文章目录
一、引言
在现代应用程序开发中,缓存是提高性能和响应速度的关键技术之一。Java 提供了多种本地缓存解决方案,每种方案都有其特点和适用场景。本文将介绍四种常见的 Java 本地缓存实现:Guava Cache、Caffeine、Ehcache 和 Spring Cache。
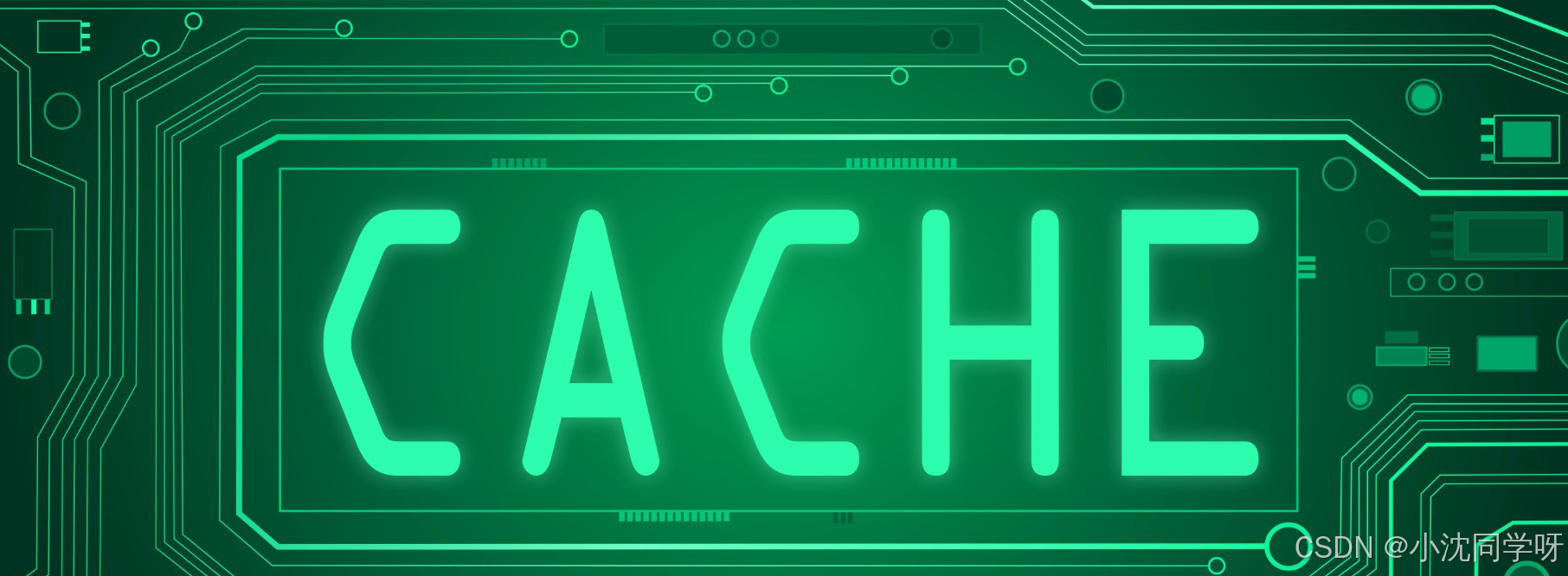
二、Guava Cache
理论介绍
Guava Cache 是 Google Guava 库的一部分,提供了轻量级的本地缓存功能。它具有以下特点:
简单易用:API 设计简洁,易于集成到项目中。
自动回收:支持基于时间或引用的自动回收机制。
并发支持:内置高效的并发控制,适合多线程环境。
实战演示
pom
bash
<dependencies>
<!-- Guava Cache -->
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
</dependencies>
示例代码
bash
/**
* LocalCacheTest
* @author senfel
* @version 1.0
* @date 2024/12/20 17:17
*/
@SpringBootTest
public class LocalCacheTest {
/**
* guavaCache
* @author senfel
* @date 2024/12/20 17:19
* @return void
*/
@Test
public void guavaCache() throws Exception{
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(100)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
return "Value for " + key;
}
});
System.out.println(cache.get("key1")); // 输出: Value for key1
}
三、Caffeine
理论介绍
Caffeine 是一个高性能的本地缓存库,继承了 Guava Cache 的优点并进行了优化。它的特点包括:
高性能:比 Guava Cache 更快,特别是在高并发环境下。
灵活配置:支持多种缓存策略,如 LRU(最近最少使用)、LFU(最不经常使用)等。
内存友好:通过弱引用和软引用来减少内存占用。
实战演示
pom
bash
<dependencies>
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.3</version>
</dependency>
</dependencies>
示例代码
bash
/**
* caffeineCache
* @author senfel
* @date 2024/12/20 17:25
* @return void
*/
@Test
public void caffeineCache() throws Exception{
Cache<String, String> cache = Caffeine.newBuilder()
.maximumSize(100)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
cache.put("key1", "value1");
System.out.println(cache.getIfPresent("key1")); // 输出: value1
}
四、Ehcache
理论介绍
Ehcache 是一个广泛使用的开源缓存框架,适用于分布式和非分布式环境。它的特点有:
丰富的特性:支持多种缓存策略、持久化、集群等功能。
配置灵活:可以通过 XML 或注解进行配置。
社区活跃:拥有庞大的用户群体和活跃的社区支持。
实战演示
pom
bash
<dependencies>
<!-- Ehcache 核心库 -->
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>2.10.6</version>
</dependency>
<!-- Ehcache 的 web 集群分布式缓存的支持 -->
