目录
[1 gameObject 和component](#1 gameObject 和component)
[2.1 使用 this.gameObject 或gameObject(注意大小写)](#2.1 使用 this.gameObject 或gameObject(注意大小写))
[2.2 获得其他信息](#2.2 获得其他信息)
[3 获取其他 GameObject的方法](#3 获取其他 GameObject的方法)
[3.1 获得自身挂载的GameObject](#3.1 获得自身挂载的GameObject)
[3.2 用find去查找其他的GameObject的名字或tag](#3.2 用find去查找其他的GameObject的名字或tag)
[3.3 去查找关联的GameObject](#3.3 去查找关联的GameObject)
[4 激活状态非为2种](#4 激活状态非为2种)
[4.1 Cube1.activeInHierarchy](#4.1 Cube1.activeInHierarchy)
[4.2 Cube1.activeSelf)](#4.2 Cube1.activeSelf))
[5 获取GameObject 上的组件](#5 获取GameObject 上的组件)
[5.1 最基础的Component组件:transform](#5.1 最基础的Component组件:transform)
[5.1.1 获得 transform组件下的具体属性最容易](#5.1.1 获得 transform组件下的具体属性最容易)
[5.2 通用方法 GetComponent<>() 方法](#5.2 通用方法 GetComponent<>() 方法)
[5.3 获取父子物体上的组件( 这几个方法,参数没搞清楚,待修改)](#5.3 获取父子物体上的组件( 这几个方法,参数没搞清楚,待修改))
[5.4 添加组件](#5.4 添加组件)
[5.5 设置激活状态](#5.5 设置激活状态)
[6 通过代码添加物体,](#6 通过代码添加物体,)
[6.1 先声明](#6.1 先声明)
[6.2 进行拖动关联](#6.2 进行拖动关联)
[6.3 生成](#6.3 生成)
[6.4 设置位置等](#6.4 设置位置等)
[6.5 销毁](#6.5 销毁)
1 gameObject 和component
- gameobject 类
- component组件时挂在gameobject上的,包括各种C#脚本
2 gameObject 与C#脚本
- C# 脚本必须挂到一个hierarchy里的gameobject上才能生效
- 但是怎么在C#脚本里,知道它 挂载它的 gameobject是谁呢?
2.1 使用 this.gameObject 或gameObject(注意大小写)
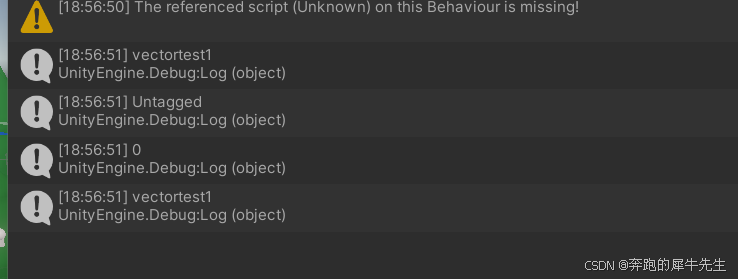
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class emptyTest : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
