*************
C++
topic: 712. 两个字符串的最小ASCII删除和 - 力扣(LeetCode)
*************
Before check the topic, I have retuened my new-buy keyboard yestarday cuz my wrist hurts, so maybe I need a low-profile keyboard.
check the topic:
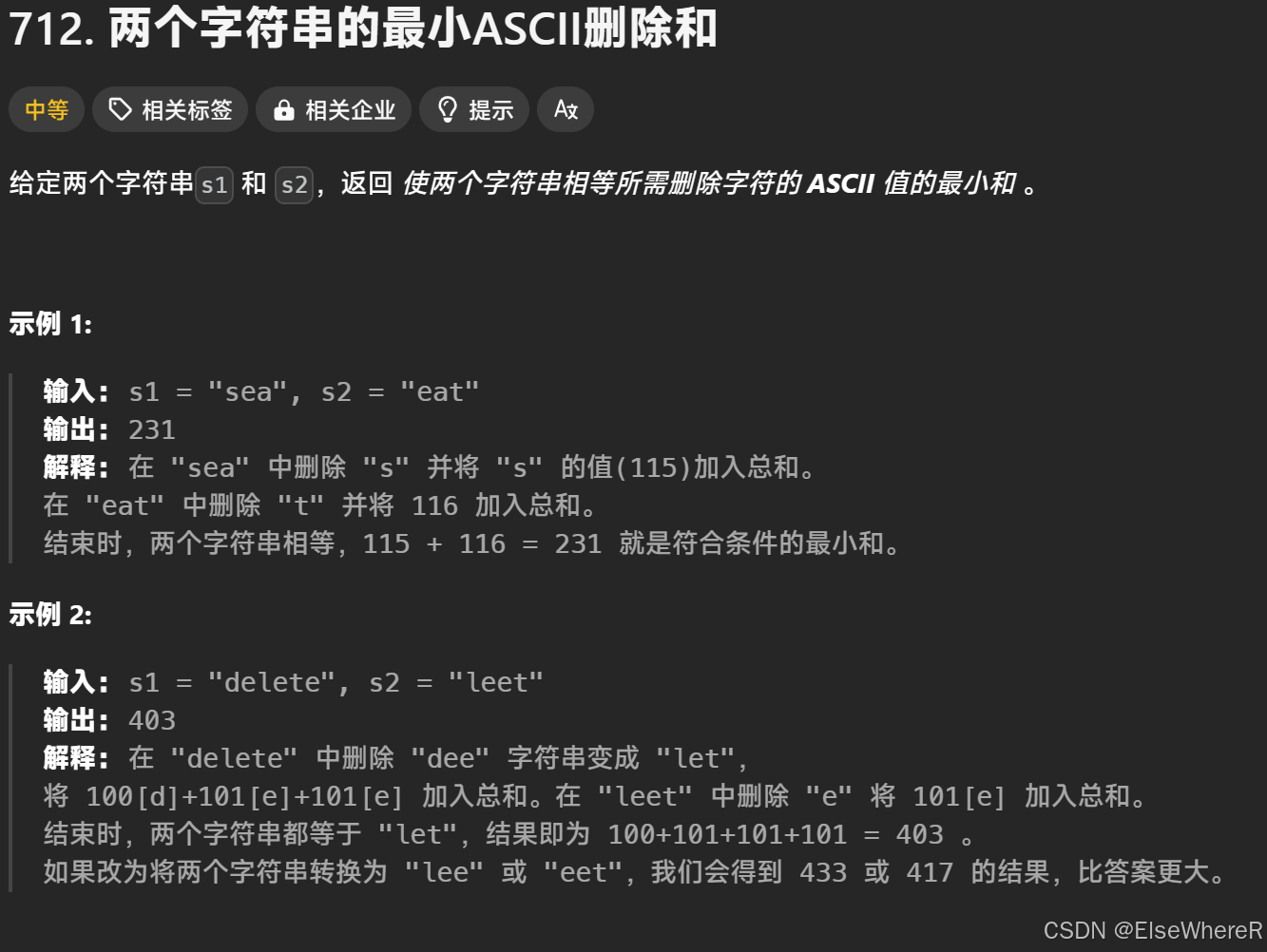
first I donnot know what is ASCII. I kook for some informations and FYI:
ASCII(American Standard Code for Information Interchange,美国信息交换标准代码)是一种字符编码标准,用于表示文本在计算机、通信设备和其它电子设备中的表示形式。ASCII码使用7位二进制数来表示128个不同的字符,包括大小写英文字母、数字0-9以及一些控制字符和标点符号。ASCII码的前32个字符(0-31)是控制字符,用于控制文本的显示和传输,比如换行(LF,ASCII码为10)和回车(CR,ASCII码为13)。剩下的96个字符(32-127)是可打印字符,包括我们常见的字母和数字。
You see, the topic in the other ways says is we have two strings, s1 and s 2, insert or erase the elements in s1, the new one eauals s2, find the least step.
Search the familiar project is my style. U gays guess what, I do find one which is
1143. 最长公共子序列 - 力扣(LeetCode), Longest Common Subsequence, LCS for short. That makes the topic eazy. Here is the spark:
-
find the LCS of s1 and s2;
-
Sum the ASCII values of the characters in string s1 that are not part of the LCS.
