目录
[1.1 基础规则](#1.1 基础规则)
[1.2 以if为例子](#1.2 以if为例子)
[2 一些写法](#2 一些写法)
[2.1 时间相关](#2.1 时间相关)
[2.2 对数写法](#2.2 对数写法)
[2.3 关于使用random](#2.3 关于使用random)
[2.4 UnityEngine.Random.value](#2.4 UnityEngine.Random.value)
[2.5 PerlinNoise 函数](#2.5 PerlinNoise 函数)
[PerlinNoise 函数本身的输出范围](#PerlinNoise 函数本身的输出范围)
[3 各种报错](#3 各种报错)
[3.1 error cs1001; identifier expected](#3.1 error cs1001; identifier expected)
[3.2 error CS0103: The name 'Math' does not exist in the current context](#3.2 error CS0103: The name 'Math' does not exist in the current context)
[3.3 error CS0266: Cannot implicitly convert type 'double' to 'float'. An explicit conversion exists (are you missing a cast?)](#3.3 error CS0266: Cannot implicitly convert type 'double' to 'float'. An explicit conversion exists (are you missing a cast?))
[3.4 error CS0103: The name 'jointRecoil' does not exist in the current context](#3.4 error CS0103: The name 'jointRecoil' does not exist in the current context)
[3.5 error CS1503: Argument 1: cannot convert from 'double' to 'float](#3.5 error CS1503: Argument 1: cannot convert from 'double' to 'float)
[3.6 error CS0103: The name 'someMethod' does not exist in the current context](#3.6 error CS0103: The name 'someMethod' does not exist in the current context)
[3.7 error CS0117: 'Math' does not contain a definition for 'Norm'](#3.7 error CS0117: 'Math' does not contain a definition for 'Norm')
[3.8 error CS0246: The type or namespace name 'NextDouble' could not be found (are you missing a using directive or an assembly reference?)](#3.8 error CS0246: The type or namespace name 'NextDouble' could not be found (are you missing a using directive or an assembly reference?))
[3.9 随机相关的报错](#3.9 随机相关的报错)
[error CS0104: 'Random' is an ambiguous reference between 'UnityEngine.Random' and 'System.Random'](#error CS0104: 'Random' is an ambiguous reference between 'UnityEngine.Random' and 'System.Random')
[3.10 error CS0029: Cannot implicitly convert type '(double newX1, double newY1)' to 'UnityEngine.Vector2'](#3.10 error CS0029: Cannot implicitly convert type '(double newX1, double newY1)' to 'UnityEngine.Vector2')
1 C#基本语法格式
1.1 基础规则
- 大小写敏感
- 命名只能以字母或下划线开头
- 注释语句 // /* */
- 强类型语言,每个变量,表达式,编译时必须先声明,明确的类型
- 引用包,using System; 对比python用的是 import numpy
1.2 以if为例子
if ()
{
语句;
}
else if ()
{
语句;
}
else
{
语句;
}
2 一些写法
2.1 时间相关
Time.deltaTime
在 Unity 游戏开发中是一个非常重要的属性,它表示从上一帧到当前帧的时间间隔。其单位是秒,但是在 Unity 内部,它被处理成了浮点数,所以它并不是精确到毫秒。
Time.deltaTime
的主要用途是用于计算每帧应该移动多远,或者是计算动画应该播放多少。如果你在更新函数(如 Update()
或 FixedUpdate()
)中使用 Time.deltaTime
,它可以确保即使目标平台的性能差异巨大,你的游戏动画和物理更新仍然能正常运行。
单位是秒?
