之前我们写过的链表基本都是不带头节点的,但是有些算法题,它就要给你来一个带头节点的玩意,我们应该如何写呢?
不带头节点的用的比较多,这里我只写头插和尾插。
那么,我们要带头节点,首先我们就要开辟一个节点出来当头节点,也就是初始化。
代码如下:
c
STnode* initlist()
{
STnode* newnode = (STnode*)malloc(sizeof(STnode));
if (newnode == NULL)
{
printf("error\n");
exit(-1);
}
newnode->next = NULL;
return newnode;
}
初始化之后我们就是完成尾插,头插,打印函数
创建新节点函数:
c
STnode* buynewnode(int val)
{
STnode* newnode = (STnode*)malloc(sizeof(STnode));
if (newnode == NULL)
{
printf("error\n");
exit(-1);
}
newnode->data = val;
newnode->next = NULL;
return newnode;
}
尾插函数:
c
void pushback(STnode* phead,int val) {
STnode* newnode=buynewnode(val);
STnode* tail = phead;
while (tail->next!= NULL)
{
tail = tail->next;
}
tail->next = newnode;
}
头插函数;
c
void pushfront(STnode* phead, int val) {
STnode* newnode = buynewnode(val);
if (phead->next == NULL)
{
pushback(phead, val);
}
else {
STnode* temp = phead->next;
phead->next = newnode;
newnode->next = temp;
}
}
打印函数:
c
void Print(STnode* phead)
{
STnode* tail = phead->next;
while (tail != NULL)
{
printf("%d->", tail->data);
tail = tail->next;
}
printf("NULL\n");
}
测试一下:
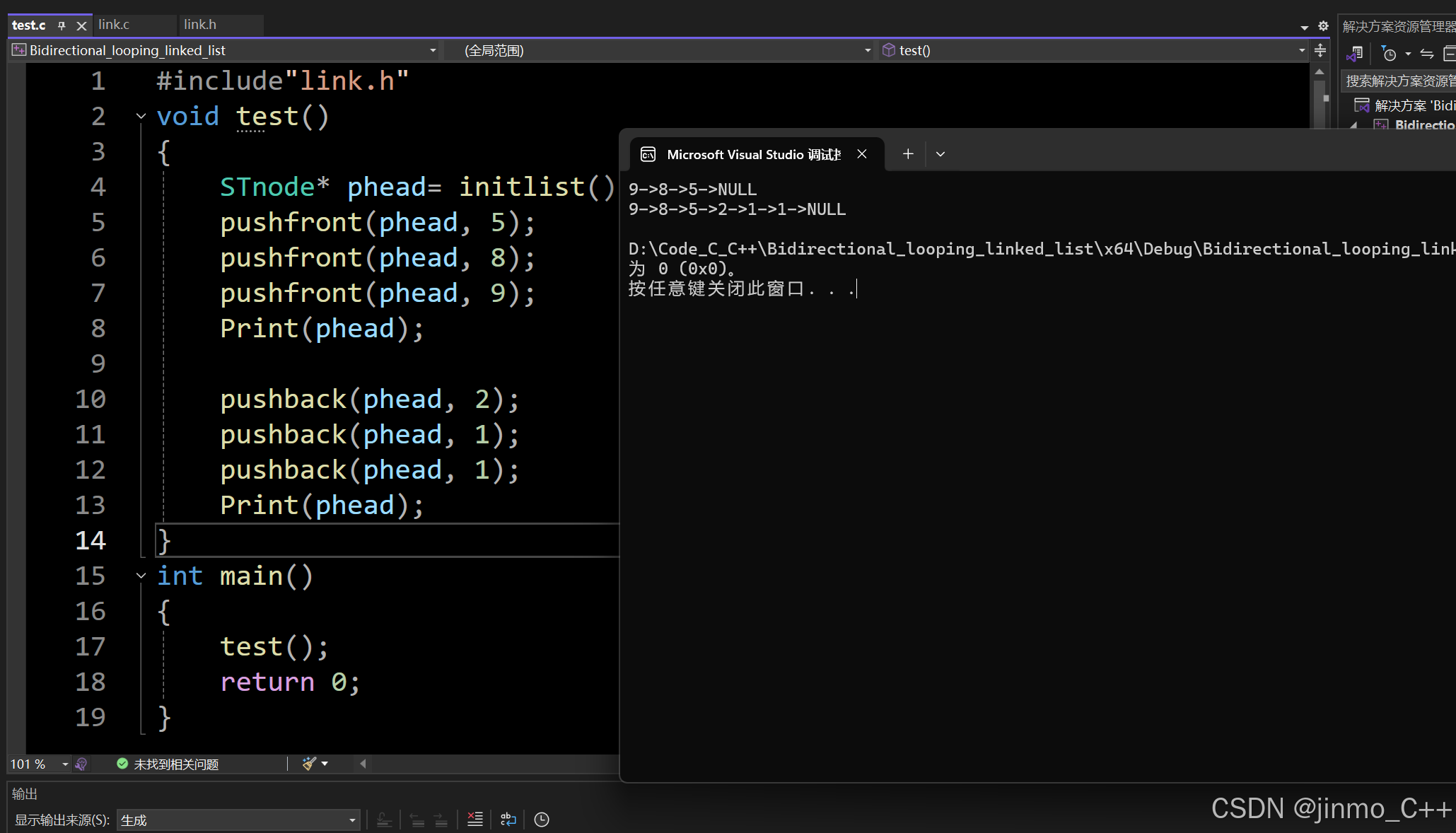
完成啦!!
头文件:
c
#pragma once
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
typedef struct link {
int data;
struct link* next;
}STnode;
void Print(STnode* phead);
STnode* buynewnode(int val);
void pushback(STnode* phead,int val);
void pushfront(STnode* phead,int val);
STnode* initlist();
函数
c
#include"link.h"
STnode* initlist()
{
STnode* newnode = (STnode*)malloc(sizeof(STnode));
if (newnode == NULL)
{
printf("error\n");
exit(-1);
}
newnode->next = NULL;
return newnode;
}
STnode* buynewnode(int val)
{
STnode* newnode = (STnode*)malloc(sizeof(STnode));
if (newnode == NULL)
{
printf("error\n");
exit(-1);
}
newnode->data = val;
newnode->next = NULL;
return newnode;
}
void pushback(STnode* phead,int val) {
STnode* newnode=buynewnode(val);
STnode* tail = phead;
while (tail->next!= NULL)
{
tail = tail->next;
}
tail->next = newnode;
}
void pushfront(STnode* phead, int val) {
STnode* newnode = buynewnode(val);
if (phead->next == NULL)
{
pushback(phead, val);
}
else {
STnode* temp = phead->next;
phead->next = newnode;
newnode->next = temp;
}
}
void Print(STnode* phead)
{
STnode* tail = phead->next;
while (tail != NULL)
{
printf("%d->", tail->data);
tail = tail->next;
}
printf("NULL\n");
}
测试:
c
#include"link.h"
void test()
{
STnode* phead= initlist();
pushfront(phead, 5);
pushfront(phead, 8);
pushfront(phead, 9);
Print(phead);
pushback(phead, 2);
pushback(phead, 1);
pushback(phead, 1);
Print(phead);
}
int main()
{
test();
return 0;
}