1、最小包围三角形 cv2.minEnclosingTriangle()
python
area,triangle = cv2.minEnclosingTriangle(contours)
**area:**最小包围三角形面积。
**triangle:**最小包围三角形的坐标,[[[230 60]],[[ 39 123]],[[185 269]]]。
**contours:**轮廓。
python
import cv2
import numpy as np
# 图像前处理
img = cv2.imread('m.png') # 原图
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # 灰度图
ret,img_binary = cv2.threshold(img_gray,127,255,cv2.THRESH_BINARY) # 二值图
# 找轮廓
contours,hierarchy = cv2.findContours(img_binary,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
# 找最小包围三角形
area,triangle = cv2.minEnclosingTriangle(contours[0])
# 定位点坐标
triangle = np.int32(triangle) # 这一步非常重要
print(triangle)
# 画轮廓
img1 = cv2.polylines(img.copy(),[triangle],True,(255,0,0),2)
cv2.imshow('img',img)
cv2.imshow('img1',img1)
cv2.waitKey(0)
cv2.destroyAllWindows()
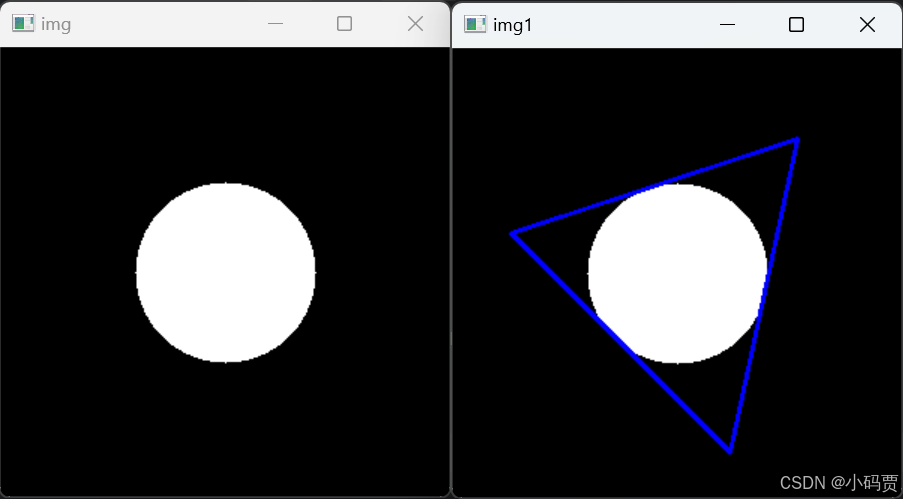
2、包围矩形 cv2.boundingRect()
python
x,y,w,h = cv2.boundingRect(contours)
**x,y,w,h:**函数返回值(x,y,w,h)元组,x,y代表矩形的左上角点坐标;w,h代表矩形宽高。
**contours:**轮廓。
python
import cv2
import numpy as np
# 图像前处理
img = cv2.imread('m.png') # 原图
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # 灰度图
ret,img_binary = cv2.threshold(img_gray,127,255,cv2.THRESH_BINARY) # 二值图
# 找轮廓
contours,hierarchy = cv2.findContours(img_binary,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
# 找包围***
x,y,w,h = cv2.boundingRect(contours[0])
