在电商领域,能够按关键字搜索并获取商品信息对于市场分析、选品和竞品研究至关重要。AliExpress(速卖通)作为全球知名的跨境电商平台,提供了丰富的商品数据。以下将详细介绍如何使用Python爬虫按关键字搜索AliExpress商品,并提供具体的代码示例。
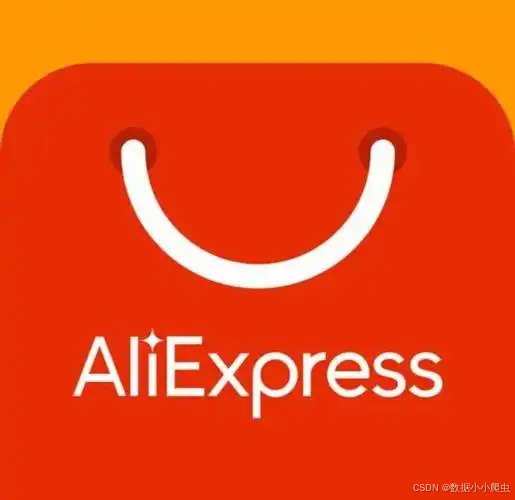
一、环境准备
在开始之前,确保你的Python环境中安装了以下库:
pip install requests beautifulsoup4 pandas
-
requests
:用于发送HTTP请求。 -
BeautifulSoup
:用于解析HTML内容。 -
pandas
:用于数据处理和存储。
二、编写爬虫代码
(一)发送HTTP请求
首先,我们需要使用requests
库来发送HTTP请求,获取AliExpress的商品搜索页面。
python
import requests
def search_products(keyword, num_pages=1):
base_url = "https://www.aliexpress.com/wholesale"
params = {
'SearchText': keyword
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
products = []
for page in range(1, num_pages + 1):
params['page'] = page
response = requests.get(base_url, params=params, headers=headers)
if response.status_code == 200:
products.extend(parse_products(response.text))
else:
print(f"Failed to retrieve data from page {page}")
return products
(二)解析HTML内容
获取到HTML内容后,我们使用BeautifulSoup
库来解析HTML,提取商品信息。
python
from bs4 import BeautifulSoup
def parse_products(html):
soup = BeautifulSoup(html, 'html.parser')
items = soup.find_all('div', class_='item')
product_list = []
for item in items:
title = item.find('a', class_='item-title').text.strip()
price = item.find('span', class_='price-current').text.strip()
link = item.find('a', class_='item-title')['href']
product_list.append({
'Title': title,
'Price': price,
'Link': link
})
return product_list
(三)整合代码并运行
最后,我们将上述代码整合,并运行爬虫程序。
python
import pandas as pd
def save_to_csv(products, filename='aliexpress_products.csv'):
df = pd.DataFrame(products)
df.to_csv(filename, index=False)
print(f"Data saved to {filename}")
if __name__ == "__main__":
keyword = input("Enter the keyword to search: ")
num_pages = int(input("Enter the number of pages to scrape: "))
products = search_products(keyword, num_pages)
save_to_csv(products)
三、注意事项
(一)遵守Robots协议
在进行网页爬取时,应该遵守目标网站的Robots协议,尊重网站的爬取规则。
(二)用户代理
在发送请求时,设置合适的用户代理(User-Agent),模拟真实用户的浏览器行为。
(三)频率控制
合理控制请求频率,避免对目标网站造成过大压力。
(四)异常处理
在实际的爬虫程序中,应该添加异常处理机制,以应对网络请求失败、解析错误等情况。
四、总结
通过本文的介绍,我们学习了如何使用Python爬虫在AliExpress上按关键字搜索商品,并将爬取到的数据保存到CSV文件中。这个过程不仅可以帮助我们快速获取商品信息,还可以为后续的数据分析和商业决策提供有力支持。希望本文对你有所帮助,祝你在数据爬取和分析的道路上取得更多成果!