题一
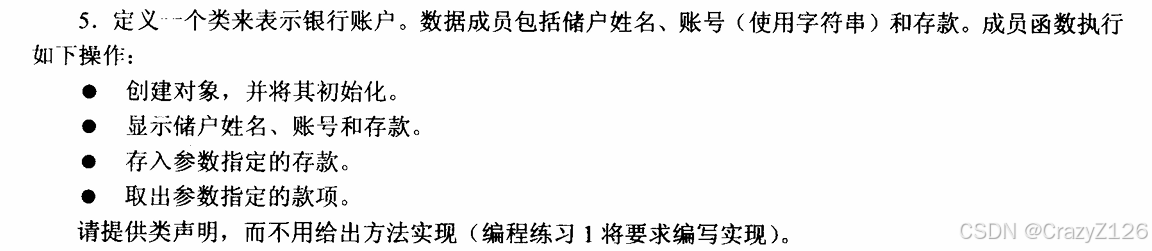

复制代码
#include <iostream>
#include <cstring>
using namespace std;
class bank_account
{
private:
char name[30];
char account_ID[30];
double balance;
public:
bank_account(char n[], char a[], double b);
void show();
void deposit(double amount);
void withdraw(double amount);
};
bank_account::bank_account(char n[], char a[], double b)
{
strcpy(name, n);
strcpy(account_ID, a);
balance = b;
}
void bank_account::show()
{
cout << "Name: " << name << endl;
cout << "Account ID: " << account_ID << endl;
cout << "Balance: " << balance << endl;
}
void bank_account::deposit(double amount)
{
balance += amount;
}
void bank_account::withdraw(double amount)
{
if (balance < amount)
{
cout << "Insufficient balance" << endl;
}
else
{
balance -= amount;
}
}
int main()
{
bank_account a("Alice", "123456", 1000);
a.show();
a.deposit(500);
a.show();
a.withdraw(2000);
a.show();
return 0;
}
题二
复制代码
#include <iostream>
#include <cstring>
using namespace std;
class Person
{
private:
static const int LIMIT = 25;
string lname;
char fname[LIMIT];
public:
Person()
{
lname = "";
fname[0] = '\0';
};
Person(const string &ln, const char *fn = "Heyyou");
void Show() const;
void FormalShow() const;
};
Person::Person(const string &ln, const char *fn)
{
lname = ln;
strcpy(fname, fn);
}
void Person::Show() const
{
cout << "name: " << fname << " " << lname << endl;
}
void Person::FormalShow() const
{
cout << "name: " << lname << ", " << fname << endl;
}
int main()
{
Person one;
Person two("Smythecraft");
Person three("Dimwiddy", "Sam");
one.Show();
cout << endl;
one.FormalShow();
cout << endl;
two.Show();
cout << endl;
two.FormalShow();
cout << endl;
three.Show();
cout << endl;
three.FormalShow();
}
题三
题四
题五
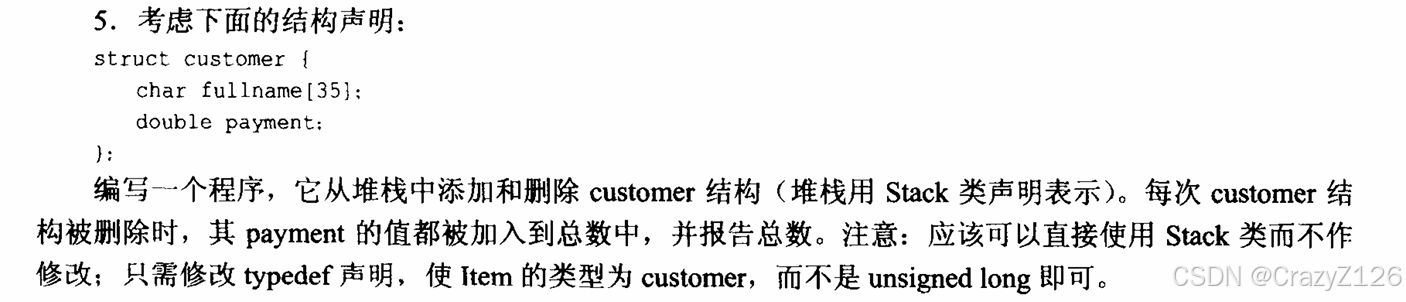
复制代码
#include <iostream>
using namespace std;
#include <cstring>
struct customer
{
char fullname[35];
double payment;
};
typedef customer Item;
class Stack
{
private:
int top;
enum
{
MAX = 3A
};
Item items[MAX];
double sum = 0;
public:
Stack();
bool isEmpty() const;
bool isFull() const;
bool push(const Item &item);
void pop(Item &item);
};
Stack::Stack()
{
top = 0;
}
bool Stack::isEmpty() const
{
return top == 0;
}
bool Stack::isFull() const
{
return top == MAX;
}
bool Stack::push(const Item &item)
{
if (top < MAX)
{
items[top] = item;
top++;
return true;
}
else
return false;
}
void Stack::pop(Item &item)
{
if (top > 0)
{
item = items[top - 1];
top--;
sum += item.payment;
cout << "Sum payment: " << sum << endl;
}
else
cout << "Stack is empty!" << endl;
}
int main()
{
Stack s;
customer c;
char ch;
cout << "Please enter A to add a customer order,\n"
<< "or P to pop a customer order,\n"
<< "or Q to quit: ";
while (cin >> ch && ch != 'Q')
//while (cin >> ch && toupper(ch) != 'Q')
while (cin.get() != '\n')
continue;
if (!isalpha(ch))
{
cout << "\a";
continue;
}
switch (ch)
{
case 'A':
case 'a':
cout << "Please enter customer name: ";
cin.getline(c.fullname, 35);
cout << "Please enter payment amount: ";
