- S pringboot开发 企业信息管理系统
- 引入资源
1.创建 项目引入页面原型
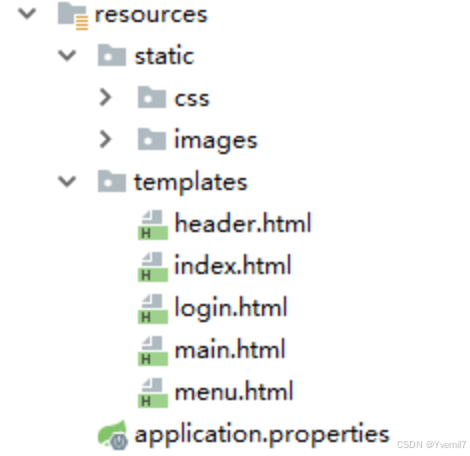
2.引入 maven 的 依赖和starters
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <dependency > <groupId >org.springframework.boot</groupId > <artifactId >spring-boot-starter-web</artifactId > </dependency > <dependency > <groupId >org.springframework.boot</groupId > <artifactId >spring-boot-starter-test</artifactId > <scope >test</scope > </dependency > <dependency > <groupId >org.springframework.boot</groupId > <artifactId >spring-boot-devtools</artifactId > <optional >true</optional > </dependency > <dependency > <groupId >org.springframework.boot</groupId > <artifactId >spring-boot-starter-thymeleaf</artifactId > </dependency > <dependency > <groupId >org.webjars</groupId > <artifactId >jquery</artifactId > <version >3.3.1</version > </dependency > <dependency > <groupId >org.mybatis.spring.boot</groupId > <artifactId >mybatis-spring-boot-starter</artifactId > <version >1.3.1</version > </dependency > <dependency > <groupId >com.alibaba</groupId > <artifactId >druid</artifactId > <version >1.0.9</version > </dependency > <dependency > <groupId >log4j</groupId > <artifactId >log4j</artifactId > <version >1.2.15</version > </dependency > <dependency > <groupId >mysql</groupId > <artifactId >mysql-connector-java</artifactId > <scope >runtime</scope > </dependency > |
-
- thymeleaf模板 处理
创建mvc的配置类TxMvcConfig
|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Configuration @MapperScan("cn.tx.springboot.mapper" ) public class TxMvcConfig implements WebMvcConfigurer{ @Override public void addViewControllers(ViewControllerRegistry registry) { registry.addViewController("/toLogin" ).setViewName("login" ); registry.addViewController("/header" ).setViewName("header" ); registry.addViewController("/index" ).setViewName("index" ); registry.addViewController("/menu" ).setViewName("menu" ); registry.addViewController("/add" ).setViewName("add" ); } @Override public void addInterceptors(InterceptorRegistry registry) { List<String> excludePatterns = new ArrayList<String>(); excludePatterns.add("/css/**" ); excludePatterns.add("/images/**" ); excludePatterns.add("/toLogin" ); excludePatterns.add("/login" ); registry.addInterceptor(new LoginInterceptor()) .addPathPatterns("/**" ) .excludePathPatterns(excludePatterns); } } |
-
- 创建数据库 环境
1.创建 数据库执行脚本
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| SET FOREIGN_KEY_CHECKS=0; -- ---------------------------- -- Table structure for my_user -- ---------------------------- DROP TABLE IF EXISTS `my_user`; CREATE TABLE `my_user` ( `pid` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(255) DEFAULT NULL, `password` varchar(255) DEFAULT NULL, `p_addr` varchar(255) DEFAULT NULL, `gender` int(11) DEFAULT NULL, `birth` date DEFAULT NULL, PRIMARY KEY (`pid`) ) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8; -- ---------------------------- -- Records of my_user -- ---------------------------- INSERT INTO `my_user` VALUES ('1', 'zhangsan', '123', '北京', '1', '2020-06-14'); |
- 配置druid 数据源
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| spring: datasource: username: root password: root url: jdbc:mysql://localhost:3306/boot_demo driver-class-name: com.mysql.jdbc.Driver type: com.alibaba.druid.pool.DruidDataSource initialSize: 5 minIdle: 5 maxActive: 20 maxWait: 60000 timeBetweenEvictionRunsMillis: 60000 minEvictableIdleTimeMillis: 300000 validationQuery: SELECT 1 FROM DUAL testWhileIdle: true testOnBorrow: false testOnReturn: false poolPreparedStatements: true filters: stat,wall,log4j maxPoolPreparedStatementPerConnectionSize: 20 useGlobalDataSourceStat: true connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500 |
- 创建 数据源的配置类
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Configuration public class DruidConfig { @ConfigurationProperties(prefix = "spring.datasource" ) @Bean public DataSource dataSource(){ return new DruidDataSource(); } } |
