简介🎁
在图像处理领域,图像分割是一项基础且关键的技术,它涉及到将图像划分为若干个具有特定属性的区域。本文将通过一个实践项目,展示如何使用Python编程语言,结合OpenCV库,对一张玫瑰花的图片进行图像分割。这个项目不仅能够帮助我们理解图像分割的基本概念,还能够提供一个实际的编程示例。
目录
[完整代码 🍠](#完整代码 🍠)
环境准备🚚
在开始之前,请确保你的开发环境中已经安装了Python、NumPy、Matplotlib以及OpenCV(即skimage
和io
模块)。这些库可以通过pip进行安装。
项目步骤💂♀️
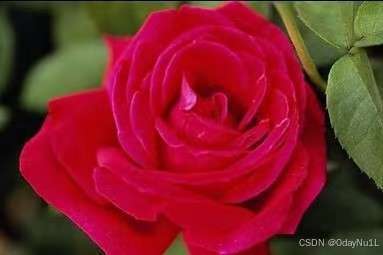
一:选取样本区域
首先,我们从图像中选取一个区域作为样本。在这个例子中,我们选择了图像中心的一个正方形区域。
python
from skimage import data, io
import numpy as np
# 读取图像
image = io.imread('flower.jpg')
# 选取样本区域
height, width, _ = image.shape
roi_size = 100 # 样本区域的边长
roi_center_x = width // 2
roi_center_y = height // 2
roi = image[roi_center_y - roi_size//2:roi_center_y + roi_size//2,
roi_center_x - roi_size//2:roi_center_x + roi_size//2]
二:计算标准差
接下来,我们计算所选区域红色通道的标准差,这将用于后续的图像分割。
python
# 提取红色通道并计算标准差
red_channel = roi[:, :, 0]
mean_value = np.mean(red_channel)
std_dev = np.std(red_channel)
三:建立模板图像空间
基于计算出的标准差,我们建立一个模板图像空间,用于区分图像中的不同区域。
python
# 建立模板图像空间
template_image = np.zeros_like(image, dtype='uint8')
for y in range(height):
for x in range(width):
if abs(image[y, x, 0] - mean_value) <= std_dev:
template_image[y, x] = image[y, x]
else:
template_image[y, x] = [0, 0, 0] # 将不符合条件的像素设置为黑色
四:根据模板图像空间分割原图像
最后,我们使用模板图像空间来分割原始图像,得到最终的分割结果。
python
# 分割原图像
segmented_image = np.where(template_image != 0, image, 0)
显示结果🥘
使用Matplotlib库,我们可以将原始图像、模板图像以及分割后的图像展示出来,以便进行比较。
python
from matplotlib import pyplot as plt
# 显示结果
plt.figure(figsize=(12, 6))
plt.subplot(1, 3, 1)
plt.title('Original Image')
plt.imshow(image)
plt.axis('off')
plt.subplot(1, 3, 2)
plt.title('Template Image')
plt.imshow(template_image)
plt.axis('off')
plt.subplot(1, 3, 3)
plt.title('Segmented Image')
plt.imshow(segmented_image)
plt.axis('off')
plt.show()
完整代码 🍠
python
from skimage import data, io
from matplotlib import pyplot as plt
import numpy as np
import math
# 读取图像
image = io.imread(r'flower.jpg')
# 一:选取样本区域
# 假设我们选取图像中心的一个正方形区域作为样本区域
height, width, _ = image.shape
roi_size = 100 # 样本区域的边长
roi_center_x = width // 2
roi_center_y = height // 2
roi = image[roi_center_y - roi_size//2:roi_center_y + roi_size//2,
roi_center_x - roi_size//2:roi_center_x + roi_size//2]
# 提取红色通道
red_channel = roi[:, :, 0]
# 二:计算标准差
mean_value = np.mean(red_channel)
std_dev = np.std(red_channel)
# 三:建立模板图像空间
r1_d = std_dev
template_image = np.zeros_like(image, dtype='uint8')
for y in range(height):
for x in range(width):
if abs(image[y, x, 0] - mean_value) <= r1_d:
template_image[y, x] = image[y, x]
else:
template_image[y, x] = [0, 0, 0] # 将不符合条件的像素设置为黑色
# 四:根据模板图像空间分割原图像
segmented_image = np.where(template_image != 0, image, 0)
# 显示结果
plt.figure(figsize=(12, 6))
plt.subplot(1, 3, 1)
plt.title('Original Image')
plt.imshow(image)
plt.axis('off')
plt.subplot(1, 3, 2)
plt.title('Template Image')
plt.imshow(template_image)
plt.axis('off')
plt.subplot(1, 3, 3)
plt.title('Segmented Image')
plt.imshow(segmented_image)
plt.axis('off')
plt.show()
结论💢
通过这个项目,我们学习了如何使用Python和OpenCV进行图像分割。这个过程涉及到了样本区域的选择、标准差的计算、模板图像空间的建立以及最终的图像分割。这个技术在许多领域都有广泛的应用,比如医学影像分析、自动驾驶车辆的视觉系统等。希望这个项目能够为你提供一些启发和帮助。