一、常见的SpringMVC注解有哪些?
1.@Controller:用于声明一个类为 Spring MVC 控制器。
2.@RequestMapping:用于将 HTTP 请求映射到特定的处理方法上。可以指定请求类型(GET、POST等)和URL路径。
3.@GetMapping:用于处理 GET 请求的简化版@RequestMapping。
4.@PostMapping:用于处理 POST 请求的简化版@RequestMapping。
5.@PutMapping:用于处理 PUT 请求。
6.@DeleteMapping:用于处理 DELETE 请求。
7.@PatchMapping:用于处理 PATCH 请求。
8.@PathVariable:用于将 URL 中的占位符参数绑定到控制器处理方法的参数上。
9.@RequestParam:用于将请求参数区绑定到控制器处理方法的参数上。
10.@RequestBody:用于将 HTTP 请求正文绑定到控制器处理方法的参数上,通常用于处理 POST 请求。
11.@ResponseBody:用于将控制器处理方法的返回值作为 HTTP 响应正文返回,通常用于处理 GET 请求。
12.@RestController:是@Controller和@ResponseBody的组合注解,用于声明一个类为 Spring MVC 控制器,并自动将返回值作为 HTTP 响应正文。
13.@ExceptionHandler:用于声明异常处理方法,可以捕获并处理控制器中抛出的异常。
14.@ResponseStatus:用于设置 HTTP 响应状态码。
15.@RequestHeader:用于将请求头信息绑定到控制器处理方法的参数上。
16.@CookieValue:用于将 Cookie 信息绑定到控制器处理方法的参数上。
17.@RequestMapping#params:用于指定请求参数条件,只有当这些参数条件满足时,请求才会被映射到对应的处理方法。
18.@RequestMapping#headers:用于指定请求头条件,只有当这些请求头条件满足时,请求才会被映射到对应的处理方法。
二、在小Demo中来体会这些注解
Attention:
本次小Demo会涉及Vue2.0和Element-UI 前端框架和UI框架,同时会涉及fetch进行Post和Get请求!
Question:
开发一个客户及订单管理系统,需要实现添加客户信息 (客户名称、客户单位、客户职位、客户生日、客户性别、客户联系方式、客户照片),展现客户信息 ,修改客户信息 ,删除客户信息 ,查询客户信息 (模糊查询、精准查询),添加客户订单 (客户id、订单编号、订单金额、下单时间),展现用户订单列表(能够根据金额和下单时间进行排序)。
三、直接上代码
目录结构:
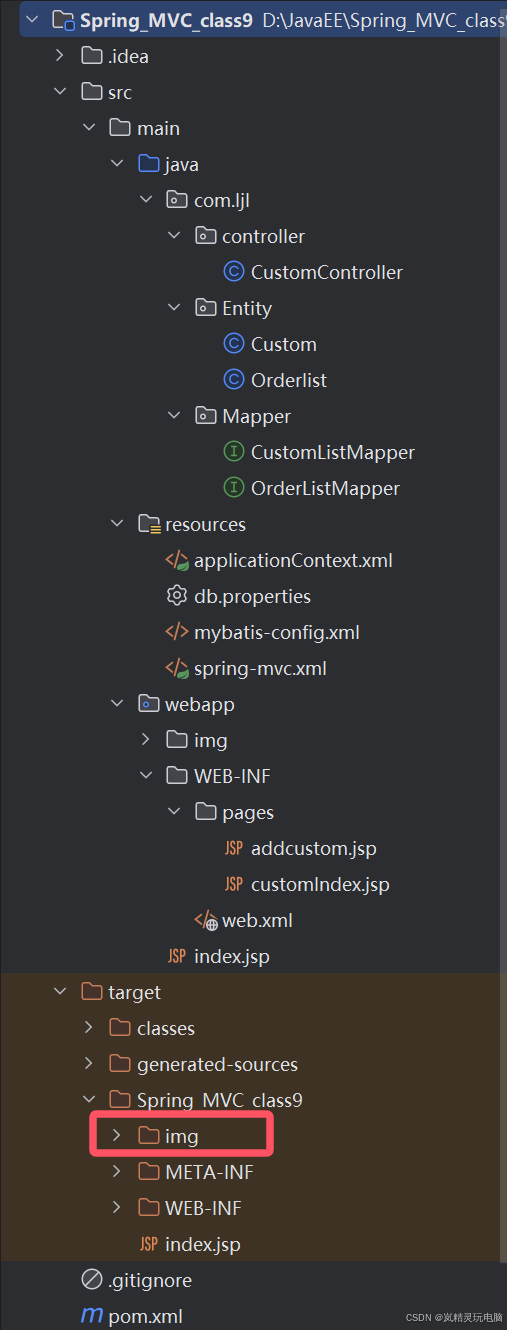
1.创建customlist数据表和orderlist数据表
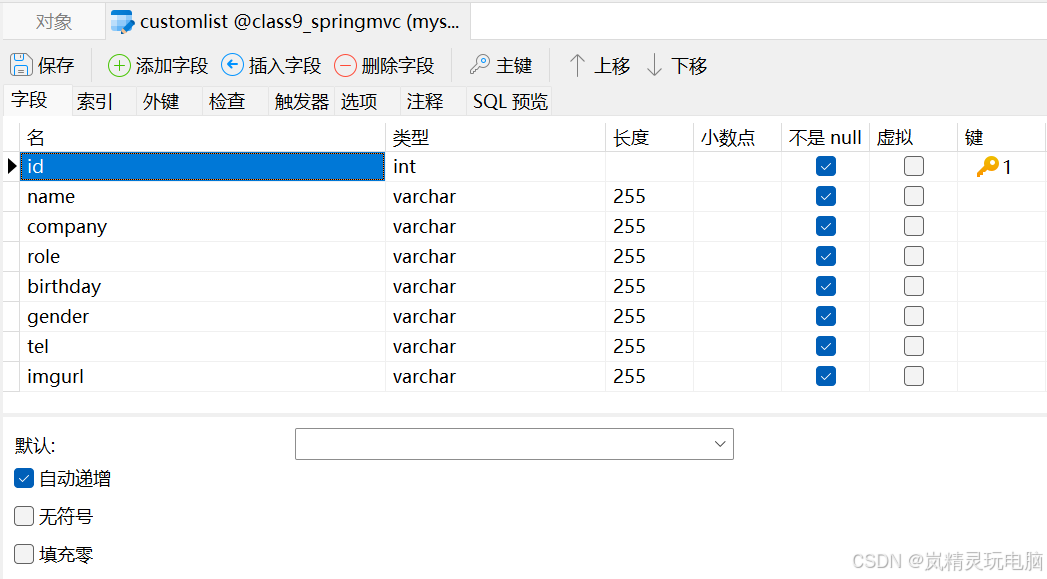
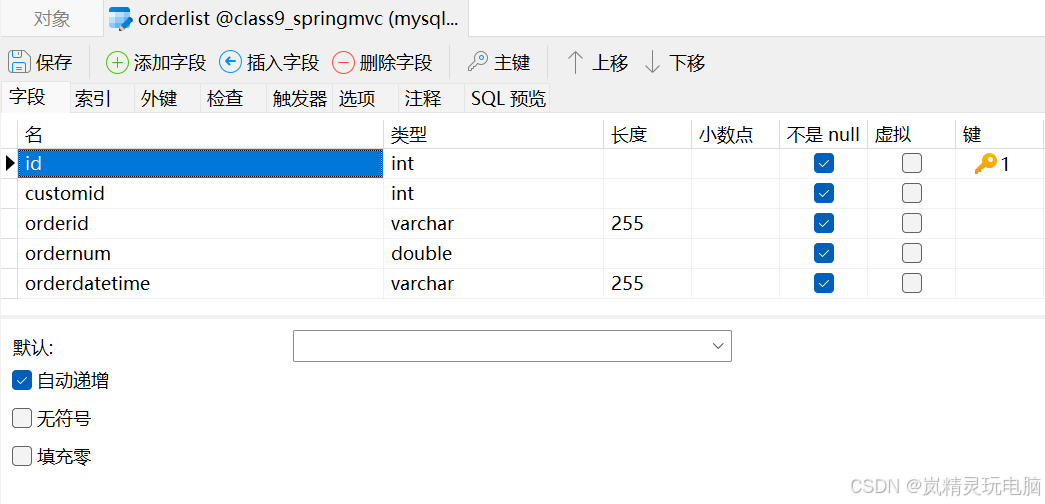
java
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for customlist
-- ----------------------------
DROP TABLE IF EXISTS `customlist`;
CREATE TABLE `customlist` (
`id` int NOT NULL AUTO_INCREMENT,
`name` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`company` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`role` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`birthday` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`gender` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`tel` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`imgurl` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 27 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin ROW_FORMAT = Dynamic;
-- ----------------------------
-- Table structure for orderlist
-- ----------------------------
DROP TABLE IF EXISTS `orderlist`;
CREATE TABLE `orderlist` (
`id` int NOT NULL AUTO_INCREMENT,
`customid` int NOT NULL,
`orderid` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
`ordernum` double NOT NULL,
`orderdatetime` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 9 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
2. 创建SpringMVC项目,引入相关依赖
java
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ljl</groupId>
<artifactId>Spring_MVC_class9</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>Spring_MVC_class9 Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring 基础配置-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
</dependency>
<!-- SpringMVC工具插件-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.10</version>
</dependency>
<!-- servlet插件-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.32</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.11</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.15.4</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.15.4</version>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
<build>
<finalName>Spring_MVC_class9</finalName>
</build>
</project>
3. 创建db.properties和mybatis-config.xml配置文件
java
mysql.driver=com.mysql.cj.jdbc.Driver
mysql.url=jdbc:mysql://localhost:3306/class9_springmvc?serverTimezone=UTC&\characterEncoding=utf-8&useUnicode=true&useSSl=false
mysql.username=root
mysql.password=pjl2003
java
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="db.properties"/>
<!-- 设置实体别名-->
<typeAliases>
<package name="com.ljl.Entity"/>
</typeAliases>
<!-- 和spring整合后 environments配置将废除-->
<environments default="development">
<environment id="development">
<!-- 使用jdbc事务管理-->
<transactionManager type="JDBC" />
<!-- 数据库连接池-->
<dataSource type="POOLED">
<property name="driver" value="${mysql.driver}" />
<property name="url" value="${mysql.url}" />
<!-- //数据库账号-->
<property name="username" value="${mysql.username}" />
<!-- //数据库密码-->
<property name="password" value="${mysql.password}" />
</dataSource>
</environment>
</environments>
<!--声明类对应的sql操作文件-->
<mappers>
<mapper class="com.ljl.Mapper.CustomListMapper"/>
<mapper class="com.ljl.Mapper.OrderListMapper"/>
</mappers>
</configuration>
4. 创建web.xml和spring-mvc.xml文件
java
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<!--允许访问jpg。 并且必须加在springmvc的servlet之前-->
<servlet-mapping>
<servlet-name>default</servlet-name>
<url-pattern>*.jpg</url-pattern>
</servlet-mapping>
<servlet>
<servlet-name>DispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>DispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<filter>
<filter-name>chinese_encoder</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>chinese_encoder</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
java
<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<mvc:annotation-driven/>
<context:component-scan base-package="com.ljl.controller"/>
<bean class="org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping" />
<bean class="org.springframework.web.servlet.mvc.SimpleControllerHandlerAdapter" />
<!-- 配置视图解释器-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/"/>
<property name="suffix" value=".jsp"/>
</bean>
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8"/>
<property name="maxUploadSize" value="2097152"/>
</bean>
<mvc:default-servlet-handler/>
</beans>
5.创建Entity-Custom和Entity-Orderlist实体类
java
package com.ljl.Entity;
public class Custom {
private int id;
private String name;
private String company;
private String role;
private String birthday;
private String gender;
private String tel;
private String imgurl;
public Custom() {
}
public Custom(int id, String name, String company, String role, String birthday, String gender, String tel, String imgurl) {
this.id = id;
this.name = name;
this.company = company;
this.role = role;
this.birthday = birthday;
this.gender = gender;
this.tel = tel;
this.imgurl = imgurl;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getImgurl() {
return imgurl;
}
public void setImgurl(String imgurl) {
this.imgurl = imgurl;
}
@Override
public String toString() {
return "Custom{" +
"id=" + id +
", name='" + name + '\'' +
", company='" + company + '\'' +
", role='" + role + '\'' +
", birthday='" + birthday + '\'' +
", gender='" + gender + '\'' +
", tel='" + tel + '\'' +
", imgurl='" + imgurl + '\'' +
'}';
}
}
java
package com.ljl.Entity;
public class Orderlist {
private int id;
private int customid;
private String orderid;
private double ordernum;
private String orderdatetime;
public Orderlist() {
}
public Orderlist(int id, int customid, String orderid, double ordernum, String orderdatetime) {
this.id = id;
this.customid = customid;
this.orderid = orderid;
this.ordernum = ordernum;
this.orderdatetime = orderdatetime;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getCustomid() {
return customid;
}
public void setCustomid(int customid) {
this.customid = customid;
}
public String getOrderid() {
return orderid;
}
public void setOrderid(String orderid) {
this.orderid = orderid;
}
public double getOrdernum() {
return ordernum;
}
public void setOrdernum(double ordernum) {
this.ordernum = ordernum;
}
public String getOrderdatetime() {
return orderdatetime;
}
public void setOrderdatetime(String orderdatetime) {
this.orderdatetime = orderdatetime;
}
@Override
public String toString() {
return "Orderlist{" +
"id=" + id +
", customid=" + customid +
", orderid='" + orderid + '\'' +
", ordernum=" + ordernum +
", orderdatetime='" + orderdatetime + '\'' +
'}';
}
}
6. 创建applicationContext.xml配置文件
java
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 自动装配Bean-->
<context:component-scan base-package="com.ljl.controller"/>
</beans>
7. 创建Mapper-CustomListMapper和Mapper-OrderListMapper文件
java
package com.ljl.Mapper;
import com.ljl.Entity.Custom;
import org.apache.ibatis.annotations.*;
import java.util.List;
public interface CustomListMapper {
//TODO 增加客户信息
@Insert("INSERT INTO customlist (name, company, role, birthday, gender, tel, imgurl) values (#{name}, #{company}, #{role}, #{birthday}, #{gender}, #{tel}, #{imgurl})")
int insertCustom(Custom custom);
//TODO 查询客户信息-全部
@Select("SELECT * FROM customlist")
List<Custom> getAllCustomList();
//TODO 查询订单信息-根据客户名称模糊查询
@Select("SELECT * FROM customlist WHERE name LIKE CONCAT('%', #{name}, '%')")
List<Custom> getOrderListByLikeCustomName(String name);
//TODO 查询订单信息-根据客户名称详细查询
@Select("SELECT * FROM customlist WHERE name = #{name}")
List<Custom> getOrderListByCustomName(String name);
//TODO 删除客户信息-根据顾客id
@Delete("DELETE FROM customlist WHERE id = #{id}")
int deleteCustomerById(int id);
//TODO 更新顾客信息-根据顾客name
@Update("UPDATE customlist SET company = #{company}, role = #{role}, birthday = #{birthday}, gender = #{gender}, tel = #{tel} WHERE name = #{name}")
