1、直接新建module进行导入,选择opencv的sdk
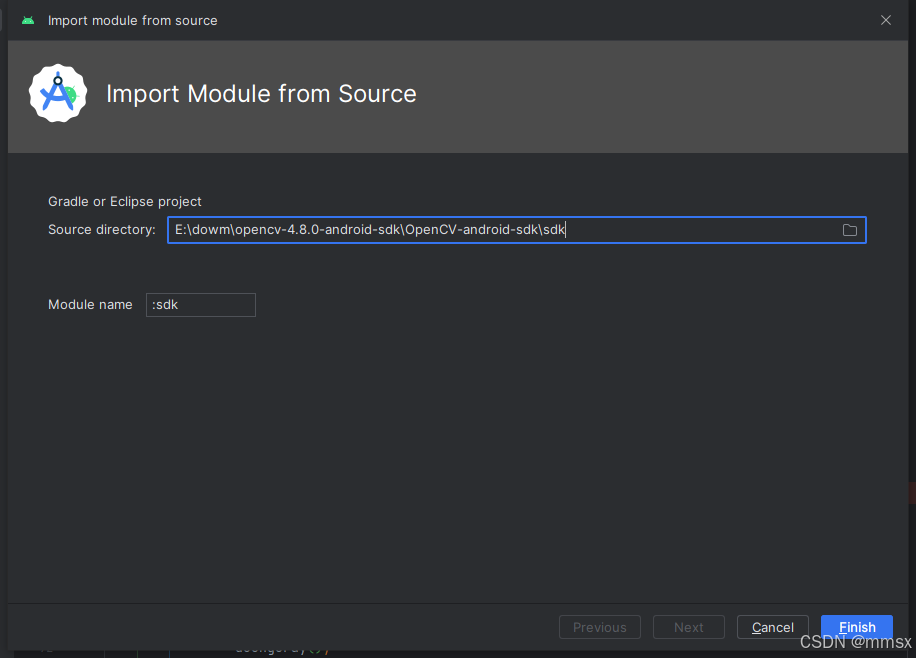
导入module模式,选择下载好的sdk,修改module name为OpenCV490。
有报错直接解决报错,没报错直接运行成功。
2、解决错误,同步成功
一般报错是gradle版本问题较多。我的报错如下:
Build file 'D:\work\OpenCVJni\OpenCV480\build.gradle' line: 92
A problem occurred evaluating project ':OpenCV480'.
> Plugin with id 'kotlin-android' not found.
注释掉
//apply plugin: 'kotlin-android'
重新同步成功。
那就直接build,运行没再报错,就可以直接引用项目进行测试。
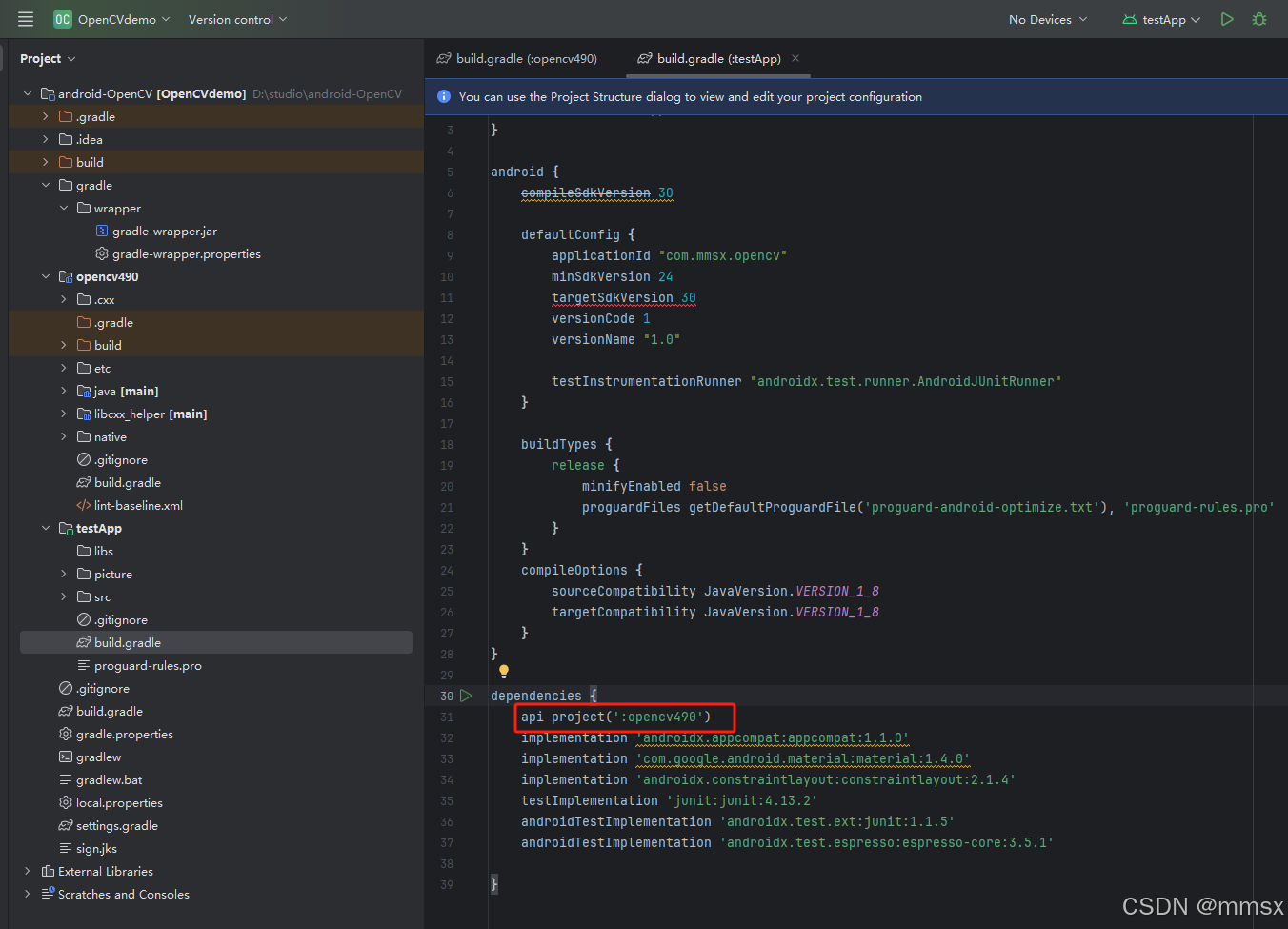
3、测试代码
public class MainActivity extends AppCompatActivity {
private Bitmap srcBitmap = null;
