国际化实现步骤
Spring Boot 3 提供了强大的国际化支持,使得应用程序可以根据用户的语言和区域偏好适配不同的语言和地区需求。
-
添加国际化资源文件: 国际化资源文件通常放在
src/main/resources
目录下,并按照不同的语言和地区命名,例如:messages.properties
:默认语言(如英文)messages_zh_CN.properties
:中文简体messages_fr.properties
:法语
-
配置 MessageSource Bean: 可以通过在
application.properties
或application.yml
中进行简单配置来加载国际化资源文件:spring: messages: basename: messages encoding: UTF-8
或者在配置类中定义
MessageSource
Bean:java@Configuration public class MessageConfig { @Bean public MessageSource messageSource() { ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource(); messageSource.setBasename("classpath:messages"); messageSource.setDefaultEncoding("UTF-8"); return messageSource; } }
-
使用国际化资源: 在代码中可以通过
MessageSource
来获取国际化消息。例如,在控制器中根据请求参数确定语言环境并获取对应的消息。 -
模板中的国际化: 如果使用 Thymeleaf 作为模板引擎,可以在模板中直接使用国际化消息。需要确保在
application.properties
中启用了国际化支持,并且在模板中使用#{}
表达式引用消息键。 -
自动检测客户端语言: Spring Boot 提供了
LocaleResolver
来自动检测和设置客户端的语言环境。可以使用AcceptHeaderLocaleResolver
或自定义的LocaleResolver
。 -
缓存本地语言设置: 若要将本地语言设置缓存,可以在自己的配置类中增加
LocaleChangeInterceptor
拦截器和实现LocaleResolver
方法。比如使用CookieLocaleResolver
将语言设置存储在 Cookie 中。 -
与 Spring Security 结合: 在使用 Spring Security 时,可以通过在资源文件中添加相应的消息并在 Spring Security 配置中使用这些消息来实现登录页面和错误信息的多语言支持。
示例
配置国际化yaml
java
spring:
messages:
encoding: UTF-8
basename: i18n/messages
profiles:
active: zh_CN
#-Dspring.profiles.active=en_US
英文
java
server:
port: 8000
spring:
jackson:
date-format: MM-dd-yyyy
中文
java
spring:
jackson:
date-format: yyyy-MM-dd
server:
port: 8000
国际化配置
java
package com.cokerlk.language;
import com.cokerlk.language.service.EnUSProductService;
import com.cokerlk.language.service.IProductService;
import com.cokerlk.language.service.ZhCNProductService;
import lombok.Data;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
@Configuration
@Data
public class I18NConfiguration {
@Value("${spring.profiles.active}")
private String locale;
@Profile("zh_CN")
@Bean
public IProductService zhCNBussService(){
