在这个教程中,您将学习如何使用Python和深度学习技术来调整图像的分辨率。我们将从基础的图像处理技术开始,逐步深入到使用预训练的深度学习模型进行图像超分辨率处理。
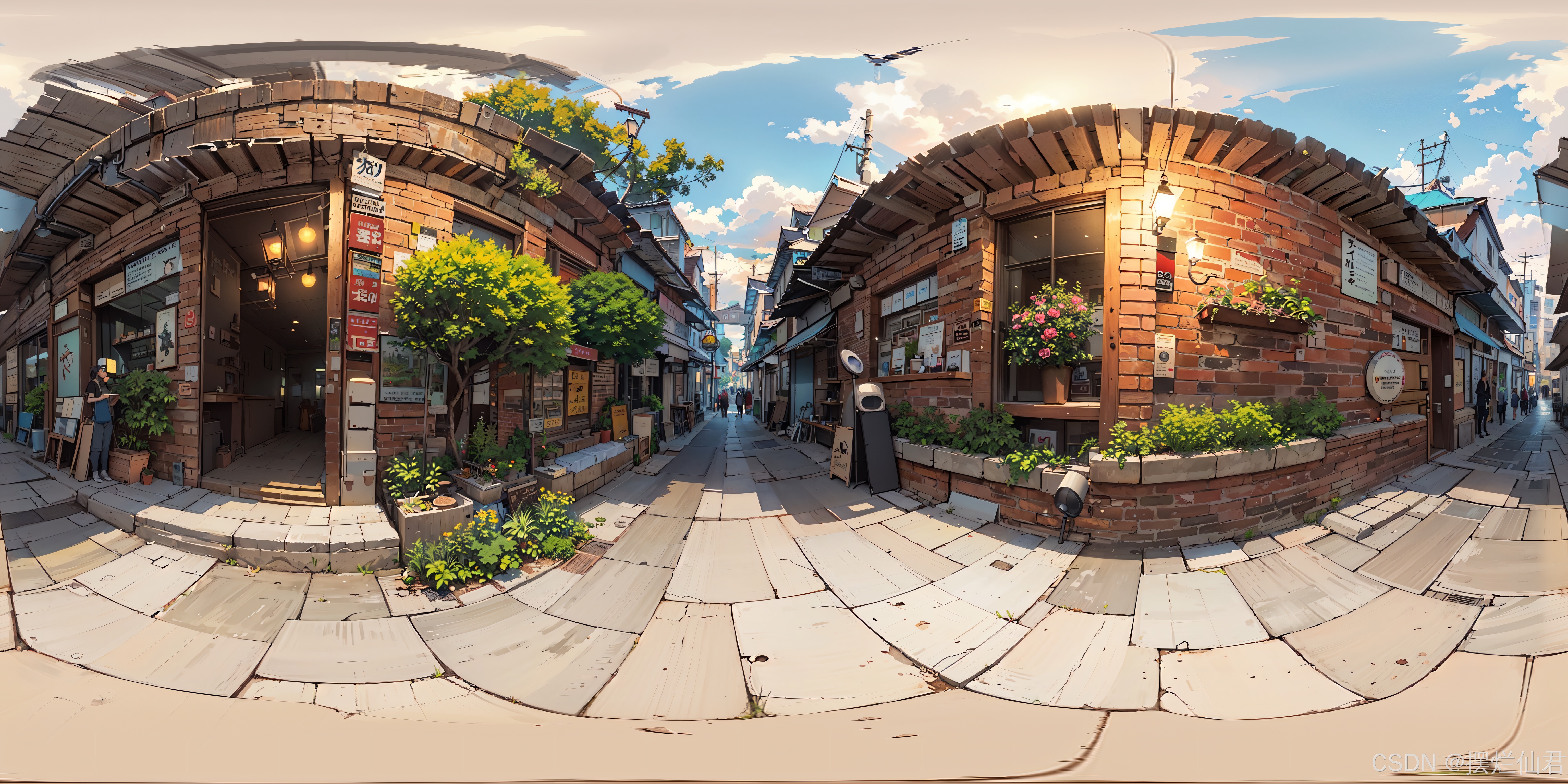
一、常规修改方法
1. 安装Pillow库
首先,你需要确保你的Python环境中已经安装了Pillow库。如果还没有安装,可以通过以下命令安装:
python
pip install Pillow
2. 导入Pillow库
在你的Python脚本中,导入Pillow库中的Image
模块:
python
from PIL import Image
3. 打开图片
使用Image.open()
函数打开你想要修改分辨率的图片:
img = Image.open("path_to_your_image.jpg")
请将"path_to_your_image.jpg"
替换为你的图片文件的实际路径。
4. 设置新的分辨率
定义新的宽度和高度。例如,如果你想将图片的尺寸加倍,可以这样做:
python
new_width = img.width * 2
new_height = img.height * 2
5. 修改图片分辨率
使用resize()
函数来修改图片的分辨率。你可以指定一个新的尺寸元组(宽度,高度),并选择一个滤镜来保持图片质量:
python
resized_img = img.resize((new_width, new_height), Image.ANTIALIAS)
Image.ANTIALIAS
是一个高质量的下采样滤镜,适用于放大图片。
6. 保存修改后的图片
最后,使用save()
函数保存修改后的图片:
python
resized_img.save("path_to_save_new_image.jpg")
将"path_to_save_new_image.jpg"
替换为你想要保存新图片的路径。
7.完整代码示例
将以上步骤合并,我们得到以下完整的代码示例:
python
from PIL import Image
# 打开图片
img = Image.open("path_to_your_image.jpg")
# 设置新的分辨率大小
new_width = img.width * 2 # 例如,将宽度放大两倍
new_height = img.height * 2 # 将高度放大两倍
# 使用ANTIALIAS滤镜来保持图片质量
resized_img = img.resize((new_width, new_height), Image.ANTIALIAS)
# 保存新的图片
resized_img.save("path_to_save_new_image.jpg")
8.注意事项
- 放大图片可能会导致图片质量下降,尤其是当放大比例较大时。
ANTIALIAS
滤镜可以帮助减少这种影响,但最好的结果通常需要更复杂的图像处理技术。 - 如果你需要缩小图片,也可以使用相同的方法,只需设置新的宽度和高度小于原始尺寸即可。
二、深度学习方法
使用深度学习来增加图片分辨率是一个相对复杂的过程,因为它涉及到神经网络模型的训练和应用。下面是一个简化的教程,介绍如何使用深度学习来增加图片分辨率,我们将使用Python和PyTorch框架,以及一个预训练的模型作为例子。
1. 安装PyTorch和相关库
首先,确保你已经安装了PyTorch。你可以访问PyTorch的官方网站来获取安装指令:PyTorch官网。安装PyTorch后,你还需要安装torchvision
和Pillow
库:
pip install torch torchvision Pillow
2. 导入必要的库
在你的Python脚本中,导入以下必要的库:
import torch
from torchvision import transforms
from PIL import Image
3. 加载预训练模型
我们将使用torchvision.models
中的一个预训练的模型。这里我们使用rationale
模型,它是一个用于图像超分辨率的模型。
import torchvision.models as models
# 加载预训练的模型
model = models.rationale(pretrained=True)
model.eval() # 设置为评估模式
4. 准备图片
将图片加载为PIL图像,然后转换为PyTorch张量:
# 打开图片
img = Image.open("path_to_your_image.jpg").convert('RGB')
# 转换为PyTorch张量
transform = transforms.Compose([
transforms.ToTensor(),
])