//使用this获取脚本挂在的gameObject
GameObject gb1=this.gameObject;
Debug.Log(gb1.name);
Debug.Log(gb1.tag);
Debug.Log(gb1.layer);
//节省的写法,也就是this.gameObject 或gameObject 指向挂载物体
//也就是gameobject就默认是自身了,可以省略this
Debug.Log(gameObject.name);
}
// Update is called once per frame
void Update()
{
}
}
2.2 获得其他信息
- Debug.Log(gb1.name);
- Debug.Log(gb1.tag);
- Debug.Log(gb1.layer);
3 获取其他 GameObject的方法
3.1 获得自身挂载的GameObject
- 获得自身就是
- this.gameObject
- 或者 省略this
- gameObject
3.2 用find去查找其他的GameObject的名字或tag
-
GameObject.Find("Cube1")
-
GameObject.FindWithTag("111")
-
GameObject test1=GameObject.Find("Cube1");
-
test1=GameObject.FindWithTag("111"); // 假设它的tag是独一无二的111
3.3 去查找关联的GameObject
- 在脚本里声明一个 GameObject
- 然后 unity编辑器里可以看到, C#脚本下面,会有名字为Cube1的公共变量。实际是GameObject 类型,然后把现在 已经创建的 Cube1 拖动过去
- 相当于,C#脚本里的 Public GameObject Cube1; 通过拖动的方式,已经被赋值了,赋的就是关联上的这个真实的Cube1
- 然后脚本里,因为声明了Cube1,且Cube1 也有值,就可以直接使用Cube1了。读到其属性都可以
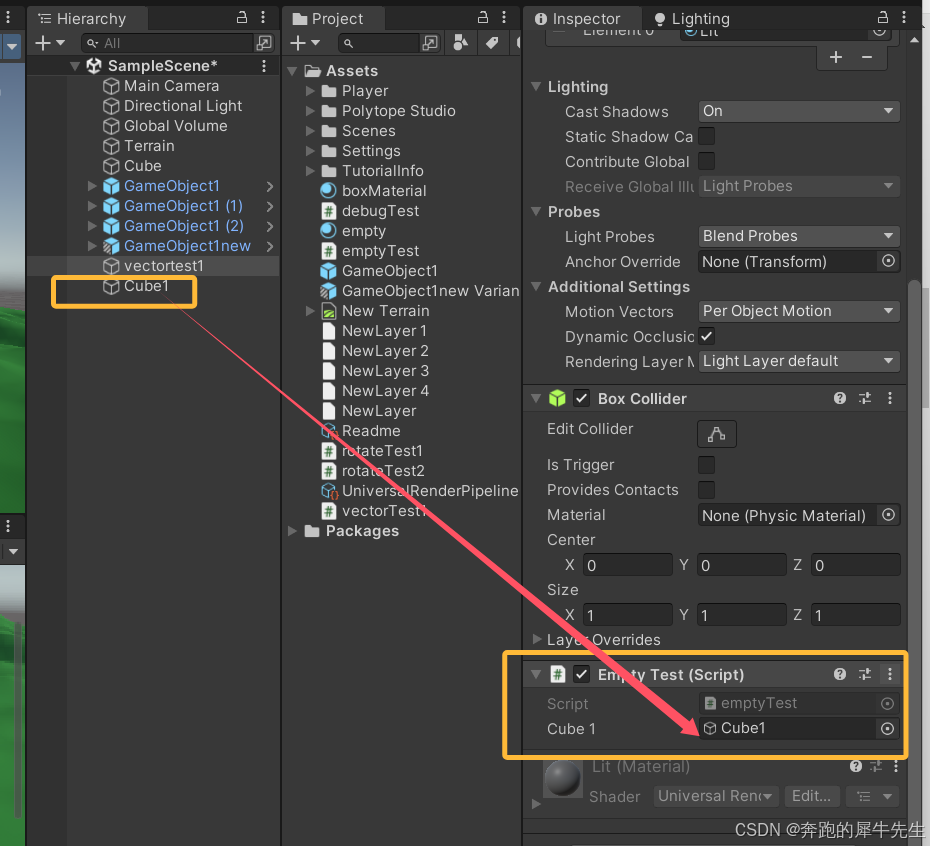
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class emptyTest : MonoBehaviour
{
public GameObject Cube1;
// Start is called before the first frame update
void Start()
{
//使用this获取脚本挂在的gameObject
GameObject gb1=this.gameObject;
Debug.Log(gb1.name);
Debug.Log(gb1.tag);
Debug.Log(gb1.layer);
//节省的写法,也就是this.gameObject 或gameObject 指向挂载物体
//也就是gameobject就默认是自身了,可以省略this
Debug.Log(gameObject.name);
