php+Mysql单页支持不同数据结构不同查询条件查搜多表实例
本来还要增加删 改 新增的,眼睛需要休息所以放弃后续制作(增删改代码已删或注释)
直接用可以用于自己多表查搜,界面还可以;有兴趣的可以自己二次开发成改删增
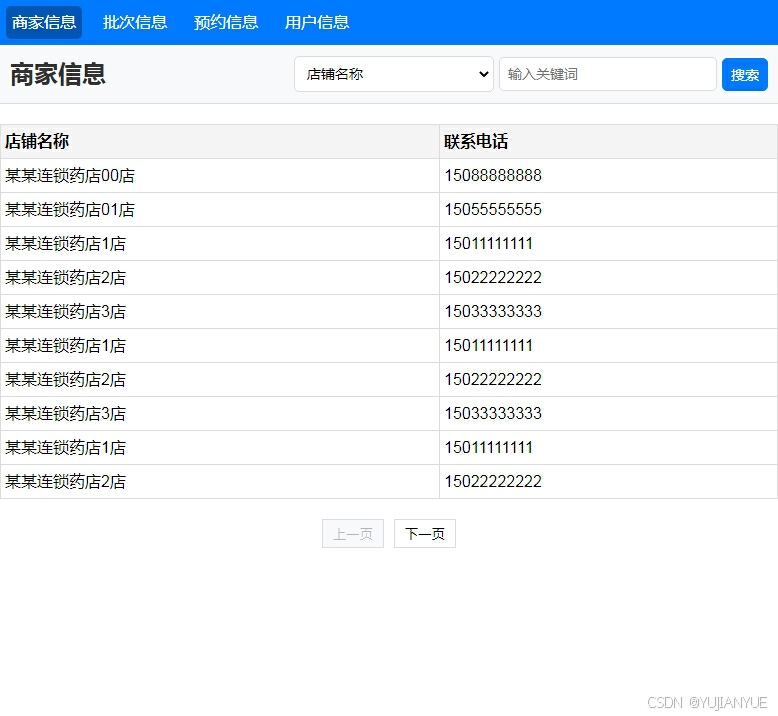
php
<?php
function connectDB() {
$host = "localhost";
$user = "yuyue";
$pass = "hMSdEpHsjex8S";
$dase = "yuyue";
$conn = new mysqli($host, $user, $pass, $dase);
if ($conn->connect_error) {
die(json_encode(['success' => false, 'message' => "连接失败:" . $conn->connect_error]));
}
return $conn;
}
$conn = connectDB();
// 表配置
$site = [
"kouzhao_shop" => [
"名称" => "商家信息",
"显示项" => ["id", "店铺名称", "店铺地址", "联系电话"],
"查询项" => ["店铺名称", "联系电话"],
"新增项" => ["店铺名称", "店铺地址", "联系电话"],
"可改项" => ["店铺名称", "店铺地址", "联系电话"]
],
"kouzhao_yuyue" => [
"名称" => "预约信息",
"显示项" => ["id", "姓名", "身份证号", "手机号码", "批次号"],
"查询项" => ["姓名", "身份证号", "手机号码"],
"新增项" => ["姓名", "身份证号", "手机号码"],
"可改项" => ["姓名", "身份证号", "手机号码"]
],
"kouzhao_pici" => [
"名称" => "批次信息",
"显示项" => ["id", "预约批次", "可约份数", "每份内容"],
"查询项" => ["预约批次", "可约份数", "每份内容"],
"新增项" => ["预约批次", "可约份数", "每份内容"],
"可改项" => ["预约批次", "可约份数", "每份内容"]
],
"kouzhao_user" => [
"名称" => "用户信息",
"显示项" => ["id", "用户名"],
"查询项" => ["用户名"],
"新增项" => ["用户名", "密码"],
"可改项" => ["密码"]
]
];
$act = isset($_GET['act']) ? $_GET['act'] : null;
if($act != ""){
header("Content-Type: application/json; charset=utf-8");
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Methods: POST");
// 获取请求数据
$postData = file_get_contents("php://input");
parse_str($postData, $params);
// 获取传入参数
$table = isset($params['table']) ? $params['table'] : null;
$action = isset($params['action']) ? $params['action'] : null;
$id = isset($params['id']) ? (int) $params['id'] : null;
$data = isset($params['data']) ? json_decode($params['data'], true) : [];
$keyword = isset($params['keyword']) ? $params['keyword'] : '';
$page = isset($params['page']) ? (int) $params['page'] : 1;
// 分页参数
$perPage = 10;
$offset = ($page - 1) * $perPage;
// 校验表是否存在
if (!isset($site[$table])) {
echo json_encode(["error" => "表[$table]不存在"]);
exit;
}
// 根据请求操作
switch ($action) {
case "list": // 获取数据列表
$queryFields = $site[$table]["查询项"];
$newddFields = $site[$table]["新增项"];
$columns = implode(",", $queryFields);
$where = "";
// 查询条件
if ($keyword !== '') {
$likeConditions = [];
foreach ($queryFields as $field) {
$likeConditions[] = "$field LIKE '%" . $conn->real_escape_string($keyword) . "%'";
}
$where = "WHERE " . implode(" OR ", $likeConditions);
}
// 获取总记录数
$countSql = "SELECT COUNT(*) AS total FROM $table $where";
$countResult = $conn->query($countSql);
$totalRows = $countResult->fetch_assoc()['total'];
// 查询数据
$sql = "SELECT $columns FROM $table $where LIMIT $offset, $perPage";
$result = $conn->query($sql);
if ($result) {
$rows = [];
while ($row = $result->fetch_assoc()) {
$rows[] = $row;
}
echo json_encode([
"data" => $rows,
"total" => $totalRows,
"perPage" => $perPage,
'filterFields' => $queryFields,
'newaddFields' => $newddFields
]);
} else {
echo json_encode(["error" => "查询失败: " . $conn->error]);
}
break;
/*
case "add": // 新增数据
$fields = $site[$table]["新增项"];
$fieldNames = implode(",", $fields);
$placeholders = implode(",", array_fill(0, count($fields), "?"));
$stmt = $conn->prepare("INSERT INTO $table ($fieldNames) VALUES ($placeholders)");
if ($stmt) {
$bindParams = [];
$bindTypes = str_repeat("s", count($fields));
$bindParams[] = &$bindTypes;
foreach ($fields as $field) {
$bindParams[] = &$data[$field];
}
call_user_func_array([$stmt, "bind_param"], $bindParams);
if ($stmt->execute()) {
echo json_encode(["success" => "新增成功"]);
} else {
echo json_encode(["error" => "新增失败: " . $stmt->error]);
}
$stmt->close();
} else {
echo json_encode(["error" => "准备语句失败: " . $conn->error]);
}
break;
case "edit": // 修改数据
$fields = $site[$table]["可改项"];
$setFields = implode(",", array_map(function ($field) {
