多态数组
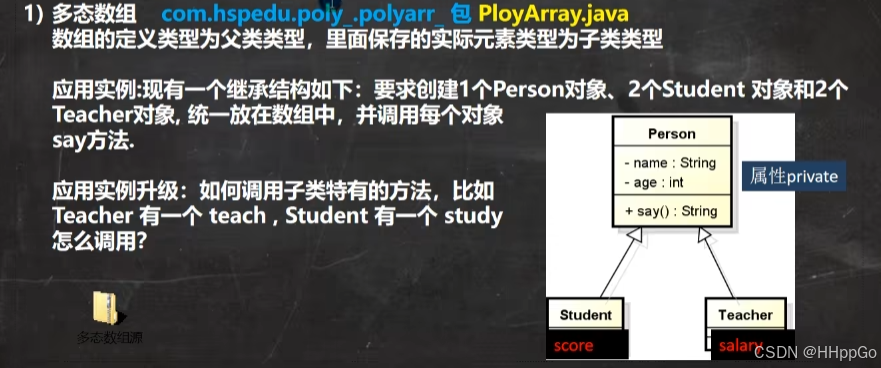
应用实例:现有一个继承结构如下:要求创建 1 个 Person 对象、2 个 Student 对象和 2 个 Teacher 对象, 统一放在数组中,并调用每个对象
代码
Person类
java
package com.hspedu.poly_.polyarr_;
import javax.swing.*;
/**
* @author:寰愬悏瓒�
* @date:2024/12/14 version:1.0
*/
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String say() {
return name +"\t" + age;
}
}
Student类
java
package com.hspedu.poly_.polyarr_;
import com.hspedu.poly_.polyarr_.Person;
/**
* @author:寰愬悏瓒�
* @date:2024/12/14 version:1.0
*/
public class Student extends Person {
private double score;
public Student(String name, int age, double score) {
super(name, age);
this.score = score;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
// 重写父类 say
@Override
public String say() {
return "学生 " + super.say() + " score = " + score;
}
}
Teacher类
java
package com.hspedu.poly_.polyarr_;
public class Teacher extends Person {
private double salary;
public Teacher(String name, int age, double salary) {
super(name, age);
this.salary = salary;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
// 重写父类的say() 方法
@Override
public String say() {
return "老师 " + super.say() + " salary= " + salary;
}
}
PloyArray类
java
package com.hspedu.poly_.polyarr_;
/**
* @author:寰愬悏瓒�
* @date:2024/12/14 version:1.0
*/
public class PloyArray {
public static void main(String[] args) {
/*
* 应用实例:现有一个继承结构如下:要求创建 1 个 Person 对象、
* 2 个 Student 对象和 2 个 Teacher 对象, 统一放在数组
中,并调用每个对象
*/
Person[] persons = new Person[5];
persons[0] = new Person("jack",20);
persons[1] = new Student("jack",18,100);
persons[2] = new Student("smith",19, 30.1);
persons[3] = new Teacher("scott",30,20000);
persons[4] = new Teacher("king",50,25000);
// 循环遍历多态数组,调用say
for(int i = 0; i < persons.length; i++ ){
// person[i] 编译类型是 Person, 运行类型是 根据实际情况由JVM来判断
System.out.println(persons[i].say());//动态绑定机制
}
}
}
应用实例升级:
如何调用子类特有的方法,比如
Teacher 有一个 teach , Student 有一个 study
怎么调用?