//直接查找其他 GameObject的名字
GameObject test1=GameObject.Find("Cube1");
//test1=GameObject.FindWithTag("Cube1");
Debug.Log(test1.name);
//对和脚本没直接关联的 GameObject,不能直接读到,必须先用find
//Debug.Log(Cube.name);
//通过声明public,且挂载了Cuble1,可以直接读到 gb
Debug.Log(Cube1.name);
Debug.Log(Cube1.activeInHierarchy);
Debug.Log(Cube1.activeSelf);
}
// Update is called once per frame
void Update()
{
}
}
4 激活状态非为2种
4.1 Cube1.activeInHierarchy
- 表示这个GameObject在真实场景Hierarchy 是否真的激活了
- 因为有可能某个物体有父物体,上面的层级如果激活勾掉了,本身active实际也是没激活的
4.2 Cube1.activeSelf)
- 表示这个GameObject在自身的激活勾选是否打上了
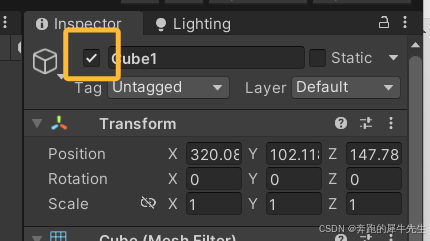
5 获取GameObject 上的组件
5.1 最基础的Component组件:transform
- 前面学习了,每个GameObject都有许多component
- 其中必须有的一个就是transform
- 即使是创建的一个空的 GameObject 都有这个transform
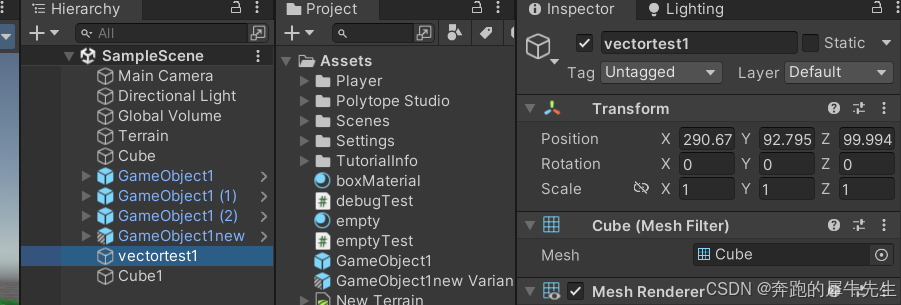
5.1.1 获得 transform组件下的具体属性最容易
直接使用下面的这两种写法即可
- this.transform
- transform
然后,因为transform组件,包括position, Rotation, Scale这几个属性,直接引用其属性即可
-
实测OK的属性
-
Debug.Log(this.transform.position);
-
Debug.Log(transform.position);
-
Debug.Log(transform.rotation);
-
Debug.Log(transform.right);
-
Debug.Log(transform.forward);
-
Debug.Log(transform.up);
-
Debug.Log(transform.localPosition);
-
Debug.Log(transform.localRotation);
-
Debug.Log(transform.localScale);
-
实测有问题的属性如下
-
//Debug.Log(transform.scale); //UNITY的全局缩放需要用transform.lossyScale
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class emptyTest : MonoBehaviour
