目录
1021、pandas.DatetimeIndex.inferred_freq属性
1022、pandas.DatetimeIndex.indexer_at_time方法
1023、pandas.DatetimeIndex.indexer_between_time方法
1024、pandas.DatetimeIndex.normalize方法
1025、pandas.DatetimeIndex.strftime方法

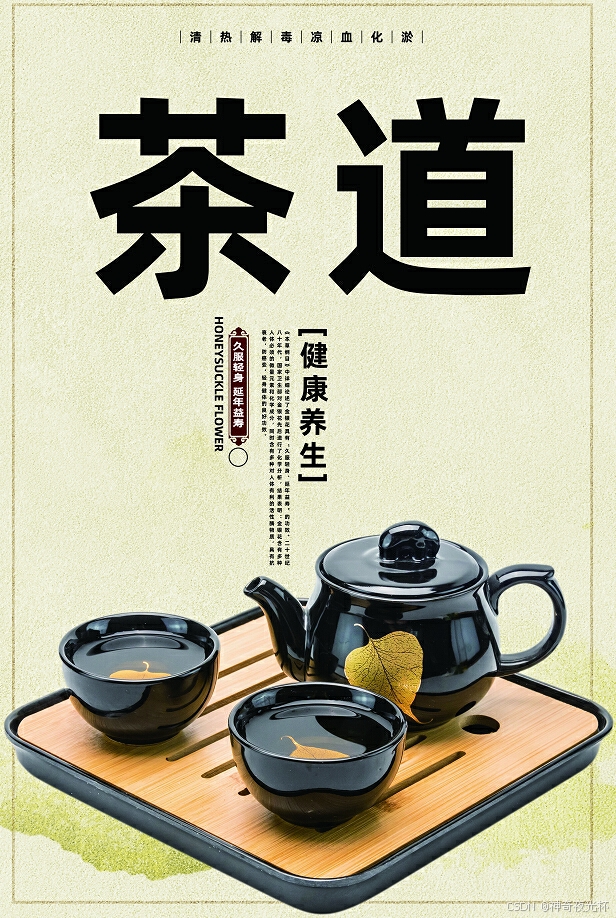

一、用法精讲
1021、pandas.DatetimeIndex.inferred_freq属性
1021-1、语法
python
# 1021、pandas.DatetimeIndex.inferred_freq属性
pandas.DatetimeIndex.inferred_freq
Tries to return a string representing a frequency generated by infer_freq.
Returns None if it can't autodetect the frequency.
1021-2、参数
无
1021-3、功能
用于获取DatetimeIndex对象的推断频率,它可以帮助用户了解时间序列数据的频率模式,在进行时间序列分析时非常重要。
1021-4、返回值
返回一个字符串,表示DatetimeIndex中日期时间的推断频率,如果无法推断出明确的频率,则返回None,推断频率可以是以下几种类型,例如:
- **'D':**日频
- **'
h
':**小时频 - **'min':**分钟频
- **'s':**秒频
- **'
ME
':**月末频 - **'
YE
':**年末频
1021-5、说明
无
1021-6、用法
1021-6-1、数据准备
python
无
1021-6-2、代码示例
python
# 1021、pandas.DatetimeIndex.inferred_freq属性
import pandas as pd
# 创建一个包含日期的DatetimeIndex
dates = pd.date_range(start='2024-11-14', periods=5, freq='YE')
datetime_index = pd.DatetimeIndex(dates)
# 获取推断的频率
frequency = datetime_index.inferred_freq
# 输出结果
print(frequency)
1021-6-3、结果输出
python
# 1021、pandas.DatetimeIndex.inferred_freq属性
# YE-DEC
1022、pandas.DatetimeIndex.indexer_at_time方法
1022-1、语法
python
# 1022、pandas.DatetimeIndex.indexer_at_time方法
pandas.DatetimeIndex.indexer_at_time(time, asof=False)
Return index locations of values at particular time of day.
Parameters:
time
datetime.time or str
Time passed in either as object (datetime.time) or as string in appropriate format ("%H:%M", "%H%M", "%I:%M%p", "%I%M%p", "%H:%M:%S", "%H%M%S", "%I:%M:%S%p", "%I%M%S%p").