cin >> c.payment;
if (s.isFull())
cout << "Stack is full!" << endl;
else
s.push(c);
break;
case 'P':
case 'p':
if (s.isEmpty())
cout << "Stack is empty!" << endl;
else
{
s.pop(c);
cout << "Customer name: " << c.fullname << endl;
cout << "Payment amount: " << c.payment << endl;
}
break;
}
cout << "Please enter A to add a customer order,\n"
<< "or P to pop a customer order,\n"
<< "or Q to quit: ";
}
cout << "Bye!" << endl;
return 0;
}
题六
复制代码
#include <iostream>
using namespace std;
class Move
{
private:
double x, y;
public:
Move(double a = 0, double b = 0);
void showmove() const;
Move add(const Move &m) const;
Move reset(double x = 0, double y = 0);
};
Move::Move(double a, double b)
{
x = a;
y = b;
}
void Move::showmove() const
{
cout << "Position: (" << x << "," << y << ")" << endl;
}
Move Move::add(const Move &m) const
{
Move temp;
temp.x = x + m.x;
temp.y = y + m.y;
return temp;
}
Move Move::reset(double x, double y)
{
this->x = x;
this->y = y;
return *this;
}
int main()
{
Move m1(1, 2);
Move m2(3, 4);
Move m3 = m1.add(m2);
m3.showmove();
m3.reset(5, 6);
m3.showmove();
return 0;
}
题七
复制代码
#include <iostream>
#include <cstring>
using namespace std;
class plorg
{
private:
int CI;
char Name[20];
public:
plorg(int ci = 50, char name[] = "Plorg");
void Amend(int ci);
void Show() const;
};
plorg::plorg(int ci, char name[])
{
CI = ci;
strcpy(Name, name);
}
void plorg::Amend(int ci)
{
CI = ci;
}
void plorg::Show() const
{
cout << "CI: " << CI << endl;
cout << "Name: " << Name << endl;
}
int main()
{
plorg p1(100, "Plorg1");
p1.Show();
p1.Amend(200);
p1.Show();
plorg p2;
p2.Show();
return 0;
}
题八
复制代码
#include <iostream>
#include <functional> // 用于 std::function
// 定义一个模板类,用于实现简单的列表
template <typename T, size_t MaxSize>
class SimpleList
{
private:
T data[MaxSize]; // 固定大小的数组存储列表元素
size_t size; // 当前列表中的元素数量
public:
// 构造函数:创建空列表
SimpleList() : size(0) {}
// 判断列表是否为空
bool isEmpty() const
{
return size == 0;
}
// 判断列表是否为满
bool isFull() const
{
return size == MaxSize;
}
// 向列表中添加数据项
bool add(const T &item)
{
if (isFull())
{
std::cerr << "列表已满,无法添加数据项。" << std::endl;
return false;
}
data[size++] = item;
return true;
}
// 访问列表中的每个数据项并对其执行某种操作
void forEach(const std::function<void(T &)> &operation)
{
for (size_t i = 0; i < size; ++i)
{
operation(data[i]);
}
}
// 显示列表中的所有数据项
void display() const
{
for (size_t i = 0; i < size; ++i)
{
std::cout << data[i] << " ";
}
std::cout << std::endl;
}
// 返回列表中的数据项数量
size_t getSize() const
{
return size;
}
};
int main()
{
// 创建一个最多存储5个整数的列表
SimpleList<int, 5> myList;
// 判断列表是否为空
if (myList.isEmpty())
{
std::cout << "列表为空。" << std::endl;
}
// 向列表中添加数据项
myList.add(10);
myList.add(20);
myList.add(30);
// 显示列表内容
std::cout << "列表内容:";
myList.display();
// 判断列表是否为满
if (myList.isFull())
{
std::cout << "列表已满。" << std::endl;
}
else
{
std::cout << "列表未满。" << std::endl;
}
// 对每个数据项执行某种操作(例如加10)
myList.forEach([](int &x)
{ x += 10; });
// 显示修改后的列表内容
std::cout << "修改后的列表内容:";
myList.display();
return 0;
}