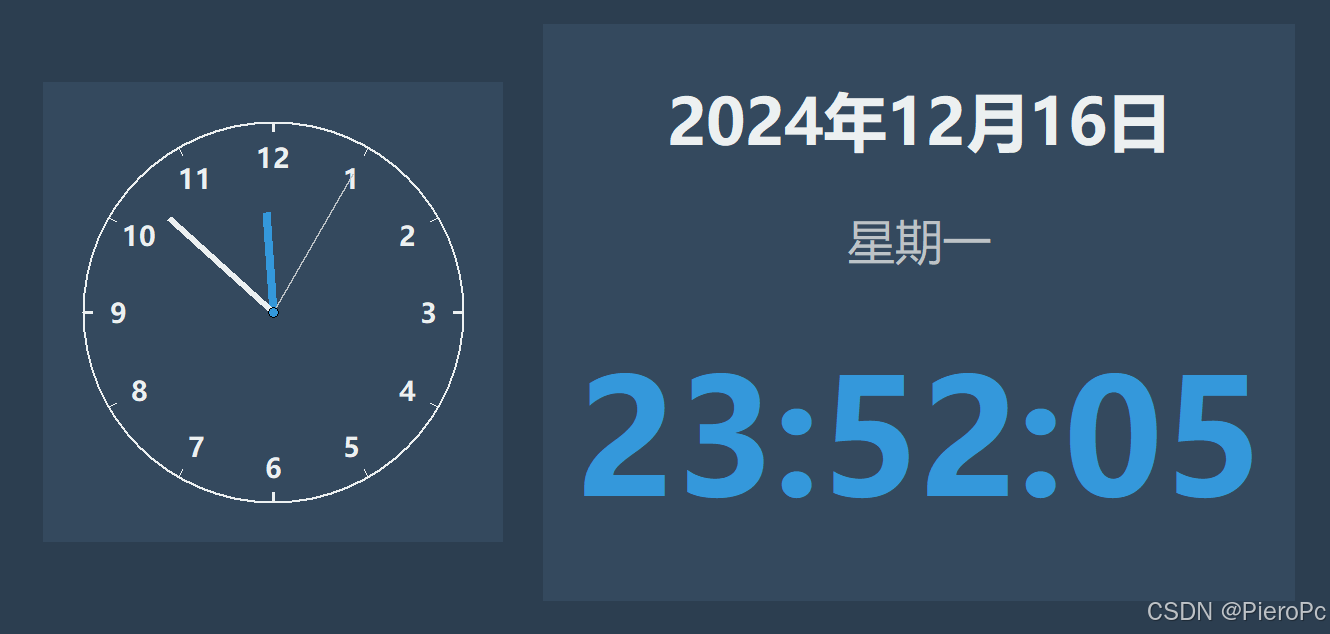
原代码:
python
# 日历式时钟
# 导入所需的库
# 作者:Hoye
# 日期:2024年12月16日
# 功能:显示当前日期、星期、时间,并显示模拟时钟
import tkinter as tk
from tkinter import ttk
import time
import math
import sys
def exit_screensaver(event=None):
root.quit()
def draw_clock_face():
# 清除画布
clock_canvas.delete("all")
# 获取当前时间
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
# 时钟外圈
clock_canvas.create_oval(10, 10, 390, 390, width=2, outline="#ECF0F1")
# 绘制刻度和数字
for i in range(12):
angle = i * math.pi/6 - math.pi/2
# 刻度线
start_x = 200 + 190 * math.cos(angle)
start_y = 200 + 190 * math.sin(angle)
end_x = 200 + 180 * math.cos(angle)
end_y = 200 + 180 * math.sin(angle)
width = 3 if i % 3 == 0 else 1
clock_canvas.create_line(start_x, start_y, end_x, end_y, fill="#ECF0F1", width=width)
# 添加数字
num = 12 if i == 0 else i
text_x = 200 + 155 * math.cos(angle)
text_y = 200 + 155 * math.sin(angle)
clock_canvas.create_text(text_x, text_y, text=str(num),
font=("Microsoft YaHei UI", 20, "bold"),
fill="#ECF0F1")
# 时针
hour_angle = (hours + minutes/60) * math.pi/6 - math.pi/2
hour_x = 200 + 100 * math.cos(hour_angle)
hour_y = 200 + 100 * math.sin(hour_angle)
clock_canvas.create_line(200, 200, hour_x, hour_y, fill="#3498DB", width=8)
# 分针
min_angle = minutes * math.pi/30 - math.pi/2
min_x = 200 + 140 * math.cos(min_angle)
min_y = 200 + 140 * math.sin(min_angle)
clock_canvas.create_line(200, 200, min_x, min_y, fill="#ECF0F1", width=6)
# 秒针
sec_angle = seconds * math.pi/30 - math.pi/2
sec_x = 200 + 160 * math.cos(sec_angle)
sec_y = 200 + 160 * math.sin(sec_angle)
