目录
931、pandas.RangeIndex.from_range类方法
933、pandas.CategoricalIndex.codes属性
934、pandas.CategoricalIndex.categories属性
935、pandas.CategoricalIndex.ordered属性

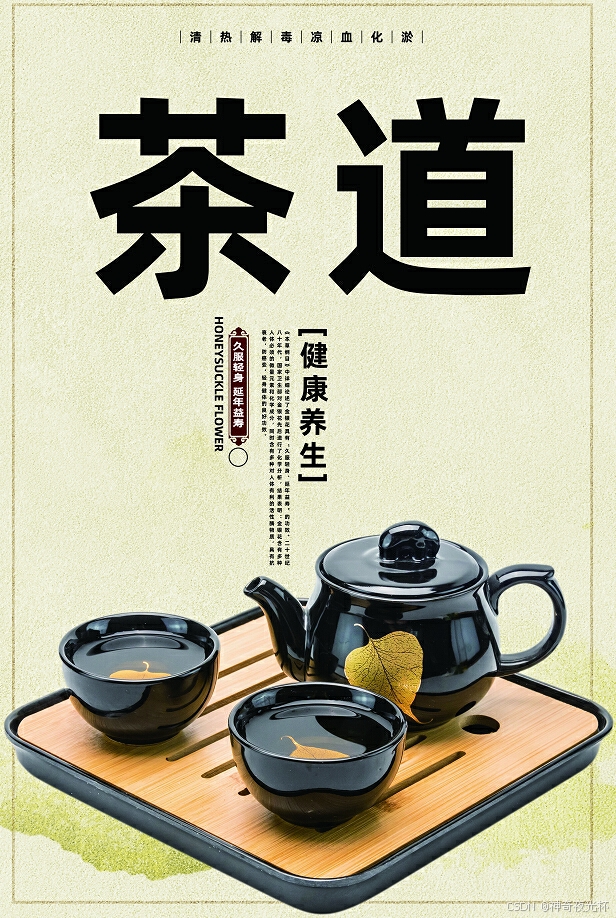

一、用法精讲
931、pandas.RangeIndex.from_range类方法
931-1、语法
python
# 931、pandas.RangeIndex.from_range类方法
classmethod pandas.RangeIndex.from_range(data, name=None, dtype=None)
Create pandas.RangeIndex from a range object.
Returns:
RangeIndex
931-2、参数
931-2-1、data**(必需)****:**range or list,表示需要用于创建RangeIndex的数据,通常是一个范围对象(如Python的range),指定了索引的起始和结束值。
931-2-2、name**(可选,默认值为None)****:**字符串,用于指定创建的索引的名称,如果没有提供,则索引将没有名称。
931-2-3、dtype**(可选,默认值为None)****:**字符串或np.dtype,指定索引的数据类型,如果设置为None,数据类型将自动推导。
931-3、功能
从给定的范围数据创建一个新的RangeIndex对象,该索引在处理大数据集时可以提高性能和内存效率,因为它使用了更紧凑的数据存储方式。
931-4、返回值
返回一个RangeIndex对象,它表示由提供的数据定义的索引,该对象可以用于数据框架或系列中,作为高效的行或列标签。
931-5、说明
无
931-6、用法
931-6-1、数据准备
python
无
931-6-2、代码示例
python
# 931、pandas.RangeIndex.from_range类方法
import pandas as pd
# 从范围创建RangeIndex
range_index = pd.RangeIndex.from_range(range(0, 10), name='my_index')
print(range_index)
931-6-3、结果输出
python
# 931、pandas.RangeIndex.from_range类方法
# RangeIndex(start=0, stop=10, step=1, name='my_index')
932、pandas.CategoricalIndex类
932-1、语法
python
# 932、pandas.CategoricalIndex类
class pandas.CategoricalIndex(data=None, categories=None, ordered=None, dtype=None, copy=False, name=None)
Index based on an underlying Categorical.
CategoricalIndex, like Categorical, can only take on a limited, and usually fixed, number of possible values (categories). Also, like Categorical, it might have an order, but numerical operations (additions, divisions, ...) are not possible.
Parameters:
data
array-like (1-dimensional)
The values of the categorical. If categories are given, values not in categories will be replaced with NaN.
categories
index-like, optional
The categories for the categorical. Items need to be unique. If the categories are not given here (and also not in dtype), they will be inferred from the data.
ordered
bool, optional
Whether or not this categorical is treated as an ordered categorical. If not given here or in dtype, the resulting categorical will be unordered.
dtype
CategoricalDtype or "category", optional
If CategoricalDtype, cannot be used together with categories or ordered.
copy
bool, default False
Make a copy of input ndarray.
name
object, optional
Name to be stored in the index.
Raises:
ValueError
If the categories do not validate.
TypeError
If an explicit ordered=True is given but no categories and the values are not sortable.