Returns:
np.ndarray[np.intp]
1022-2、参数
1022-2-1、time**(必需)****:**字符串或datetime.time对象,表示需要匹配的时间,格式通常是'HH:MM'。
1022-2-2、asof**(可选,默认值为False)****:**布尔值,如果设置为True,该方法将返回所提供时间之前的最近索引,而不是所有匹配的索引。
1022-3、功能
用于查找特定时间在DatetimeIndex中的索引位置,该方法允许你根据给定的时间字符串或时间对象,获取所有匹配的索引,其参数asof还可以进一步定义返回的行为。
1022-4、返回值
返回一个整数数组,表示所有匹配或最近匹配的索引位置,如果没有匹配项,则返回一个空数组。
1022-5、说明
无
1022-6、用法
1022-6-1、数据准备
python
无
1022-6-2、代码示例
python
# 1022、pandas.DatetimeIndex.indexer_at_time方法
import pandas as pd
# 创建一个日期范围
date_rng = pd.date_range(start='2024-11-14', end='2024-11-18', freq='h')
datetime_index = pd.DatetimeIndex(date_rng)
# 查找特定时间 (12:00)
indexer_all = datetime_index.indexer_at_time('12:00')
print("All indices for time 12:00:", indexer_all)
print("Corresponding dates:", datetime_index[indexer_all])
1022-6-3、结果输出
python
# 1022、pandas.DatetimeIndex.indexer_at_time方法
# All indices for time 12:00: [12 36 60 84]
# Corresponding dates: DatetimeIndex(['2024-11-14 12:00:00', '2024-11-15 12:00:00',
# '2024-11-16 12:00:00', '2024-11-17 12:00:00'],
# dtype='datetime64[ns]', freq=None)
1023、pandas.DatetimeIndex.indexer_between_time方法
1023-1、语法
python
# 1023、pandas.DatetimeIndex.indexer_between_time方法
pandas.DatetimeIndex.indexer_between_time(start_time, end_time, include_start=True, include_end=True)
Return index locations of values between particular times of day.
Parameters:
start_time, end_time
datetime.time, str
Time passed either as object (datetime.time) or as string in appropriate format ("%H:%M", "%H%M", "%I:%M%p", "%I%M%p", "%H:%M:%S", "%H%M%S", "%I:%M:%S%p","%I%M%S%p").
include_start
bool, default True
include_end
bool, default True
Returns:
np.ndarray[np.intp]
1023-2、参数
1023-2-1、start_time**(必需)****:**字符串或datetime.time对象,表示时间范围的起始时间。
1023-2-2、end_time**(必需)****:**字符串或datetime.time对象,表示时间范围的结束时间。
1023-2-3、include_start**(可选,默认值为True)****:**布尔值,如果为True,则包含起始时间的索引。
1023-2-4、include_end**(可选,默认值为True)****:**布尔值,如果为True,则包含结束时间的索引。
1023-3、功能
用于查找在指定时间范围内的索引位置,该方法非常适合处理时间序列数据,尤其是在你需要筛选特定时间段的数据时。
1023-4、返回值
返回一个整数数组,表示在指定时间范围内的所有匹配索引位置,如果没有匹配项,则返回一个空数组。
1023-5、说明
无
1023-6、用法
1023-6-1、数据准备
python
无
1023-6-2、代码示例
python
# 1023、pandas.DatetimeIndex.indexer_between_time方法
import pandas as pd
# 创建一个日期范围
date_rng = pd.date_range(start='2024-11-14', end='2024-11-17', freq='h')
datetime_index = pd.DatetimeIndex(date_rng)
# 查找在特定时间范围内的索引 (例如 10:00 到 12:00)
indexer = datetime_index.indexer_between_time('10:00', '12:00')
print("Indices between 10:00 and 12:00:", indexer)
print("Corresponding dates:", datetime_index[indexer])
# 查找不包含起始时间的索引
indexer_exclude_start = datetime_index.indexer_between_time('10:00', '12:00', include_start=False)
print("Indices between 10:00 and 12:00 (excluding start):", indexer_exclude_start)
print("Corresponding dates:", datetime_index[indexer_exclude_start])
1023-6-3、结果输出
python
# 1023、pandas.DatetimeIndex.indexer_between_time方法
# Indices between 10:00 and 12:00: [10 11 12 34 35 36 58 59 60]
# Corresponding dates: DatetimeIndex(['2024-11-14 10:00:00', '2024-11-14 11:00:00',
# '2024-11-14 12:00:00', '2024-11-15 10:00:00',
# '2024-11-15 11:00:00', '2024-11-15 12:00:00',
# '2024-11-16 10:00:00', '2024-11-16 11:00:00',
# '2024-11-16 12:00:00'],
# dtype='datetime64[ns]', freq=None)
# Indices between 10:00 and 12:00 (excluding start): [11 12 35 36 59 60]
# Corresponding dates: DatetimeIndex(['2024-11-14 11:00:00', '2024-11-14 12:00:00',
# '2024-11-15 11:00:00', '2024-11-15 12:00:00',
# '2024-11-16 11:00:00', '2024-11-16 12:00:00'],
# dtype='datetime64[ns]', freq=None)
1024、pandas.DatetimeIndex.normalize方法
1024-1、语法
python
# 1024、pandas.DatetimeIndex.normalize方法
pandas.DatetimeIndex.normalize(*args, **kwargs)
Convert times to midnight.