# 画轮廓
img1 = cv2.rectangle(img.copy(),(x,y),(x+w,y+h),(255,0,0),2)
cv2.imshow('img',img)
cv2.imshow('img1',img1)
cv2.waitKey(0)
cv2.destroyAllWindows()
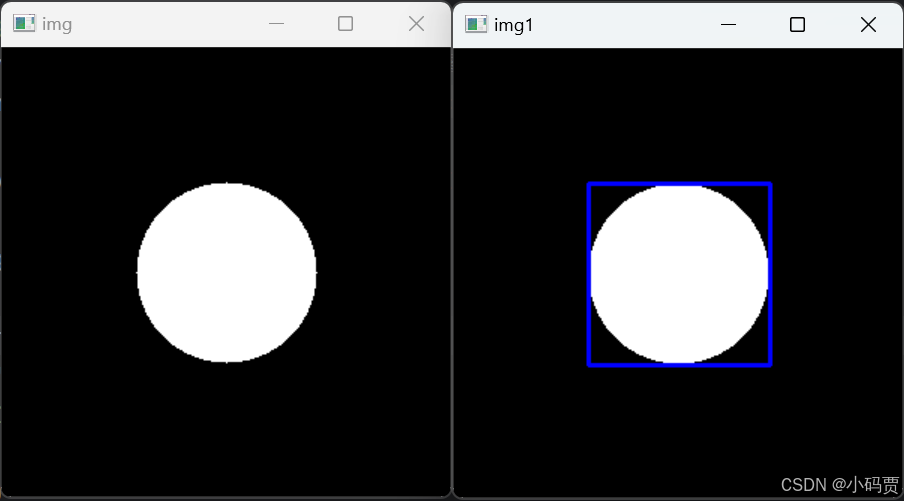
3、最小包围矩形 cv2.minAreaRect()
retval = cv2.minAreaRect(contours)
**retval:**函数返回值,((x,y),(w,h),angle),(x,y)矩形中心点坐标,(w,h)矩形的宽高,angle:旋转角度,正值为顺时针,负值为逆时针。
**contours:**轮廓。
points = cv2.boxPoints(retval)
**points:**矩形四个顶点坐标。
retval:cv2.minAreaRect() 函数返回值。
python
import cv2
import numpy as np
# 图像前处理
img = cv2.imread('m.png') # 原图
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # 灰度图
ret,img_binary = cv2.threshold(img_gray,127,255,cv2.THRESH_BINARY) # 二值图
# 找轮廓
contours,hierarchy = cv2.findContours(img_binary,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
# 找最小包围矩形
box = cv2.minAreaRect(contours[0])
# 定位点坐标
points = cv2.boxPoints(box)
points = np.int32(points)
# 画轮廓
img1 = cv2.drawContours(img.copy(),[points],0,(255,0,0),2)
cv2.imshow('img',img)
cv2.imshow('img1',img1)
cv2.waitKey(0)
cv2.destroyAllWindows()
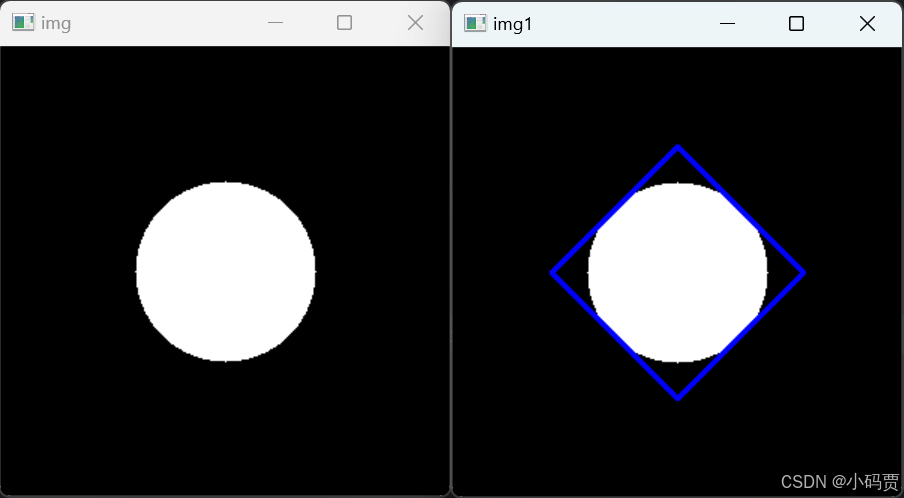
4、最小包围圆 cv2.minAreaRect()
python
center,radius = cv2.minEnclosingCircle(contours)
center:圆心的下(x,y)坐标。
radins:圆的半径。
contours:轮廓。
python
import cv2
import numpy as np
# 图像前处理
img = cv2.imread('fang.png') # 原图
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # 灰度图