return "$field = ?";
}, $fields));
$stmt = $conn->prepare("UPDATE $table SET $setFields WHERE id = ?");
if ($stmt) {
$bindParams = [];
$bindTypes = str_repeat("s", count($fields)) . "i";
$bindParams[] = &$bindTypes;
foreach ($fields as $field) {
$bindParams[] = &$data[$field];
}
$bindParams[] = &$id;
call_user_func_array([$stmt, "bind_param"], $bindParams);
if ($stmt->execute()) {
echo json_encode(["success" => "修改成功"]);
} else {
echo json_encode(["error" => "修改失败: " . $stmt->error]);
}
$stmt->close();
} else {
echo json_encode(["error" => "准备语句失败: " . $conn->error]);
}
break;
case "delete": // 删除数据
$stmt = $conn->prepare("DELETE FROM $table WHERE id = ?");
if ($stmt) {
$stmt->bind_param("i", $id);
if ($stmt->execute()) {
echo json_encode(["success" => "删除成功"]);
} else {
echo json_encode(["error" => "删除失败: " . $stmt->error]);
}
$stmt->close();
} else {
echo json_encode(["error" => "准备语句失败: " . $conn->error]);
}
break;
default:
echo json_encode(["error" => "无效的操作"]);
}
*/
$conn->close();
exit();
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>管理员多表数据管理</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
line-height: 1.6;
}
.nav-bar {
display: flex;
justify-content: flex-start;
background: #007bff;
padding: 6px;
}
.nav-bar a {
color: white;
text-decoration: none;
margin-right: 15px;
padding: 4px 6px;
border-radius: 5px;
}
.nav-bar a.active {
background: #0056b3;
}
.header {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
background: #f8f9fa;
border-bottom: 1px solid #ddd;
flex-wrap: wrap; /* 支持换行 */
}
b {
color: red;
}
.header h1 {
margin: 0;
font-size: 24px;
color: #333;
}
.actions {
display: flex;
align-items: center;
gap: 5px;
flex-wrap: wrap; /* 支持按钮换行 */
}
.actions select,
.actions input {
padding: 8px;
font-size: 14px;
border: 1px solid #ddd;
border-radius: 5px;
width: 200px;
}
.actions button {
padding: 6px 8px;
font-size: 14px;
color: white;
background-color: #007bff;
border: 1px solid #007bff;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.actions button:hover {
background-color: #0056b3;
}
.actions button:active {
background-color: #004085;
}
@media (max-width: 768px) {
.header {
flex-direction: column; /* 小屏幕下调整布局 */
align-items: flex-start;
gap: 15px;
}
.actions {
width: 100%; /* 使操作区域独占一行 */
}
.actions select,
.actions input {
width: 100%; /* 输入框与选择框全宽 */
}
.actions button {
width: 100%; /* 按钮全宽 */
}
}
table {
width: 100%;
border-collapse: collapse;
margin: 20px 0;
}
table th, table td {
border: 1px solid #ddd;
padding: 4px;
text-align: left;
}
table th {
background: #f4f4f4;
}
.form-container {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: white;
border: 1px solid #ddd;
padding: 20px 0;
z-index: 1000;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.overlay {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.5);
z-index: 999;
}
.pagination {
display: flex;
justify-content: center;
margin: 20px 0;
}
.pagination button {
padding: 5px 10px;
margin: 0 5px;
border: 1px solid #ddd;
background: white;
cursor: pointer;
}
.pagination button.disabled {
background: #f8f9fa;
cursor: not-allowed;
}
</style>
</head>
<body>
<!-- 顶部导航 -->
<div class="nav-bar" id="nav-bar">
<!-- 动态生成的表导航 -->
</div>
<!-- 主内容 -->
<div class="header">
<h1 id="table-title">数据管理</h1>
<div class="actions">
<select id="filter-field"></select>
<input type="text" id="filter-keyword" placeholder="输入关键词">
<button onclick="fetchData()">搜索</button>
</div>
</div>
<!-- 数据表 -->
<table id="data-table">
<thead></thead>
<tbody></tbody>
</table>
<!-- 分页 -->
<div class="pagination" id="pagination"></div>
<!-- 遮罩层 -->
<div class="overlay" id="overlay"></div>
<script>
let currentTable = "";
let currentPage = 1;
let totalPages = 1;
// 配置导航
const site = {
