerifyCodeBtn->setText("发送验证码");
sendVerifyCodeBtn->setStyleSheet(btnWhiteStyle);
//提交按钮
QPushButton* submitBtn = new QPushButton();
submitBtn->setText("登录");
submitBtn->setFixedHeight(40);
submitBtn->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Fixed);
submitBtn->setStyleSheet(btnGreenStyle);
//创建"切换到用户名"模式按钮
QPushButton* userModeBtn = new QPushButton();
userModeBtn->setFixedSize(100,40);
userModeBtn->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
userModeBtn->setText("切换到用户名");
userModeBtn->setStyleSheet(btnWhiteStyle);
//切换登陆注册模式按钮
QPushButton* switchModeBtn = new QPushButton();
switchModeBtn->setFixedSize(100,40);
switchModeBtn->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
switchModeBtn->setText("注册");
switchModeBtn->setStyleSheet(btnWhiteStyle);
//添加到布局管理器
layout->addWidget(titleLabel,0,0,1,7);
layout->addWidget(phoneEdit,1,0,1,7);
layout->addWidget(verifyCodeEdit,2,0,1,5);
layout->addWidget(sendVerifyCodeBtn,2,5,1,2);
layout->addWidget(userModeBtn,3,0,1,2);
layout->addWidget(switchModeBtn,3,5,1,2);
layout->addWidget(submitBtn,4,1,1,5);
}
那么这一集就先到这里!