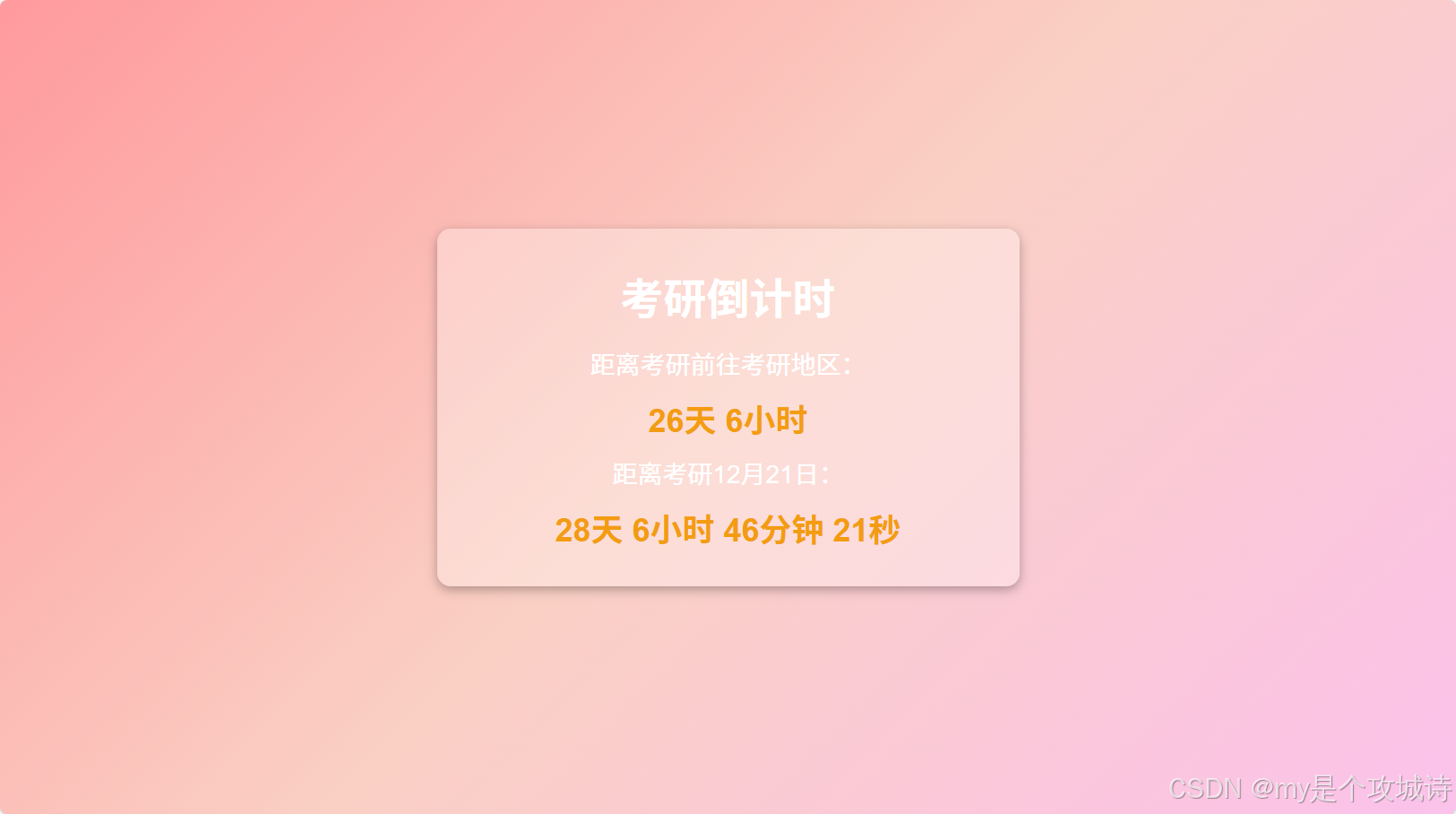
html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>考研倒计时</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Arial', sans-serif;
background: linear-gradient(135deg, #ff9a9e, #fad0c4, #fbc2eb); /* 更柔和的渐变色 */
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
color: #fff;
overflow: hidden;
}
.container {
text-align: center;
background: rgba(255, 255, 255, 0.3); /* 半透明背景 */
padding: 40px 50px; /* 增加内边距 */
border-radius: 15px;
box-shadow: 0 4px 12px rgba(0, 0, 0, 0.3);
width: 100%;
max-width: 650px; /* 增加最大宽度 */
}
h1 {
font-size: 48px; /* 增大标题字体 */
margin-bottom: 20px;
color: #fff;
font-weight: bold;
}
p {
font-size: 28px; /* 增大文本字体 */
margin: 15px 0;
color: #fff;
white-space: nowrap; /* 防止换行 */
}
span {
font-weight: bold;
font-size: 36px; /* 增大倒计时字体 */
color: #f39c12;
}
/* 动画效果 */
.countdown p {
animation: fadeIn 1s ease-out;
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
</style>
</head>
<body>
<div class="container">
<div class="countdown">
<h1>考研倒计时</h1>
<p>距离考研前往考研地区:</p>
<span id="countdown1"></span>
<p>距离考研12月21日:</p>
<span id="countdown2"></span>
</div>
</div>
<script>
// 禁用右键菜单
document.addEventListener('contextmenu', function(event) {
event.preventDefault(); // 禁用右键菜单
});
// 禁用左键点击
document.addEventListener('mousedown', function(event) {
if (event.button === 0) { // 0 表示左键
event.preventDefault(); // 禁用左键点击
}
});
// 设置考研倒计时日期
const examDepartDate = new Date("2024-12-19T00:00:00");
const examStartDate = new Date("2024-12-21T00:00:00");
// 更新倒计时函数
function updateCountdown() {
const now = new Date();
// 计算出发日期倒计时
const time1 = examDepartDate - now;
const days1 = Math.floor(time1 / (1000 * 60 * 60 * 24));
const hours1 = Math.floor((time1 % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes1 = Math.floor((time1 % (1000 * 60 * 60)) / (1000 * 60));
const seconds1 = Math.floor((time1 % (1000 * 60)) / 1000);
// 计算考试日期倒计时
const time2 = examStartDate - now;
const days2 = Math.floor(time2 / (1000 * 60 * 60 * 24));
const hours2 = Math.floor((time2 % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes2 = Math.floor((time2 % (1000 * 60 * 60)) / (1000 * 60));
const seconds2 = Math.floor((time2 % (1000 * 60)) / 1000);
// 显示倒计时
document.getElementById("countdown1").innerHTML = `${days1}天 ${hours1}小时 `;
document.getElementById("countdown2").innerHTML = `${days2}天 ${hours2}小时 ${minutes2}分钟 ${seconds2}秒 `;
// 如果倒计时结束
if (time1 < 0) {
document.getElementById("countdown1").innerHTML = "已出发";
}
if (time2 < 0) {
document.getElementById("countdown2").innerHTML = "考试开始";
}
}
// 每秒更新一次倒计时
setInterval(updateCountdown, 1000);
</script>
</body>
</html>