批量插入数据是指一次性插入多条记录到数据库中,这可以提高数据插入的效率。
前提条件
- 已经安装了MySQL数据库。
- 已经创建了数据库和表。
- 已经添加了MySQL JDBC驱动到项目的路径中。
如果没有添加jdbc可以参考:jdbc连接mysql(5种方式,循序渐进!!!)-CSDN博客
示例数据库和表
假设我们有一个名为t1的数据库,其中有一个名为users
的表,结构如下:
sql
CREATE TABLE t1 (
id INT ,
name VARCHAR(50)
);
Java代码示例
以下是一个Java程序,展示如何使用JDBC批量插入数据到t1表:
**注意:**一定要配置配置rewriteBatchedStatements参数
mysql.properties文件内容:
java
user=root
password=root
url=jdbc:mysql://localhost:3306/mydb?rewriteBatchedStatements=true
driver=com.mysql.cj.jdbc.Driver
优化点:
- 使用
addBatch()
缓存SQL,executeBatch()
批量提交。 - 分批次处理(如每500条提交一次)。
java
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Properties;
public class TestMysql {
public static void main(String[] args) {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
// 1. 加载配置文件
Properties properties = new Properties();
properties.load(new FileInputStream("src/mysql.properties"));
String url = properties.getProperty("url");
String user = properties.getProperty("user"); // 修正参数名更规范
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
// 2. 注册驱动并获取连接
Class.forName(driver);
connection = DriverManager.getConnection(url, user, password);
// 3. 关闭自动提交,开启事务
connection.setAutoCommit(false);
// 4. 准备SQL语句(建议常量用大写)
String sql = "INSERT INTO t1 (id, name) VALUES (?, ?)"; // 明确字段名
preparedStatement = connection.prepareStatement(sql);
// 5. 批量插入50万条数据
int batchSize = 500;
long start = System.currentTimeMillis();
for (int i = 0; i < 500000; i++) {
preparedStatement.setInt(1, i + 1); // 参数索引从1开始
preparedStatement.setString(2, "张三");
preparedStatement.addBatch();
// 每500条提交一次(注意i从0开始,需+1判断)
if ((i + 1) % batchSize == 0) { // 修正条件判断
preparedStatement.executeBatch();
preparedStatement.clearBatch();
}
}
// 6. 提交剩余未满batchSize的数据
preparedStatement.executeBatch();
// 7. 手动提交事务
connection.commit();
long end = System.currentTimeMillis();
System.out.println("插入50万数据花费时间:"+(end-start));
} catch (ClassNotFoundException | IOException e) {
e.printStackTrace();
} catch (SQLException e) {
// 8. 异常回滚事务
try {
if (connection != null) {
connection.rollback();
}
} catch (SQLException ex) {
ex.printStackTrace();
}
e.printStackTrace();
} finally {
try {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
结果如下:(用了4秒左右)
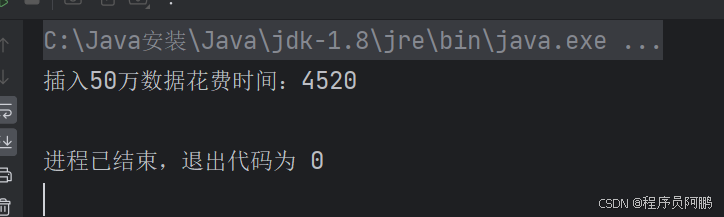
在navicat查询数量:
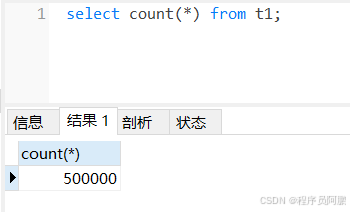