int updateCustomerInfo(Custom custom);
}
java
package com.ljl.Mapper;
import com.ljl.Entity.Orderlist;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface OrderListMapper {
//TODO 增加订单信息
@Insert("INSERT INTO orderlist (customid, orderid, ordernum, orderdatetime) values (#{customid}, #{orderid}, #{ordernum}, #{orderdatetime})")
int insertorder(Orderlist orderlist);
//TODO 查询订单信息-全部
//TODO 查询班级下所有学生的信息
@Select("SELECT * FROM orderlist WHERE customid = #{customid}")
List<Orderlist> selectOrderlistByCustomid(int customid);
}
8. 编辑index.jsp前端界面
html
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>CustomWelcome</title>
<!-- import CSS -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
</head>
<body>
<div id="index" class="big_box">
<h1 class="tittle">欢迎使用{{ sys_name }}客户管理系统</h1>
<el-row>
<el-button type="primary" round style="width: 14%" @click="gosystem">开始使用</el-button>
</el-row>
</div>
</body>
<!-- import Vue before Element -->
<script src="https://unpkg.com/vue@2/dist/vue.js"></script>
<!-- import JavaScript -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<script>
new Vue({
el: '#index',
data: function() {
return {
sys_name:"岚精灵"
}
},
methods: {
gosystem(){
this.$message.success("欢迎使用岚精灵员工系统!");
// 跳转到指定的URL
// 设置延迟1秒后跳转
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 1000); // 1000毫秒等于1秒
}
}
})
</script>
<style>
*{
margin: 0;
padding: 0;
}
body {
background-color: #9CFE;
}
.big_box{
width: 100%;
text-align: center;
margin: 0 auto;
}
.tittle{
margin-top: 250px;
font-size: 55px;
color: white;
margin-bottom: 170px;
}
</style>
</html>
9.创建pages-customIndex.jsp和addcustom.jsp前端界面
html
<%--
Created by IntelliJ IDEA.
User: 26520
Date: 2024/11/21
Time: 13:40
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>customSystem</title>
<!-- import CSS -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
</head>
<body>
<div id="cindex" class="cindex">
<h1 style="text-align: center;color: white;margin-bottom: 35px">岚精灵客户管理系统</h1>
<%-- 搜索框--%>
<div style="margin-bottom: 40px;">
<%-- 这里的键盘事件,由于使用了Element-ui,需要加上.native进行限制,因为在Element-ui中对active进行了封装,需要加上native进行限制--%>
<el-input placeholder="请输入客户名称" v-model="search" class="input-with-select" @keyup.enter.native="search_ks">
<el-select v-model="select" slot="prepend" placeholder="请选择">
<el-option label="模糊查询" value="1"></el-option>
<el-option label="准确查询" value="2"></el-option>
</el-select>
<el-button @click="search_ks" slot="append" icon="el-icon-search" @keyup.enter.native="search_ks"></el-button>
</el-input>
</div>
<%-- 内容展示框--%>
<el-table height="550"
:data="tableData"
style="width: 100%"
:row-class-name="tableRowClassName"
cell-class-name="table-cell-center"
:default-sort = "{prop: 'orderdatetime', order: 'descending'}">
<el-table-column
width="70"
prop="id"
label="id"
sortable>
</el-table-column>
<el-table-column
prop="name"
label="客户名称"
sortable>
</el-table-column>
<el-table-column
prop="company"
label="客户单位">
</el-table-column>
<el-table-column
width="90"
prop="role"
label="客户职位">
</el-table-column>
<el-table-column
prop="birthday"
label="客户生日"
sortable>
</el-table-column>
<el-table-column
width="110"
prop="gender"
label="客户性别"
sortable>
</el-table-column>
<el-table-column
prop="tel"
label="联系方式">
</el-table-column>
<el-table-column
label="头像">
<template slot-scope="scope">
<el-image
style="width: 100px; height: 100px"
:src="scope.row.imgurl"
:fit="cover">
</el-image>
</template>
</el-table-column>
<el-table-column label="客户操作" width="220">
<template slot-scope="scope">
<el-button
size="mini"
@click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button
size="mini"
type="danger"
@click="handleDelete(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
<el-table-column label="订单操作" width="230">
<template slot-scope="scope">
<el-button
size="mini"
type="success"
@click="handledd(scope.$index, scope.row)">订单列表</el-button>
<el-button
size="mini"
type="primary"
@click="handlenewdd(scope.$index, scope.row)">新建订单</el-button>
</template>
</el-table-column>
</el-table>
<div class="dibu_button">
<el-row style="display: inline-block">
<el-button type="primary" plain @click="addcustom">添加客户</el-button>
</el-row>
<el-row style="display: inline-block; margin-left: 30px">
<el-button type="info" plain @click="go_out">退出系统</el-button>
</el-row>
</div>
<%-- 这里开始编辑弹窗--%>
<%-- 删除弹窗--%>
<el-dialog
title="删除客户"
:visible.sync="deleteVisible"
width="30%"
:before-close="handleClose">
<span>是否确定删除该客户?</span>
<span slot="footer" class="dialog-footer">
<el-button @click="deleteVisible = false">取 消</el-button>
<el-button type="primary" @click="deletecustom(),deleteVisible = false">确 定</el-button>
</span>
</el-dialog>
<%-- 编辑客户信息--%>
<el-dialog title="编辑客户信息" :visible.sync="editVisible">
<el-form ref="form" :model="form" :label-position="right" label-width="80px" action="">
<el-form-item label="姓名">
<el-input v-model="form.name" :disabled="true"></el-input>
</el-form-item>
<el-form-item label="单位">
<el-input v-model="form.company"></el-input>
</el-form-item>
<el-form-item label="职位">
<el-input v-model="form.role"></el-input>
</el-form-item>
<el-form-item label="生日">
<el-date-picker
v-model="form.birthday"
type="date"
style="width: 100%"
placeholder="选择日期">
</el-date-picker>
</el-form-item>
<el-form-item label="性别">
<el-select style="width: 100%" v-model="form.gender" placeholder="请选择">
<el-option
v-for="item in genderchange"
:key="item.value"
:label="item.label"
:value="item.value">
</el-option>
</el-select>
</el-form-item>
<el-form-item label="电话">
<el-input v-model="form.tel"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="editVisible = false">取 消</el-button>
<el-button type="primary" @click="editcustom(),editVisible = false">修 改</el-button>
</div>
</el-dialog>
<%-- 客户订单列表--%>
<el-dialog title="订单列表" :visible.sync="ddlistVisible">
<el-table :data="ddlist" :default-sort = "{prop: 'orderdatetime', order: 'descending'}">
<el-table-column property="orderid" label="订单编号(Order ID)"></el-table-column>
<el-table-column property="ordernum" label="订单金额(RMB)" sortable></el-table-column>
<el-table-column property="orderdatetime" label="下单时间(TIME)" sortable></el-table-column>
</el-table>
</el-dialog>
<%-- 新增信息--%>
<el-dialog title="新增订单" :visible.sync="newddVisible">
<el-form ref="newddform" :model="newddform" :label-position="right" label-width="80px" action="">
<el-form-item label="金额">
<el-input v-model="newddform.ordernum"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="newddVisible = false">取 消</el-button>
<el-button type="primary" @click="newdd(),newddVisible = false">新 增</el-button>
</div>
</el-dialog>
</div>
</body>
<!-- import Vue before Element -->
<script src="https://unpkg.com/vue@2/dist/vue.js"></script>
<!-- import JavaScript -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<script>
new Vue({
el: '#cindex',
data: function() {
return {
search: '',
// 这里默认情况下是模糊查询
select: '1',
tableData: [],
changeid:"",
indexnum:"",
editVisible: false,
deleteVisible: false,
ddlistVisible:false,
newddVisible:false,
form: {
name: '',
company:"",
role:"",
birthday:"",
gender:"",
tel:""
},
genderchange: [{
value: '男',
label: '男'
}, {
value: '女',
label: '女'
}, {
value: '其他',
label: '其他'
}],
ddlist:[],
newddform:{
customid:"",
ordernum:""
}
}
},
methods: {
handleClose(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
},
handleEdit(index, row) {
this.changeid = row.id;
this.editVisible = true;
this.indexnum = index;
this.form.name = this.tableData[index].name;
this.form.company = this.tableData[index].company;
this.form.role = this.tableData[index].role;
this.form.birthday = this.tableData[index].birthday;
this.form.gender = this.tableData[index].gender;
this.form.tel = this.tableData[index].tel;
console.log(index, row.id);
},
handleDelete(index, row) {
this.changeid = row.id;
this.deleteVisible = true;
console.log(index, row.id);
},
handledd(index, row){
this.ddlistVisible = true;
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/selectorderlistBycustomid?customid=' + row.id, {
method: 'POST', // 设置请求方法为 POST
headers: {
'Accept': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
this.ddlist = data;
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
},
handlenewdd(index, row){
this.newddform.customid = row.id;
this.newddVisible = true;
},
tableRowClassName({row, rowIndex}) {
if (rowIndex === 1) {
return 'warning-row';
} else if (rowIndex === 3) {
return 'success-row';
}
return '';
},
addcustom(){
this.$message.warning("加载中!");
// 跳转到指定的URL
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/goaddcustom';
}, 300);
},
fetchData() {
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/selectAllCustomList', {
headers: {
'Accept': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
this.tableData = data;
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
},
search_ks(){
// 实现点击查询功能,先判断是模糊查询还是准确查询,查询出来的数据保存在tableData[]中
//模糊查询
if (this.search === ""){
this.$message.error("请输入需要查询的客户姓名!")