{
public GameObject Cube1;
// Start is called before the first frame update
void Start()
{
//使用this获取脚本挂在的gameObject
GameObject gb1=this.gameObject;
Debug.Log(gb1.name);
Debug.Log(gb1.tag);
Debug.Log(gb1.layer);
//节省的写法,也就是this.gameObject 或gameObject 指向挂载物体
//也就是gameobject就默认是自身了,可以省略this
Debug.Log(gameObject.name);
//直接查找其他 GameObject的名字
GameObject test1=GameObject.Find("Cube1");
//test1=GameObject.FindWithTag("Cube1");
Debug.Log(test1.name);
//对和脚本没直接关联的 GameObject,不能直接读到,必须先用find
//Debug.Log(Cube.name);
//通过声明public,且挂载了Cuble1,可以直接读到 gb
Debug.Log(Cube1.name);
Debug.Log(Cube1.activeInHierarchy);
Debug.Log(Cube1.activeSelf);
// 获得组件的属性
Debug.Log(this.transform.position);
Debug.Log(transform.position);
Debug.Log(transform.localPosition);
Debug.Log(transform.rotation);
Debug.Log(transform.localRotation);
//Debug.Log(transform.scale);
Debug.Log(transform.lossyScale);
Debug.Log(transform.localScale);
Debug.Log(transform.right);
Debug.Log(transform.forward);
Debug.Log(transform.up);
// 获得组件的属性,通用的方法
}
// Update is called once per frame
void Update()
{
}
}
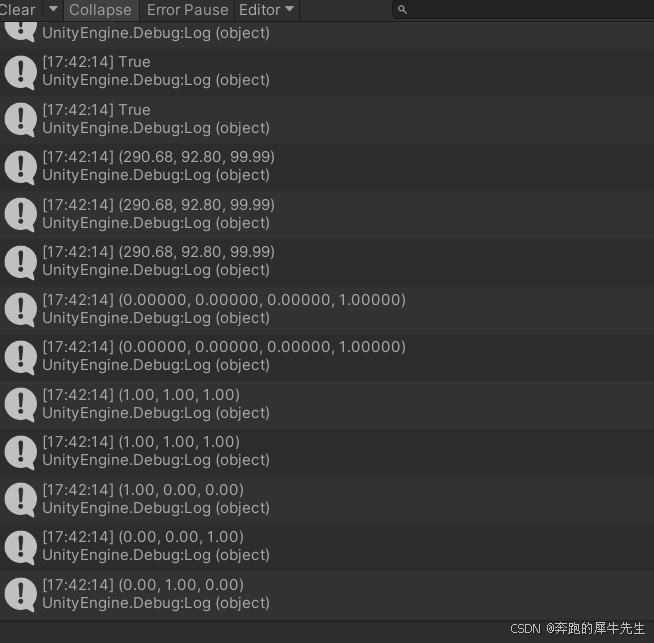
5.2 通用方法 GetComponent<>() 方法
- GetComponent<>()
- 比如
- GetComponent<BoxCollider>()
// 获得组件的属性,通用的方法
Component c1=GetComponent<BoxCollider>();
BoxCollider c2=GetComponent<BoxCollider>();
Debug.Log(c1);
Debug.Log(c2);
Debug.Log(c1.name);
