运算符重载
myString.h
#ifndef MYSTRING_H
#define MYSTRING_H
#include <cstring>
#include <iostream>
using namespace std;
class myString
{
private:
char *str; //记录c风格的字符串
int size; //记录字符串的实际长度
int capacity; //记录字符串的容量
public:
//无参构造
myString():size(10), capacity(10)
{
str = new char[size]; //构造出一个长度为10的字符串
}
//有参构造
myString(const char *s); //有参构造 string s("hello wirld");
//有参构造
myString(int n, char ch); //string s(5, 'A');
//析构函数
~myString();
void show();
//拷贝构造函数
myString(const myString &other);
//拷贝赋值函数
myString& operator=(const myString &other);
//判空函数
bool empty() const;
//size函数
int getSize() const;
//c_str函数
const char* c_str() const;
//at函数
char &at(int index);
//二倍扩容
void resize(int newCapacity);
//实现+=运算符重载
myString& operator+=(const myString &other);
//取地址运算符重载
myString* operator&();
//将[]运算符重载
char& operator[](const int index);
//
//将+重载
myString& operator+(const myString &other);
//将==重载
bool operator==(const myString &other) const;
//将!=重载
bool operator!=(const myString &other) const;
//将>重载
bool operator>(const myString &other) const;
//将<重载
bool operator<(const myString &other) const;
//将>=重载
bool operator>=(const myString &other) const;
//将<=重载
bool operator<=(const myString &other) const;
// 友元函数,重载<<运算符
friend ostream& operator<<(ostream &os, const myString &s)
{
os << s.str;
return os;
}
// 友元函数,重载>>运算符
friend istream& operator>>(istream &is, const myString &s)
{
is>> s.str;
return is;
}
};
#endif // MYSTRING_H
myString.cpp
#include"myString.h"
//有参构造
myString::myString(const char *s)
{
if(s)
{
size=strlen(s);
capacity=size+1;
str=new char[size];
strcpy(str, s);
}else {
size = 0;
capacity = 10;
str = new char[size];
}
}
//有参构造
myString::myString(int n, char ch): size(n), capacity(n + 1)
{
str = new char[size];
memset(str, ch, n);
}
//析构函数
myString::~myString()
{
delete[]str;
}
void myString::show()
{
cout<<"字符串为:"<<this->str<<endl;
}
//拷贝构造函数
myString::myString(const myString &other): size(other.size), capacity(other.capacity)
{
str = new char[size];
strcpy(str, other.str);
}
//拷贝赋值函数
myString &myString::operator=(const myString &other)
{
if (this != &other)
{
delete[] str;
size = other.size;
capacity = other.capacity;
str = new char[size];
strcpy(str, other.str);
}
return *this;
}
//判空函数
bool myString::empty() const
{
return size == 0;
}
//size函数
int myString::getSize() const
{
