枚举
概念:将一组常量组织起来,在这之前表示一组常量通常使用定义常量的方式。
java
public static final int RED = 1;
public static final int GREEN = 2;
public static final int BLACK = 3;
枚举的使用
java
public enum enumTest {
RED(0,"RED"),GREEN(1,"GREEN"),BLUE(2,"BLUE"),
BLACK(3,"BLACK"),WHITE(4,"WHITE");
public int ordinal;
public String color;
enumTest(int ordinal, String color) {
this.ordinal = ordinal;
this.color = color;
}
public static void main1(String[] args) {
enumTest enumTest=RED;
switch (enumTest){
case RED -> {
System.out.println("RED");
break;
}
case BLACK -> {
System.out.println("BLACK");
break;
}
case BLUE -> {
System.out.println("BLUE");
break;
}
case WHITE -> {
System.out.println("WHITE");
break;
}
default -> {
System.out.println("无法匹配");
break;
}
}
}
}
枚举的常用方法
|-------------|------------------|
| values() | 以数组形式返回枚举类型的所有成员 |
| ordinal() | 获取枚举成员的索引位置 |
| valueOf() | 将普通字符串转换为枚举实例 |
| compareTo() | 比较两个枚举成员在定义时的顺序 |
java
public static void main(String[] args) {
TestEnum[] testEnums = TestEnum.values();
for (int i = 0; i < testEnums.length; i++) {
System.out.println(testEnums[i] +" " +testEnums[i].ordinal());
}
TestEnum testEnum = TestEnum.valueOf("WHITE");
System.out.println(testEnum);
System.out.println(WHITE.compareTo(BLACK));
}
枚举通过反射来拿到实例的对象
java
public class Test1 {
public static void main(String[] args) {
try {
Class<?> c1 = Class.forName("enumdemo.enumTest");
Constructor<?> constructor = c1.getDeclaredConstructor
(String.class, int.class, int.class, String.class);
constructor.setAccessible(true);
enumTest enumTest = (enumTest) constructor.newInstance(9, "YELLOW");
System.out.println(enumTest);
} catch (ClassNotFoundException e) {
throw new RuntimeException(e);
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
} catch (InvocationTargetException e) {
throw new RuntimeException(e);
} catch (InstantiationException e) {
throw new RuntimeException(e);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
}
}
}
注:枚举本身就是一个类,其构造方法默认为私有的,枚举可以避免反射和序列化问题。
希望能对大家有所帮助!!!
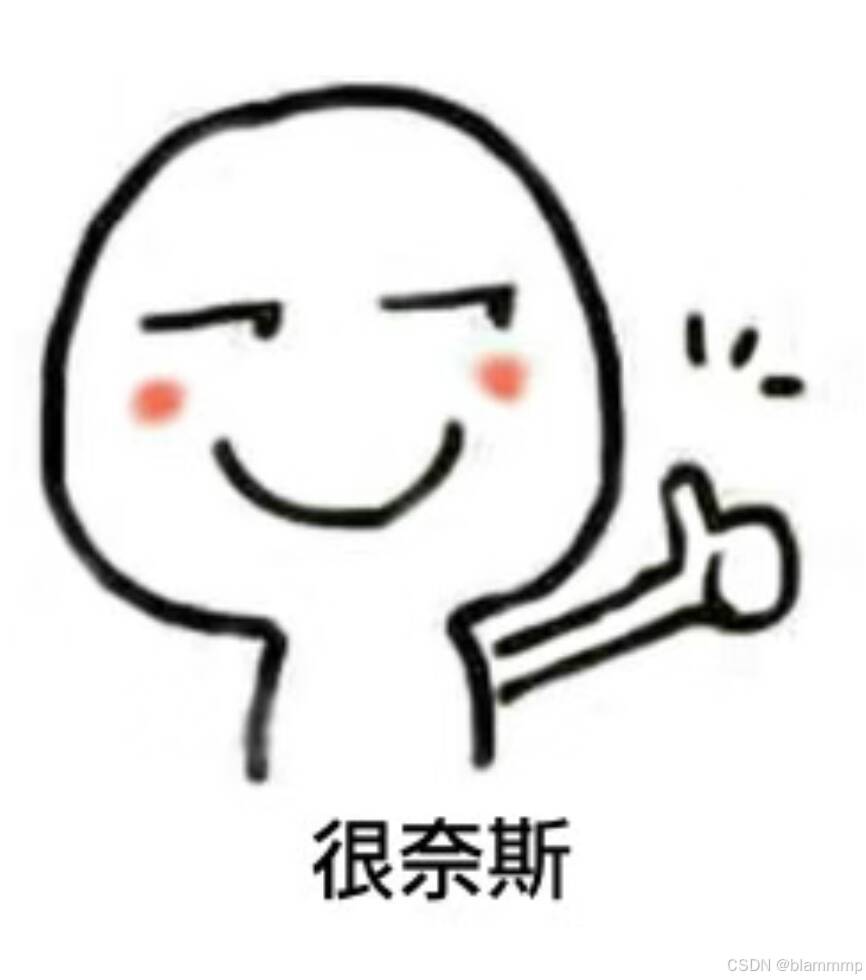