Debug.Log(c2.center);
实际写,发现有2个要注意的点
因为我现在并没有系统学习C#, 学到这,对unity里的C#的命名规范的推测大致是
像各种类型,比如 Vector3, GameObject,要每个单词首字母都大写,推测原因就是这些都可以算是类吧,可以用来new一个个的实例的
各种命令语句也是这样,比如 Debug.Log();
但是各种实例下的属性的命名,一般就是,第1个单词的首字母小写,后面如果有其他单词则是第2个单词首字母大写,比较像 驼峰命名法 ,比如下面这种。
但是这仅限于,对象.属性这种写法,如果是作为参数等传递,还是要第1个单词的首字母大写的
- transform.position
- transform.localPosition
- 作为参数时
- Component c1=GetComponent<BoxCollider>();
- 暂时看到的是这样,希望总结是对的
- 大概是这个规律吧,不然这些大小写规律还挺难记,嘿嘿
还有1个声明变量规律
比如首次使用变量时,必须严格的声明变量的类型
但是有时候好像只要声明其属于的比较精确的大类也是可以的,不一定非得很精确到小类上,
Component c1=GetComponent<BoxCollider>();
BoxCollider c2=GetComponent<BoxCollider>();
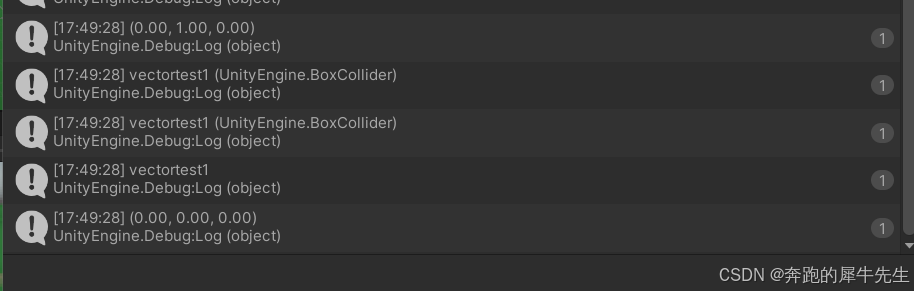
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class emptyTest : MonoBehaviour
{
public GameObject Cube1;
// Start is called before the first frame update
void Start()
{
//使用this获取脚本挂在的gameObject
GameObject gb1=this.gameObject;
Debug.Log(gb1.name);
Debug.Log(gb1.tag);
Debug.Log(gb1.layer);
//节省的写法,也就是this.gameObject 或gameObject 指向挂载物体
//也就是gameobject就默认是自身了,可以省略this
Debug.Log(gameObject.name);
//直接查找其他 GameObject的名字
GameObject test1=GameObject.Find("Cube1");
//test1=GameObject.FindWithTag("Cube1");
Debug.Log(test1.name);
//对和脚本没直接关联的 GameObject,不能直接读到,必须先用find
//Debug.Log(Cube.name);
//通过声明public,且挂载了Cuble1,可以直接读到 gb
Debug.Log(Cube1.name);
Debug.Log(Cube1.activeInHierarchy);
Debug.Log(Cube1.activeSelf);