}else {
if (this.select === "1"){
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/selectCustomLikeName?CustomName=' + this.search, {
method: 'POST', // 设置请求方法为 POST
headers: {
'Accept': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
this.$message.success("查询成功!")
this.tableData = data;
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
}else if (this.select === "2") {
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/selectCustomByName?CustomName=' + this.search, {
method: 'POST', // 设置请求方法为 POST
headers: {
'Accept': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
this.$message.success("查询成功!")
this.tableData = data;
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
}
}
},
go_out(){
this.$message.success("退出成功!")
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/';
}, 300);
},
deletecustom(){
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/deleteCustomByid?id=' + this.changeid, {
method: 'POST', // 设置请求方法为 POST
headers: {
'Accept': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
this.$message.success("删除成功!")
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 300);
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
},
checkForm() {
let allFilled = true;
for (let key in this.form) {
if (!this.form[key] && this.form[key] !== 0) { // 假设0是一个有效的值
allFilled = false;
break;
}
}
return allFilled;
},
editcustom(){
if (!this.checkForm()) {
this.$message.error("请填写所有必填字段");
return;
}
if (this.form.birthday instanceof Date) {
// 格式化生日日期为 yyyy-mm-dd
// this.form.birthday = this.form.birthday ? this.form.birthday.toISOString().split('T')[0] : '';
// 获取年、月、日
const year = this.form.birthday.getFullYear();
const month = (this.form.birthday.getMonth() + 1).toString().padStart(2, '0'); // 月份从0开始,所以加1
const day = this.form.birthday.getDate().toString().padStart(2, '0');
// 格式化生日日期为 yyyy-mm-dd
this.form.birthday = year+"-"+month+"-"+day;
}
// 创建URLSearchParams对象并添加数据
const params = new URLSearchParams();
params.append('name', this.form.name);
params.append('company', this.form.company);
params.append('role', this.form.role);
params.append('birthday', this.form.birthday);
params.append('gender', this.form.gender);
params.append('tel', this.form.tel);
console.log(params);
// 发送POST请求
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/editcustom', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
},
body: params // URLSearchParams对象会自动转换为URL编码的字符串
})
.then(response => {
if (response.ok) {
