使用cargo新建一个rust项目
bash
cargo new gui_demo
cd gui_demo
编辑Cargo.toml文件 ,添加iced依赖
rust
[package]
name = "gui_demo"
version = "0.1.0"
edition = "2021"
[dependencies]
iced = "0.4.2"
编辑src/main.rs文件:
rust
use iced::{button, widget::{Button, Column, Text}, Application, Command, Element, Settings, Subscription};
use iced::executor::Default as Executor;
// 定义应用程序的状态
struct RustGuiApp {
count: i32,
button_state: button::State,
}
// 定义应用程序的消息类型
#[derive(Debug, Clone, Copy)]
enum Message {
IncrementPressed,
}
impl Application for RustGuiApp {
type Executor = Executor;
type Message = Message;
type Flags = ();
fn new(_flags: ()) -> (Self, Command<Self::Message>) {
(
RustGuiApp {
count: 0,
button_state: button::State::new(),
},
Command::none(),
)
}
// 设置窗口标题
fn title(&self) -> String {
String::from("Rust GUI Example")
}
fn update(&mut self, message: Self::Message) -> Command<Self::Message> {
if let Message::IncrementPressed = message {
self.count += 1;
}
Command::none()
}
fn view(&mut self) -> Element<Self::Message> {
let button = Button::new(&mut self.button_state, Text::new("Increment"))
.on_press(Message::IncrementPressed);
// 添加文字和一些你想加的东西
Column::new()
.push(Text::new("Hello, Rust GUI!").size(50))
.push(Text::new(self.count.to_string()).size(50))
.push(button)
.into()
}
fn subscription(&self) -> Subscription<Self::Message> {
Subscription::none()
}
}
fn main() -> iced::Result {
RustGuiApp::run(Settings::default())
}
最后使用命令运行
bash
cargo run
运行效果展示,还是挺不错滴
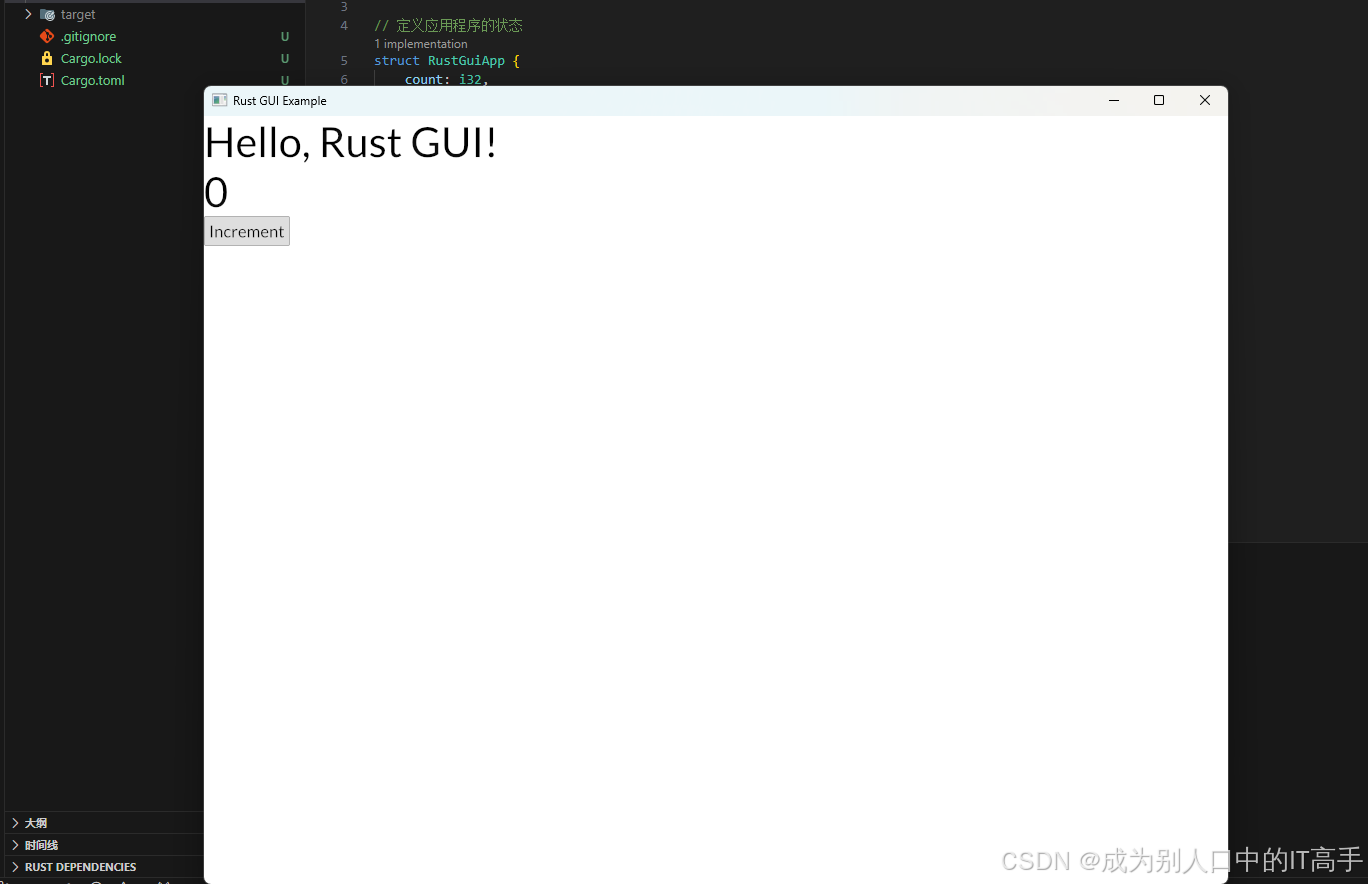