// 获得组件的属性
Debug.Log(this.transform.position);
Debug.Log(transform.position);
Debug.Log(transform.localPosition);
Debug.Log(transform.rotation);
Debug.Log(transform.localRotation);
//Debug.Log(transform.scale);
Debug.Log(transform.lossyScale);
Debug.Log(transform.localScale);
Debug.Log(transform.right);
Debug.Log(transform.forward);
Debug.Log(transform.up);
// 获得组件的属性,通用的方法
Component c1=GetComponent<BoxCollider>();
BoxCollider c2=GetComponent<BoxCollider>();
Debug.Log(c1);
Debug.Log(c2);
Debug.Log(c1.name);
Debug.Log(c2.center);
//获取当前物体的子物体
//获取当前物体的父物体的
//创建
//销毁
}
// Update is called once per frame
void Update()
{
}
}
5.3 获取父子物体上的组件( 这几个方法,参数没搞清楚,待修改)
//获取当前物体的子物体的属性
Component c3=GetComponentInChildren<BoxCollider>();
Debug.Log("子物体");
Debug.Log(c3.name);
Debug.Log(c3.transform.position);
Component c4=GetComponentInParent<Transform>();
Debug.Log("父物体");
Debug.Log(c4.name);
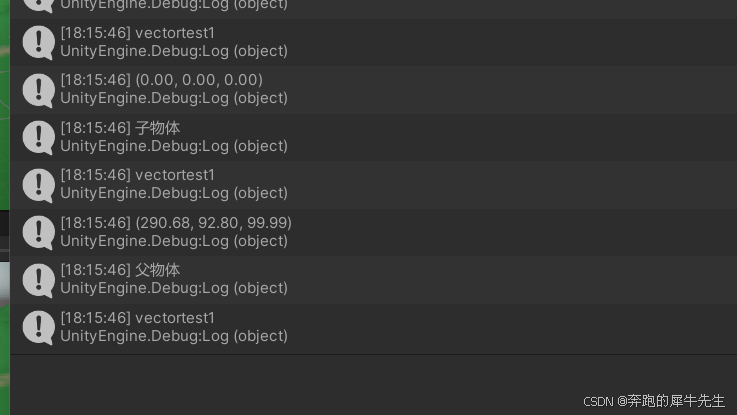
5.4 添加组件
- gameObject.AddComponent<AudioSource>();
- 首先这是一个方法
- gameObject.AddComponent<>();
- <> 需要写组件的名字,注意首字母要大写
//添加1个组件
gameObject.AddComponent<AudioSource>();
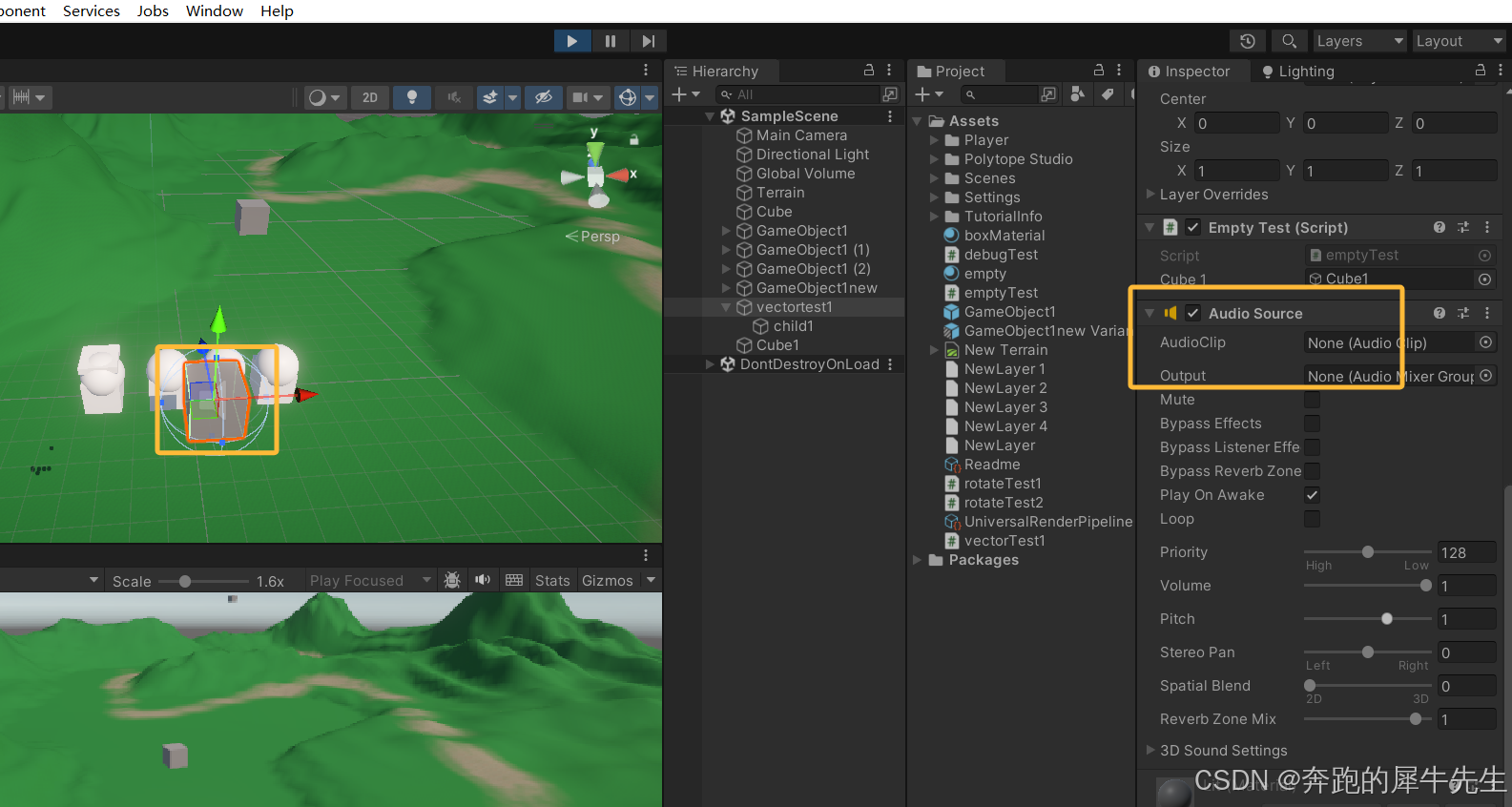
5.5 设置激活状态
- 找到某个gameObject以后
- 设置其激活状态图
- gameObject.SetActive(true)
6 通过代码添加物体,
- 最好是添加prefab,因为单独添加一个物品不适合copy操作,批量修改等
6.1 先声明
- public GameObject prefab1;
6.2 进行拖动关联
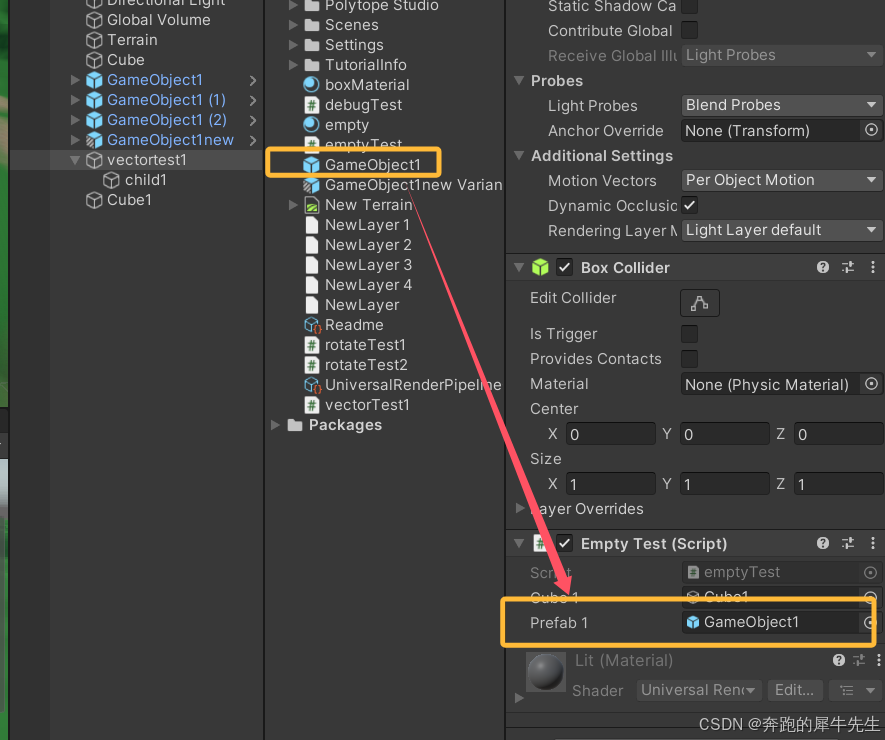