932-2、参数
932-2-1、data**(可选,默认值为None)****:**array-like,表示要转化为分类索引的数据,可以是列表、数组或其他类似结构。
932-2-2、categories**(可选,默认值为None)****:**array-like/Categorical,指定类别的唯一值,如果提供,data的值必须是这些类别之一;如果未提供,pandas将自动推断类别。
932-2-3、ordered**(可选,默认值为None)****:**布尔值,指定类别是否有顺序,当设置为True时,类别之间的排序关系得到保留;当为False时,类别是无序的。
932-2-4、dtype**(可选,默认值为None)****:**str/np.dtype/CategoricalDtype/None,指定索引的数据类型,可以选择使用具体的分类数据类型,也可以让pandas自动推导。
932-2-5、copy**(可选,默认值为False)****:**布尔值,如果设置为True, 将对输入的数据进行复制;如果为False,将尽量使用现有的数据。
932-2-6、name**(可选,默认值为None)****:**字符串,用于为索引指定名称,如果未提供,索引将没有名称。
932-3、功能
允许用户创建一个专门处理分类数据的索引,可以有效地进行数据筛选、分组和聚合操作,它的主要优势在于内存效率和性能,尤其是在类别数量相对于数据集大小时。
932-4、返回值
返回一个CategoricalIndex对象,该对象可以用于数据框架或系列,作为行或列的标签,便于进一步统计分析和数据处理。
932-5、说明
无
932-6、用法
932-6-1、数据准备
python
无
932-6-2、代码示例
python
# 932、pandas.CategoricalIndex类
import pandas as pd
# 创建包含类别索引的数据
data = ['apple', 'banana', 'apple', 'orange']
categories = ['apple', 'banana', 'orange']
cat_index = pd.CategoricalIndex(data, categories=categories, ordered=True, name='fruits')
print(cat_index)
print(cat_index.isin(['apple', 'banana']))
932-6-3、结果输出
python
# 932、pandas.CategoricalIndex类
# CategoricalIndex(['apple', 'banana', 'apple', 'orange'], categories=['apple', 'banana', 'orange'], ordered=True, dtype='category', name='fruits')
# [ True True True False]
933、pandas.CategoricalIndex.codes属性
933-1、语法
python
# 933、pandas.CategoricalIndex.codes属性
property pandas.CategoricalIndex.codes
The category codes of this categorical index.
Codes are an array of integers which are the positions of the actual values in the categories array.
There is no setter, use the other categorical methods and the normal item setter to change values in the categorical.
Returns:
ndarray[int]
A non-writable view of the codes array.
933-2、参数
无
933-3、功能
用于获取CategoricalIndex对象中每个分类值的整数编码。
933-4、返回值
返回一个NumPy数组,其中每个元素代表对应位置上分类值的编码值,便于进一步的数据分析或操作。
933-5、说明
无
933-6、用法
933-6-1、数据准备
python
无
933-6-2、代码示例
python
# 933、pandas.CategoricalIndex.codes属性
import pandas as pd
# 创建一个CategoricalIndex
categories = pd.CategoricalIndex(["apple", "banana", "apple", "orange", "banana"])
print("CategoricalIndex: ", categories)
# 使用codes获取整数编码
codes = categories.codes
print("Codes: ", codes)
933-6-3、结果输出
python
# 933、pandas.CategoricalIndex.codes属性
# CategoricalIndex: CategoricalIndex(['apple', 'banana', 'apple', 'orange', 'banana'], categories=['apple', 'banana', 'orange'], ordered=False, dtype='category')
# Codes: [0 1 0 2 1]
934、pandas.CategoricalIndex.categories属性
934-1、语法
python
# 934、pandas.CategoricalIndex.categories属性
property pandas.CategoricalIndex.categories
The categories of this categorical.
Setting assigns new values to each category (effectively a rename of each individual category).
The assigned value has to be a list-like object. All items must be unique and the number of items in the new categories must be the same as the number of items in the old categories.
Raises:
ValueError
If the new categories do not validate as categories or if the number of new categories is unequal the number of old categories
934-2、参数
无
934-3、功能
用于返回CategoricalIndex对象中所有唯一且排序的类别。
934-4、返回值
返回一个Index对象,包含了所有类别值,并按照指定或默认的顺序排列,它通常在进行类别数据的处理时用来确认类别的取值范围。
934-5、说明
无
934-6、用法
934-6-1、数据准备
python
无
934-6-2、代码示例
python
# 934、pandas.CategoricalIndex.categories属性
import pandas as pd
# 创建一个CategoricalIndex
categories = pd.CategoricalIndex(["apple", "banana", "apple", "orange", "banana"])
print("CategoricalIndex: ", categories)
# 使用categories获取类别信息
category_values = categories.categories
print("Categories: ", category_values)
934-6-3、结果输出
python
# 934、pandas.CategoricalIndex.categories属性
# CategoricalIndex: CategoricalIndex(['apple', 'banana', 'apple', 'orange', 'banana'], categories=['apple', 'banana', 'orange'], ordered=False, dtype='category')
# Categories: Index(['apple', 'banana', 'orange'], dtype='object')
935、pandas.CategoricalIndex.ordered属性
935-1、语法
python
# 935、pandas.CategoricalIndex.ordered属性
property pandas.CategoricalIndex.ordered
Whether the categories have an ordered relationship.
935-2、参数
无
935-3、功能
-
有序分类(ordered=True):当设置为True时,表示该分类数据是有序的。也就是说,类别之间存在一种可比较的顺序关系,在许多分析任务中非常重要,尤其是当类别具有自然的顺序时,比如评分(如"低"、"中"、"高")或等级(如"新手"、"中级"、"高级")。
-
无序分类(ordered=False):当设置为False时,表示该分类数据是无序的。类别之间没有定义的顺序关系,因此在比较这些类别时没有逻辑意义,适用于例如颜色、城市名等没有内在顺序的分类数据。
935-4、返回值
返回一个布尔值,如果ordered=True,则会返回True;否则返回False。
935-5、说明
无
935-6、用法
935-6-1、数据准备
python
无
935-6-2、代码示例
python
# 935、pandas.CategoricalIndex.ordered属性
import pandas as pd
# 创建一个有序的CategoricalIndex
ordered_index = pd.CategoricalIndex(['低', '中', '高'], ordered=True)
print("Ordered:", ordered_index.ordered)
# 创建一个无序的CategoricalIndex
unordered_index = pd.CategoricalIndex(['红', '绿', '蓝'], ordered=False)
print("Ordered:", unordered_index.ordered)
935-6-3、结果输出
python
# 935、pandas.CategoricalIndex.ordered属性
# Ordered: True
# Ordered: False