return response.text(); // 或者 response.json() 如果响应是JSON格式
}
throw new Error('Network response was not ok.');
})
.then(data => {
console.log(data);
this.$message.success("用户信息修改成功!");
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 300); // 1000毫秒等于1秒
})
.catch(error => {
console.error('Error:', error);
this.$message.error("数用户信息修改失败!");
});
},
newdd(){
if (this.newddform.customid === ""){
this.$message.error("请填写所有必填字段");
}else {
const params = new URLSearchParams();
params.append('customid', this.newddform.customid);
params.append('ordernum', this.newddform.ordernum);
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/addorder', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
},
body: params // URLSearchParams对象会自动转换为URL编码的字符串
})
.then(response => {
if (response.ok) {
return response.text(); // 或者 response.json() 如果响应是JSON格式
}
throw new Error('Network response was not ok.');
})
.then(data => {
console.log(data);
this.$message.success("订单添加成功!");
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 300); // 1000毫秒等于1秒
})
.catch(error => {
console.error('Error:', error);
this.$message.error("订单添加失败!");
});
}
}
},
created() {
this.fetchData(); // 当组件创建后调用 fetchData 方法
},
})
</script>
<style>
*{
margin: 0;
padding: 0;
}
body {
background-color: #9CFE;
}
.cindex{
width: 85%;
padding-top: 45px;
text-align: center;
margin: 0 auto;
}
.el-select .el-input {
width: 130px;
}
.input-with-select .el-input-group__prepend {
background-color: #e6e5e6;
}
.el-table .warning-row {
background: #fbf7f7;
}
.el-table .success-row {
background: #f0f9eb;
}
.dibu_button{
width: 100%;
margin: 0 auto;
margin-top: 30px;
text-align: center;
}
</style>
</html>
html
<%--
Created by IntelliJ IDEA.
User: 26520
Date: 2024/11/21
Time: 13:40
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>customSystem</title>
<!-- import CSS -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
</head>
<body>
<div id="addcustom" class="big">
<div class="from">
<h1 style="text-align: center;color: #9CFE;font-size: 35px;margin-bottom: 40px;margin-top: 40px">添加客户</h1>
<el-form ref="form" :model="form" :label-position="right" label-width="80px" action="">
<el-form-item label="姓名">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item label="单位">
<el-input v-model="form.company"></el-input>
</el-form-item>
<el-form-item label="职位">
<el-input v-model="form.role"></el-input>
</el-form-item>
<el-form-item label="生日">
<el-date-picker
v-model="form.birthday"
type="date"