return size;
}
// c_str函数
const char *myString::c_str() const
{
return str;
}
// at函数
char &myString::at(int index)
{
if (empty()||index < 0 || index >= size)
{
cout<<"访问元素失败"<<endl;
}
return str[index];
}
//二倍扩容
void myString::resize(int newCapacity)
{
char *newStr = new char[newCapacity];
strcpy(newStr, str);
delete[] str;
str = newStr;
capacity = newCapacity;
}
//实现+=运算符重载
myString &myString::operator+=(const myString &other)
{
int newSize = size + other.size;
if (newSize >= capacity) {
resize(newSize * 2);
}
strcat(str, other.str);
size = newSize;
return *this;
}
//取地址运算符重载
myString *myString::operator&()
{
return this;
}
//将[]运算符重载
char &myString::operator[](const int index)
{
if(index<0||index>=size)
{
cout<<"重载失败"<<endl;
}
return str[index];
}
//将+重载
myString &myString::operator+(const myString &other)
{
int newSize=size+other.size;
if (newSize >= capacity) {
resize(newSize * 2);
}
strcpy(this->str,str);
strcat(this->str,other.str);
return *this;
}
//将==重载
bool myString::operator==(const myString &other) const
{
return strcmp(str,other.str)==0;
}
//将!=重载
bool myString::operator!=(const myString &other) const
{
return strcmp(str,other.str)!=0;
}
//将>重载
bool myString::operator>(const myString &other) const
{
return strcmp(str,other.str)>0;
}
//将<重载
bool myString::operator<(const myString &other) const
{
return strcmp(str,other.str)<0;
}
//将>=重载
bool myString::operator>=(const myString &other) const
{
return strcmp(str,other.str)>=0;
}
//将<=重载
bool myString::operator<=(const myString &other) const
{
return strcmp(str,other.str)<=0;
}
main.cpp
#include"myString.h"
int main()
{
myString s1("Hello");
myString s2(" World");
s1 += s2;
s1.show(); // 输出 "Hello World"
cout << "size: " << s1.getSize() << endl; // 输出 "Size: 11"
cout<<s1[0]<<endl;
myString s3=s1+s2;
s3.show();
myString s4("aaaaa");
myString s5("bbbbb");
if(s4==s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
if(s4!=s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
if(s4>s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
if(s4<s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
if(s4>=s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
if(s4<=s5)
{
cout<<"yes"<<endl;
}else{
cout<<"no"<<endl;
}
myString s6;
cin>>s6;
s6.show();
return 0;
}
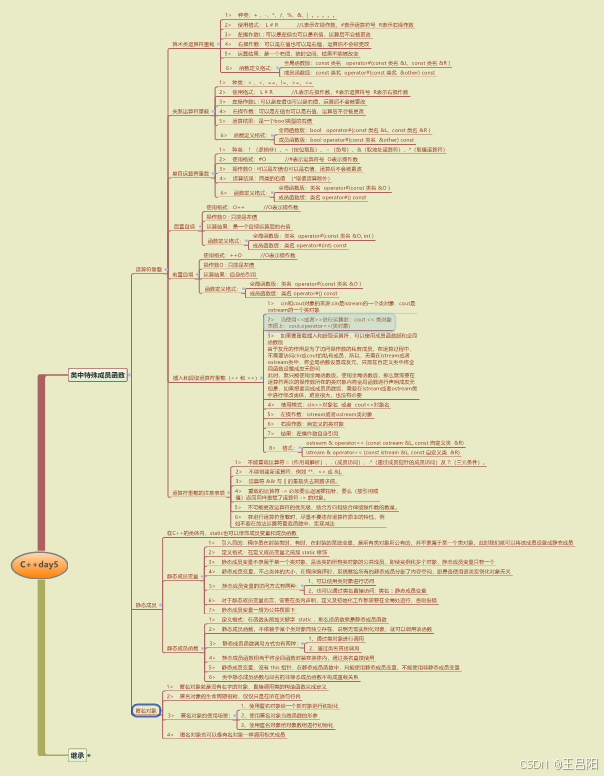
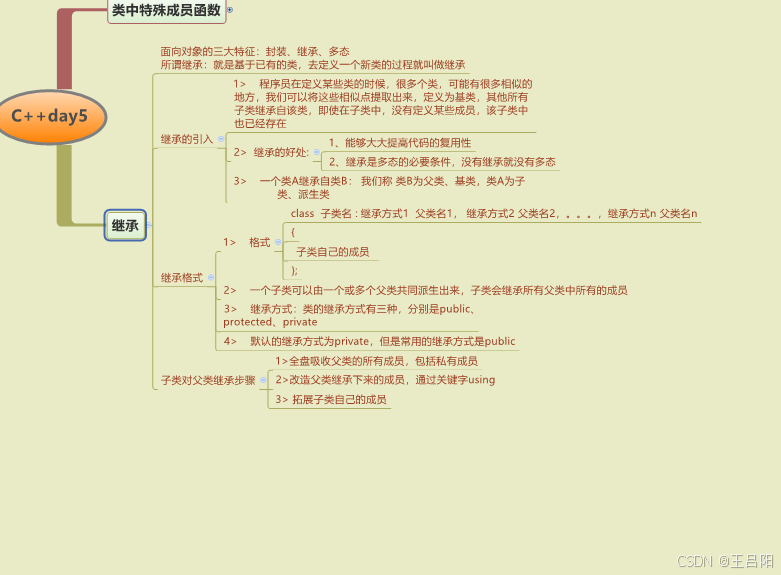