img_tensor = transform(img).unsqueeze(0) # 添加批次维度
5. 应用模型
将图片张量传递给模型,并获取输出:
# 应用模型
with torch.no_grad(): # 不需要计算梯度
output = model(img_tensor)
6. 保存结果
将模型的输出转换回PIL图像,并保存:
# 将输出转换回PIL图像
output = output.squeeze(0) # 移除批次维度
output_img = transforms.ToPILImage()(output)
# 保存图片
output_img.save("path_to_save_new_image.jpg")
7.完整代码示例
将以上步骤合并,我们得到以下完整的代码示例:
import torch
from torchvision import transforms, models
from PIL import Image
# 加载预训练的模型
model = models.rationale(pretrained=True)
model.eval()
# 打开图片并转换为张量
img = Image.open("path_to_your_image.jpg").convert('RGB')
transform = transforms.Compose([
transforms.ToTensor(),
])
img_tensor = transform(img).unsqueeze(0)
# 应用模型
with torch.no_grad():
output = model(img_tensor)
# 将输出转换回PIL图像并保存
output = output.squeeze(0)
output_img = transforms.ToPILImage()(output)
output_img.save("path_to_save_new_image.jpg")
8.注意事项
- 这个例子使用的是一个预训练的模型,它可能不是专门为超分辨率训练的,因此结果可能不如专门的超分辨率模型。
- 深度学习模型通常需要大量的计算资源,特别是在训练阶段。使用预训练模型可以减少这些需求。
- 这个例子没有涉及到模型的训练过程,因为训练一个深度学习模型是一个复杂且耗时的过程,需要大量的数据和计算资源。
三、更复杂情况的图像调整
1.方法讲解
在这个示例中,我们将使用torchvision.transforms
模块中的Resize
函数来调整图像大小。这个模块提供了多种插值方法,包括最近邻插值、双线性插值和双三次插值等。
python
import torch
from torchvision import transforms
from PIL import Image
# 打开图像
img_path = "path_to_your_image.jpg"
img = Image.open(img_path)
# 定义不同的插值方法
# 最近邻插值
nearest_transform = transforms.Compose([
transforms.Resize((256, 256), interpolation=transforms.InterpolationMode.NEAREST),
transforms.ToTensor()
])
# 双线性插值
bilinear_transform = transforms.Compose([
transforms.Resize((256, 256), interpolation=transforms.InterpolationMode.BILINEAR),
transforms.ToTensor()
])
# 双三次插值
bicubic_transform = transforms.Compose([
transforms.Resize((256, 256), interpolation=transforms.InterpolationMode.BICUBIC),
transforms.ToTensor()
])
# 应用插值方法并转换为张量
nearest_img = nearest_transform(img)
bilinear_img = bilinear_transform(img)
bicubic_img = bicubic_transform(img)
# 打印输出张量的形状以确认尺寸
print("Nearest Neighbor:", nearest_img.shape)
print("Bilinear:", bilinear_img.shape)
print("Bicubic:", bicubic_img.shape)
2.代码解释
- 图像加载 :使用
PIL.Image.open
函数加载图像。 - 定义插值方法 :使用
transforms.Resize