-
Sum the ASCII values of the characters in string s2 that are not part of the LCS.
-
Sum the values.
That is the answer.
Have an eye on how to solve 1143. 最长公共子序列 - 力扣(LeetCode), the soal is as follow
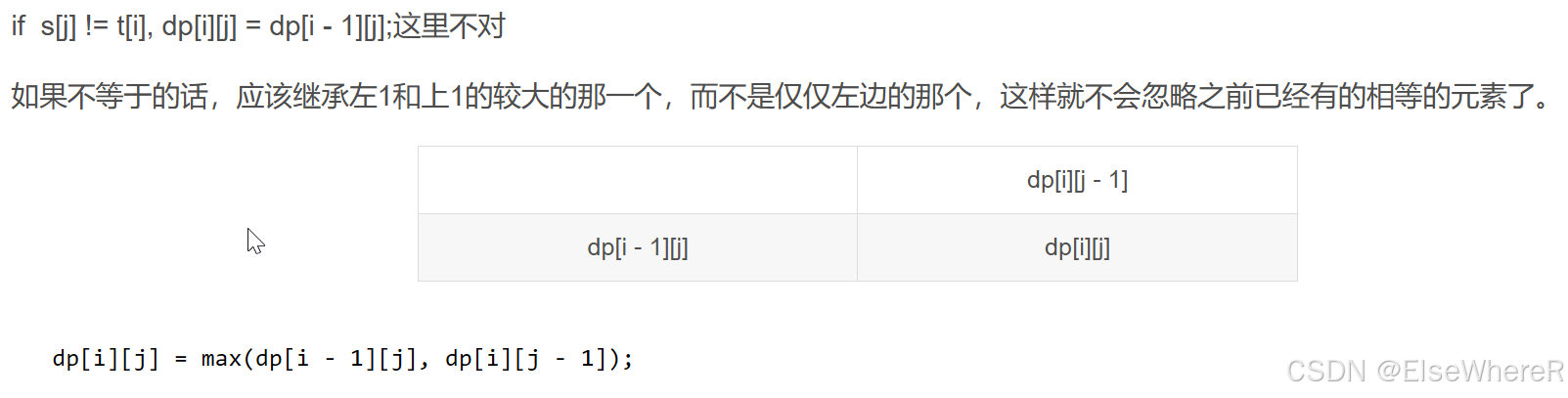
Asshole-eye always superise me. and the code is
cpp
class Solution {
public:
int longestCommonSubsequence(string text1, string text2) {
// write the follows without hesitation
int m = text1.size();
int n = text2.size();
vector<vector<int>> dp(m + 1, vector(n + 1, 0));
// main code
for (int i = 1; i <= m; i++){
for (int j = 1; j <= n; j++){
if (text1[i-1] == text2[j-1]){ // special attention to the number
dp[i][j] = dp[i-1][j-1] + 1;
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]); // more ez formula
}
}
}
return dp[m][n];
}
};
But in this case, use the soal again,
cpp
class Solution {
public:
// sum LCS's ASCII
int getLCSAsciiSum(string s1, string s2) {
int m = s1.size();
int n = s2.size();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, 0));
// main code
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1[i - 1] == s2[j - 1]) { // special attention to the number
dp[i][j] = dp[i - 1][j - 1] + int(s1[i - 1]);
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]); // more ez formula
}
}
}
return dp[m][n];
}
};
then how to sum the ASCII? easy way to sum:
cpp
class Solution {
public:
// sum LCS's ASCII
int getLCSAsciiSum(string s1, string s2) {
int m = s1.size();
int n = s2.size();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, 0));
// main code
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1[i - 1] == s2[j - 1]) { // special attention to the number
dp[i][j] = dp[i - 1][j - 1] + int(s1[i - 1]);
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]); // more ez formula
}
}
}
return dp[m][n];
}
// sum ASCII
int getAsciiSum(string s) {
int sum = 0;
for (char c : s) {
sum += int(c);
}
return sum;
}
};
another spark is,
-
Sum the ASCII values of the characters in string s1 that are not part of the LCS.
-
Sum the ASCII values of the characters in string s2 that are not part of the LCS.
-
Sum the values.
use math to caculate is
sum = sum[s1] + sum[s2] - 2 * sum[LCS]
cpp
class Solution {
public:
// sum LCS's ASCII
int getLCSAsciiSum(string s1, string s2) {
int m = s1.size();
int n = s2.size();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, 0));
// main code
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1[i - 1] == s2[j - 1]) { // special attention to the number
dp[i][j] = dp[i - 1][j - 1] + int(s1[i - 1]);
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]); // more ez formula
}
}
}
return dp[m][n];
}
// sum ASCII
int getAsciiSum(string s) {
int sum = 0;
for (char c : s) {
sum += int(c);
}
return sum;
}
// main code
int minimumDeleteSum(string s1, string s2) {
int sum1 = getAsciiSum(s1);
int sum2 = getAsciiSum(s2);
int sum_LCS = getLCSAsciiSum(s1, s2);
return sum1 + sum2 - 2 * sum_LCS;
}
};
Here we go.