总目录
前言
详细介绍using的使用
一、using 是什么
- using 是C# 中的关键字。
- using 关键字表明程序使用的是给定命名空间中的名称。
二、using的使用
1、使用using引入命名空间
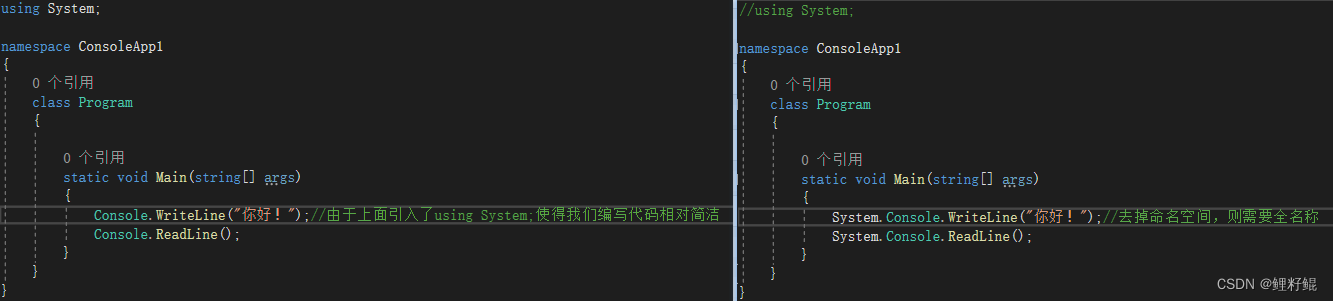
2、using指令
- using static
using static 指令命名了一种类型,无需指定类型名称即可访问其静态成员和嵌套类型
案例如下:
平常工作中我们可以将将一些路径比较长的类使用 using static 进行简化,但是代码过于简洁可能会影响可读性,这个具体情况具体分析。
csharp
using static Color;
enum Color
{
Red,
Green,
Blue
}
class Program
{
public static void Main()
{
Color color = Green;
}
}
3、using别名
using 别名需要注意
csharp
using s = System.Text;
using 指令的声明中不能使用 using 别名。
using s.RegularExpressions; // 这样使用会报错
如何为命名空间定义和使用 using 别名 案例如下:
csharp
namespace PC
{
// Define an alias for the nested namespace.
using Project = PC.MyCompany.Project;
class A
{
void M()
{
// Use the alias
var mc = new Project.MyClass();
}
}
namespace MyCompany
{
namespace Project
{
public class MyClass { }
}
}
}
4、using语句
using 语句提供可确保正确使用 IDisposable 对象的方便语法。
using语句具有即开即关的功能,当using中的代码运行完会自动释放资源
csharp
string manyLines = @"This is line one
This is line two
Here is line three
The penultimate line is line four
This is the final, fifth line.";
using (var reader = new StringReader(manyLines))
{
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
using 声明不需要大括号:
csharp
string manyLines = @"This is line one
This is line two
Here is line three
The penultimate line is line four
This is the final, fifth line.";
using var reader = new StringReader(manyLines);
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
使用try 和finally 可以实现using相同的结果,代码如下:
csharp
string manyLines = @"This is line one
This is line two
Here is line three
The penultimate line is line four
This is the final, fifth line.";
//请注意,使用额外的大括号为对象创建有限范围
{
var reader = new StringReader(manyLines);
try
{
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
finally
{
reader?.Dispose();
}
}
这里通过一个小案例补充说明一下 大括号的作用:
csharp
static void Main(string[] args)
{
{
var str = "abcdefg";
int length= str.Length ;
}
//在大括号{ }外我们是无法访问到 str 这个变量的
//这就是大括号的作用:创建有限范围
}
可在单个 using 语句中声明一个类型的多个实例,如下面的示例中所示。 注意,在单个语句中声明多个变量时,不能使用隐式类型的变量 (var):
csharp
string numbers = @"One
Two
Three
Four.";
string letters = @"A
B
C
D.";
using (StringReader left = new StringReader(numbers),
right = new StringReader(letters))
{
string? item;
do
{
item = left.ReadLine();
Console.Write(item);
Console.Write(" ");
item = right.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
/* 以上代码如果不要大括号则如下:
using StringReader left = new StringReader(numbers),
right = new StringReader(letters);
string? item;
do
{
item = left.ReadLine();
Console.Write(item);
Console.Write(" ");
item = right.ReadLine();
Console.WriteLine(item);
} while (item != null);
*/
三、全局引用
所有案例均用 控制台应用程序 创建
1、 global using
向 using 指令添加 global 修饰符意味着 using 将应用于编译中的所有文件(通常是一个项目)。 global using 指令被添加到 C# 10 中。 其语法为:
csharp
global using <fully-qualified-namespace>;
可以将指令添加到 global using 任何源文件。 通常,你想要将它们保存在单个位置。 global using 指令的顺序并不重要,无论是在单个文件中,还是在文件之间。
1. 使用案例1
当我们需要使用Debug.WriteLine
的时候,需要分别在项目的每个类中使用 using System.Diagnostics;
csharp
using System.Diagnostics;
namespace ConsoleApp2
{
internal class Program
{
static void Main(string[] args)
{
Debug.WriteLine("Hello World");
Console.WriteLine("Hello, World!");
}
}
}
csharp
using System.Diagnostics;
