在 Bash 中获取 Python 模块的变量列表可以通过使用 python -c
来运行 Python 代码并输出变量名列表。
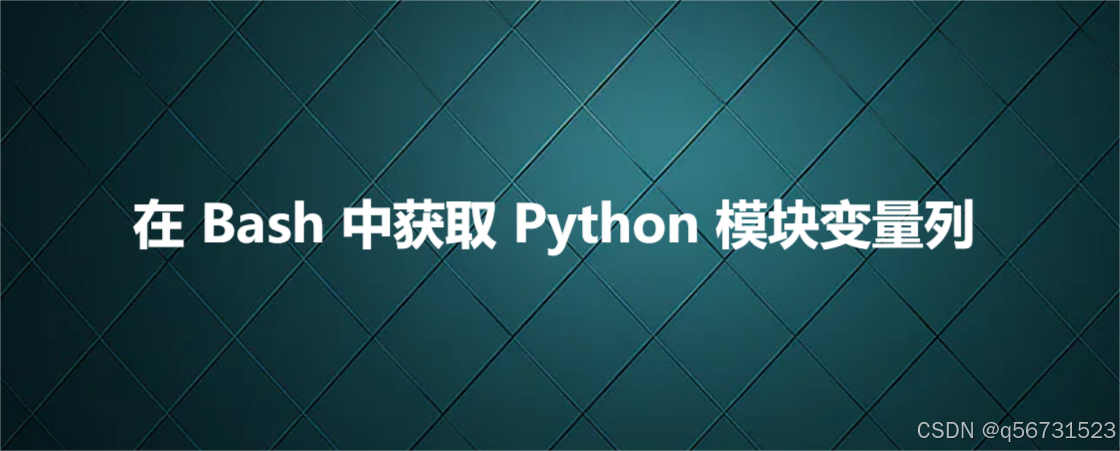
1、问题背景
在编写 Bash 补全脚本时,需要获取已安装 Python 模块中与模式匹配的所有变量。为了避免解析注释等内容,希望仅使用 Python 相关功能。
2、解决方案
方法一:使用 Python -c 执行单行 Python 脚本
如果只想执行单行 Python 脚本,可以使用 python -c 命令。例如:
bash
python -c "import os; print dir(os)"
输出结果为:
['DirEntry', 'F_OK', 'MutableMapping', 'O_APPEND', 'O_CREAT', 'O_EXCL', 'O_RDONLY', 'O_RDWR', 'O_TRUNC', 'O_WRONLY', 'P_NOWAIT', 'P_NOWAITO', 'P_WAIT', 'POSIX_FADV_DONTNEED', 'POSIX_FADV_NOREUSE', 'POSIX_FADV_NORMAL', 'POSIX_FADV_RANDOM', 'POSIX_FADV_SEQUENTIAL', 'POSIX_FADV_WILLNEED', 'R_OK', 'S_IEXEC', 'S_IFBLK', 'S_IFCHR', 'S_IFDIR', 'S_IFIFO', 'S_IFLNK', 'S_IFREG', 'S_IFMT', 'S_IMODE', 'S_ISBLK', 'S_ISCHR', 'S_ISDIR', 'S_ISFIFO', 'S_ISLNK', 'S_ISREG', 'S_ISUID', 'S_ISVTX', 'ST_APPEND', 'ST_MANDLOCK', 'ST_NOATIME', 'ST_NODEV', 'ST_NODIRATIME', 'ST_NOSUID', 'ST_RDONLY', 'ST_RELATIME', 'ST_SYNCHRONOUS', 'ST_SIZE', 'W_OK', 'X_OK', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'abc', 'access', 'altsep', 'argv', 'basestring', 'bool', 'bytearray', 'bytes', 'callable', 'choices', 'classdict', 'classmethod', 'clearcache', 'close', 'coerce_argspec', 'compile', 'complex', 'copyright', 'credits', 'dd', 'deque', 'device_encoding', 'dir', 'displayhook', 'divmod', 'encode', 'enumerate', 'environ', 'EOFError', 'error', 'execfile', 'execv', 'execve', 'exit', 'expandtabs', 'expandvars', 'False', 'fdopen', 'file', 'filter', 'float', 'floordiv', 'flush', 'format', 'freevars', 'fspath', 'fstat', 'fstypename', 'ftruncate', 'func_code', 'func_defaults', 'func_dict', 'func_doc', 'func_globals', 'func_name', 'func_newparty', 'func_newparty', 'func_self', 'functools', 'gc', 'get_doc', 'get_file_type', 'getcwd', 'getdefaultencoding', 'getfilesystemencoding', 'getloadavg', 'globals', 'hasattr', 'hash', 'help', 'hex', 'id', 'import', 'imputil', 'index', 'input', 'int', 'isinstance', 'issubclass', 'iter', 'iterafter', 'iterator', 'join', 'key', 'kwonlyargcount', 'len', 'listdir', 'locals', 'long', 'lookup', 'lseek', 'mac_ver', 'makefuncs', 'manpath', 'makedirs', 'map', 'max', 'maxsize', 'memoryview', 'min', 'mkfifo', 'mkstemp', 'mktime', 'mmap', 'mode', 'module', 'modulefinder', 'msilib', 'multimethods', 'name', 'nbits', 'nbytes', 'new_class', 'next', 'nonlocal', 'not', 'Null', 'numbers', 'object', 'oct', 'open', 'ord', 'os2emxpath', 'oserror', 'ospath', 'pdir', 'pipe', 'popen', 'pop', 'popen2', 'pow', 'preexec_fn', 