引言
在 Python 中,序列 是一种有序的数据结构,广泛应用于数据存储、操作和处理。序列的一个显著特点是支持通过索引 访问数据。常见的序列类型包括字符串(str
)、列表(list
)和元组(tuple
)。这些序列各有特点,既可以存储简单的字符,也可以存储复杂的对象。
为了帮助初学者掌握 Python 中的序列操作,本文将围绕字符串 、列表 和元组这三种序列类型,详细介绍其定义、常用方法和具体示例。
一、字符串
1. 定义
字符串(str
)是 Python 中最常见的不可变序列,用于存储字符数据。它由一系列字符组成,支持索引 和切片操作,常用于文本处理、数据存储和交互。
示例
python
s = "Hello, Python!"
print(type(s)) # 输出 <class 'str'>
2. 字符串的索引与切片
(1) 索引
字符串中的每个字符都可以通过索引访问,索引从 0
开始,负索引表示从右向左计数。
python
s = "Hello, Python!"
print(s[0]) # 输出 'H'
print(s[-1]) # 输出 '!'
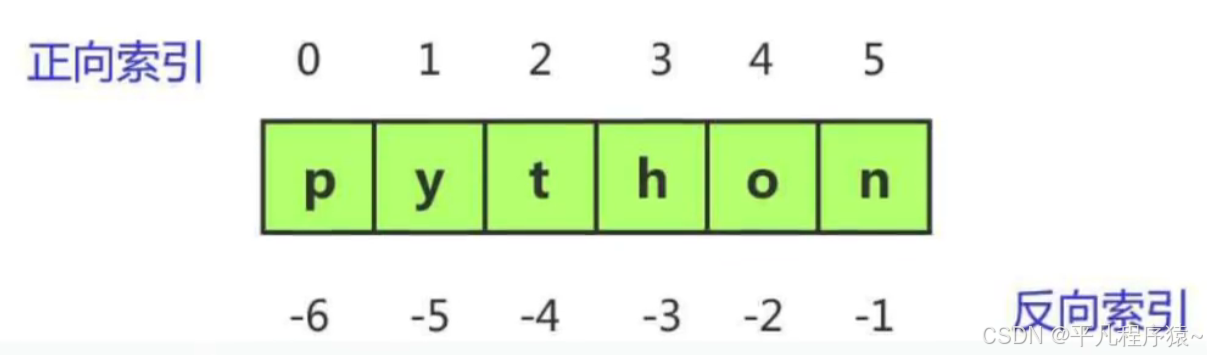
(2) 切片
切片用于提取字符串的一部分,格式为 [start:stop:step]
:
- start:切片的起始索引(包括)。
- stop:切片的结束索引(不包括)。
- step:切片步长,默认为 1。
python
s = "Hello, Python!"
print(s[0:5]) # 输出 'Hello'(索引 0 到 4)
print(s[7:]) # 输出 'Python!'(从索引 7 到末尾)
print(s[::-1]) # 输出 '!nohtyP ,olleH'(字符串反转)
3. 常用字符串方法
(1) 查询与定位
方法 | 作用 | 示例 |
---|---|---|
find |
返回子串首次出现的位置,找不到返回 -1 | "hello".find("l") → 2 |
index |
返回子串首次出现的位置,找不到抛出异常 | "hello".index("l") → 2 |
count |
返回子串出现的次数 | "hello".count("l") → 2 |
python
s = "hello world"
print(s.find("world")) # 输出 6
print(s.count("o")) # 输出 2
(2) 大小写转换
方法 | 作用 | 示例 |
---|---|---|
upper |
转换为全大写 | "hello".upper() → "HELLO" |
lower |
转换为全小写 | "HELLO".lower() → "hello" |
swapcase |
大小写互换 | "Hello".swapcase() → "hELLO" |
capitalize |
首字母大写,其余小写 | "hello world".capitalize() → "Hello world" |
title |
每个单词首字母大写 | "hello world".title() → "Hello World" |
python
s = "hello python"
print(s.upper()) # 输出 'HELLO PYTHON'
print(s.title()) # 输出 'Hello Python'
(3) 分割与连接
方法 | 作用 | 示例 |
---|---|---|
split |
按指定分隔符分割字符串,返回列表 | "a,b,c".split(",") → ['a', 'b', 'c'] |
join |
用指定字符连接列表中的元素,返回字符串 | " ".join(["a", "b", "c"]) → "a b c" |
strip |
去除首尾指定字符(默认空格) | " hello ".strip() → "hello" |
python
s = "apple,banana,cherry"
fruits = s.split(",")
print(fruits) # 输出 ['apple', 'banana', 'cherry']
print(" | ".join(fruits)) # 输出 'apple | banana | cherry'
(4) 判断类方法
方法 | 作用 | 示例 |
---|---|---|
startswith |
判断是否以指定字符串开头 | "hello".startswith("he") → True |
endswith |
判断是否以指定字符串结尾 | "hello".endswith("lo") → True |
isalpha |
检查是否只包含字母 | "abc".isalpha() → True |
isdigit |
检查是否只包含数字 | "123".isdigit() → True |
isalnum |
检查是否只包含字母和数字 | "abc123".isalnum() → True |
python
s = "Python3"
print(s.isalnum()) # 输出 True
print(s.isdigit()) # 输出 False
二、列表
1. 定义
列表(list
)是 Python 中最常用的可变序列类型。它可以存储任意类型的对象,并支持动态地添加、删除或修改元素。列表适用于需要频繁更改元素的场景。
示例
python
lst = [1, "hello", 3.14]
print(type(lst)) # 输出 <class 'list'>
2. 列表的操作
(1) 索引与切片
列表支持通过索引访问单个元素以及切片操作访问子列表。
python
lst = [10, 20, 30, 40, 50]
print(lst[0]) # 输出 10
print(lst[-1]) # 输出 50
print(lst[1:4]) # 输出 [20, 30, 40]
(2) 增删元素