clock_canvas.create_line(200, 200, sec_x, sec_y, fill="#BDC3C7", width=2)
# 中心点
clock_canvas.create_oval(195, 195, 205, 205, fill="#3498DB")
# 每秒更新
root.after(1000, draw_clock_face)
def update_clock():
current_time = time.localtime()
year = current_time.tm_year
month = current_time.tm_mon
day = current_time.tm_mday
weekday = current_time.tm_wday
hours = current_time.tm_hour
minutes = current_time.tm_min
seconds = current_time.tm_sec
date_str = f"{year}年{month:02d}月{day:02d}日"
weekday_str = ["星期一", "星期二", "星期三", "星期四", "星期五", "星期六", "星期日"][weekday]
time_str = f"{hours:02d}:{minutes:02d}:{seconds:02d}"
date_label.config(text=date_str)
weekday_label.config(text=weekday_str)
time_label.config(text=time_str)
root.after(1000, update_clock)
# 创建主窗口
root = tk.Tk()
root.title("蓝动力电脑-桌面时钟")
# 设置全屏
root.attributes('-fullscreen', True) # 全屏显示
root.attributes('-topmost', True) # 窗口置顶
root.config(cursor="none") # 隐藏鼠标光标
# 绑定退出事件
root.bind('<Key>', exit_screensaver) # 任意键退出
root.bind('<Motion>', exit_screensaver) # 鼠标移动退出
root.bind('<Button>', exit_screensaver) # 鼠标点击退出
root.bind('<Escape>', exit_screensaver) # ESC键退出
# 获取屏幕尺寸
screen_width = root.winfo_screenwidth()
screen_height = root.winfo_screenheight()
# 设置背景渐变色
main_frame = tk.Frame(root)
main_frame.pack(expand=True, fill='both')
main_frame.configure(bg='#2C3E50')
# 创建内容框架
content_frame = tk.Frame(main_frame, bg='#2C3E50', padx=20, pady=20)
content_frame.pack(expand=True)
# 创建左侧模拟时钟框架
analog_frame = tk.Frame(content_frame, bg='#34495E', padx=30, pady=30)
analog_frame.pack(side='left', padx=20)
# 创建模拟时钟画布
clock_canvas = tk.Canvas(
analog_frame,