return new ZhCNProductService();
}
@Profile("en_US")
@Bean
public IProductService enUSBussService(){
return new EnUSProductService();
}
}
产品接口
java
package com.cokerlk.language.service;
import java.util.Map;
public interface IProductService {
Map<String,String> getProduct();
}
中文产品
java
package com.cokerlk.language.service;
import com.cokerlk.language.I18NConfiguration;
import jakarta.annotation.Resource;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.MessageSource;
import java.util.Date;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
@Slf4j
public class ZhCNProductService implements IProductService {
@Resource
I18NConfiguration i18NConfiguration;
@Resource
MessageSource messageSource;
@Override
public Map getProduct() {
log.info("中文");
Map result = new HashMap();
result.put("create-date", new Date());
result.put("text", messageSource.getMessage("product_name", null, Locale.of(i18NConfiguration.getLocale())));
return result;
}
}
英文产品
java
package com.cokerlk.language.service;
import com.cokerlk.language.I18NConfiguration;
import jakarta.annotation.Resource;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.MessageSource;
import java.util.Date;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
@Slf4j
public class EnUSProductService implements IProductService {
@Resource
I18NConfiguration i18NConfiguration;
@Resource
MessageSource messageSource;
@Override
public Map<String,String> getProduct() {
log.info("英文");
Map result = new HashMap();
result.put("create-date", new Date());
result.put("text", messageSource.getMessage("product_name", null, Locale.of(i18NConfiguration.getLocale())));
return result;
}
}
message配置
java
#messages.properties
product_name=huawei mate 70
#messages_en_US.properties
product_name=Hua wei mate 70
#messages_zh_CN.properties
product_name=华为mate70
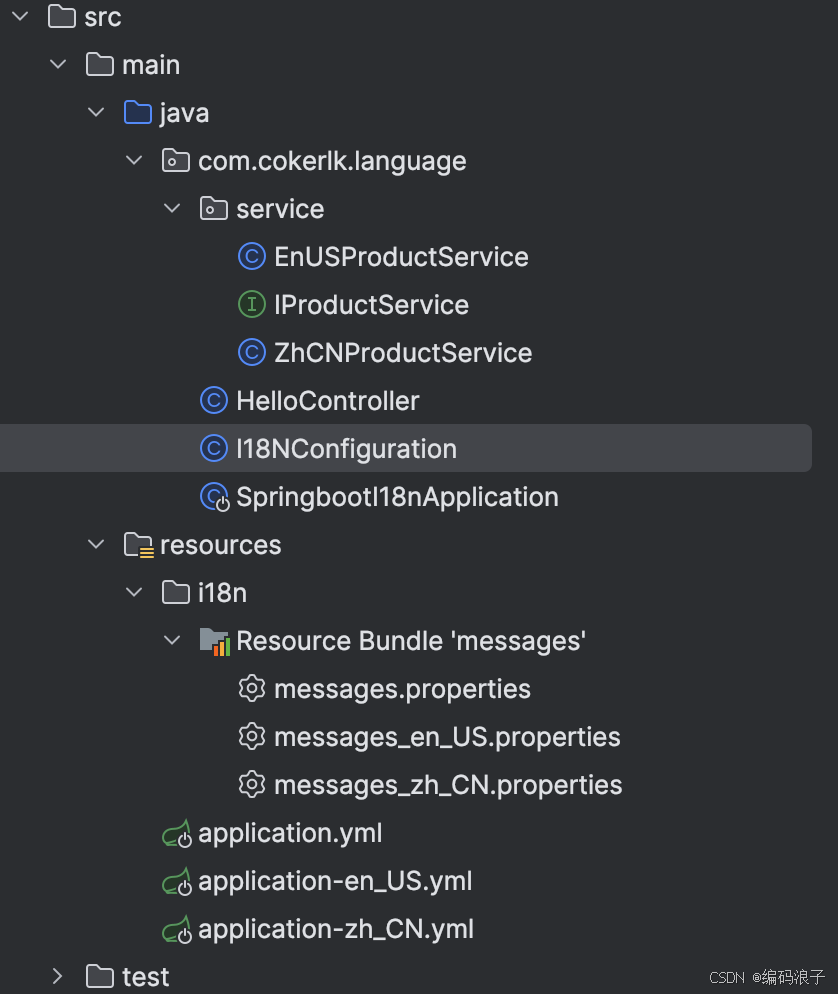
测试结果
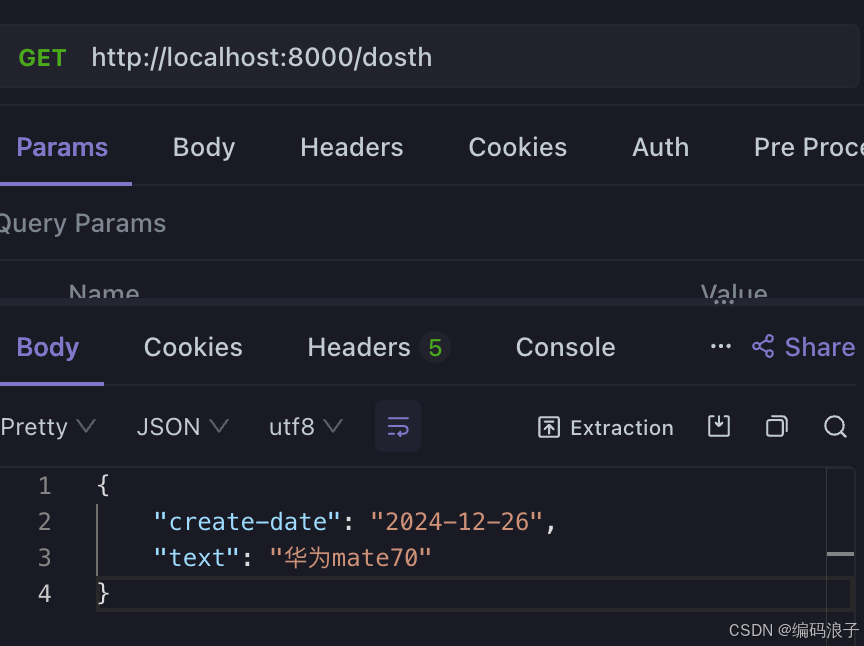