1. 141. 环形链表
给你一个链表的头节点 head ,判断链表中是否有环。
如果链表中有某个节点,可以通过连续跟踪 next 指针再次到达,则链表中存在环。 为了表示给定链表中的环,评测系统内部使用整数 pos 来表示链表尾连接到链表中的位置(索引从 0 开始)。注意:pos 不作为参数进行传递 。仅仅是为了标识链表的实际情况。
如果链表中存在环 ,则返回 true 。 否则,返回 false 。
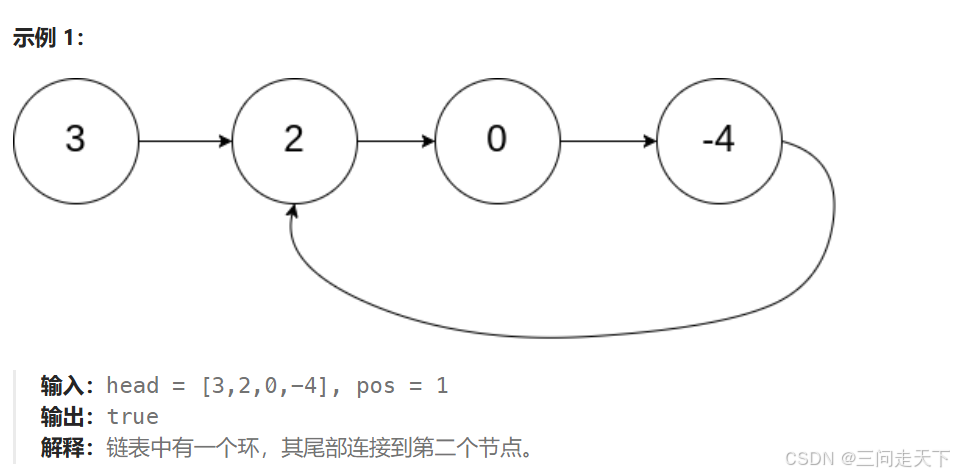
c
class Solution {
public:
bool hasCycle(ListNode *head) {
if (head == nullptr) return false;
ListNode* low = head, *fast = head;
while (fast && fast->next){
low = low->next;
fast = fast->next->next;
if (low == fast) return true;
}
return false;
}
};
2. 二叉树的最近公共祖先
给定一个二叉树, 找到该树中两个指定节点的最近公共祖先。
百度百科中最近公共祖先的定义为:"对于有根树 T 的两个节点 p、q,最近公共祖先表示为一个节点 x,满足 x 是 p、q 的祖先且 x 的深度尽可能大(一个节点也可以是它自己的祖先)。"
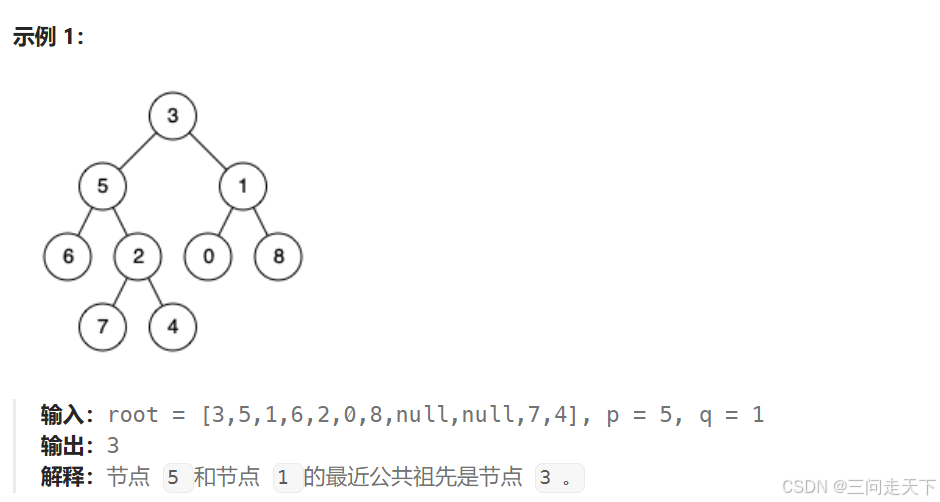
方法一:使用栈
c
class Solution {
public:
bool treePath(TreeNode* root, stack<TreeNode*> &st, TreeNode* target){
if (root == nullptr) return false;
st.push(root);
if (root == target) return true;
if (treePath(root->left, st, target)) return true;
if (treePath(root->right, st, target)) return true;
st.pop();
return false;
}
TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) {
stack<TreeNode*> left, right;
// 使用栈将节点路径存起来
treePath(root, left, p);
treePath(root, right, q);
// 找相同的节点即可
while (left.top() != right.top()){
if (left.size() > right.size()) left.pop();
else right.pop();
}
return left.top();
}
};
方法二:递归
c
class Solution {
public:
TreeNode* ret;
bool treePath(TreeNode* root, TreeNode* p, TreeNode* q)
{
if (root == nullptr) return false;
bool lson = treePath(root->left, p, q);
bool rson = treePath(root->right, p, q);
if ((lson && rson) || ((root->val == p->val || root->val == q->val) && (lson || rson)))
ret = root;
return lson || rson || (root->val == p->val || root->val == q->val);
}
TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) {
treePath(root, p, q);
return ret;
}
};