style="width: 100%"
placeholder="选择日期">
</el-date-picker>
</el-form-item>
<el-form-item label="性别">
<el-select style="width: 100%" v-model="form.gender" placeholder="请选择">
<el-option
v-for="item in genderchange"
:key="item.value"
:label="item.label"
:value="item.value">
</el-option>
</el-select>
</el-form-item>
<el-form-item label="电话">
<el-input v-model="form.tel"></el-input>
</el-form-item>
<div class="upload" style="height: 50px;margin-bottom: 60px">
<el-upload
class="upload-demo"
ref="upload"
action="${pageContext.request.contextPath}/upload"
:on-preview="handlePreview"
:on-remove="handleRemove"
name="imgfile"
:auto-upload="false">
<el-button slot="trigger" size="small" type="primary">选取文件</el-button>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
</div>
<el-button type="primary" @click="add">立即创建</el-button>
<el-button type="danger" @click="back" style="margin-bottom: 40px">立即返回</el-button>
</el-form>
</div>
</div>
</body>
<!-- import Vue before Element -->
<script src="https://unpkg.com/vue@2/dist/vue.js"></script>
<!-- import JavaScript -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<script>
new Vue({
el: '#addcustom',
data: function() {
return {
form: {
name: '',
company:"",
role:"",
birthday:"",
gender:"",
tel:"",
fileList:[]
},
pickerOptions: {
disabledDate(time) {
return time.getTime() > Date.now();
},
},
genderchange: [{
value: '男',
label: '男'
}, {
value: '女',
label: '女'
}, {
value: '其他',
label: '其他'
}],
}
},
methods: {
handleRemove(file, fileList) {
console.log(file, fileList);
},
handlePreview(file) {
console.log(file);
},
checkForm() {
let allFilled = true;
for (let key in this.form) {
if (!this.form[key] && this.form[key] !== 0) { // 假设0是一个有效的值
allFilled = false;
break;
}
}
return allFilled;
},
add(){
if (!this.checkForm()) {
this.$message.error("请填写所有必填字段");
return;
}
this.$refs.upload.submit();
setTimeout(() => {
}, 500); // 1000毫秒等于1秒
if (this.form.birthday instanceof Date) {
// 格式化生日日期为 yyyy-mm-dd
// this.form.birthday = this.form.birthday ? this.form.birthday.toISOString().split('T')[0] : '';
// 获取年、月、日
const year = this.form.birthday.getFullYear();
const month = (this.form.birthday.getMonth() + 1).toString().padStart(2, '0'); // 月份从0开始,所以加1
const day = this.form.birthday.getDate().toString().padStart(2, '0');
// 格式化生日日期为 yyyy-mm-dd
this.form.birthday = year+"-"+month+"-"+day;
}
// 创建URLSearchParams对象并添加数据
const params = new URLSearchParams();
params.append('name', this.form.name);