2.2 对数写法
- 自然对数,
- double naturalLog = Math.Log(number);
- // 计算以10为底的对数
- double log10 = Math.Log10(number)
有些其他地方写法是 log(number, e) log(number, 10) 这样的
2.3 关于使用random
- using System;
- Random random = new Random();
- int randomInt = random.Next(0, int.MaxValue);
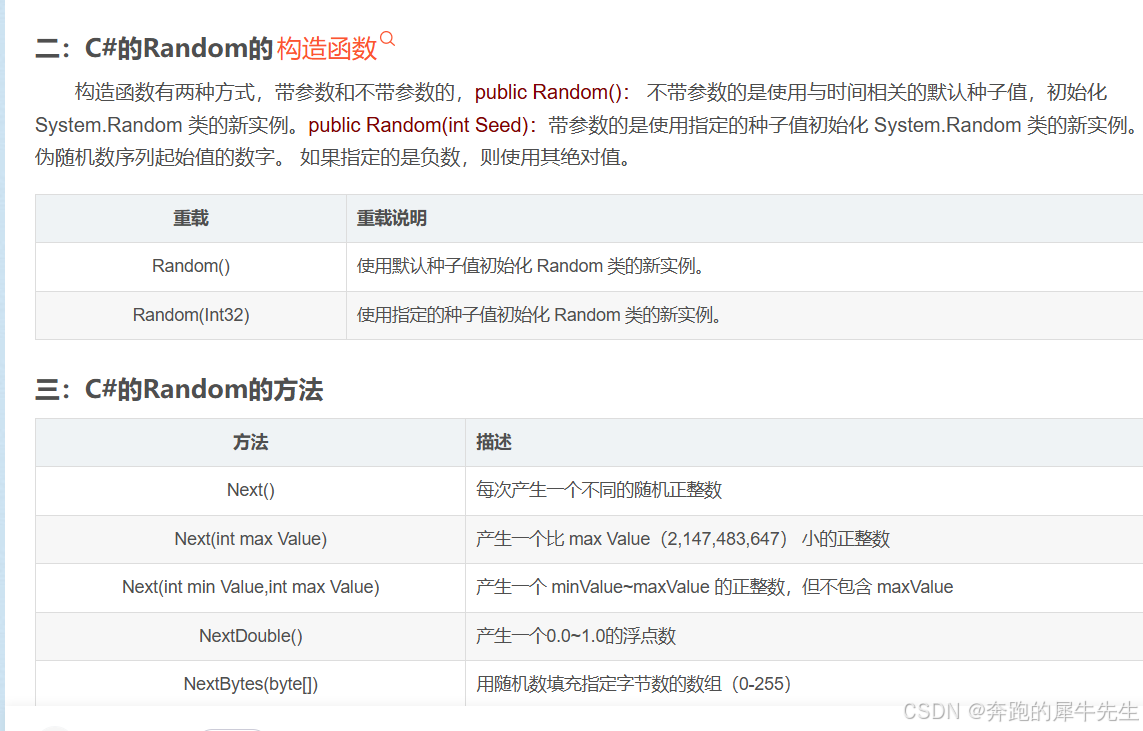
2.4 UnityEngine.Random.value
UnityEngine.Random.value 返回[0,1]
2.5 PerlinNoise
函数
PerlinNoise
函数本身的输出范围
在 Unity 中, Mathf.PerlinNoise
函数的返回值范围是在 [0, 1]
区间内。也就是说,对于单独调用 Mathf.PerlinNoise(x, y)
(其中 x
和 y
为传入的坐标参数),它返回的结果最小是 0
,最大是 1
currentJitter = new Vector2( Mathf.PerlinNoise(Time.time * 2, 0f) - 0.5f,Mathf.PerlinNoise(0f, Time.time * 2) - 0.5f ) * (2f * 0.1);
- 原始 Perlin 噪声值范围:Mathf.PerlinNoise
[0, 1]
- 减去 0.5 后范围:
[-0.5, 0.5]
- 乘以 0.2 后范围:
[-0.1, 0.1]
3 各种报错
3.0 unity里对C#报错内容超级详细
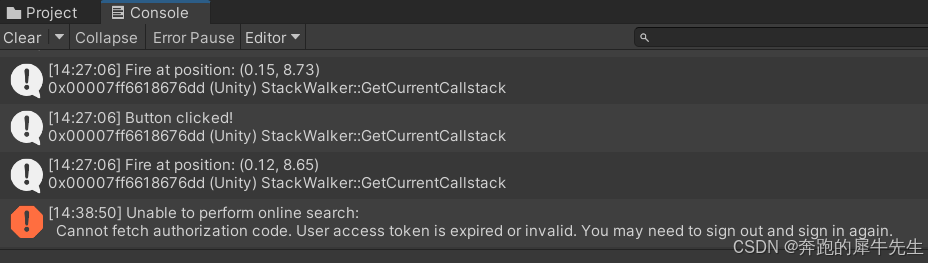
unity里,会自动报错,很好用
比如 cross.cs(28,19):error cs1001; identifier expected
表示
- cross.cs 文件的
- (line 28,19字符) 报错,
- 错误类型cs1001
- 错误内容 ; identifier expected
3.1 error cs1001; identifier expected
; identifier expected 表示,希望/需要这有;但是实际缺少标识符
一般是;
int = 10; // 这里缺少了变量名
Object obj = new(); // 这里缺少类名
3.2 error CS0103: The name 'Math' does not exist in the current context
- 很多方法,都需要引用math下的方法,比如math.sin()
- 但是,使用math包,和python一样,需要先导入
- C#需要文件开头加一句,using System;
3.3 error CS0266: Cannot implicitly convert type 'double' to 'float'. An explicit conversion exists (are you missing a cast?)
error CS0266: Cannot implicitly convert type 'double' to 'float'. An explicit conversion exists (are you missing a cast?)
报错解释:
错误 CS0266 表示不能隐式地将 double 类型转换为 float 类型。由于 double 类型具有比 float 更大的有效数字范围和精度,因此不能直接将 double 值赋给需要 float 类型的变量。
解决方法:
使用显式转换:通过使用强制转换操作符将 double 值转换为 float 类型。例如,如果有一个 double 变量 d,你可以通过 (float)d 来得到它的 float 近似值。
double x = 123.456;
float f = (float) x;
因为C# 是严格声明的,强类型的语言
有些地方要求是 double类型的,但是在其他一些地方需要用float,所以需要转换,不能直接用
为什么不能直接用,比如 double 直接当成float 会丢失部分数据的
C# 错误 CS0266 表示你正在尝试将 double
(双精度浮点数)赋值给 float
(单精度浮点数)变量,而没有进行显式转换。这是因为 double
的精度(64 位)高于 float
(32 位),从高精度类型隐式转换为低精度类型可能会导致数据丢失。
以下是修复此问题的方法,以及说明和最佳实践:
1. 显式转换:
最常见和最直接的解决方案是使用显式转换:
3.4 error CS0103: The name 'jointRecoil' does not exist in the current context
-
error CS0103: The name 'jointRecoil' does not exist in the current context
-
报错解释:
错误 CS0103 表示名为 'jointRecoil' 的标识符在当前上下文中不存在。这通常意味着你在代码中引用了一个变量、方法或类型,但是在你的当前作用域或者当前的命名空间中,编译器找不到这个名称的定义。
-
变量 jointRecoil 没有先声明类型就直接使用了!不行,必须先声明类型
3.5 error CS1503: Argument 1: cannot convert from 'double' to 'float
error CS1503: Argument 1: cannot convert from 'double' to 'float
报错解释:
错误 CS1503 表示方法调用的参数类型不匹配。具体来说,是指方法期望的是一个 float
类型的参数,但是你提供的是一个 double
类型的参数。
需要转换数值类型
3.6 error CS0103: The name 'someMethod' does not exist in the current context
error CS0103: The name 'someMethod' does not exist in the current context
使用的 someMethod 这个方法,并没有先在代码中实现
3.7 error CS0117: 'Math' does not contain a definition for 'Norm'
error CS0117: 'Math' does not contain a definition for 'Norm'
C# 里并没有默认的 Math.norm() 生成正态分布的方法
错误解释:
错误 CS0117 表示您尝试调用的 Math
类中不存在名为 Norm
的方法或定义。这通常意味着您正在尝试使用不存在的成员来执行操作。
3.8 error CS0246: The type or namespace name 'NextDouble' could not be found (are you missing a using directive or an assembly reference?)
error CS0246: The type or namespace name 'NextDouble' could not be found (are you missing a using directive or an assembly reference?)