-
- 编写 数据层
1.创建 mybatis的配置类 Mybatis Config
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Configuration public class MybatisConfig { @Bean public ConfigurationCustomizer getCustomizer(){ return new ConfigurationCustomizer() { @Override public void customize(org.apache.ibatis.session.Configuration configuration) { configuration.setMapUnderscoreToCamelCase(true ); } }; } } |
2.创建 数据层UserMapper
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public interface MyUserMapper { @Options(useGeneratedKeys = true , keyProperty = "pid" ) @Insert("insert into my_user(pid, username, password, p_addr, gender, birth)values(" + "#{pid}, #{username}, #{password}, #{pAddr}, #{gender}, #{birth})" ) public void insert(MyUser user) ; @Select("select * from my_user" ) public List<MyUser> selectUsers(); @Select("select * from my_user t where t.username = #{username} and t.password = #{password}" ) public MyUser selectUsersById(Map<String, String> map); @Select("select * from my_user t where t.pid = #{pid}" ) public MyUser selectUsersById1(int userId); @Update("update my_user set " + "username = #{username}," + "password=#{password}, " + "p_addr= #{pAddr}, " + "gender=#{gender}, " + "birth=#{birth} " + "where pid = #{pid}" ) public void update(MyUser user) ; @Delete("delete from my_user where pid = #{pid}" ) public void delete(int pid) ; } |
-
- 编写 Service
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public interface MyUserService { public void insert(MyUser user) ; public void update(MyUser user) ; public List<MyUser> selectUsers(); public MyUser selectUsersById(Map<String, String> map); public MyUser selectUsersById1(int userId); public void delete(int pid) ; } |
实现类略(参见视频)
-
- 页面 处理
-
main页面处理
|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <html lang= "en" xmlns: th = "http://www.thymeleaf.org" > <head > <meta http-equiv= "Content-Type" content= "text/html; charset=utf-8" /> <title >首页</title > </head > <frameset cols= "*" rows= "136, *" id= "frame_main" border= "0" > <frame src= "header" noresize= "noresize" name= "header" > <frameset cols= "240, *" > <frame th :src= "@{/menu}" name= "menu" /> <frame th :src= "@{/main}" name= "main" > </frameset > </frameset > |
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <a th :href= "@{/add}" >添加</a > <table class= "mt" align= "center" > <tr > <td >用户名</td > <td >密码</td > <td >地址</td > <td >生日</td > <td >操作</td > </tr > <tr th :each= "user:{users}"**** \> \<****td**** ****th**** ****:text=**** ****"{user.username}" ></td > <td th :text= "{user.password}"**** \>\****td**** \> \<****td**** ****th**** ****:text=**** ****"{user.pAddr}" ></td > <td th :text= "{#calendars.format(user.birth,'yyyy-MM-dd')}"**** \>\****td**** \> \<****td**** \> \<****a**** ****th**** ****:href=**** ****"@{/getUser(pid={user.pid})}" >修改</a > <a th :href= "@{/delete(pid=${user.pid})}" >删除</a > </td > </tr > </table > |
2.添加页面处理
引入thymeleaf命名空间后
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <form th :action= "@{/save}" method= "post" > <table class= "mt" align= "center" > <tr > <td >用户名</td > <td ><input name= "username" type= "text" /></td > </tr > <tr > <td >密码</td > <td ><input name= "password" type= "password" /></td > </tr > <tr > <td >地址</td > <td ><input name= "pAddr" type= "text" /></td > </tr > <tr > <td >生日</td > <td ><input name= "birth" type= "text" /></td > </tr > <tr > <td >性别</td > <td ><input name= "gender" type= "text" /></td > </tr > <tr > <td ></td > <td ><input type= "submit" /></td > </tr > </table > </form > |
2.修改页面处理