private Bitmap dstBitmap = null;
private ImageView imageView = null;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// opencv初始化
if (!OpenCVLoader.initDebug()) {
// Handle initialization error
return;
}
imageView = findViewById(R.id.imageView);
srcBitmap = BitmapFactory.decodeResource(getResources(), R.mipmap.image); // 替换为你的图片资源
findViewById(R.id.load).setOnClickListener(v -> {
imageView.setImageBitmap(srcBitmap);
});
findViewById(R.id.equalize).setOnClickListener(v -> {
// 加载成功后,进行直方图均衡化操作
dstBitmap = equalizeHistogram(srcBitmap);
imageView.setImageBitmap(dstBitmap);
});
}
private Bitmap equalizeHistogram(Bitmap srcBitmap) {
Mat srcMat = new Mat();
Mat dstMat = new Mat();
Utils.bitmapToMat(srcBitmap, srcMat);
// 将图片转换为灰度图
Imgproc.cvtColor(srcMat, srcMat, Imgproc.COLOR_BGR2GRAY);
// 将源图像的通道转换为单通道
List<Mat> channels = new ArrayList<>();
Core.split(srcMat, channels);
// 对每个通道进行直方图均衡化
Imgproc.equalizeHist(channels.get(0), channels.get(0));
// 合并通道
Core.merge(channels, dstMat);
// 将处理后的图像转换回RGB格式
Imgproc.cvtColor(dstMat, dstMat, Imgproc.COLOR_GRAY2BGR);
