前言
Ajax的核心是XMLHttpRequest
对象,它允许浏览器在不刷新页面的情况下与服务器通信,从而实现局部更新页面内容的效果。本文将通过几个具体的代码示例,详细介绍如何使用XMLHttpRequest
对象进行GET和POST请求,并解释每个步骤的作用和注意事项。
1. 创建XMLHttpRequest对象
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 兼容性处理
let xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
console.log(xhr);
</script>
</body>
</html>
代码解析
在这段代码中,我们创建了一个XMLHttpRequest
对象。为了确保兼容性,我们使用了条件判断:
window.XMLHttpRequest
:现代浏览器都支持这种方式。new ActiveXObject('Microsoft.XMLHTTP')
:用于兼容IE6等旧版本浏览器。
通过这种方式,我们可以确保代码在不同浏览器环境下都能正常工作。
2. 发送GET请求
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 1. 创建XMLHttpRequest对象
let xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
// 2. 与服务器建立连接
xhr.open('get', 'http://localhost:8888/test/first', true);
// 3. 发送请求
xhr.send();
// 4. 等待响应
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && /^(2|3)\d{2}$/.test(xhr.status)) {
console.log(xhr.responseText);
}
};
</script>
</body>
</html>
代码解析
这段代码展示了如何发送一个GET请求:
- 创建对象:同上一步骤。
- 建立连接 :使用
xhr.open()
方法指定请求方式(GET)、URL和是否异步(true表示异步)。 - 发送请求 :调用
xhr.send()
方法发送请求。 - 等待响应 :通过
xhr.onreadystatechange
事件监听器来处理服务器响应。当readyState
为4且状态码为2xx或3xx时,表示请求成功,可以处理响应数据。
3. 发送POST请求
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="button" value="请求数据">
<script>
const btn = document.querySelector('input');
btn.onclick = function () {
let xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
xhr.open('post', 'http://localhost:8888/test/fourth', true);
xhr.onload = function () {
console.log(JSON.parse(xhr.responseText));
};
xhr.setRequestHeader('content-type', 'application/x-www-form-urlencoded');
xhr.send('name=张三&age=18');
};
</script>
</body>
</html>

代码解析
这段代码展示了如何发送一个POST请求:
- 获取页面元素 :通过
document.querySelector
选择按钮元素,并为其添加点击事件。 - 创建对象:同上。
- 建立连接 :使用
xhr.open()
方法指定请求方式(POST)、URL和是否异步。 - 注册事件 :设置
xhr.onload
事件处理函数,用于处理服务器返回的数据。 - 设置请求头 :使用
xhr.setRequestHeader()
方法设置请求头,指定内容类型为application/x-www-form-urlencoded
,即表单格式。 - 发送请求 :通过
xhr.send()
方法发送请求体数据,数据格式为键值对字符串。
4. 处理JSON数据
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE-edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
let str = '{"id": 1, "name": "苹果", "price": 30}';
console.log(str); // 输出原始JSON字符串
let obj = JSON.parse(str);
console.log(obj); // 输出解析后的对象
obj.price = 50; // 修改价格
str = JSON.stringify(obj);
console.log(str); // 输出修改后的JSON字符串