定义不同的插值方法,包括最近邻、双线性和双三次插值。 - 应用插值方法:将定义的插值方法应用到图像上,并转换为PyTorch张量。
四、超分辨率处理
以下是一个使用PyTorch和ESPCN(Efficient Sub-Pixel Convolutional Neural Network)模型的示例,这是一个轻量级的超分辨率模型,适合用于图像的放大和质量提升。
1.安装必要的库
首先,确保您已经安装了PyTorch和相关的库。如果还没有安装,可以使用以下命令:
pip install torch torchvision
2.ESPCN模型代码
以下是使用ESPCN模型进行图像超分辨率的完整代码:
python
import torch
from torch import nn
from torch.nn import functional as F
from torchvision import transforms
from PIL import Image
# 定义ESPCN模型
class ESPCN(nn.Module):
def __init__(self, num_filters=64, upscale_factor=2):
super(ESPCN, self).__init__()
self.conv1 = nn.Conv2d(3, num_filters, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(num_filters, num_filters, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(num_filters, 3 * upscale_factor ** 2, kernel_size=3, padding=1)
self.pixel_shuffle = nn.PixelShuffle(upscale_factor)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = self.pixel_shuffle(self.conv3(x))
return x
# 初始化模型
model = ESPCN(upscale_factor=2) # 假设我们要将图像放大2倍
model.load_state_dict(torch.load('espcn.pth')) # 加载预训练的模型权重
model.eval()
# 图像预处理
preprocess = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# 加载图像
img_path = "path_to_your_image.jpg"
img = Image.open(img_path)
img = img.resize((img.width // 2, img.height // 2)) # 首先将图像缩小2倍
img_tensor = preprocess(img).unsqueeze(0) # 增加批次维度
# 使用ESPCN模型进行超分辨率
with torch.no_grad():
sr_img = model(img_tensor).squeeze(0)
# 图像后处理
postprocess = transforms.Compose([
transforms.Normalize(mean=[-0.485/0.229, -0.456/0.224, -0.406/0.225], std=[1/0.229, 1/0.224, 1/0.225]),
transforms.ToPILImage()
])
# 将超分辨率后的图像张量转换回PIL图像并保存
sr_img = postprocess(sr_img)
sr_img.save("upsampled_image.jpg")
3.代码解释
- ESPCN模型定义:定义了一个简单的ESPCN模型,它包含三个卷积层和一个像素洗牌层(PixelShuffle)来实现上采样。
- 模型初始化和权重加载 :初始化ESPCN模型并加载预训练的权重。这里假设您已经有了一个预训练的权重文件
espcn.pth
。 - 图像预处理:定义了一个预处理流程,包括转换为张量和归一化。
- 图像加载和缩小:加载图像,并首先将其缩小2倍,这是因为ESPCN模型是用于将低分辨率图像放大的。
- 超分辨率:将预处理后的图像通过ESPCN模型进行超分辨率处理。
- 图像后处理:定义了一个后处理流程,包括反归一化和转换回PIL图像。
- 保存图像:将超分辨率后的图像保存到文件。
请注意,这个代码示例假设您已经有了一个预训练的ESPCN模型权重文件。如果您没有这个文件,您需要自己训练模型或者从网上找到相应的预训练权重。此外,您可能需要根据您的具体需求调整模型结构和参数。