params.append('company', this.form.company);
params.append('role', this.form.role);
params.append('birthday', this.form.birthday);
params.append('gender', this.form.gender);
params.append('tel', this.form.tel);
// 发送POST请求
fetch('http://localhost:8090/Spring_MVC_class9_war_exploded/addcustom', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
},
body: params // URLSearchParams对象会自动转换为URL编码的字符串
})
.then(response => {
if (response.ok) {
return response.text(); // 或者 response.json() 如果响应是JSON格式
}
throw new Error('Network response was not ok.');
})
.then(data => {
console.log(data);
this.$message.success("数据添加成功!");
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 300); // 1000毫秒等于1秒
})
.catch(error => {
console.error('Error:', error);
this.$message.error("数据添加失败!");
});
},
back(){
this.$message.warning("请稍等!");
// 跳转到指定的URL
// 设置延迟1秒后跳转
setTimeout(() => {
window.location.href = 'http://localhost:8090/Spring_MVC_class9_war_exploded/customIndex';
}, 300); // 1000毫秒等于1秒
}
}
})
</script>
<style>
*{
margin: 0;
padding: 0;
}
body {
background-color: #9CFE;
}
.big{
width: 70%;
padding-top: 100px;
text-align: center;
margin: 0 auto;
}
.from{
width: 40%;
padding-right: 40px;
text-align: center;
margin: 0 auto;
border: 1px solid #ffffff;
border-radius: 8px;
background-color: white;
}
</style>
</html>
10.创建controller-CustomController文件
java
package com.ljl.controller;
import com.ljl.Entity.Custom;
import com.ljl.Entity.Orderlist;
import com.ljl.Mapper.CustomListMapper;
import com.ljl.Mapper.OrderListMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.UUID;
@Controller
@CrossOrigin(origins = "*")
public class CustomController {
//主页
@RequestMapping("/customIndex")
public String customIndex() {
return "customIndex";
}
//添加客户界面
@RequestMapping("/goaddcustom")
public String goaddcustom() {
return "addcustom";
}
public String img_url;
//添加客户到sql,再返回到主页
@RequestMapping("/addcustom")
public String addcustom(Custom custom) {
if (custom.getName() != null) {
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
custom.setImgurl(img_url);
int result_code = customListMapper.insertCustom(custom);
sqlSession.commit();
if (result_code == 1) {
System.out.println("新增客户成功!");
}else {
System.out.println("新增客户失败!");
}
}
return "customIndex";
}
//获取全部客户信息
@GetMapping(value = "selectAllCustomList", produces = "application/json")
@ResponseBody
public List<Custom> selectAllCustomList() {
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
List<Custom> custom = customListMapper.getAllCustomList();