ret,img_binary = cv2.threshold(img_gray,127,255,cv2.THRESH_BINARY) # 二值图
# 找轮廓
contours,hierarchy = cv2.findContours(img_binary,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
# 找包围***
(x,y),radius = cv2.minEnclosingCircle(contours[0])
# 数据处理
center = (int(x),int(y)) # 这一步非常重要
radius = int(radius)
# 画轮廓
img1 = cv2.circle(img.copy(),center,radius,(255,0,0),2)
cv2.imshow('img',img)
cv2.imshow('img1',img1)
cv2.waitKey(0)
cv2.destroyAllWindows()
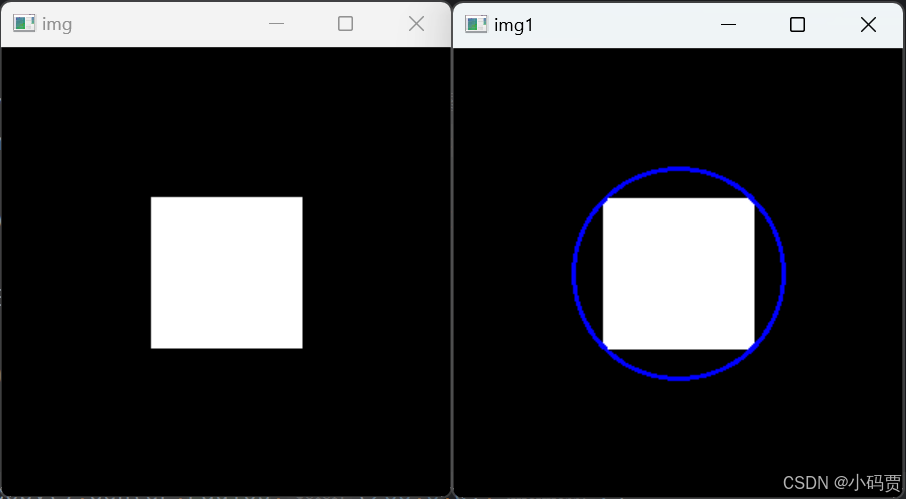
5、最优拟合椭圆cv2.minAreaRect()
python
ellipse = cv2.fitEllipse(contours)
**ellipse:**函数返回值,((x,y),(a,b),angle)。(x,y)中心点坐标;(a,b)长短轴直径;angle旋转角度,正值为顺时针,负值为逆时针。
**contours:**轮廓。
python
import cv2
import numpy as np
# 图像前处理
img = cv2.imread('juxing.png') # 原图
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # 灰度图
ret,img_binary = cv2.threshold(img_gray,127,255,cv2.THRESH_BINARY) # 二值图
# 找轮廓
contours,hierarchy = cv2.findContours(img_binary,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
print(len(contours[0])) # len(contours[0]) 必须大于 5 ,否则 ellipse = cv2.fitEllipse(contours[0])会报错
# 找包围***
ellipse = cv2.fitEllipse(contours[0])
# 画轮廓
img1 = cv2.ellipse(img.copy(),ellipse,(255,0,0),2)
cv2.imshow('img',img)
cv2.imshow('img1',img1)
cv2.waitKey(0)
cv2.destroyAllWindows()
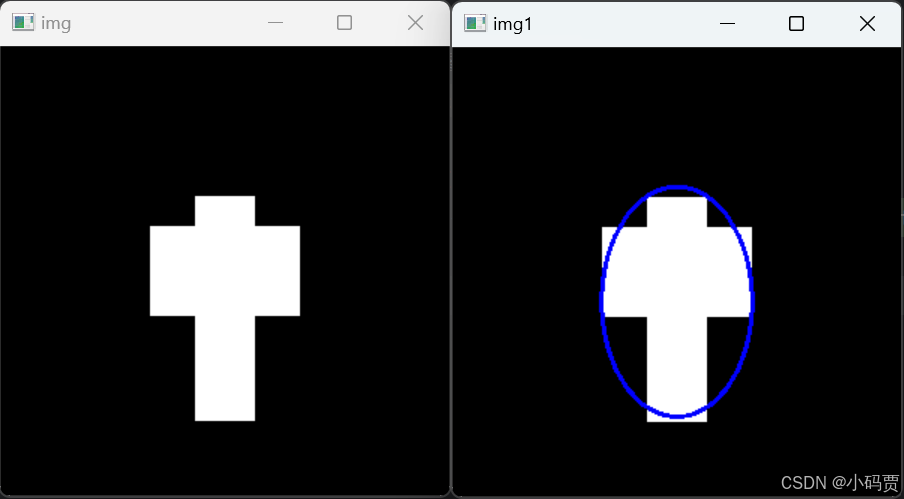