错误解释:
错误 CS0246 表示编译器无法在当前上下文中找到名为 'NextDouble' 的类型或命名空间。这通常意味着 'NextDouble' 没有正确引用或定义。
就是没有先进行声明,确定明确的类型
解决方法:
确保您已经引用了包含 'NextDouble' 方法的命名空间。对于 .NET 中的随机数生成,您需要引用 System 命名空间,因为 NextDouble 方法定义在 System 命名空间下的 Random 类中。
3.9 随机相关的报错
error CS0104: 'Random' is an ambiguous reference between 'UnityEngine.Random' and 'System.Random'
错误 CS0104:"Random"是不明确的引用,存在于"UnityEngine.Random"和"System.Random"之间。
这个错误通常发生在 Unity (一个游戏开发引擎) 中,因为 UnityEngine
和 System
命名空间都包含一个名为 Random
的类。当你在代码中只使用 Random
时,编译器不知道你指的是哪一个。
以下是几种解决方法:
1. 明确指定命名空间(推荐):
最常见也是最推荐的解决方案是在声明或使用 Random
类时指定完整的命名空间
UnityEngine.Random.Range(0, 10); // 生成一个介于 0(包含)和 10(不包含)之间的随机整数
float randomFloat = UnityEngine.Random.value; // 生成一个介于 0.0f 和 1.0f(包含)之间的随机浮点数
3.10 error CS0029: Cannot implicitly convert type '(double newX1, double newY1)' to 'UnityEngine.Vector2'
错误 CS0029:"无法将类型'(double newX1, double newY1)'隐式转换为'UnityEngine.Vector2'"表示你正尝试将类型为 (double newX1, double newY1)
(一个包含两个 double 值的元组)的值直接赋给类型为 UnityEngine.Vector2
的变量。C# 不会自动将元组转换为 Vector2
。
以下是如何修复此问题以及每种解决方案的原理:
1. 显式创建 Vector2
:
这是最常见和推荐的解决方案。使用元组的值作为 x 和 y 分量创建一个新的 Vector2
对象。
using UnityEngine;
// ... 其他代码 ... (double newX1, double newY1) myTuple = (5.0, 3.2); // 示例元组
Vector2 myVector = new Vector2((float)myTuple.newX1, (float)myTuple.newY1);
// 或者使用解构语法,更简洁
(C# 7.0 及更高版本):
(double x, double y) = myTuple; Vector2 myVector2 = new Vector2((float)x, (float)y);
spearRadius=0.1*Math.Log(bulletsNumInCd*5);
double randomFloat = UnityEngine.Random.value;
angle1 = UnityEngine.Random.value * 360 ;
radius1 =UnityEngine.Random.value * spearRadius ;
newX1=radius1* Math.Cos(angle1*Math.PI/180);
newY1=radius1* Math.Sin(angle1*Math.PI/180);
//newPos1 = newX1 , newY1 ;
Vector2 newPos1 = new Vector2((float)newX1, (float)newY1);
不能这么写
newPos1 = (newX1, newY1);
newPos1 = ((float)newX1, (float)newY1);
必须
最常见的错误是尝试用逗号来创建一个类似"元组"的东西。C# 中没有内置的用逗号直接创建坐标或点的语法。你需要使用合适的类型,例如 Point
、PointF
、Vector2
或自定义的结构体。
根据你的项目类型和需求选择合适的方法。如果是在 Windows 窗体或 WPF 应用程序中处理图形,Point
或 PointF
通常就足够了。如果需要向量运算,则使用 Vector2
。如果需要更复杂的表示,则创建自定义的结构体。
请记住进行必要的类型转换,因为 Math.Cos
和 Math.Sin
返回 double
,而 Point
使用 int
,PointF
和 Vector2
使用 float
。
必须用下面这种结构体才能生成
Vector2 newPos1 = new Vector2((float)newX1, (float)newY1);