开发《飞机大战》游戏:Pygame 经典射击游戏教程
运行效果
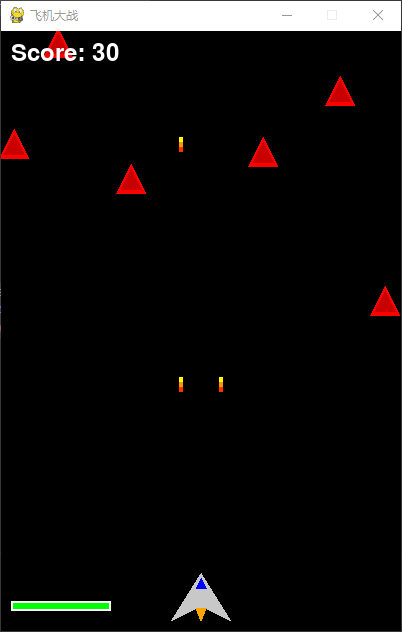
飞机有点丑,其他功能等待你的开发。
介绍
在这篇文章中,我们将使用 Pygame 库创建一个简单的经典射击游戏------《飞机大战》。该游戏具有多个功能,包括玩家飞机、敌机、子弹、道具、Boss 战斗等元素。游戏逻辑相对简单,但却能为新手提供良好的学习案例,帮助理解如何使用 Pygame 开发2D游戏。
本文将详细讲解游戏的各个部分,包括玩家控制、敌人生成、碰撞检测、Boss 机制以及游戏中的道具系统。
项目结构
我们将通过以下几个关键部分构建游戏:
- 游戏界面和窗口设置
- 玩家飞机类 (Player)
- 敌机类 (Enemy)
- 子弹类 (Bullet)
- 道具类 (PowerUp)
- Boss战斗类 (Boss)
- 碰撞检测和游戏循环
下面的代码展示了如何用 Pygame 组合这些元素,并通过简单的游戏机制实现飞行射击游戏。
代码解析
1. 初始化 Pygame
首先,我们初始化 Pygame 并设置窗口大小和游戏标题:
python
pygame.init()
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('飞机大战')
这里的 WINDOW_WIDTH
和 WINDOW_HEIGHT
定义了游戏窗口的大小,而 screen
对象则用来绘制所有游戏图形。
2. 颜色定义
在代码中,我们定义了一些常用颜色,方便后续绘制:
python
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
GREEN = (0, 255, 0)
ORANGE = (255, 165, 0)
PINK = (255, 192, 203)
HEALTH_GREEN = (0, 255, 0)
HEALTH_RED = (255, 0, 0)
3. 血条绘制函数
我们创建了一个 draw_health_bar
函数来显示玩家和 Boss 的血条。这是一个简单的矩形条,颜色根据血量比例变化:
python
def draw_health_bar(surf, x, y, health, max_health, width=100, height=10):
pct = health / max_health
fill = pct * width
outline_rect = pygame.Rect(x, y, width, height)
fill_rect = pygame.Rect(x, y, fill, height)
pygame.draw.rect(surf, HEALTH_RED, outline_rect)
if pct > 0:
pygame.draw.rect(surf, HEALTH_GREEN, fill_rect)
pygame.draw.rect(surf, WHITE, outline_rect, 2)
4. 玩家飞机类 (Player)
玩家飞机是游戏的核心控制对象,包含了如下功能:
- 飞行控制:通过键盘的左右方向键移动飞机。
- 自动射击:玩家可以启用自动射击,定期发射子弹。
- 道具系统:玩家可以拾取道具,获得速度提升、快速射击或护盾。
python
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface((60, 48), pygame.SRCALPHA)
pygame.draw.polygon(self.image, (200, 200, 200), [(30, 0), (0, 48), (25, 35), (35, 35), (60, 48)])
pygame.draw.polygon(self.image, BLUE, [(25, 15), (35, 15), (30, 5)])
pygame.draw.polygon(self.image, ORANGE, [(25, 35), (35, 35), (30, 48)])
self.rect = self.image.get_rect()
self.rect.centerx = WINDOW_WIDTH // 2
self.rect.bottom = WINDOW_HEIGHT - 10
self.speed = 5
self.shoot_delay = 250
self.last_shot = pygame.time.get_ticks()
self.auto_shoot = True
self.powerups = {}
self.shield = False
self.original_speed = self.speed
self.max_health = 100
self.health = self.max_health
玩家飞机拥有多个状态变量,如 speed
(速度)、shoot_delay
(射击延迟)等。通过 update()
方法,玩家飞机每帧更新位置和状态。
5. 敌机类 (Enemy)
敌机类负责生成敌人并让它们向下移动。敌机的碰撞检测和位置更新也在 update()
方法中完成。
python
class Enemy(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface((30, 30), pygame.SRCALPHA)
pygame.draw.polygon(self.image, RED, [(15, 0), (0, 30), (30, 30)])
self.rect = self.image.get_rect()
self.rect.x = random.randrange(WINDOW_WIDTH - self.rect.width)
self.rect.y = random.randrange(-100, -40)
self.speedy = random.randrange(2, 5)
self.speedx = random.randrange(-2, 3)
def update(self):
self.rect.y += self.speedy
self.rect.x += self.speedx
if self.rect.top > WINDOW_HEIGHT or self.rect.left < -25 or self.rect.right > WINDOW_WIDTH + 25:
self.rect.x = random.randrange(WINDOW_WIDTH - self.rect.width)
self.rect.y = random.randrange(-100, -40)
self.speedy = random.randrange(2, 5)