namespace ConsoleApp2
{
internal class Demo1
{
public void Show()
{
Debug.WriteLine("Show");
}
}
}
如果当前的项目中有多个地方都引用了 using System.Diagnostics;
我们可以考虑使用 global using
,如下所示:
csharp
global using System.Diagnostics;
namespace ConsoleApp2
{
internal class Program
{
static void Main(string[] args)
{
Debug.WriteLine("Hello World");
Console.WriteLine("Hello, World!");
}
}
}
csharp
namespace ConsoleApp2
{
internal class Demo1
{
public void Show()
{
Debug.WriteLine("Show");
}
}
}
在当前项目的任何文件中 使用了 global using
,就可以作用于当前项目的所有文件,global using
的作用和声明的位置无关 。
2. 使用案例2 - 最佳使用
通过将 global using
保存在项目中的一个文件中,可以更轻松地管理 global using
的使用。
- 在项目中建立一个类文件,然后命令为
GlobalUsings
- 将项目中所有需要使用全局引用的命名空间全部写在
GlobalUsings
中 - 这样将极大的方便我们对于全部引用的管理和使用
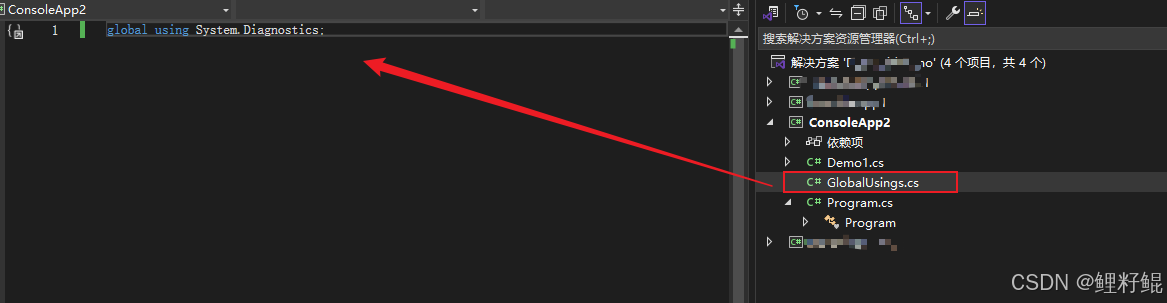
csharp
namespace ConsoleApp2
{
internal class Program
{
static void Main(string[] args)
{
Debug.WriteLine("Hello World");
Console.WriteLine("Hello, World!");
}
}
}
csharp
namespace ConsoleApp2
{
internal class Demo1
{
public void Show()
{
Debug.WriteLine("Show");
}
}
}
2、global using static
修饰 global 符可以与 static 修饰符组合。 global修饰符可以应用于 using alias 指令。 在这两种情况下,指令的作用域都是当前编译中的所有文件。 以下示例允许在项目中的所有文件中使用 System.Math 中声明的所有方法:
csharp
global using static System.Math;
1. 使用案例
接着上面的案例,
在 Main
方法中,加入一行
csharp
static void Main(string[] args)
{
Math.Min(1, 2);
Debug.WriteLine("Hello World");
Console.WriteLine("Hello, World!");
}
再在 GlobalUsings
文件中,加入一行 global using static System.Math;
GlobalUsings.cs
内容如下:
csharp
global using System.Diagnostics;
global using static System.Math;
此时Main 方法中对于 Math.Min 方法的调用 可以 省略 Math,这可以极大的简化我们的代码
csharp
static void Main(string[] args)
{
Min(1, 2);
Debug.WriteLine("Hello World");
Console.WriteLine("Hello, World!");
}
3、<Using> 项
可以通过将 项添加到项目文件(例如 <Using Include="My.Awesome.Namespace" />
)来全局包含命名空间。
继续接着上面的案例,在项目文件( .csproj
文件)中加入 以下几行标签
xml
<ItemGroup>
<Using Include="System.Diagnostics"/>
<Using Static="true" Include="System.Math"/>
</ItemGroup>
作用和GlobalUsings.cs
作用一样(GlobalUsings.cs
文件可以删除了):
csharp
global using System.Diagnostics;
global using static System.Math;
xml
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net8.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
<Nullable>enable</Nullable>
</PropertyGroup>
<ItemGroup>
<Using Include="System.Diagnostics"/>
<Using Static="true" Include="System.Math"/>
</ItemGroup>
</Project>
4、 扩展
上面在项目中使用GlobalUsings.cs
文件 或者 在 .csproj
文件中加入<using>
都是仅对当前的一个项目有效,如果一个解决方案中,多个项目都有相同的全局引用。可以操作如下:
- 在解决方案项的 解决方案文件夹中新建一个
GlobaUsings.props
文件 - 在该文件中,定义一些全局引用的项
xml
<Project>
<ItemGroup>
<Using Include="System.Diagnostics"/>
<Using Static="true" Include="System.Math"/>
</ItemGroup>
</Project>
- 在需要
GlobaUsings.props
文件的项目中,使用 Import 导入到指定.csproj
文件中
xml
<Project Sdk="Microsoft.NET.Sdk">
<!--导入项目配置-->
<Import Project="../GlobaUsings.props" />
<Import Project="../Package.props" />
<Import Project="../xxxx.props" />
<!--其他项目配置-->
</Project>
如果解决方案中的每个项目都会使用到 GlobaUsings.props
中的配置,可以考虑直接将 该配置导入到Directory.Build.props
配置文件中(或直接将配置写在Directory.Build.props
中)
结语
回到目录页: C# 知识汇总
希望以上内容可以帮助到大家,如文中有不对之处,还请批评指正。
参考资料:
C#文档 - 语言参考 - using
C#文档 - using 指令
C#命名空间
.NET SDK 项目的 MSBuild 引用
C# 控制台应用模板可生成顶级语句
.NET 项目 SDK