为了区分度明显,又换可一个黄色材质的预制体,先做了gb,然后拖到project来形成prefab
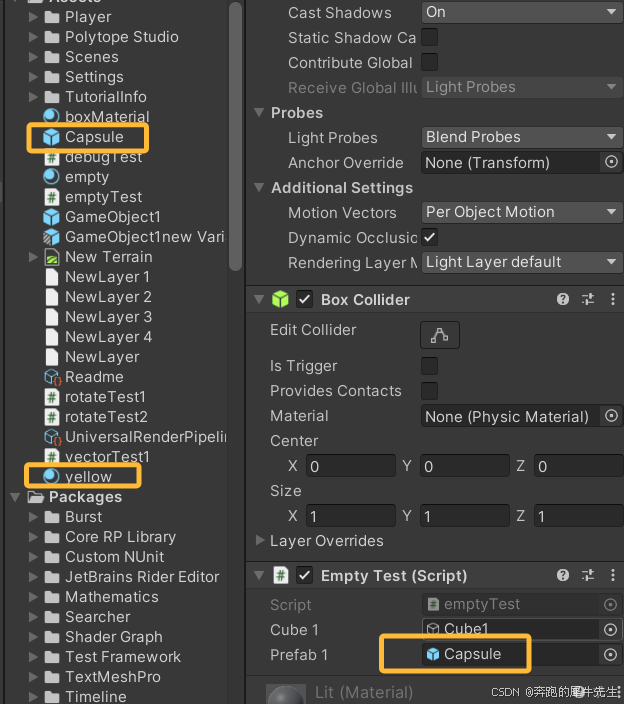
6.3 生成
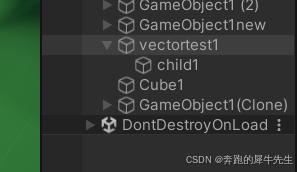
6.4 设置位置等
-
Instantiate(prefab1);
-
生成位置不知道为啥那么远
-
Instantiate(prefab1,transform);
-
生成的prefab将作为脚本的父物体的子物体
-
Instantiate(prefab1,transform.position,Quaternion.identity);
-
Vector3 v11=new Vector3(1,1,1);
-
GameObject gb11=Instantiate(prefab1,transform.position+v11,Quaternion.identity);
-
把新生成的故意挪开一定距离,方便辨认
//创建一个依赖prefab的gb
Instantiate(prefab1);
Instantiate(prefab1,transform);
Instantiate(prefab1,transform.position,Quaternion.identity);
Vector3 v11=new Vector3(1,1,1);
GameObject gb11=Instantiate(prefab1,transform.position+v11,Quaternion.identity);
//销毁
//Destory(gb11);
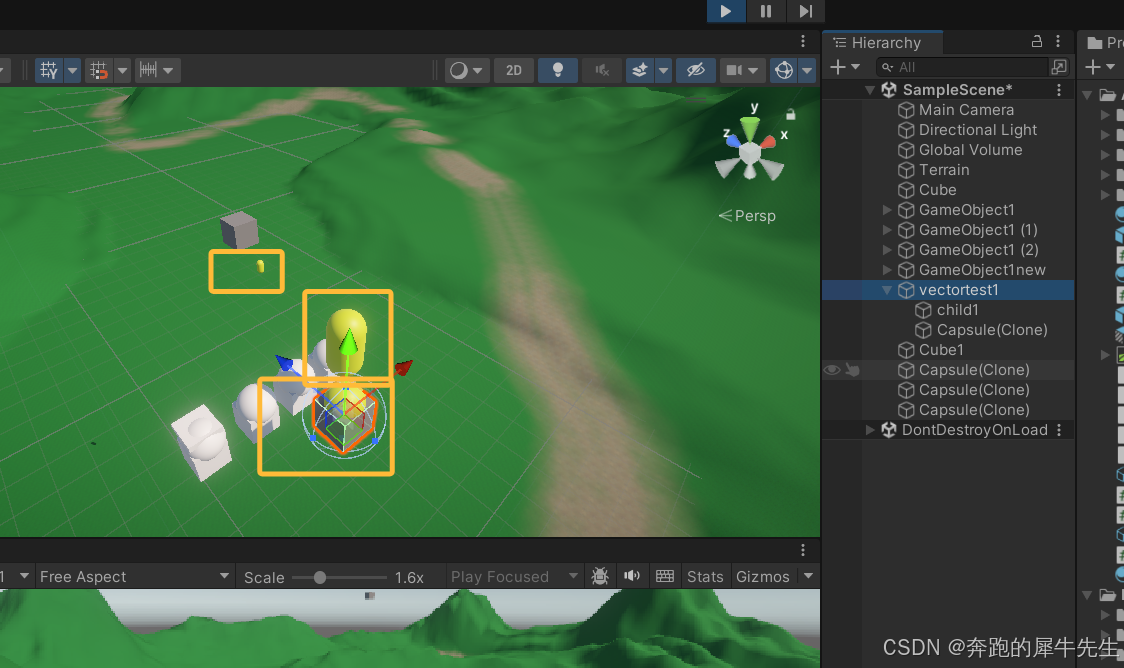
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class emptyTest : MonoBehaviour
{
public GameObject Cube1;
//获取prefab
public GameObject prefab1;
// Start is called before the first frame update
void Start()
{
//使用this获取脚本挂在的gameObject
GameObject gb1=this.gameObject;
Debug.Log(gb1.name);
Debug.Log(gb1.tag);
Debug.Log(gb1.layer);
//节省的写法,也就是this.gameObject 或gameObject 指向挂载物体
//也就是gameobject就默认是自身了,可以省略this
Debug.Log(gameObject.name);
//直接查找其他 GameObject的名字
GameObject test1=GameObject.Find("Cube1");
//test1=GameObject.FindWithTag("Cube1");
Debug.Log(test1.name);
//对和脚本没直接关联的 GameObject,不能直接读到,必须先用find
//Debug.Log(Cube.name);