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <form th :action= "@{/update}" method= "post" > <input type= "hidden" name= "pid" th :value= "{user.pid}"**** /\> \<****table**** ****class=**** ****"mt"**** ****align=**** ****"center"**** \> \<****tr**** \> \<****td**** \>用户名\****td**** \> \<****td**** \>\<****input**** ****name=**** ****"username"**** ****type=**** ****"text"**** ****th**** ****:value=**** ****"{user.username}" /></td > </tr ><tr > <td >密码</td > <td ><input name= "password" type= "password" th :value= "{user.password}"**** /\>\****td**** \> \****tr**** \>\<****tr**** \> \<****td**** \>地址\****td**** \> \<****td**** \>\<****input**** ****name=**** ****"pAddr"**** ****type=**** ****"text"**** ****th**** ****:value=**** ****"{user.pAddr}" /></td > </tr > <tr > <td >地址</td > <td ><input name= "gender" type= "text" th :value= "{user.gender}"**** /\>\****td**** \> \****tr**** \> \<****tr**** \> \<****td**** \>生日\****td**** \> \<****td**** \>\<****input**** ****name=**** ****"birth"**** ****type=**** ****"text"**** ****th**** ****:value=**** ****"{#calendars.format(user.birth, 'yyyy-MM-dd')}" /></td > </tr ><tr > <td ></td > <td ><input type= "submit" /></td > </tr > </table > </form > |
-
- 用户 登录
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd" > <html lang= "en" xmlns: th = "http://www.thymeleaf.org" > <head > <meta http-equiv= "Content-Type" content= "text/html; charset=utf-8" /> <title >用户登录</title > <!--<link href="css/login.css" rel="stylesheet" type="text/css" />--> <link th :href= "@{/css/login.css}" rel= "stylesheet" type= "text/css" /> <script th :src= "@{/webjars/jquery/3.3.1/jquery.js}" ></script > <script > function login1 () { **** (****"form"**** ).submit(); } \****script**** \> \****head**** \> \<****body**** \> \<****div**** ****id=**** ****"login"**** \> \<****div**** ****id=**** ****"top"**** \> \<****div**** ****id=**** ****"top_left"**** \>\<****img**** ****src=**** ****"images/login_03.gif"**** /\>\****div**** \> \<****div**** ****id=**** ****"top_center"**** \>\****div**** \> \****div**** \> \<****form**** ****th**** ****:action=**** ****"@{/login}"**** ****method=**** ****"post"**** \> \<****div**** ****id=**** ****"center"**** \> \<****div**** ****id=**** ****"center_left"**** \>\****div**** \> \<****div**** ****id=**** ****"center_middle"**** \> \<****div**** ****style=**** ****"**** ****text-align**** : ****center**** ; ****color**** : ****red**** ****"**** ****th**** ****:text=**** ****"{tip}" ></div > <div id= "user" >用 户 <input type= "text" name= "username" /> </div > <div id= "password" >密 码 <input type= "password" name= "password" /> </div > <div id= "btn" > <a href= "#" οnclick= " login1 ()" >登录</a > <a href= "#" >清空</a > </div > </div > <div id= "center_right" ></div > </div > </form > <div id= "down" > <div id= "down_left" > <div id= "inf" > <span class= "inf_text" >版本信息</span > <span class= "copyright" >信息管理系统 v2.0</span > </div > </div > <div id= "down_center" ></div > </div > </div > </body > </html > |
登录的Controller逻辑
|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @PostMapping("/login" ) public String login(HttpSession session, String username, String password, Model model) { Map<String, String> map = new HashMap<String, String>(); map.put("username" , username); map.put("password" , password); MyUser user = userService .selectUsersById(map); if (user != null ){ session.setAttribute("user" , user); return "redirect:index" ; }else { model.addAttribute("tip" ," 用户名或者密码错误 " ); return "login" ; } } |
Header模板页面
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <div id= "main" > <div id= "welcome" >欢迎你回来 <span th :text= "{session.user.username}"**** ****th**** ****:if=**** ****"{not #strings.isEmpty(session.user)}" ></span > <a target= "_top" th :text= " 请登录 " th :href= "@{/toLogin}" th :if= "${#strings.isEmpty(session.user)}" ></a > <img src= "images/clock.gif" /> 学习是最好的投资</div > <div id= "adminop" > <ul > <li ><a href= "#" >站点首页</a ></li > <li ><a href= "javascript: parent .location. reload ();" >管理首页</a ></li > <li ><a href= "javascript: parent .location. reload ();" >退出管理</a ></li > <li ><a href= "#" >站点首页</a ></li > </ul > </div > </div > |