一、uv坐标
相当于x、y轴,通过自定义uv坐标可以截取所需的纹理范围
javascript
<template>
<div id="container"></div>
</template>
<script setup>
import * as THREE from "three";
import { onMounted } from "vue";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
// 场景
const scene = new THREE.Scene();
scene.background = new THREE.Color(0x666666);
// 相机
const camera = new THREE.PerspectiveCamera();
// camera.position.x = 10;
camera.position.y = 0;
camera.position.z = 2;
// 创建矩形
const geometry = new THREE.PlaneGeometry(1, 1)
const texture = new THREE.TextureLoader().load('/textureMsg/cloud.jpeg') // 纹理贴图
// UV像素的取值范围 分别为四个顶点的坐标 左上 右上 左下 右下
const uv = new Float32Array([
0, 1,
1, 1,
0, 0,
1, 0
])
// UV像素的颜色值
// const colors = new Float32Array([
// 1.0, 0.0, 0.0, // 红色
// 0.0, 1.0, 0.0, // 绿色
// 0.0, 0.0, 1.0 // 蓝色
// ]);
// const colorAttribute = new THREE.BufferAttribute(colors, 3); // 每个颜色有3个分量 (r, g, b)
// geometry.setAttribute('color', colorAttribute);
// // 设置矩形的uv
geometry.attributes.uv = new THREE.BufferAttribute(uv, 2) // uv坐标 2表示只有x,y 3是x,y,z
// 材质
const material = new THREE.MeshBasicMaterial({
side: THREE.DoubleSide, // 双面可见
map: texture, // 纹理贴图
// vertexColors: true // 顶点颜色
})
console.log(geometry, 'geometry')
// 创建网格
const cube = new THREE.Mesh(geometry, material)
scene.add(cube)
// 添加坐标辅助线
const axesHelper = new THREE.AxesHelper( 5 );
scene.add( axesHelper );
onMounted(() => {
// 渲染器
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
// 将渲染器添加到页面
document.getElementById("container").appendChild(renderer.domElement);
// 轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
// 动画
function animate() {
// 更新动画
requestAnimationFrame(animate);
// 轨道控制器更新
controls.update();
renderer.render(scene, camera);
}
animate();
});
</script>
二、效果
1、原图
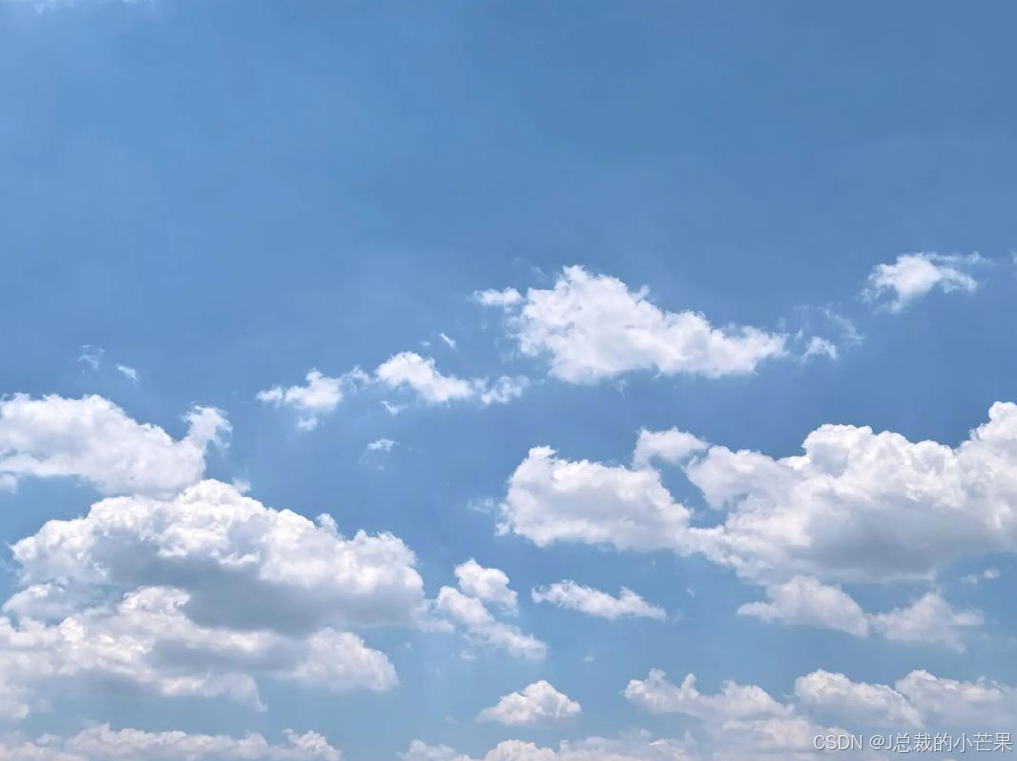
2、截取后
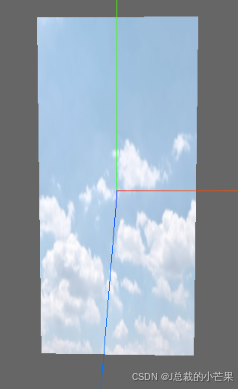