go
package Shape
import "math"
type Point struct{
X float64
Y float64
}
func (point *Point)GetDistance(other *Point) float64{
return math.Sqrt(math.Pow((point.X-other.X),2)+math.Pow((point.Y-other.Y),2))
}
go
package Circle
import "awesomeProject/Shape"
type Circle struct {
Heart Shape.Point
Radius float64
}
func (circle *Circle)IsPointInCircle(point *Shape.Point) bool{
return circle.Heart.GetDistance(point) <= circle.Radius
}
go
package main
import (
"awesomeProject/Circle"
"awesomeProject/Shape"
"fmt"
"math/rand"
"time"
)
const total = 1000000
func main() {
point := Shape.Point{1, 1}
circle := Circle.Circle{point, 1}
rand.Seed(time.Now().UnixNano())
count := 0
for i := 0; i < total;i++{
x := rand.Float64()*2
y := rand.Float64()*2
pointTmp := Shape.Point{x, y}
if(circle.IsPointInCircle(&pointTmp)){
count++
}
}
fmt.Println(float64(count * 4.0) / total)
}
go.mod
module awesomeProject
go 1.12
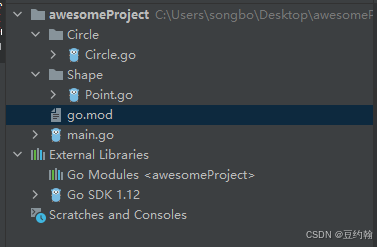