线性表
顺序表
顺序表的静态分配
cs
//线性表的元素类型为 ElemType
//顺序表的静态分配
#define MaxSize=10
typedef int ElemType;
typedef struct{
ElemType data[MaxSize];
int length;
}SqList;
顺序表的动态分配
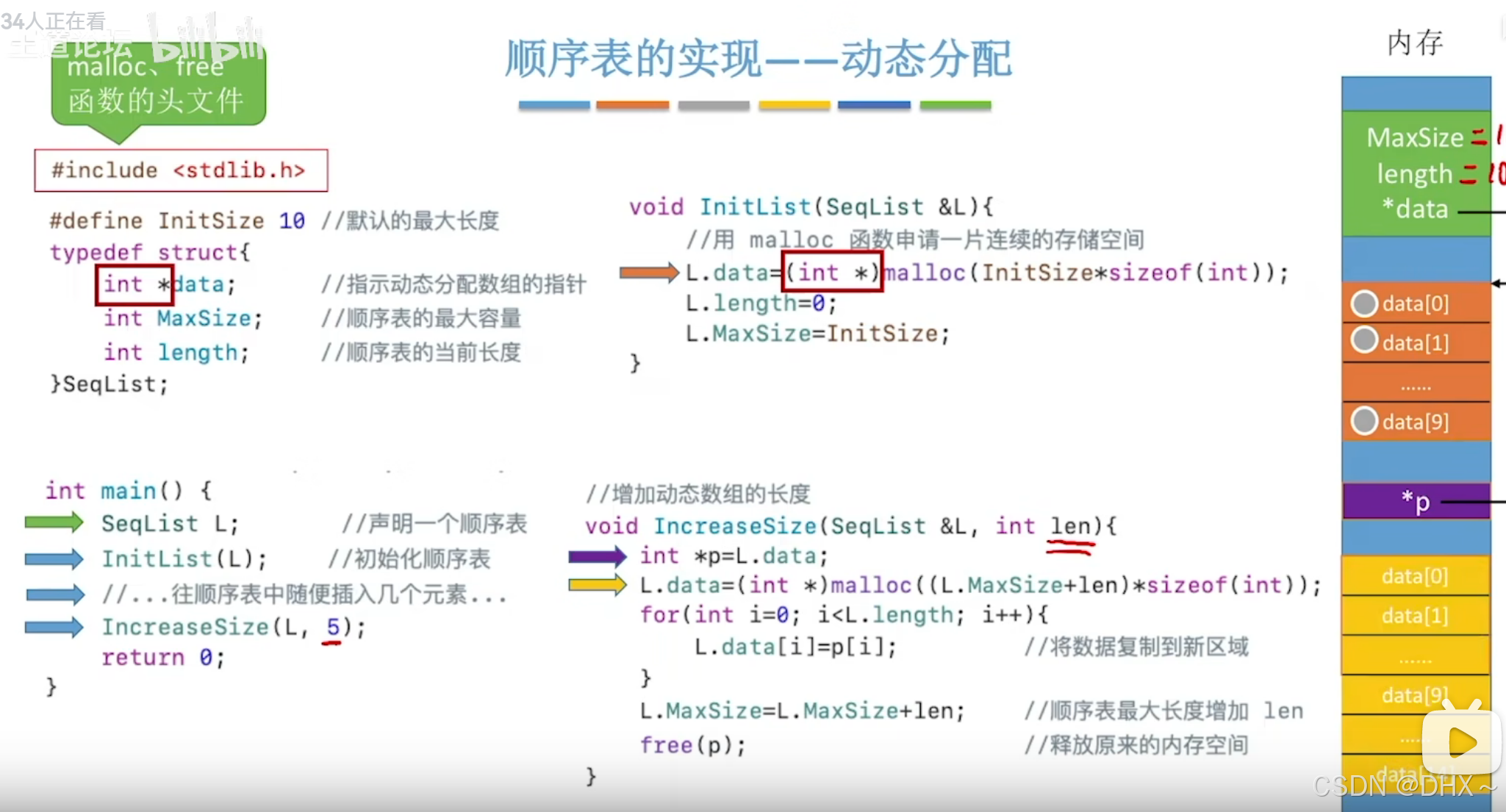
cs
//顺序表的动态分配
#define InitSize 10
typedef struct{
ElemType * data;
int MaxSize
int length;
}SqList;
//初始化
void InitList(SqList &L)
{
L.data=(ElemType *)malloc(InitSize*sizeof(ElemType));
L.length=0;
L.MaxSize=InitSize;
}
插入操作 O(n)
cs
//插入操作
#define MaxSize=10
typedef int ElemType;
typedef struct{
ElemType data[MaxSize];
int length;
}SqList;
bool ListInsert(SqList &L,int i,int e)
{
if(i<1||i>L.length+1) return false;
if(L.length==MaxSize) return false;
for(int j=L.length;j>=i;j--)
{
L.data[j]=L.data[j-1];
}
L.data[i-1]=e;
return true;
}
删除操作 O(n)
cs
//删除操作
#define MaxSize=10
typedef int ElemType;
typedef struct{
ElemType data[MaxSize];
int length;
}SqList;
bool ListDelete(SqList &L,int i,ElemType &e)
{
if(i<1||i>L.length) return false;
e=L.data[i-1];
for(int j=i;j<L.length;j++)
{
L.data[j-1]=L.data[j];
}
L.length--;
return true;
}
按值查找****O(n)
cs
int LocateElem(SqList L,ElemType e)
{
int i;
for(i=0;i<L.length;i++)
{
if(L.data[i]==e) return i+1;
}
return 0;
}