当你准备了一堆alpha 通过循环进行simulate的时候,由于登录过期等问题可能会出现如下报错
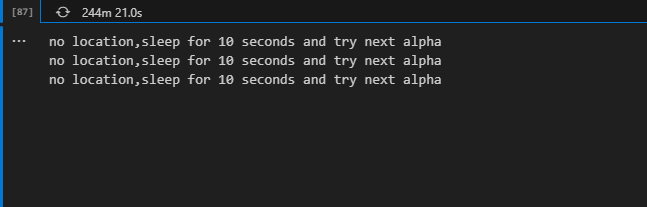
处理办法就是从中断的地方重新登录,重新开始,下面是一个解决问题的示例代码:
python
from time import sleep
import json
import requests
from os.path import expanduser
def login():
# Load credentials
with open(expanduser('brain_credentials.txt')) as f:
credentials = json.load(f)
# Extract username and password from the list
username, password = credentials
# Create a session object
sess = requests.Session()
# Set up basic authentication
sess.auth = HTTPBasicAuth(username, password)
# Send a POST request to the API for authentication
response = sess.post('https://api.worldquantbrain.com/authentication')
# Print response status and content for debugging
print(response.status_code)
print(response.text)
return sess
def main():
alpha_list = [] # 这里应该是你的 alpha 列表,你需要根据实际情况填充
sess = login()
index = 0 # 记录当前处理的 alpha 的索引
while index < len(alpha_list):
alpha = alpha_list[index]
try:
sim_resp = sess.post(
"https://api.worldquantbrain.com/simulations",
json=alpha,
)
sim_progress_url = sim_resp.headers.get('Location')
if sim_progress_url:
while True:
sim_progress_resp = sess.get(sim_progress_url)
retry_after_sec = float(sim_progress_resp.headers.get("Retry-After", 0))
if retry_after_sec == 0: # simulation done!
break
sleep(retry_after_sec)
alpha_id = sim_progress_resp.json()["alpha"] # the final simulation result
print(alpha_id)
else:
print("no location, sleep for 10 seconds and try next alpha")
sleep(10)
except Exception as e:
print(f"An error occurred: {e}")
print("Re-login and try again from the next alpha.")
sess = login()
index += 1 # 从下一个 alpha 开始
index += 1
if __name__ == "__main__":
main()