//通过声明public,且挂载了Cuble1,可以直接读到 gb
Debug.Log(Cube1.name);
Debug.Log(Cube1.activeInHierarchy);
Debug.Log(Cube1.activeSelf);
// 获得组件的属性
Debug.Log(this.transform.position);
Debug.Log(transform.position);
Debug.Log(transform.localPosition);
Debug.Log(transform.rotation);
Debug.Log(transform.localRotation);
//Debug.Log(transform.scale);
Debug.Log(transform.lossyScale);
Debug.Log(transform.localScale);
Debug.Log(transform.right);
Debug.Log(transform.forward);
Debug.Log(transform.up);
// 获得组件的属性,通用的方法
Component c1=GetComponent<BoxCollider>();
BoxCollider c2=GetComponent<BoxCollider>();
Debug.Log(c1);
Debug.Log(c2);
Debug.Log(c1.name);
Debug.Log(c2.center);
//获取当前物体的子物体的属性
Component c3=GetComponentInChildren<BoxCollider>();
Debug.Log("子物体");
Debug.Log(c3.name);
Debug.Log(c3.transform.position);
Component c4=GetComponentInParent<Transform>();
Debug.Log("父物体");
Debug.Log(c4.name);
//添加1个组件
gameObject.AddComponent<AudioSource>();
//创建一个依赖prefab的gb
Instantiate(prefab1);
Instantiate(prefab1,transform);
Instantiate(prefab1,transform.position,Quaternion.identity);
Vector3 v11=new Vector3(1,1,1);
GameObject gb11=Instantiate(prefab1,transform.position+v11,Quaternion.identity);
//销毁
//Destory(gb11);
}
// Update is called once per frame
void Update()
{
}
}
6.5 销毁
GameObject gb11=Instantiate(prefab1,transform.position+v11,Quaternion.identity);
//销毁
Destory(gb11);