'print', 'property', 'proxy_new', 'putenv', 'quote', 'quote_from_bytes', 'raise', 'randbytes', 'range', 'raw_input', 'read', 'readlink', 'readlines', 'readline', 're', 'realloc', 'reduce', 'reduce_ex', 'refcount', 'reload', 'repr', 'reset', 'rest', 'reversed', 'rmdir', 'round', 'seek', 'send', 'sep', 'setattr', 'setenv', 'setrecursionlimit', 'singledispatch', 'size', 'slice', 'sorted', 'spawnl', 'spawnle', 'spawnlp', 'spawnlpe', 'spawnv', 'spawnve', 'spawnvp', 'spawnvpe', 'split', 'splitlines', 'sqrt', 'ssencode', 'stderr', 'stdin', 'stdout', 'str', 'strict_typehint', 'StringIO', 'string', 'subclasses', 'super', 'symlink', 'sys', 'sysconfig', 'sysflags', 'sysgetcheckinterval', 'sysgetdefaultencoding', 'sysgetfilesystemencoding', 'sysgetrefcount', 'syslog', 'tarfile', 'tempfile', 'this', 'time', 'times', 'tmpfile', 'tokenize', 'trace', 'truncate', 'try', 'ttyname', 'tuple', 'type', 'typevars', 'unichr', 'unicode', 'uniform', 'unlink', 'unparse', 'unshelve', 'update', 'utime', 'va_arg', 'va_copy', 'va_end', 'va_list', 'va_start', 'vars', 'wait', 'waitpid', 'walk', 'warn', 'warnings', 'where', 'write', 'xreadlines', 'zip']
方法二:使用 Python -c 过滤变量
如果想根据模式过滤变量,可以使用以下命令:
bash
python -c "import os; print [x for x in dir(os) if x.startswith('r')]"
输出结果为:
['read', 'readlink', 'remove', 'removedirs', 'rename', 'renames', 'rmdir']
设你有一个 Python 模块(文件)mymodule.py
,内容如下:
# mymodule.py
x = 10
y = 20
z = 30
def my_function():
pass
要在 Bash 中获取该模块中的所有变量(即非函数、非内置的全局变量),可以使用以下步骤:
方法:使用 dir()
函数结合过滤
- 使用
python -c
运行 Python 脚本。 - 使用
dir()
获取模块中的所有名称。 - 使用
inspect
模块过滤出变量(排除函数、类、模块等)。
示例代码
python -c "
import mymodule
import inspect
variables = [name for name, value in vars(mymodule).items() if not inspect.isbuiltin(value) and not inspect.isfunction(value) and not inspect.ismodule(value) and not inspect.isclass(value)]
print(' '.join(variables))
"
说明
vars(mymodule).items()
:获取模块的所有属性。inspect.isbuiltin
、inspect.isfunction
、inspect.ismodule
、inspect.isclass
:过滤掉内置对象、函数、模块和类,保留纯变量。print(' '.join(variables))
:将变量名列表以空格分隔的形式打印出来。
执行结果
在执行上述命令后,输出会是:
x y z
这表示 mymodule
中的三个变量 x
、y
、z
。
扩展
如果需要进一步处理输出内容,可以在 Bash 中将其保存为数组:
variables=($(python -c "
import mymodule
import inspect
variables = [name for name, value in vars(mymodule).items() if not inspect.isbuiltin(value) and not inspect.isfunction(value) and not inspect.ismodule(value) and not inspect.isclass(value)]
print(' '.join(variables))
"))
这样,variables
数组就包含了所有变量名,你可以在 Bash 中进一步使用。