System.out.println("系统正在获取所有客户信息");
System.out.println(custom);
return custom;
}
//模糊查询客户信息-根据name模糊查询
@PostMapping(value = "selectCustomLikeName", produces = "application/json")
@ResponseBody
public List<Custom> selectCustomLikeName(HttpServletRequest request){
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
List<Custom> custom = customListMapper.getOrderListByLikeCustomName(request.getParameter("CustomName"));
System.out.println("用户正在进行模糊查询");
return custom;
}
//准确查询客户信息-根据name准确查询
@PostMapping(value = "selectCustomByName", produces = "application/json")
@ResponseBody
public List<Custom> selectCustomByName(HttpServletRequest request){
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
List<Custom> custom = customListMapper.getOrderListByCustomName(request.getParameter("CustomName"));
System.out.println("用户正在进行精确查询");
return custom;
}
//删除客户信息-根据id删除
@PostMapping(value = "deleteCustomByid", produces = "application/json")
@ResponseBody
public int deleteCustomByid(HttpServletRequest request){
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
System.out.println(request.getParameter("id"));
int id = Integer.parseInt(request.getParameter("id"));
int code = customListMapper.deleteCustomerById(id);
sqlSession.commit();
return code;
}
//修改客户信息
@RequestMapping("editcustom")
@ResponseBody
public int editcustom(Custom custom) {
if (custom.getName() != null) {
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
CustomListMapper customListMapper = sqlSession.getMapper(CustomListMapper.class);
System.out.println(custom);
int result_code = customListMapper.updateCustomerInfo(custom);
sqlSession.commit();
if (result_code == 1) {
System.out.println("修改客户成功!");
return 1;
}else {
System.out.println("修改客户失败!");
return 0;
}
}
return 0;
}
//准确查询客户订单信息-根据customid准确查询
@PostMapping(value = "selectorderlistBycustomid", produces = "application/json")
@ResponseBody
public List<Orderlist> selectorderlistBycustomid(HttpServletRequest request){
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
OrderListMapper orderListMapper = sqlSession.getMapper(OrderListMapper.class);
int customid = Integer.parseInt(request.getParameter("customid"));
List<Orderlist> orderlists = orderListMapper.selectOrderlistByCustomid(customid);
return orderlists;
}
//添加订单信息
@RequestMapping("addorder")
@ResponseBody
public void addorder(Orderlist orderlist) {
SqlSessionFactory ssf = null;
try {
InputStream input = Resources.getResourceAsStream("mybatis-config.xml");