"kouzhao_shop": "商家信息",
"kouzhao_pici": "批次信息",
"kouzhao_yuyue": "预约信息",
"kouzhao_user": "用户信息"
};
// 初始化页面
window.onload = function () {
initNavBar();
loadTable(Object.keys(site)[0]);
};
// 初始化导航栏
function initNavBar() {
const navBar = document.getElementById("nav-bar");
navBar.innerHTML = "";
for (let table in site) {
const link = document.createElement("a");
link.textContent = site[table];
link.href = "#";
link.onclick = () => loadTable(table);
link.id = `nav-${table}`;
navBar.appendChild(link);
}
}
// 加载表格
function loadTable(table) {
currentTable = table;
currentPage = 1;
document.getElementById("table-title").textContent = site[table];
document.querySelectorAll(".nav-bar a").forEach(a => a.classList.remove("active"));
document.getElementById(`nav-${table}`).classList.add("active");
fetchData();
}
// 获取数据
function fetchData() {
const filterField = document.getElementById("filter-field").value || "";
const filterKeyword = document.getElementById("filter-keyword").value || "";
const url = "?act=do";
const params = {
table: currentTable,
action: "list",
keyword: filterKeyword,
page: currentPage
};
postRequest(url, params, (response) => {
renderTable(response.data);
renderPagination(response.total, response.perPage);
updateFilterFields(response.filterFields);
});
}
function updateFilterFields(fields) {
const filterFieldSelect = document.getElementById("filter-field");
filterFieldSelect.innerHTML = ""; // 清空现有选项
if (fields && fields.length > 0) {
fields.forEach(field => {
const option = document.createElement("option");
option.value = field;
option.textContent = field;
filterFieldSelect.appendChild(option);
});
}
}
// 渲染表格
function renderTable(data) {
const filterField = document.getElementById("filter-field").value || "";
const filterKeyword = document.getElementById("filter-keyword").value || "";
const table = document.getElementById("data-table");
table.tHead.innerHTML = "";
table.tBodies[0].innerHTML = "";
if (!data || data.length === 0) {
const row = document.createElement("tr");
const cell = document.createElement("td");
cell.colSpan = 5;
cell.innerHTML = "暂无<b>"+filterField+"</b>=<b>"+filterKeyword+"</b>数据";
row.appendChild(cell);
table.tBodies[0].appendChild(row);
return;
}
const fields = Object.keys(data[0]);
const headRow = document.createElement("tr");
fields.forEach(field => {
const th = document.createElement("th");
th.textContent = field;
headRow.appendChild(th);
});
table.tHead.appendChild(headRow);
data.forEach(row => {
const tr = document.createElement("tr");
fields.forEach(field => {
const td = document.createElement("td");
td.textContent = row[field];
tr.appendChild(td);
});
table.tBodies[0].appendChild(tr);
});
}
// 渲染分页
function renderPagination(total, perPage) {
totalPages = Math.ceil(total / perPage);
const pagination = document.getElementById("pagination");
pagination.innerHTML = "";
const addButton = (text, disabled, onClick) => {
const button = document.createElement("button");
button.textContent = text;
button.disabled = disabled;
button.className = disabled ? "disabled" : "";
button.onclick = onClick;
pagination.appendChild(button);
};
addButton("上一页", currentPage === 1, () => changePage(currentPage - 1));
addButton("下一页", currentPage === totalPages, () => changePage(currentPage + 1));
}
// 改变页码
function changePage(page) {
if (page > 0 && page <= totalPages) {
currentPage = page;
fetchData();
}
}
// 通用POST请求
function postRequest(url, params, callback) {
const xhr = new XMLHttpRequest();
xhr.open("POST", url);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onload = () => callback(JSON.parse(xhr.responseText));
xhr.send(Object.keys(params).map(key => `${key}=${encodeURIComponent(params[key])}`).join("&"));
}
</script>
</body>
</html>