The time component of the date-time is converted to midnight i.e. 00:00:00. This is useful in cases, when the time does not matter. Length is unaltered. The timezones are unaffected.
This method is available on Series with datetime values under the .dt accessor, and directly on Datetime Array/Index.
Returns:
DatetimeArray, DatetimeIndex or Series
The same type as the original data. Series will have the same name and index. DatetimeIndex will have the same name.
1024-2、参数
1024-2-1、*args**(可选)****:**其他位置参数,为后续扩展功能做预留。
1024-2-2、**kwargs**(可选)****:**其他关键字参数,为后续扩展功能做预留。
1024-3、功能
用于将DatetimeIndex中的所有时间戳调整为相同的日期部分,具体来说就是将时间部分归零,对于比较或对齐时间序列数据非常有用。
1024-4、返回值
返回一个新的DatetimeIndex,其中所有的时间部分都被设置为00:00:00(即午夜)。
1024-5、说明
无
1024-6、用法
1024-6-1、数据准备
python
无
1024-6-2、代码示例
python
# 1024、pandas.DatetimeIndex.normalize方法
import pandas as pd
# 创建一个包含多个日期时间的DatetimeIndex
dates = pd.to_datetime(['2024-11-14 10:30:00', '2024-11-15 12:45:00', '2024-11-16 15:00:00'])
datetime_index = pd.DatetimeIndex(dates)
# 归一化DatetimeIndex
normalized_index = datetime_index.normalize()
print("原始DatetimeIndex:")
print(datetime_index)
print("\n归一化后的DatetimeIndex:")
print(normalized_index)
1024-6-3、结果输出
python
# 1024、pandas.DatetimeIndex.normalize方法
# 原始DatetimeIndex:
# DatetimeIndex(['2024-11-14 10:30:00', '2024-11-15 12:45:00',
# '2024-11-16 15:00:00'],
# dtype='datetime64[ns]', freq=None)
#
# 归一化后的DatetimeIndex:
# DatetimeIndex(['2024-11-14', '2024-11-15', '2024-11-16'], dtype='datetime64[ns]', freq='D')
1025、pandas.DatetimeIndex.strftime方法
1025-1、语法
python
# 1025、pandas.DatetimeIndex.strftime方法
pandas.DatetimeIndex.strftime(date_format)
Convert to Index using specified date_format.
Return an Index of formatted strings specified by date_format, which supports the same string format as the python standard library. Details of the string format can be found in python string format doc.
Formats supported by the C strftime API but not by the python string format doc (such as "%R", "%r") are not officially supported and should be preferably replaced with their supported equivalents (such as "%H:%M", "%I:%M:%S %p").
Note that PeriodIndex support additional directives, detailed in Period.strftime.
Parameters:
date_format
str
Date format string (e.g. "%Y-%m-%d").
Returns:
ndarray[object]
NumPy ndarray of formatted strings.
1025-2、参数
1025-2-1、date_format**(必需)****:**一个字符串,表示日期和时间的格式,与Python的strftime方法一致,您可以使用各种格式代码来指定要显示的日期和时间信息。
1025-3、功能
用于将DatetimeIndex中的日期时间对象格式化为指定的字符串格式,该方法通常用于将时间戳转换为更易读的字符串格式,以便于展示或记录。
1025-4、返回值
返回一个包含格式化字符串的NumPy数组(numpy.ndarray),每个元素对应于DatetimeIndex中的相应时间戳。
1025-5、说明
无
1025-6、用法
1025-6-1、数据准备
python
无
1025-6-2、代码示例
python
# 1025、pandas.DatetimeIndex.strftime方法
import pandas as pd
# 创建一个包含多个日期时间的DatetimeIndex
dates = pd.to_datetime(['2024-11-14 10:30:00', '2024-11-15 12:45:00', '2024-11-16 15:00:00'])
datetime_index = pd.DatetimeIndex(dates)
# 使用strftime格式化日期和时间
formatted_dates = datetime_index.strftime('%Y-%m-%d %H:%M:%S')
print("原始DatetimeIndex:")
print(datetime_index)
print("\n格式化后的字符串:")
print(formatted_dates)
1025-6-3、结果输出
python
# 1025、pandas.DatetimeIndex.strftime方法
# 原始DatetimeIndex:
# DatetimeIndex(['2024-11-14 10:30:00', '2024-11-15 12:45:00',
# '2024-11-16 15:00:00'],
# dtype='datetime64[ns]', freq=None)
#
# 格式化后的字符串:
# Index(['2024-11-14 10:30:00', '2024-11-15 12:45:00', '2024-11-16 15:00:00'], dtype='object')