前言
迭代器模式可以使用统一的接口来遍历不同类型的集合对象,而不需要关心其内部的具体实现。
代码
csharp
//迭代器接口
public interface Iterator
{
bool HashNext();
object Next();
}
//集合接口
public interface Collection
{
Iterator CreateIterator();
}
//元素迭代器
public class ElementIterator : Iterator
{
private string[] elements;
private int index = 0;
public ElementIterator(string[] elements)
{
this.elements = elements;
}
public bool HashNext()
{
return index < elements.Length;
}
public object Next()
{
if (index < elements.Length)
{
return elements[index++];
}
return null;
}
}
//元素集合
public class ElementCollection : Collection
{
private string[] elements;
public ElementCollection(string[] elements)
{
this.elements = elements;
}
public Iterator CreateIterator()
{
return new ElementIterator(elements);
}
}
/*
* 行为型模式:Behavioral Pattern
* 迭代器模式:Iterator Pattern
*/
internal class Program
{
static void Main(string[] args)
{
string[] elements = { "A", "B", "C", "D", "E", "F",};
Collection collection = new ElementCollection(elements);
Iterator iterator = collection.CreateIterator();
while(iterator.HashNext())
{
Console.WriteLine(iterator.Next().ToString());
}
Console.ReadLine();
}
}
结果
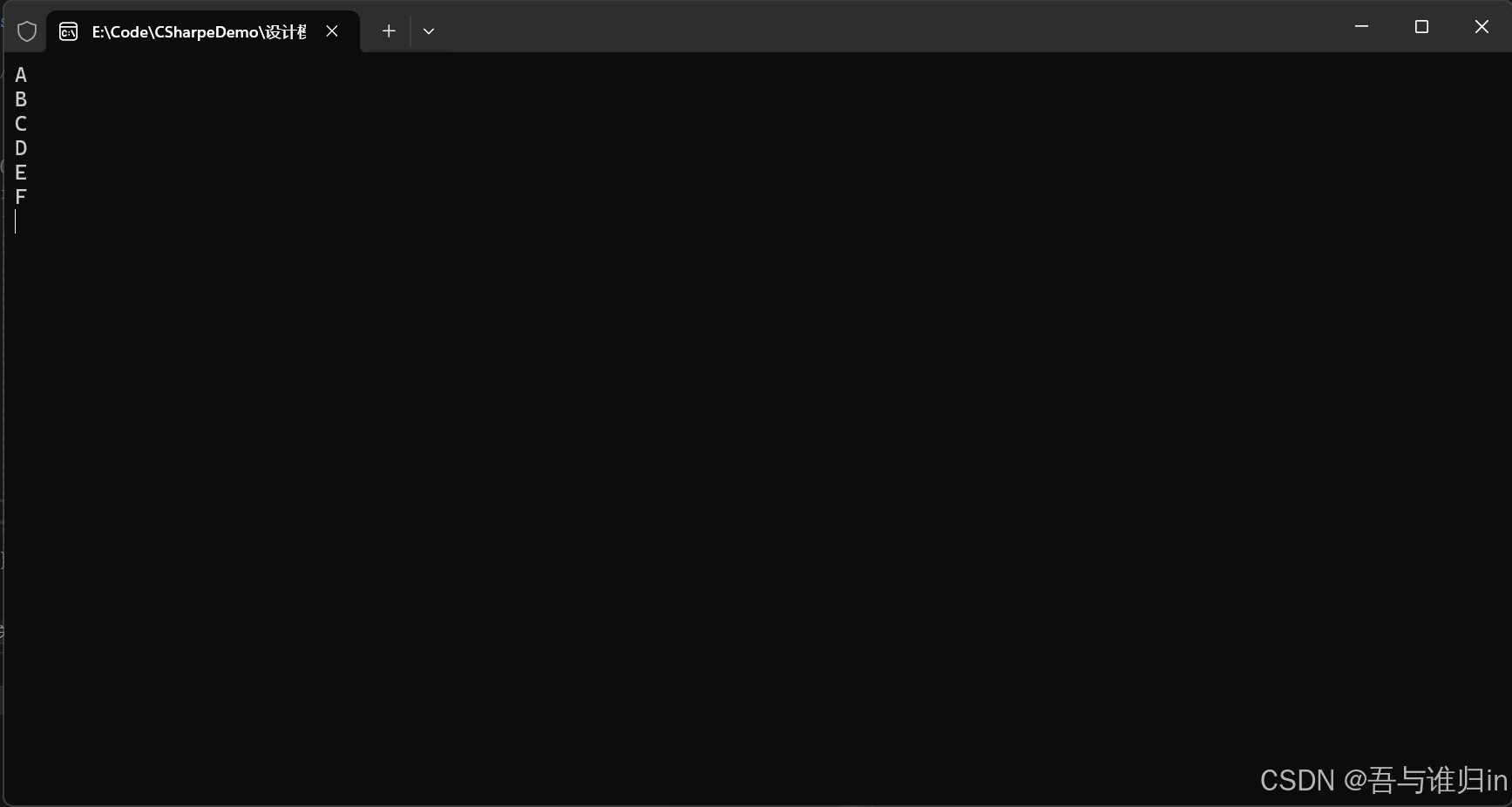