大纲
题目
地址
https://leetcode.com/problems/valid-word/description/
内容
A word is considered valid if:
- It contains a minimum of 3 characters.
- It contains only digits (0-9), and English letters (uppercase and lowercase).
- It includes at least one vowel.
- It includes at least one consonant.
You are given a string word.
Return true if word is valid, otherwise, return false.
Notes:
- 'a', 'e', 'i', 'o', 'u', and their uppercases are vowels.
- A consonant is an English letter that is not a vowel.
Example 1:
Input: word = "234Adas"
Output: true
Explanation:
This word satisfies the conditions.
Example 2:
Input: word = "b3"
Output: false
Explanation:
The length of this word is fewer than 3, and does not have a vowel.
Example 3:
Input: word = "a3 e " O u t p u t : f a l s e E x p l a n a t i o n : T h i s w o r d c o n t a i n s a ′ e" Output: false Explanation: This word contains a ' e"Output:falseExplanation:Thiswordcontainsa′' character and does not have a consonant.
Constraints:
- 1 <= word.length <= 20
- word consists of English uppercase and lowercase letters, digits, '@', '#', and '$'.
解题
这题要检测一个数是否符合以下特点:
- 至少包含3个字符
- 只能包含大小写字母和数字
- 至少有一个a、e、i、o、u大写或小写的字母
- 至少包含一个非a、e、i、o、u大写或小写的字母
解法也很简单,按这些条件把规则写好即可。
cpp
#include <string>
using namespace std;
class Solution {
public:
bool isValid(string word) {
if (word.size() < 3) {
return false;
}
bool vowel = false;
bool consonant = false;
for (char c : word) {
if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u'
|| c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U') {
vowel = true;
} else if ( (c >= 'a' && c <= 'z')
|| (c >= 'A' && c <= 'Z'))
{
consonant = true;
}
else if (c >= '0' && c <= '9') {
continue;
}
else {
return false;
}
}
return vowel && consonant;
}
};
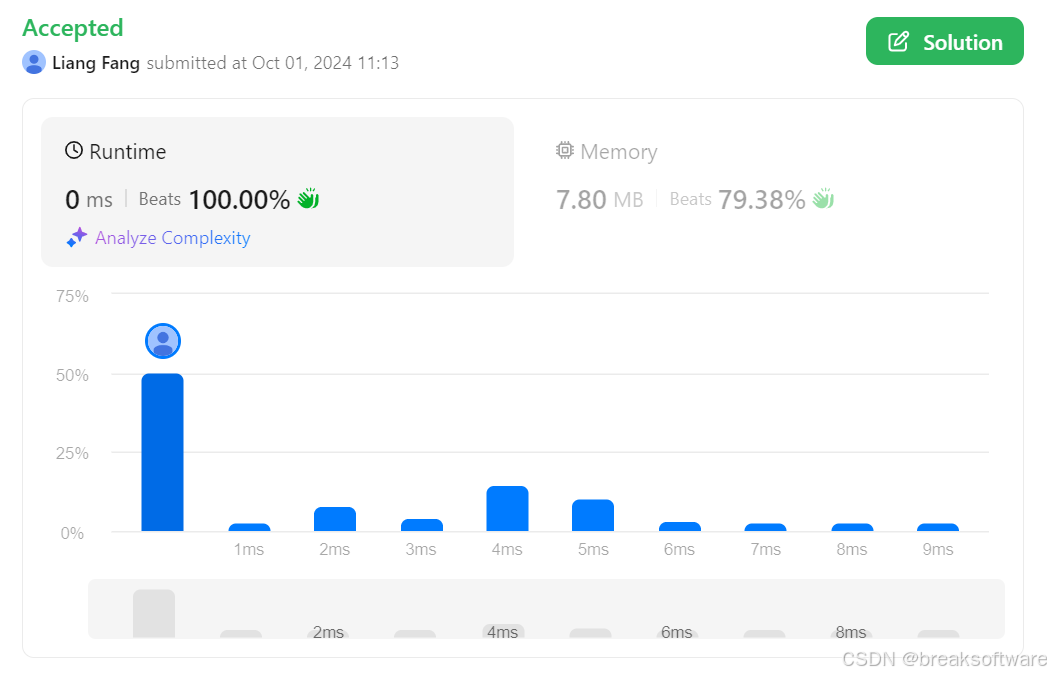
代码地址
https://github.com/f304646673/leetcode/tree/main/3136-Valid-Word/cplusplus