Bitmap equalizedBitmap = Bitmap.createBitmap(dstMat.cols(), dstMat.rows(), Bitmap.Config.ARGB_8888);
Utils.matToBitmap(dstMat, equalizedBitmap);
// 释放资源
srcMat.release();
dstMat.release();
return equalizedBitmap;
}
}
xml布局文件
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorWhite"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="80dp"
android:orientation="horizontal">
<Button
android:id="@+id/load"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="15dp"
android:background="@color/colorPrimaryDark"
android:text="加载"
android:textColor="@color/colorWhite" />
<Button
android:id="@+id/equalize"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="15dp"
android:background="@color/colorPrimaryDark"
android:text="直方图均衡"
android:textColor="@color/colorWhite" />
</LinearLayout>
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorWhite">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:src="@mipmap/image" />
</FrameLayout>
</androidx.appcompat.widget.LinearLayoutCompat>
代码截图
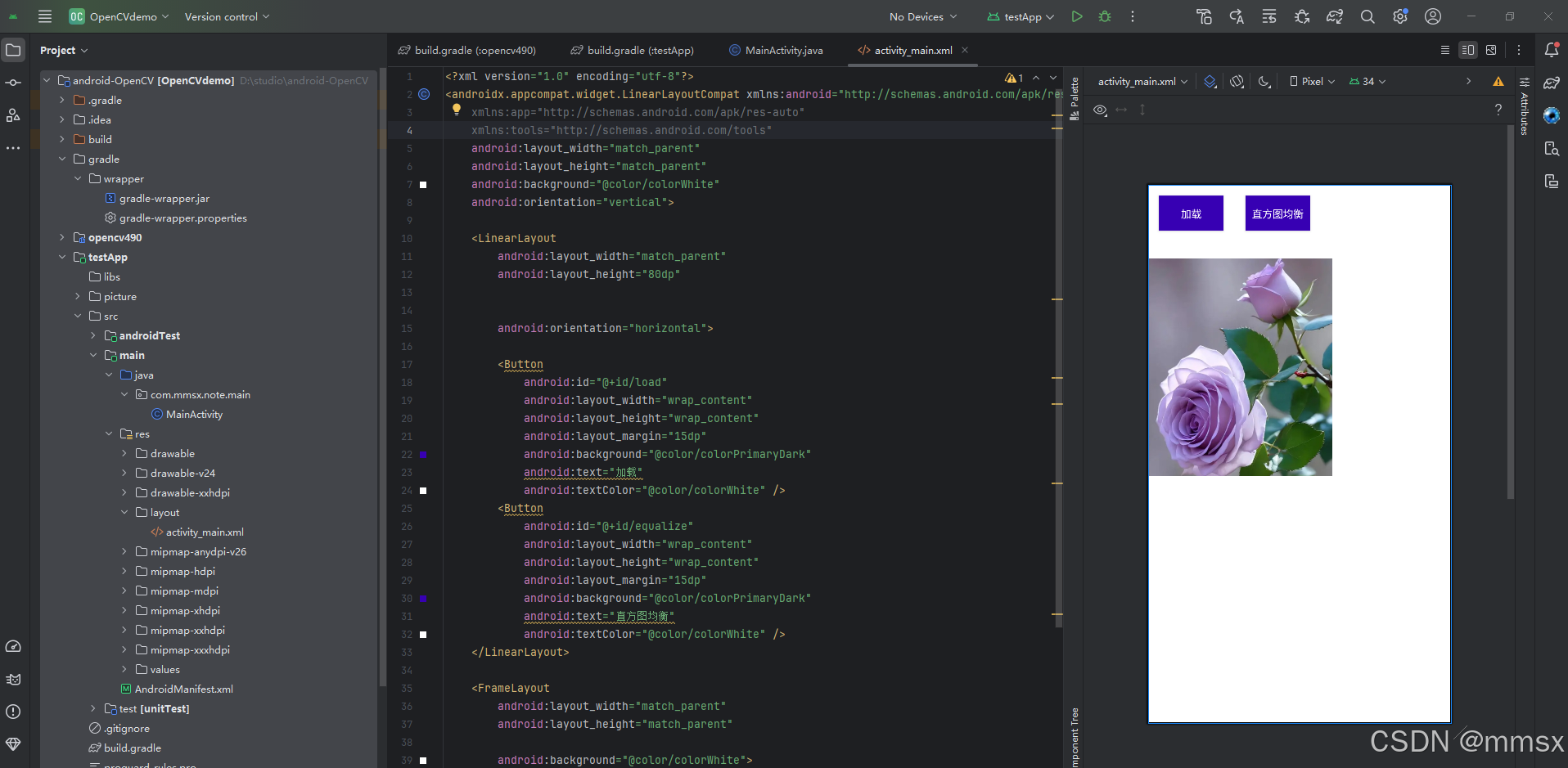
4、运行结果
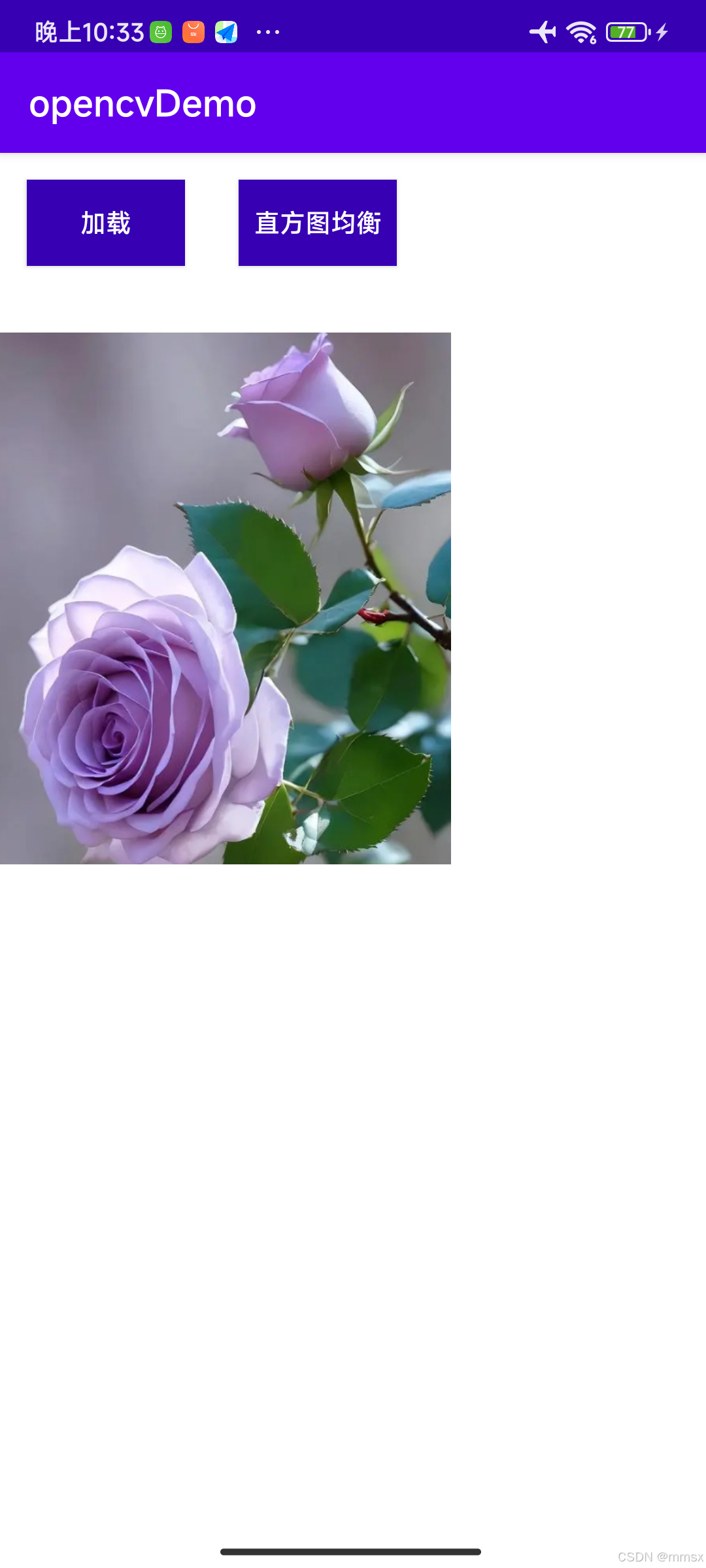
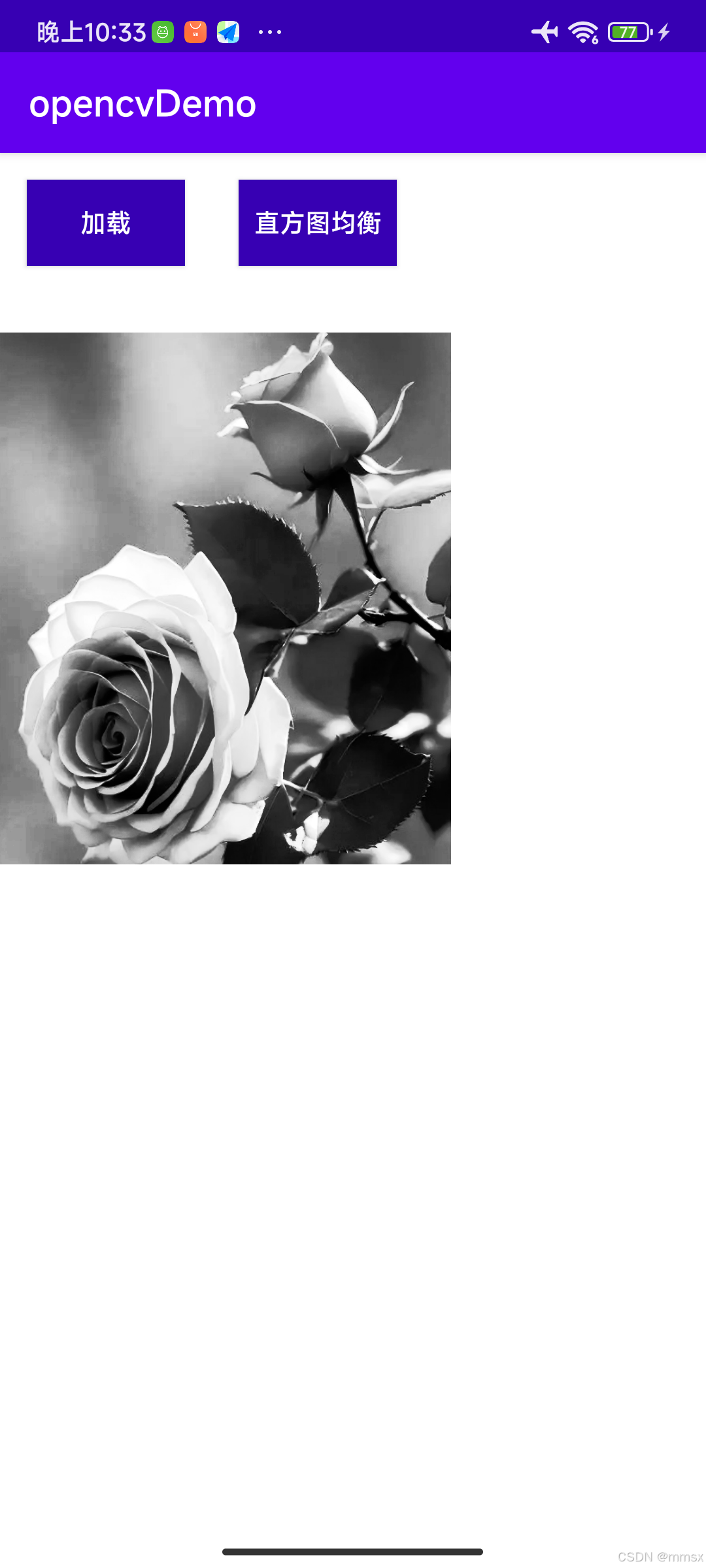
如果需要编译任意一个opencv版本搞不定的话,可以找我寻求帮助。