</script>
</body>
</html>
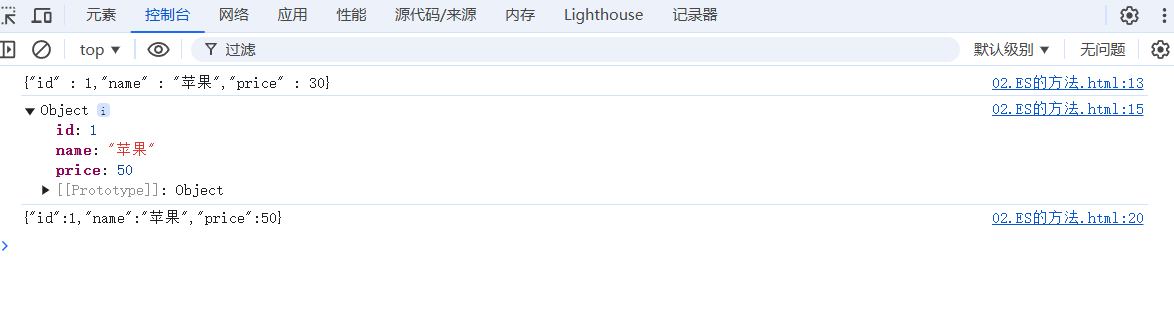
代码解析
这段代码展示了如何处理JSON数据:
- 定义JSON字符串:定义一个包含商品信息的JSON字符串。
- 解析JSON字符串 :使用
JSON.parse()
方法将JSON字符串转换为JavaScript对象。 - 修改对象属性:直接操作对象属性,如修改商品价格。
- 序列化对象 :使用
JSON.stringify()
方法将JavaScript对象转换回JSON字符串。
5. 同步与异步请求
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE-edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 异步请求
let xhrAsync = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
xhrAsync.open('get', 'http://localhost:8888/test/second', true);
xhrAsync.onload = function () {
console.log(JSON.parse(xhrAsync.responseText));
};
xhrAsync.send();
// 同步请求
let xhrSync = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
xhrSync.open('get', 'http://localhost:8888/test/second', false);
xhrSync.send();
console.log(JSON.parse(xhrSync.responseText));
</script>
</body>
</html>
代码解析
这段代码展示了同步和异步请求的区别:
- 异步请求 :通过设置
open()
方法的第三个参数为true
,请求会在后台执行,不会阻塞主线程。响应处理通过onload
事件完成。 - 同步请求 :通过设置
open()
方法的第三个参数为false
,请求会阻塞主线程,直到服务器响应返回。响应处理可以直接在send()
之后进行。
需要注意的是,同步请求会导致页面卡顿,通常不推荐使用,除非有特殊需求。
6. 表单提交与AJAX结合
代码片段
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE-edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="http://www.baidu.com" method="post">
<label for="">
请输入姓名:
<input type="text" name="userName" id="">
</label>
<label for="">
请输入密码:
<input type="password" name="password" id="">
</label>
<p><input type="submit" value="注册"></p>
</form>
<script>
const form = document.querySelector('form');
form.addEventListener('submit', function (event) {
event.preventDefault(); // 阻止默认提交行为
let xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
xhr.open('post', 'http://localhost:8888/test/register', true);
xhr.setRequestHeader('content-type', 'application/x-www-form-urlencoded');
xhr.onload = function () {
console.log(JSON.parse(xhr.responseText));
};
xhr.send(`userName=${form.userName.value}&password=${form.password.value}`);
});
</script>
</body>
</html>
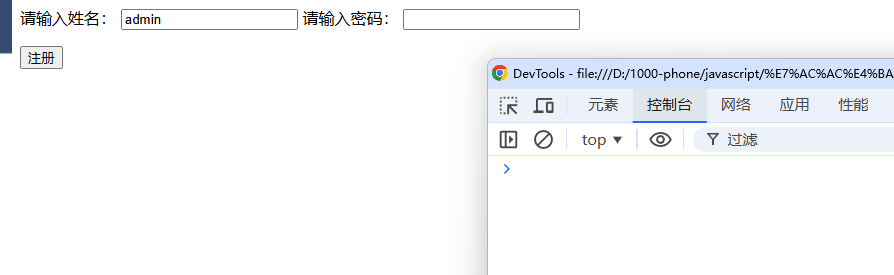
代码解析
这段代码展示了如何将表单提交与AJAX结合:
- 阻止默认提交行为 :通过
event.preventDefault()
防止表单自动提交。 - 创建并配置
XMLHttpRequest
对象:同上。 - 发送POST请求:从表单中获取用户输入的数据,并将其作为请求体发送。
- 处理响应 :通过
xhr.onload
事件处理服务器返回的数据。
结尾
通过上述代码示例,我们详细介绍了如何使用XMLHttpRequest
对象进行GET和POST请求、处理JSON数据、以及同步与异步请求的区别。这些技术点在实际开发中非常常用,掌握它们可以帮助开发者构建更加高效和响应迅速的Web应用。