ssf = new SqlSessionFactoryBuilder().build(input);
} catch (IOException e) {
e.printStackTrace();
}
SqlSession sqlSession = ssf.openSession();
OrderListMapper orderListMapper = sqlSession.getMapper(OrderListMapper.class);
// 获取当前时间的时间戳(毫秒)
long currentTimeMillis = System.currentTimeMillis();
// 加上 22 毫秒
long newTimeMillis = currentTimeMillis + orderlist.getCustomid();
// 将新的时间戳转换为 String 类型
String timeStampString = String.valueOf(newTimeMillis);
// 获取当前日期和时间
LocalDateTime now = LocalDateTime.now();
// 定义日期时间格式
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
// 格式化日期时间
String formattedDateTime = now.format(formatter);
Orderlist orderlist1 = new Orderlist();
orderlist1.setCustomid(orderlist.getCustomid());
orderlist1.setOrderid("LJL" + timeStampString);
orderlist1.setOrdernum(orderlist.getOrdernum());
orderlist1.setOrderdatetime(formattedDateTime);
orderListMapper.insertorder(orderlist1);
sqlSession.commit();
}
@RequestMapping("/uploadfile")
public String uploadfile(){
return "uploadfile";
}
// 文件上传
@RequestMapping("/upload")
public void upload(MultipartFile imgfile) throws IOException {
String oldName = imgfile.getOriginalFilename();
String suffix = oldName.substring(oldName.lastIndexOf("."));
String newName = UUID.randomUUID() + suffix;
imgfile.transferTo(new File("D:\\JavaEE\\Spring_MVC_class9\\target\\Spring_MVC_class9\\img\\" + newName));
Custom custom = new Custom();
custom.setImgurl(newName);
img_url = "img/" + newName;
System.out.println(newName);
}
}
11. 在target-Spring_MVC_class9创建img静态图片路径
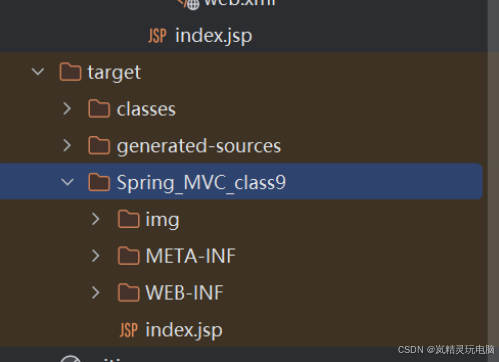
12.编辑Tomcat服务器
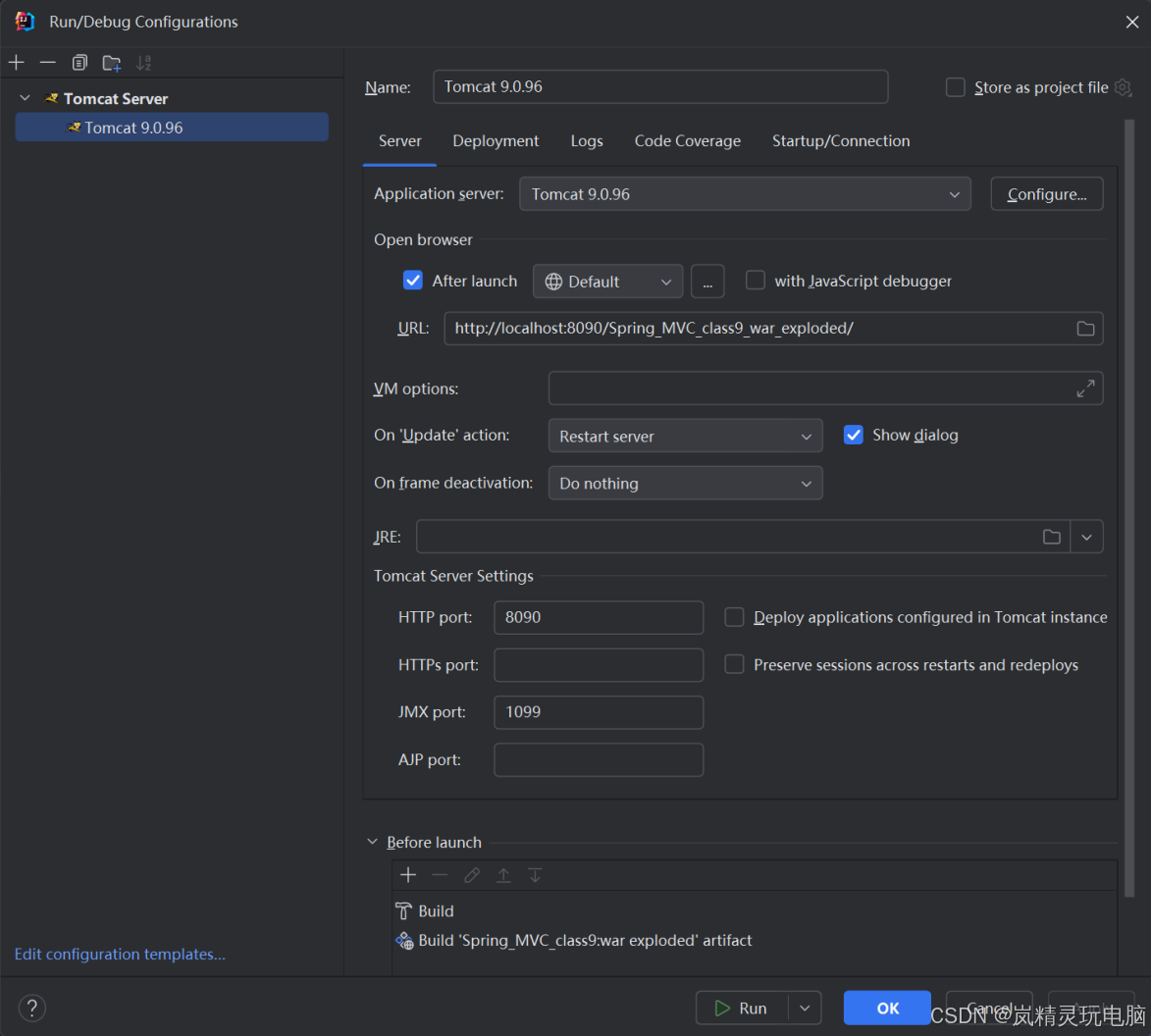
四、查看演示效果
1.引导界面
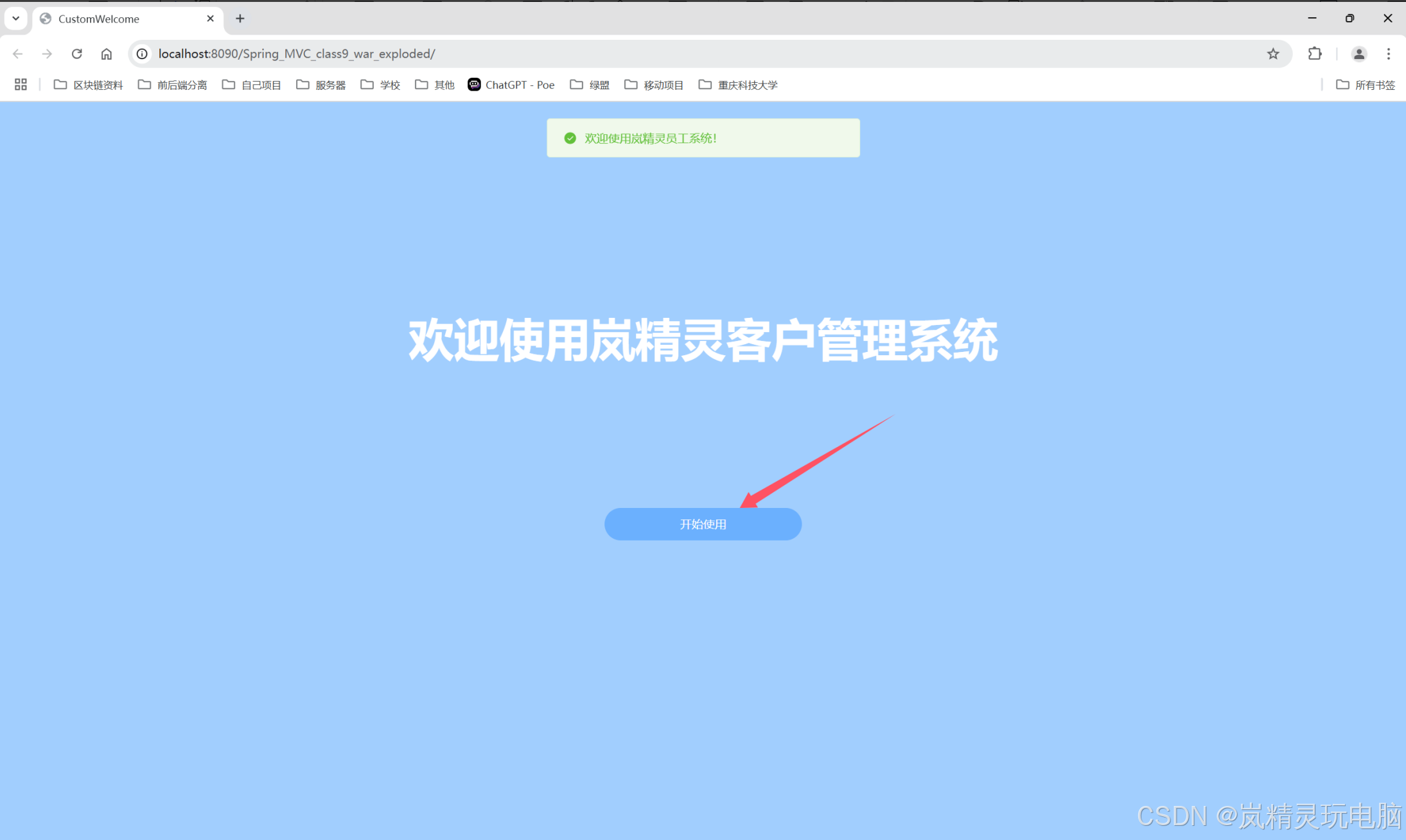
2.进入系统主页
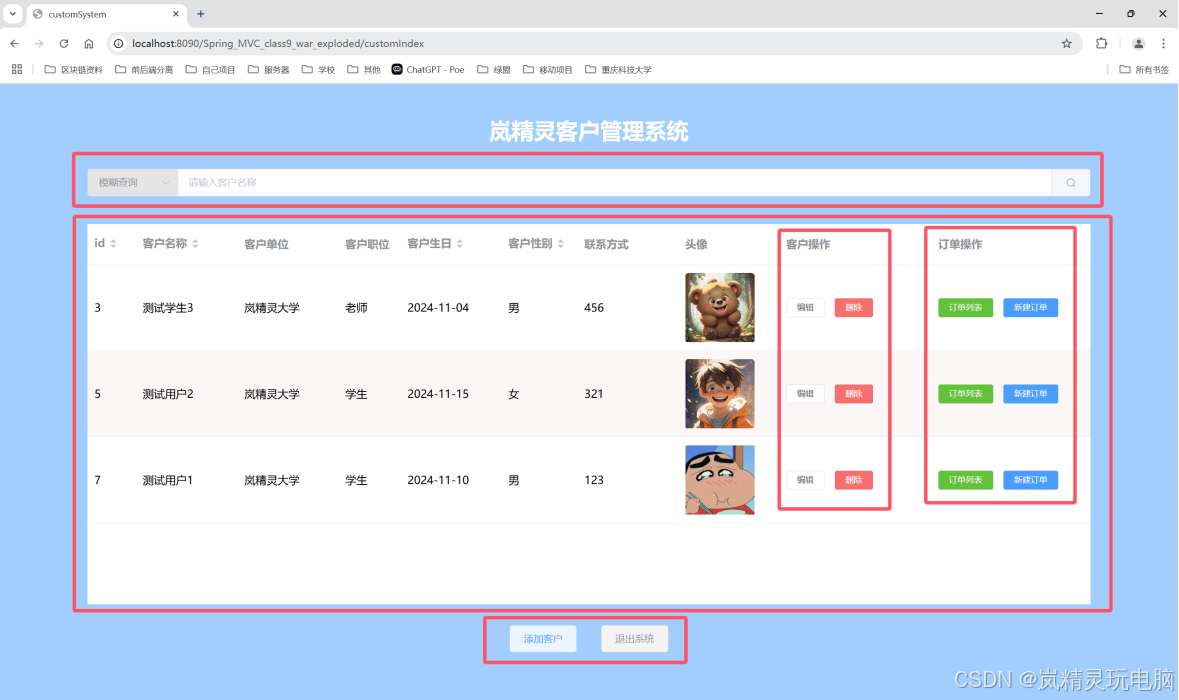
3.模糊搜索客户(可根据名称搜索,可根据年龄等排序)
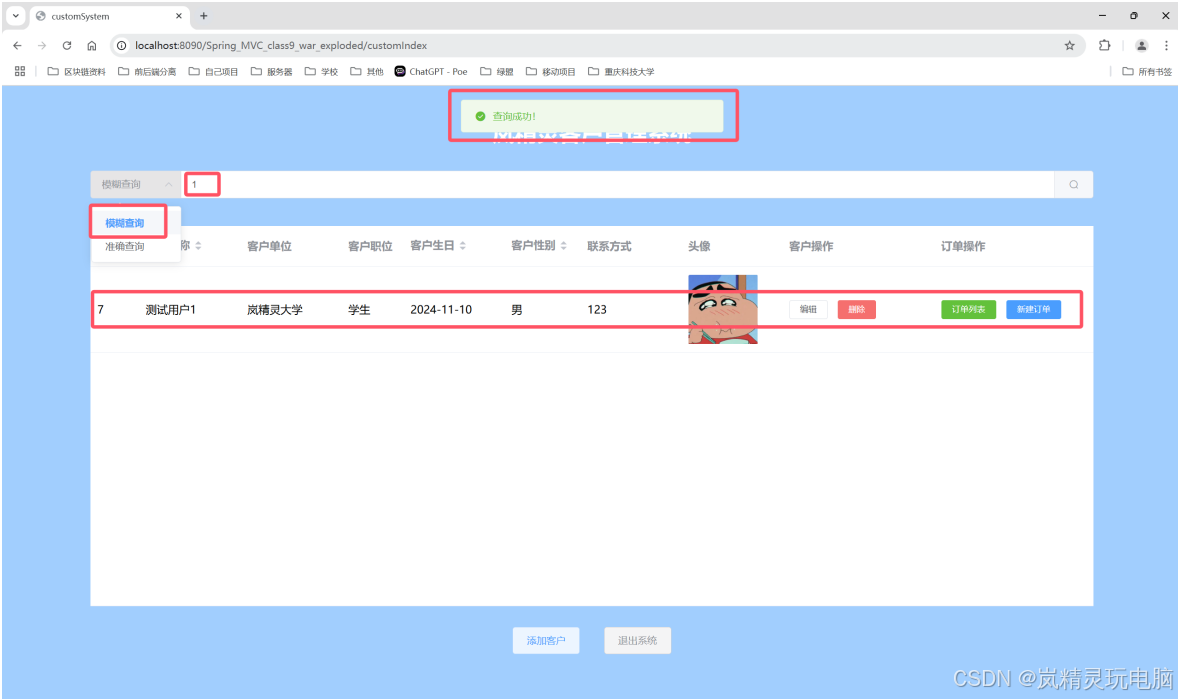
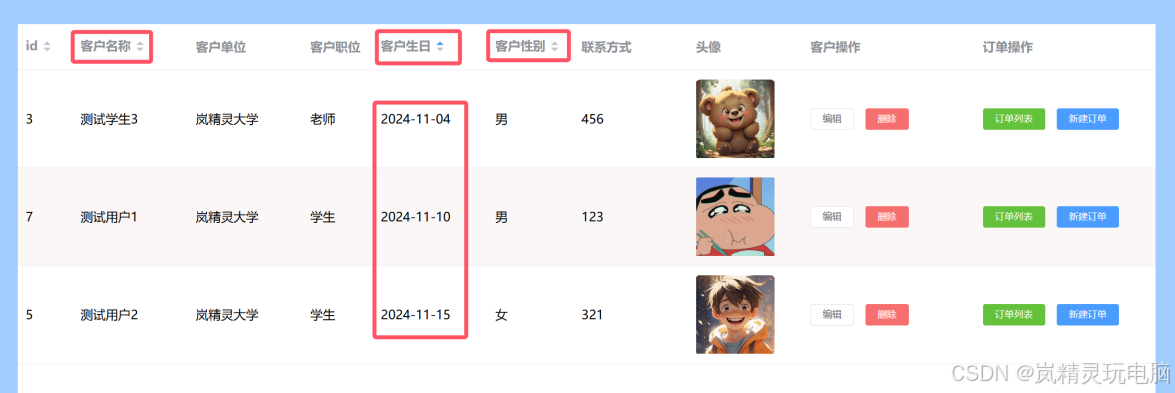
4.准确搜索客户
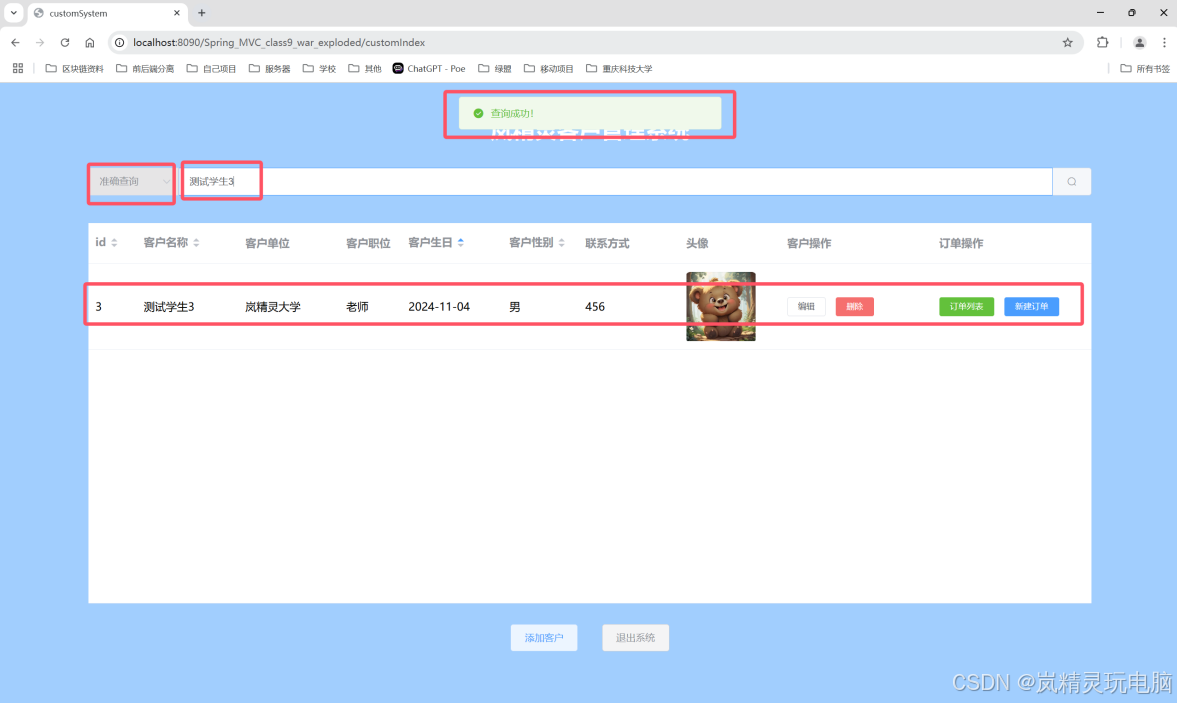
5.新增客户信息
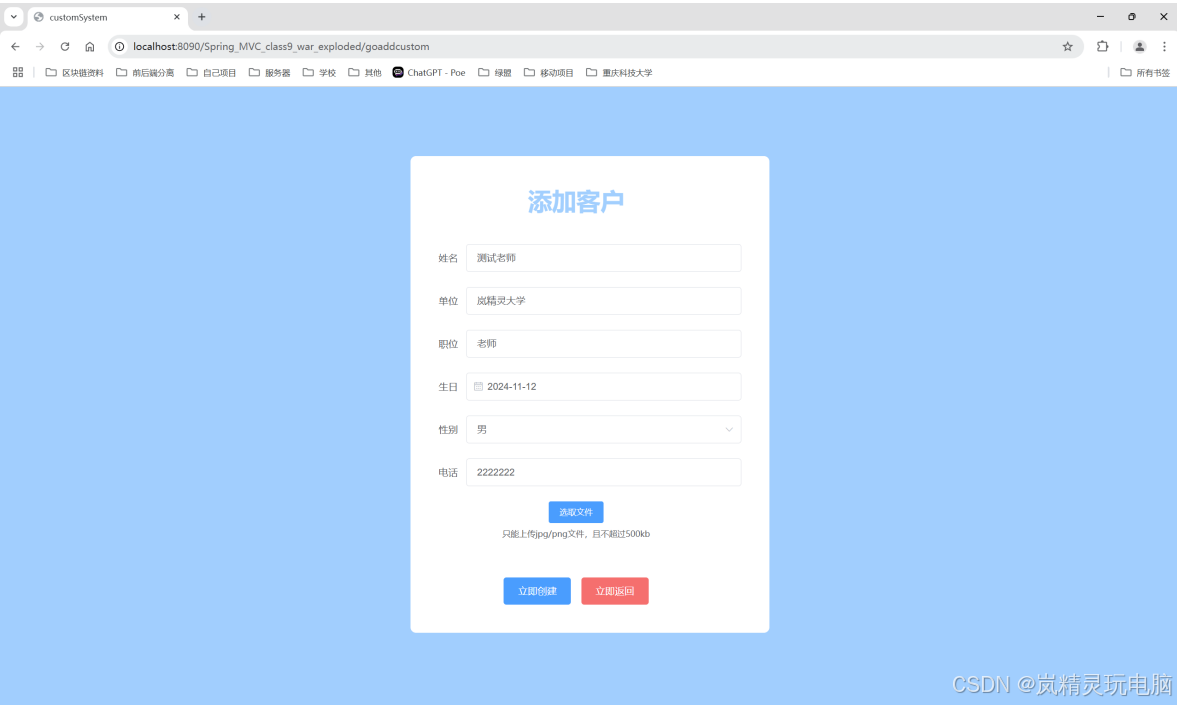
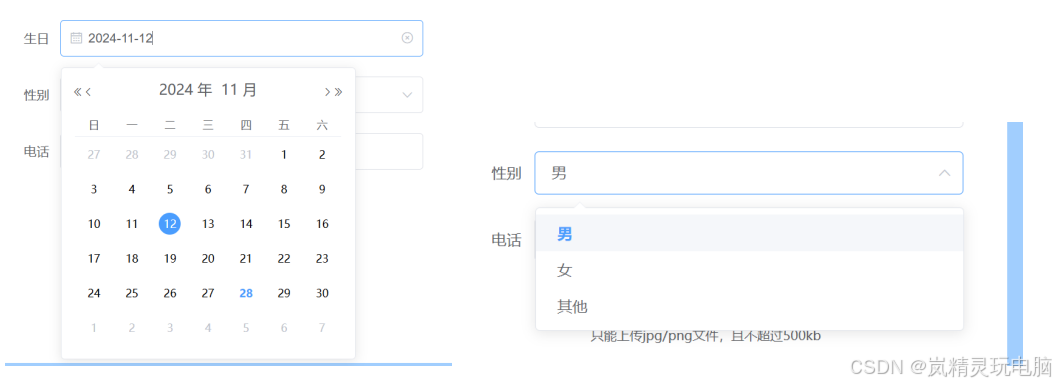
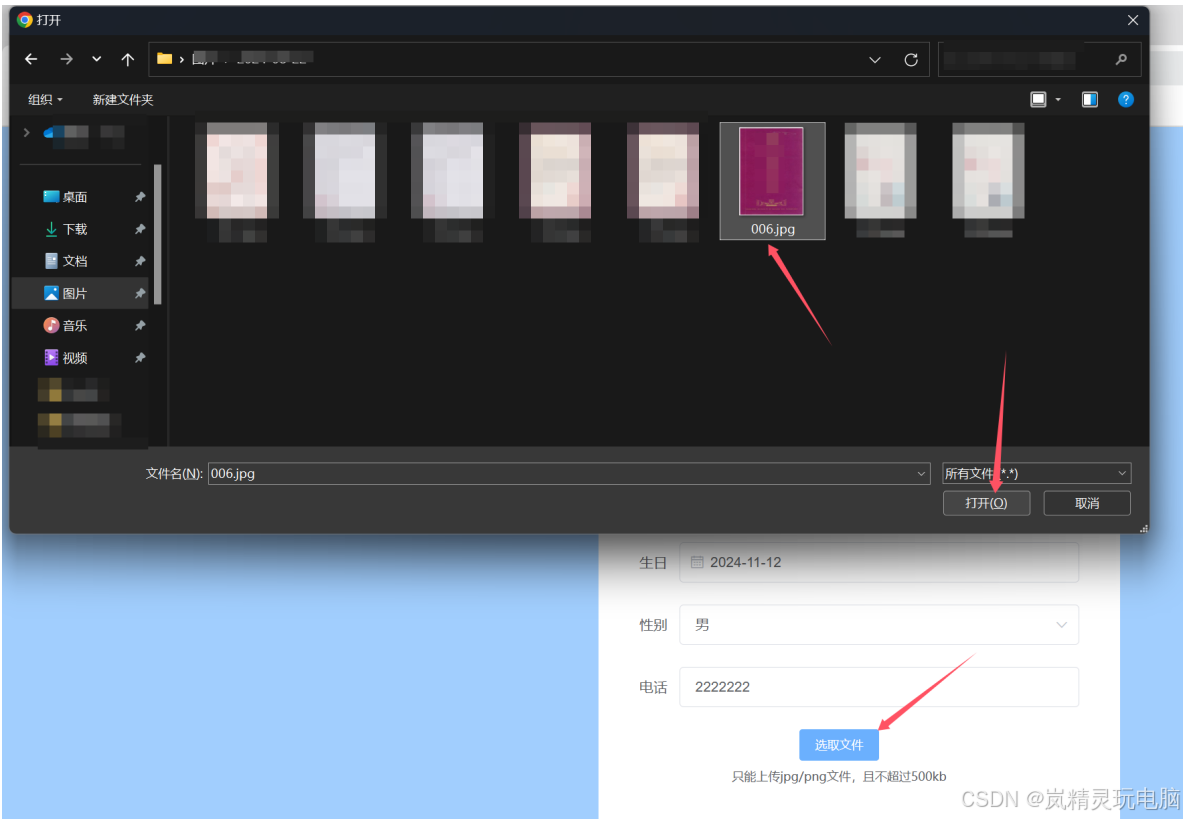
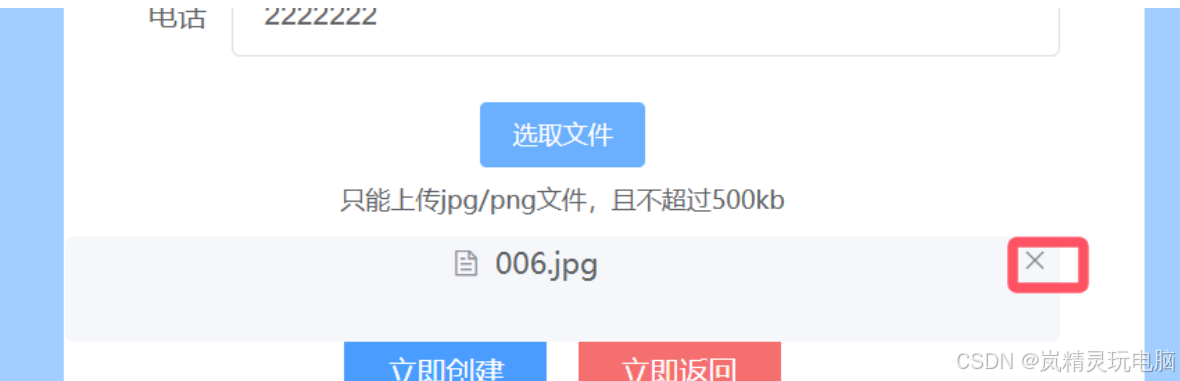
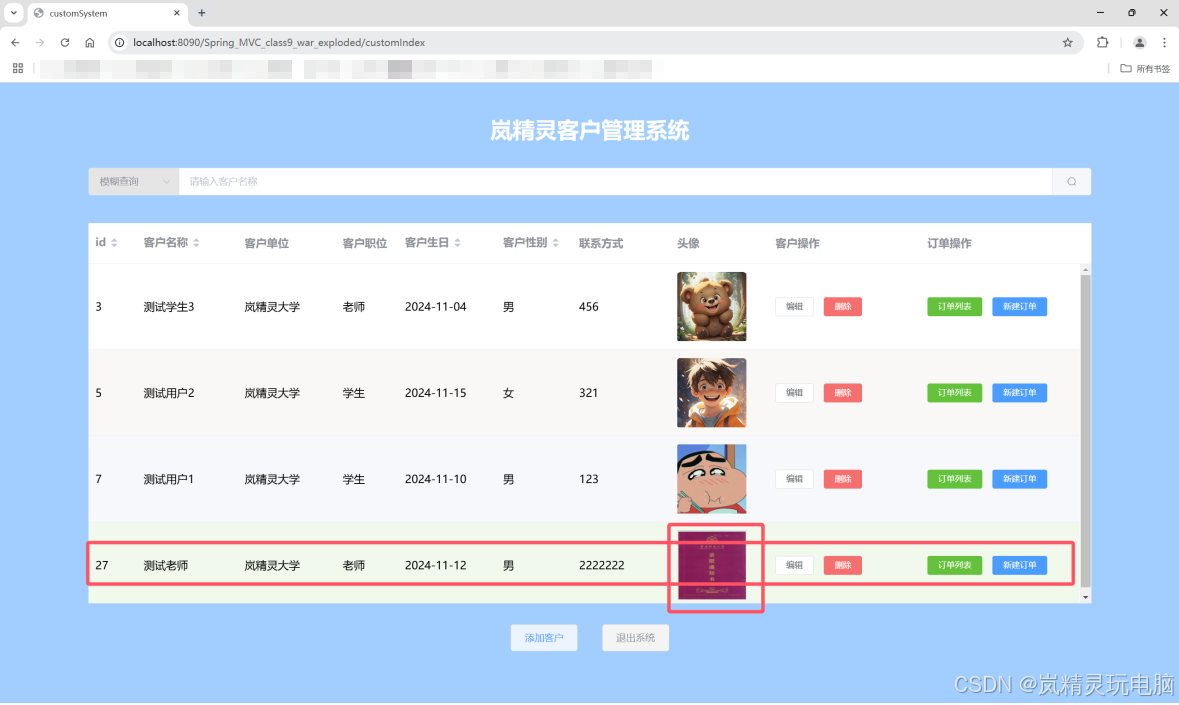

6.编辑客户信息
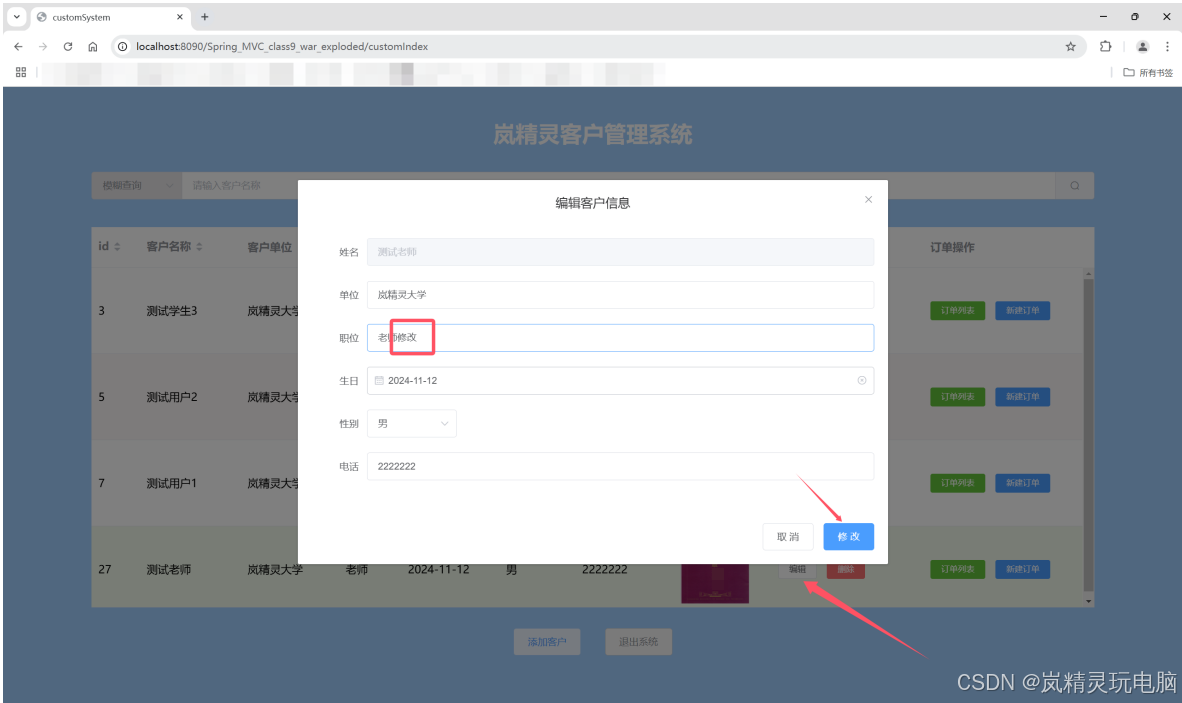


7.删除客户信息
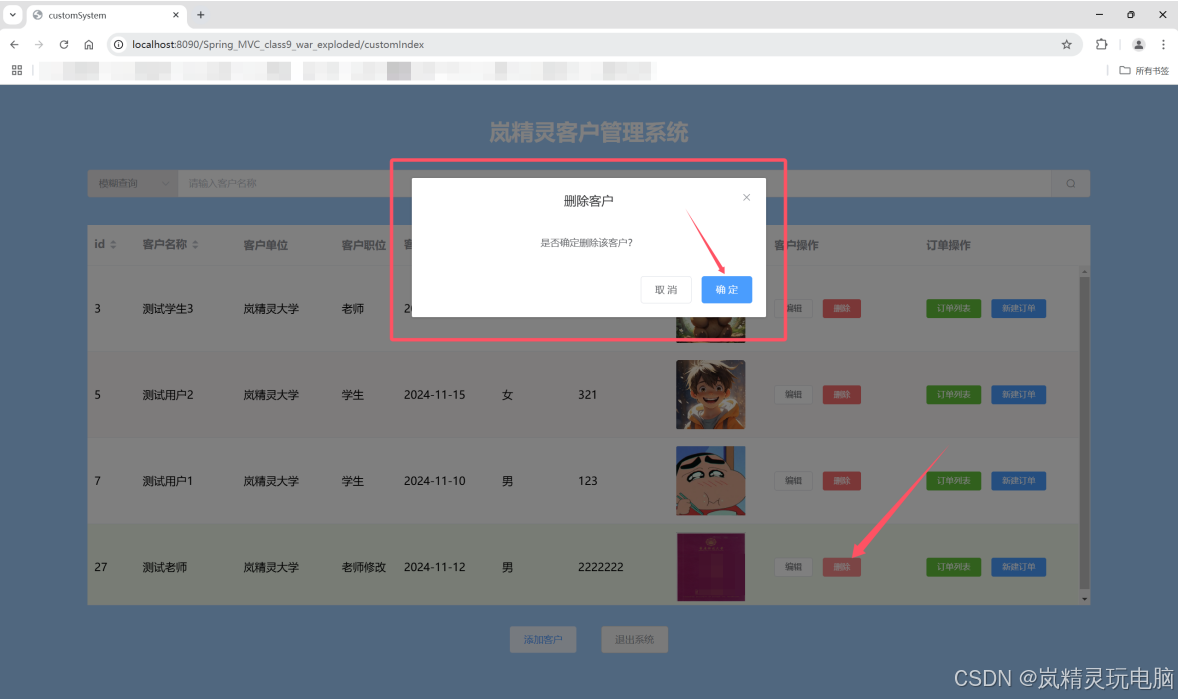
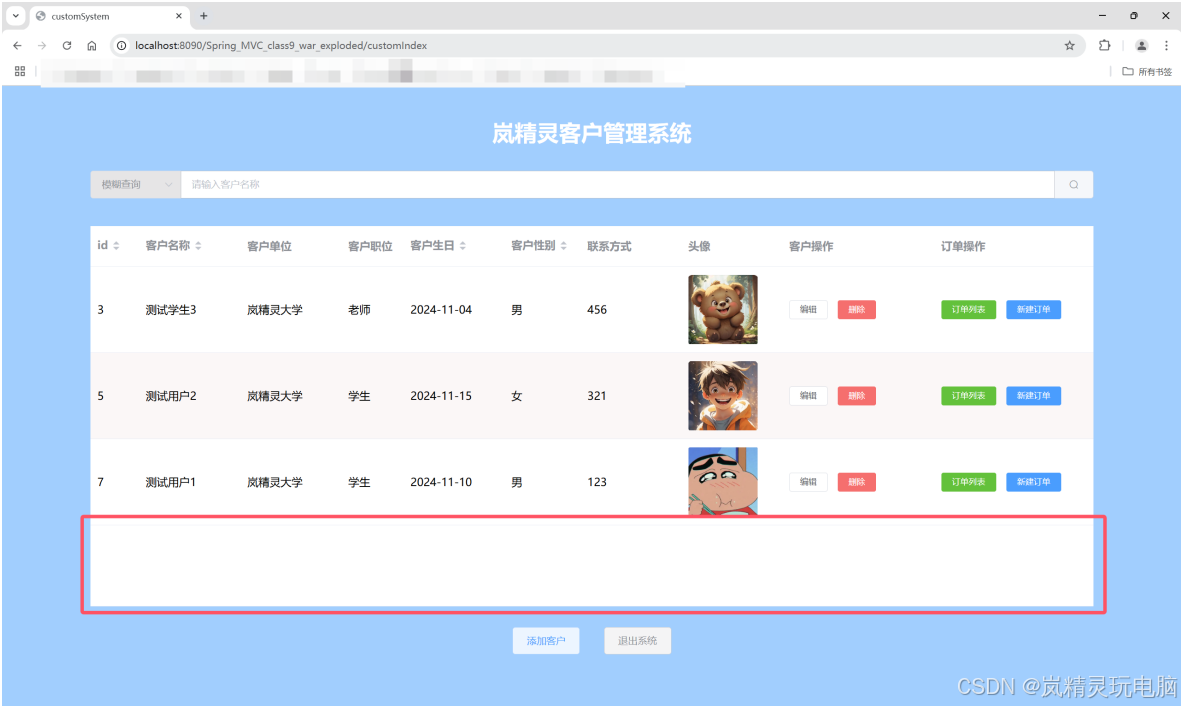

8.新增对应客户订单
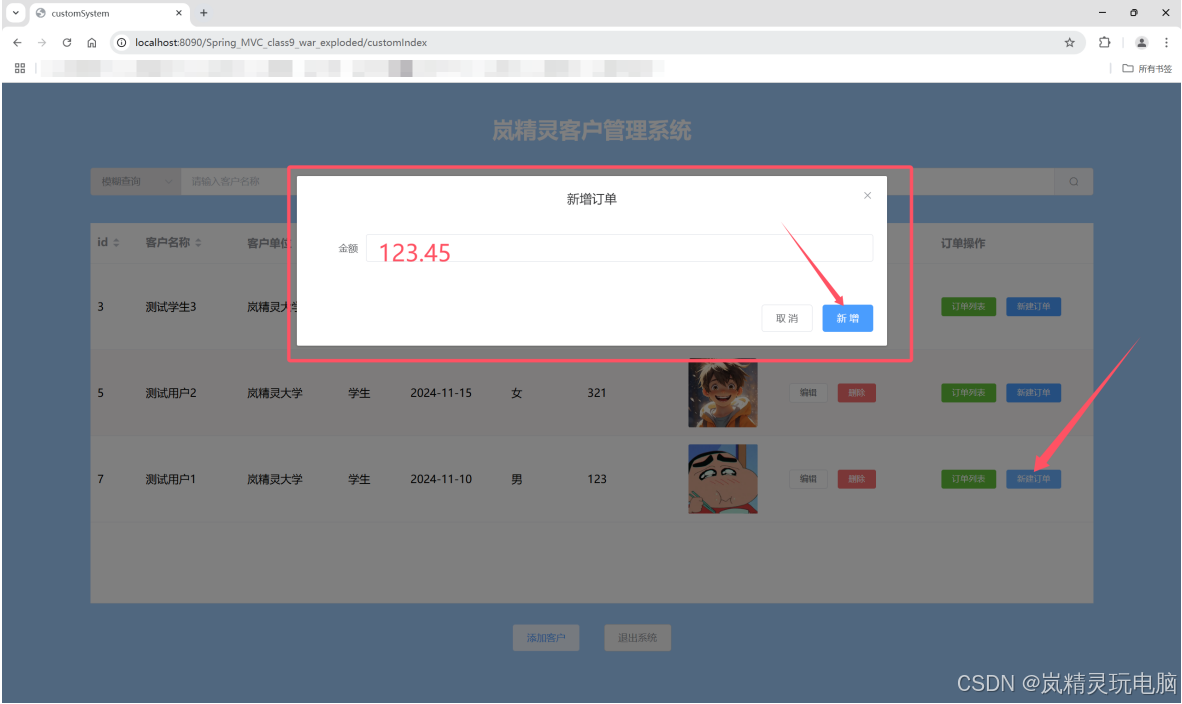
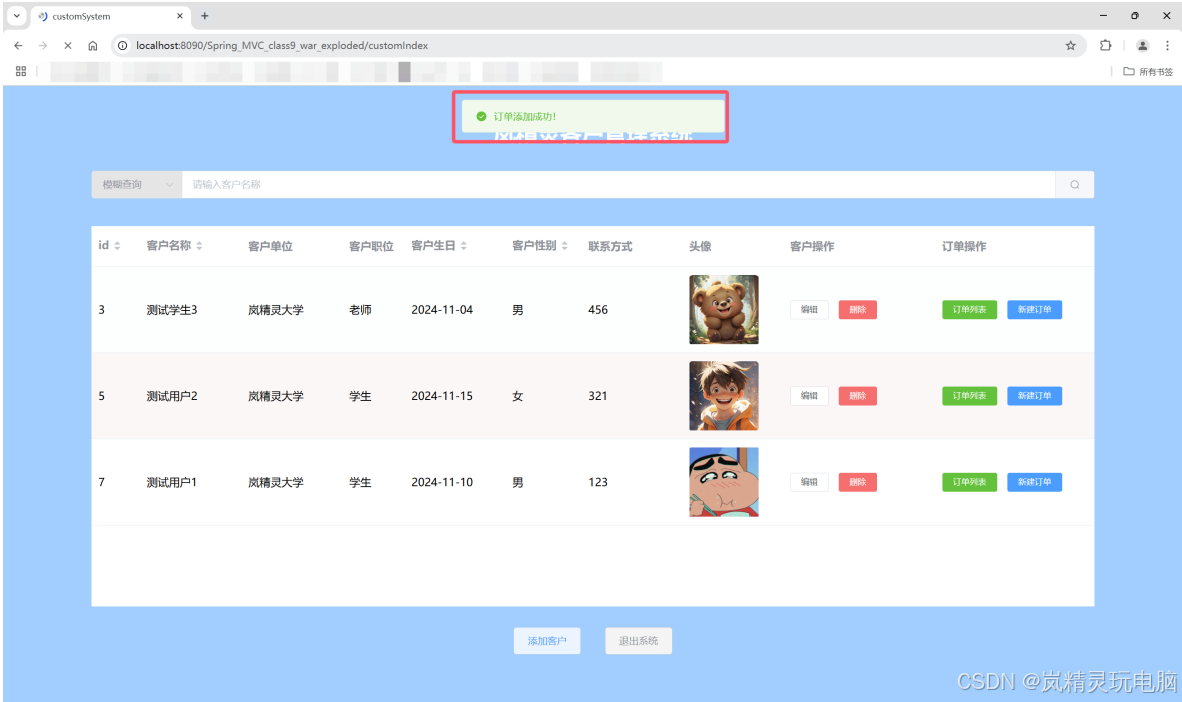
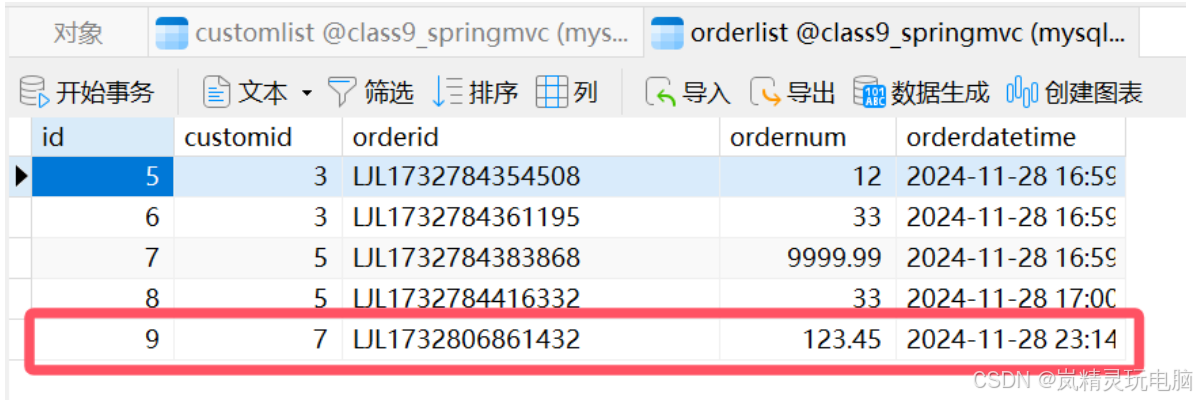
9.查询对应客户信息订单(可根据下单时间和订单金额进行排序)
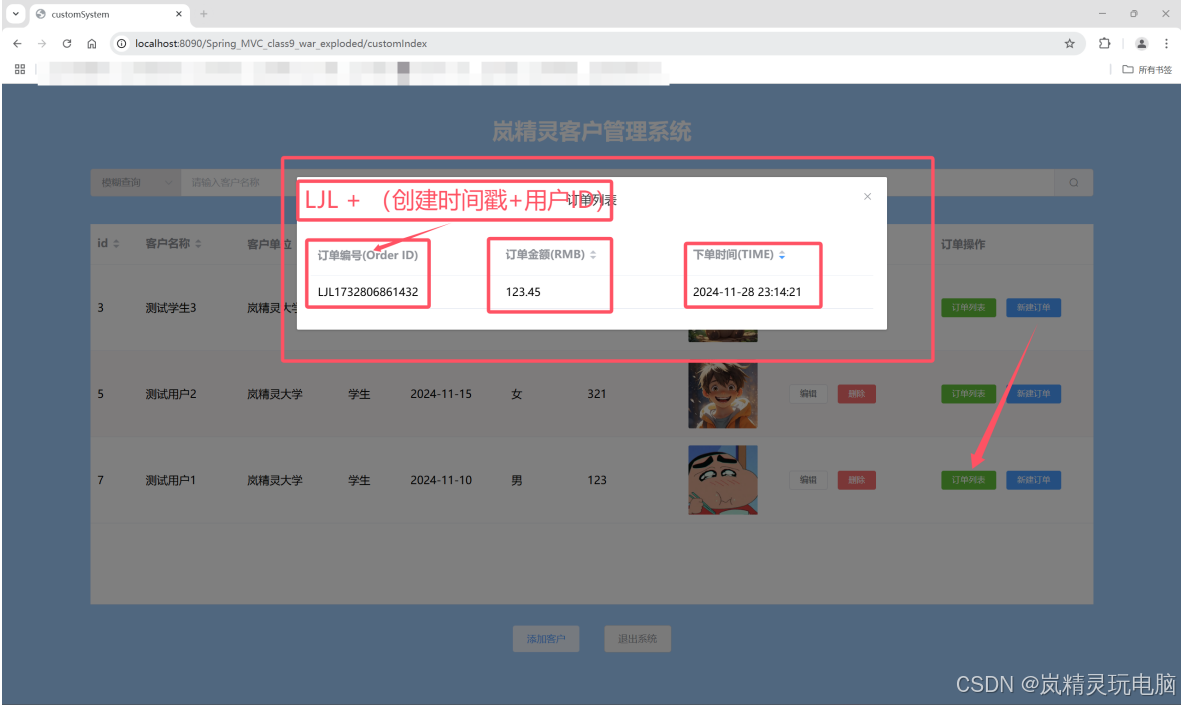