Teacher类增加teach()方法
java
// 特有的方法
public void teach() {
System.out.println("老师 " + getName() +" 正在上课");
}
Student类增加study()方法
java
// 特有方法
public void study() {
System.out.println("学生 " + getName() + " 正在学java");
}
修改PloyArray类
java
package com.hspedu.poly_.polyarr_;
public class PloyArray {
public static void main(String[] args) {
/*
* 应用实例:现有一个继承结构如下:要求创建 1 个 Person 对象、
* 2 个 Student 对象和 2 个 Teacher 对象, 统一放在数组
中,并调用每个对象
*/
Person[] persons = new Person[5];
persons[0] = new Person("jack",20);
persons[1] = new Student("mary",18,100);
persons[2] = new Student("smith",19, 30.1);
persons[3] = new Teacher("scott",30,20000);
persons[4] = new Teacher("king",50,25000);
// 循环遍历多态数组,调用say
for(int i = 0; i < persons.length; i++ ){
// person[i] 编译类型是 Person, 运行类型是 根据实际情况由JVM来判断
System.out.println(persons[i].say());//动态绑定机制
if(persons[i] instanceof Student) { // 判断person[i] 的运行类型是不是 Student
Student student = (Student)persons[i]; //向下转型
student.study();
// 也可以使用一条语句 ((Student)persons[i]).study();
} else if(persons[i] instanceof Teacher) {
Teacher teacher = (Teacher)persons[i];
teacher.teach();
} else if(persons[i] instanceof Person){
} else {
System.out.println("你的类型有问题,请自己检查...");
}
}
}
}
多态参数
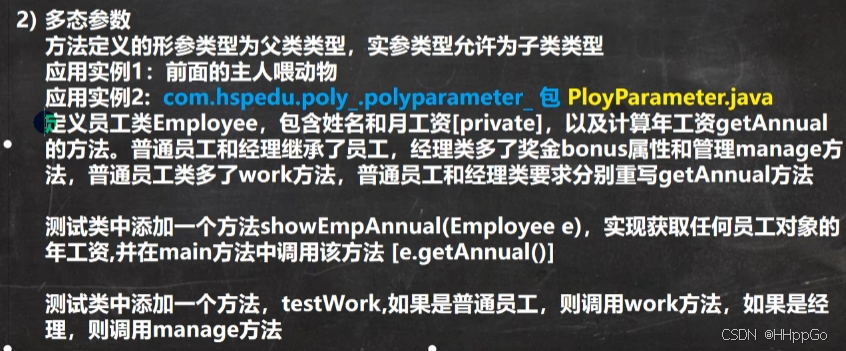
代码:
Employee类
java
package com.hspedu.poly_.polyparameter_;
public class Employee {
private String name;
private double salary;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
//得到年工资的方法
public double getAnnual() {
return 12*salary;
}
}
Manager类
java
package com.hspedu.poly_.polyparameter_;
public class Manager extends Employee {
private double bonus;
public Manager(String name, double salary, double bonus) {
super(name, salary);
this.bonus = bonus;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
public void manage(){
System.out.println("经理 " + getName() + " is manageing");
}
//重写获取年薪方法
@Override
public double getAnnual() {
return super.getAnnual()+bonus;
}
}
Worker类
java
package com.hspedu.poly_.polyparameter_;
public class Worker extends Employee{
public Worker(String name, double salary) {
super(name, salary);
}
public void work() {
System.out.println("员工 " + getName() + " is working");
}
//因为普通员工没有其它收入,则直接调用父类方法
@Override
public double getAnnual() {
return super.getAnnual();
}
}
Ployparameter类
java
package com.hspedu.poly_.polyparameter_;
import com.sun.corba.se.spi.orbutil.threadpool.Work;
public class Ployparameter {
public static void main(String[] args) {
Worker tom = new Worker("tom", 2500);
Manager milian = new Manager("milian", 5000, 20000);
Ployparameter ployparameter = new Ployparameter();
ployparameter.showEmpAnnual(tom);
ployparameter.showEmpAnnual(milian);
ployparameter.testWork(tom);
ployparameter.testWork(milian);
}
//实现获取任何员工对象的年工资,并在 main 方法中调用该方法 [e.getAnnual()]
public void showEmpAnnual(Employee e) {
System.out.println(e.getAnnual());//动态绑定机制
}
//添加一个方法,testWork,如果是普通员工,则调用 work 方法,如果是经理,则调用 manage 方法
public void testWork(Employee e) {
if(e instanceof Worker) {
((Worker) e).work(); //向下转型
} else if(e instanceof Manager) {
((Manager) e).manage(); //向下转型
} else {
System.out.println("你的类型不对,不做任何处理");
}
}
}