width=400,
height=400,
bg='#34495E',
highlightthickness=0
)
clock_canvas.pack()
# 创建右侧数字时钟容器
clock_frame = tk.Frame(content_frame, bg='#34495E', padx=30, pady=30)
clock_frame.pack(side='right', padx=20)
# 日期标签
date_label = tk.Label(
clock_frame,
font=("Microsoft YaHei UI", 48, "bold"),
fg="#ECF0F1",
bg="#34495E"
)
date_label.pack(pady=20)
# 星期标签
weekday_label = tk.Label(
clock_frame,
font=("Microsoft YaHei UI", 36),
fg="#BDC3C7",
bg="#34495E"
)
weekday_label.pack(pady=20)
# 时间标签
time_label = tk.Label(
clock_frame,
font=("Microsoft YaHei UI", 120, "bold"),
fg="#3498DB",
bg="#34495E"
)
time_label.pack(pady=30)
# 添加版权信息
footer_label = tk.Label(
main_frame,
text="蓝动力电脑 © 2024",
font=("Microsoft YaHei UI", 14),
fg="#95A5A6",
bg="#2C3E50"
)
footer_label.pack(side='bottom', pady=15)
# 启动时钟更新
update_clock()
draw_clock_face()
# 启动主循环
root.mainloop()
代码简说:
添加了 exit_screensaver 函数处理退出事件
设置窗口属性:
• root.attributes('-fullscreen', True) 实现全屏显示
• root.attributes('-topmost', True) 使窗口始终置顶
• root.config(cursor="none") 隐藏鼠标光标
- 绑定各种退出事件:
• 键盘按键
• 鼠标移动
• 鼠标点击
打包成exe 再改 成 .scr
python
import PyInstaller.__main__
PyInstaller.__main__.run([
'9_日历式时钟.py',
'--name=蓝动力时钟屏保',
'--noconsole',
'--onefile',
# '--icon=clock.ico', # 如果您有图标文件的话
'--windowed',
])
py setup.py
打包完成后,在 dist 目录下找到生成的 exe 文件
将 exe 文件复制一份,改名为 .scr 后缀 • 例如:蓝动力时钟屏保.exe → 蓝动力时钟屏保.scr
将 .scr 文件复制到 Windows 系统目录:
• 通常是 C:\Windows\System32
• 或者 C:\Windows\SysWOW64(64位系统)
- 在 Windows 设置中设置屏保:
• 右键桌面 → 个性化
• 锁屏界面 → 屏幕保护程序设置
• 在屏幕保护程序下拉菜单中选择"蓝动力时钟屏保"
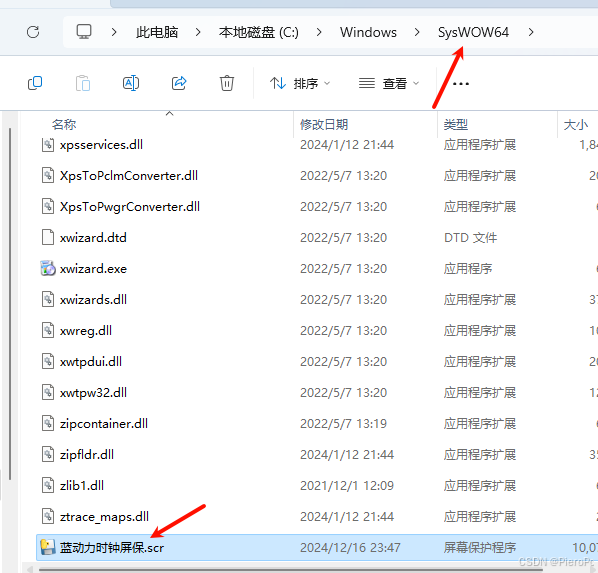
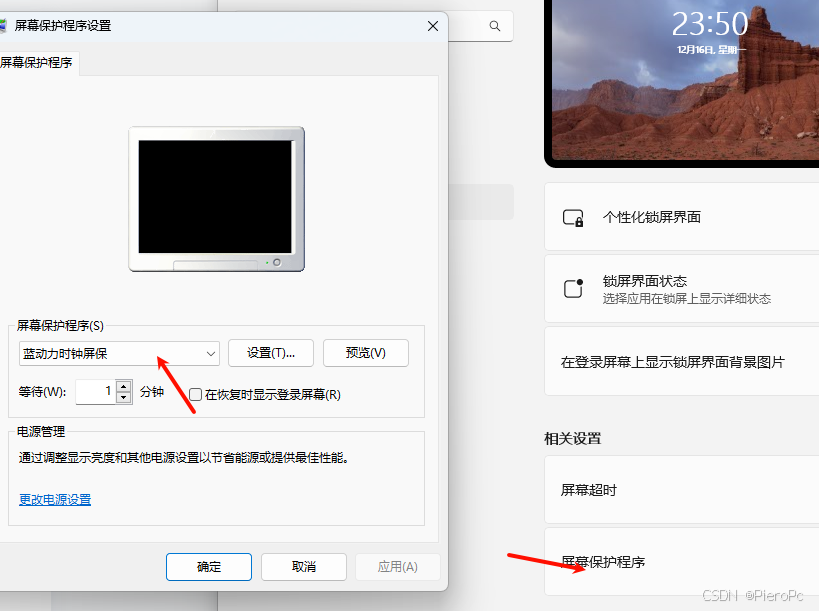