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache-web</artifactId>
<version>2.0.4</version>
</dependency>
</dependencies>
ehcache.xml
bash
<!-- ehcache.xml -->
<ehcache>
<cache name="exampleCache"
maxEntriesLocalHeap="100"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"/>
</ehcache>
示例代码
bash
/**
* ehcacheCache
* @author senfel
* @date 2024/12/20 17:31
* @return void
*/
@Test
public void ehcacheCache() throws Exception{
CacheManager cacheManager = CacheManager.create("D:\\workspace\\cce-demo\\src\\main\\resources\\ehcache.xml");
Ehcache cache = cacheManager.getCache("exampleCache");
cache.put(new Element("key1", "value1"));
System.out.println(cache.get("key1").getObjectValue()); // 输出: value1
}
五、Spring Cache
理论介绍
Spring Cache 是 Spring 框架提供的缓存抽象层,可以与多种缓存实现无缝集成。它的特点包括:
声明式缓存:通过注解简化缓存逻辑的实现。
高度集成:与 Spring 生态系统紧密集成,方便与其他组件协同工作。
灵活选择:支持多种缓存提供者,如 ConcurrentMapCache、Ehcache、Caffeine 等。
实战演示
pom
bash
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.3</version>
</dependency>
yaml
bash
spring:
cache:
type: caffeine
caffeine:
spec: maximumSize=100,expireAfterWrite=10m
示例代码
bash
/**
* CacheService
* @author senfel
* @version 1.0
* @date 2024/12/20 17:45
*/
@Service
public class CacheService {
/**
* getData
* @param key
* @author senfel
* @date 2024/12/20 17:53
* @return java.lang.String
*/
@Cacheable(value = "myCache")
public String getData(String key) {
// 模拟耗时操作
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Value for " + key;
}
}
bash
@Resource
private CacheService cacheService;
/**
* testCache
* @param key
* @author senfel
* @date 2024/12/20 18:00
* @return java.lang.String
*/
@RequestMapping("/testCache")
public String testCache(String key) {
return cacheService.getData(key);
}
六、总结
综上所述,Guava Cache 简单易用,自动回收 ,适合小型应用,对性能要求不高;Caffeine高性能,灵活配置 高并发环境,适合对性能敏感的应用;Ehcache功能丰富,配置灵活,适合分布式系统,需要复杂缓存策略;Spring Cache 是声明式缓存,高度集成 ,适合Spring 应用,需要快速集成缓存。在实际的开放中,我们可以根据具体需求选择合适的缓存方案,可以显著提升应用程序的性能和用户体验。