self.speedx = random.randrange(-2, 3)
6. Boss 战斗类 (Boss)
当玩家的分数达到300时,游戏会召唤出一个 Boss。Boss 有多个阶段,并且会发射不同类型的子弹。每个阶段的攻击方式不同,增加了游戏的难度。
python
class Boss(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface((100, 80), pygame.SRCALPHA)
pygame.draw.polygon(self.image, (150, 0, 0), [(50, 0), (0, 40), (20, 60), (80, 60), (100, 40)])
self.rect = self.image.get_rect()
self.rect.centerx = WINDOW_WIDTH // 2
self.rect.top = -100
self.speedx = 2
self.health = 500
self.max_health = 500
self.last_shot = pygame.time.get_ticks()
self.shoot_delay = 1000
self.phase = 1 # Boss战斗阶段
self.entering = True # Boss入场状态
7. 道具类 (PowerUp)
在敌机被击毁时,玩家有一定几率掉落道具,帮助玩家提升速度、射击频率或获得护盾。每种道具都有不同的效果和持续时间。
python
class PowerUp(pygame.sprite.Sprite):
def __init__(self, center):
super().__init__()
self.type = random.choice(list(POWERUP_TYPES.keys()))
self.image = pygame.Surface((20, 20), pygame.SRCALPHA)
pygame.draw.circle(self.image, POWERUP_TYPES[self.type]['color'], (10, 10), 10)
self.rect = self.image.get_rect()
self.rect.center = center
self.speedy = 3
def update(self):
self.rect.y += self.speedy
if self.rect.top > WINDOW_HEIGHT:
self.kill()
8. 游戏主循环
在主循环中,我们处理所有的事件、更新精灵状态、碰撞检测、道具生成以及绘制屏幕内容。
python
running = True
clock = pygame.time.Clock()
while running:
clock.tick(60)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
keys = pygame.key.get_pressed()
if keys[pygame.K_SPACE]:
player.shoot()
all_sprites.update()
hits = pygame.sprite.groupcollide(enemies, bullets, True, True)
for hit in hits:
score += 10
if random.random() < 0.2:
powerup = PowerUp(hit.rect.center)
all_sprites.add(powerup)
powerups.add(powerup)
hits = pygame.sprite.spritecollide(player, powerups, True)
for hit in hits:
player.apply_powerup(hit.type)
score += 5
hits = pygame.sprite.spritecollide(player, enemies, False)
if hits and not player.shield:
running = False
if score >= 300 and boss is None:
boss = Boss()
all_sprites.add(boss)
if boss:
hits = pygame.sprite.spritecollide(boss, bullets, True)
for hit in hits:
boss.health -= 10
if boss.health <= 0:
boss.kill()
boss = None
score += 200
hits = pygame.sprite.spritecollide(player, boss_bullets, True)
for hit in hits:
if not player.shield:
player.health -= 20
if player.health <= 0:
running = False
screen.fill(BLACK)
all_sprites.draw(screen)
score_text = font.render(f'Score: {score}', True, WHITE)
screen.blit(score_text, (10, 10))
powerup_y = 40
for powerup_type in player.powerups:
powerup_text = font.render(f'{powerup_type.title()} active!', True,
POWERUP_TYPES[powerup_type]['color'])
screen.blit(powerup_text, (10, powerup_y))
powerup_y += 30
draw_health_bar(screen, 10, WINDOW_HEIGHT - 30, player.health, player.max_health)
if boss:
draw_health_bar(screen, WINDOW_WIDTH//2 - 100, 20, boss.health, boss.max_health, 200)
pygame.display.flip()
总结
通过 Pygame,我们可以轻松地制作一个包含玩家飞机、敌机、子弹、道具以及 Boss 战斗的射击游戏。本文的代码展示了如何使用 Pygame 创建各种游戏对象,并通过精灵类管理它们的行为。
希望本文能帮助你了解如何用 Pygame 构建一个简单的游戏,并为你未来的游戏开发打下基础!