方法 | 作用 | 示例 |
---|---|---|
append |
在列表末尾添加元素 | [1].append(2) → [1, 2] |
insert |
在指定位置插入元素 | [1, 3].insert(1, 2) → [1, 2, 3] |
remove |
移除第一个匹配的元素 | [1, 2, 3].remove(2) → [1, 3] |
pop |
移除并返回指定索引的元素 | [1, 2, 3].pop(1) → 返回 2,列表变 [1, 3] |
clear |
清空列表 | [1, 2, 3].clear() → [] |
python
lst = [1, 2, 3]
lst.append(4)
print(lst) # 输出 [1, 2, 3, 4]
lst.pop(1)
print(lst) # 输出 [1, 3, 4]
lst.clear()
print(lst) # 输出 []
(3) 排序与反转
方法 | 作用 | 示例 |
---|---|---|
sort |
对列表原地排序 | [3, 1, 2].sort() → [1, 2, 3] |
reverse |
原地反转列表 | [1, 2, 3].reverse() → [3, 2, 1] |
sorted |
返回排序后的新列表 | sorted([3, 1, 2]) → [1, 2, 3] |
python
lst = [3, 1, 4, 2]
lst.sort()
print(lst) # 输出 [1, 2, 3, 4]
lst.reverse()
print(lst) # 输出 [4, 3, 2, 1]
(4) 遍历列表
python
lst = ["apple", "banana", "cherry"]
for fruit in lst:
print(fruit)
(5) 嵌套与列表解析
列表支持嵌套,即列表中的元素也可以是列表,同时可以使用列表解析快速生成列表。
python
nested_list = [[1, 2], [3, 4], [5, 6]]
print(nested_list[1][1]) # 输出 4
squares = [x**2 for x in range(5)]
print(squares) # 输出 [0, 1, 4, 9, 16]
三、元组
1. 定义
元组(tuple
)是不可变的序列类型,用于存储多个值。元组在需要保护数据不被修改时非常有用,例如作为函数的返回值或字典的键。
示例
python
tpl = (10, 20, 30)
print(type(tpl)) # 输出 <class 'tuple'>
2. 元组的操作
(1) 索引与切片
元组支持通过索引访问单个元素以及切片操作。
python
tpl = (10, 20, 30, 40, 50)
print(tpl[0]) # 输出 10
print(tpl[1:4]) # 输出 (20, 30, 40)
(2) 元组方法
方法 | 作用 | 示例 |
---|---|---|
count |
返回指定值的出现次数 | (1, 2, 3, 1).count(1) → 2 |
index |
返回指定值的索引位置 | (1, 2, 3).index(2) → 1 |
python
tpl = (1, 2, 3, 1)
print(tpl.count(1)) # 输出 2
print(tpl.index(3)) # 输出 2
(3) 遍历元组
python
tpl = ("apple", "banana", "cherry")
for fruit in tpl:
print(fruit)
(4) 元组解包
元组可以快速解包,用于将元组的值赋给多个变量。
python
tpl = (1, 2, 3)
a, b, c = tpl
print(a, b, c) # 输出 1 2 3
四、序列的通用操作
1. 数学运算符
序列支持 +
和 *
操作。
python
print([1, 2] + [3, 4]) # 输出 [1, 2, 3, 4]
print([1, 2] * 2) # 输出 [1, 2, 1, 2]
2. 成员判断
使用 in
和 not in
检查元素是否在序列中。
python
print(3 in [1, 2, 3]) # 输出 True
3. Python 内置函数
以下内置函数常用于操作序列:
函数 | 功能 |
---|---|
len() |
返回序列的长度 |
max() |
返回序列中的最大值 |
min() |
返回序列中的最小值 |
sum() |
返回序列中所有元素的总和 |
sorted() |
返回排序后的新序列(不会修改原序列) |
enumerate() |
返回索引和值组成的可迭代对象 |
reversed() |
返回序列的反转迭代器 |
all() |
检查序列中的所有元素是否为真 |
any() |
检查序列中是否至少有一个元素为真 |
python
lst = [1, 2, 3, 4]
print(len(lst)) # 输出 4
print(max(lst)) # 输出 4
print(sum(lst)) # 输出 10
print(sorted(lst, reverse=True)) # 输出 [4, 3, 2, 1]
for index, value in enumerate(lst):
print(index, value) # 输出索引和值
print(all([True, 1, "hello"])) # 输出 True
print(any([False, 0, ""])) # 输出 False
总结
通过以上内容,您已经全面掌握了 Python 中字符串、列表和元组的定义、常用操作方法和技巧。Python 的序列类型提供了灵活且强大的功能,可以轻松应对数据操作的需求。无论是在开发中处理字符串、操作列表,还是使用元组保持数据的不可变性,这些知识都可以帮助您写出更高效的代码。希望本文内容能够为您的 Python 编程之旅增添助力!