需求
项目想要生成一个更新版本号,格式为v2.0.20250101
。
其中v2.0
为版本号,更新时进行配置;20250101
为更新日期,版本更新时自动生成。
实现思路
创建一个配置文件version.properties
,在其中配置版本号;
properties
# 版本号
version.number=v2.0
# 版本更新时间
version.build-date=maven-build-timestamp
再写一个类VersionController
,读取配置文件中的属性值,
同时定义一个接口,将version.number
和version.build-date
拼接后返回,即为版本号
前端调用这个接口,拿到版本号后展示。
如何读取自定义配置文件,可以看我写的这篇文章:读取自定义配置文件的属性值
Maven replacer插件替换更新时间
这里有一个比较麻烦的点是,版本更新时间 我不想手动去写,而是在版本更新时自动生成就好。
这里就要用到 maven 的一个插件 replacer ,这个插件可以在 maven 构建过程中执行文本替换操作。
因此,使用这个插件,在 maven 打包时将配置文件version.properties
的 version.build-date
值,替换为 maven 的打包时间,即实现了版本更新时间的自动生成。
xml
<build>
<plugins>
<!--replacer插件,用于替换src/main/resources/version.properties中的版本更新时间,以生成版本号-->
<plugin>
<groupId>com.google.code.maven-replacer-plugin</groupId>
<artifactId>replacer</artifactId>
<version>1.5.3</version>
<executions>
<execution>
<!-- 可以在这个阶段进行代码编译、资源文件处理、生成文档、运行单元测试等;使用这个无法直接替换class文件的值 -->
<!--<phase>prepare-package</phase>-->
<!-- 可以在这个阶段设置一些默认的属性值、配置系统属性、加载外部资源文件等 -->
<phase>initialize</phase>
<goals>
<goal>replace</goal>
</goals>
</execution>
</executions>
<configuration>
<!--指定文件路径-->
<basedir>${basedir}/src/main/resources</basedir>
<!--指定具体的文件名-->
<includes>
<include>**/version.properties</include>
</includes>
<replacements>
<replacement>
<!--替换的目标文本-->
<token>maven-build-timestamp</token>
<!--替换后的值-->
<value>${maven.build.timestamp}</value>
</replacement>
</replacements>
</configuration>
</plugin>
</plugins>
</build>
完整代码
定义配置文件src/main/resources/version.properties
properties
# 版本号
version.number=v2.0
# 版本更新时间
version.build-date=maven-build-timestamp
pom.xml 中使用 replacer插件:
- 插件效果:在 maven 打包时将配置文件
version.properties
的version.build-date
值,替换为 maven 的打包时间。 - 打包时间的格式可以通过 properties 属性
maven.build.timestamp.format
更改。
xml
<properties>
<!--定义打包时间的格式-->
<!--yyyyMMddHHmmss-->
<maven.build.timestamp.format>yyyyMMdd</maven.build.timestamp.format>
</properties>
<build>
<plugins>
<!--replacer插件,用于替换src/main/resources/version.properties中的版本更新时间,以生成版本号-->
<plugin>
<groupId>com.google.code.maven-replacer-plugin</groupId>
<artifactId>replacer</artifactId>
<version>1.5.3</version>
<executions>
<execution>
<!-- 可以在这个阶段进行代码编译、资源文件处理、生成文档、运行单元测试等;使用这个无法直接替换class文件的值 -->
<!--<phase>prepare-package</phase>-->
<!-- 可以在这个阶段设置一些默认的属性值、配置系统属性、加载外部资源文件等 -->
<phase>initialize</phase>
<goals>
<goal>replace</goal>
</goals>
</execution>
</executions>
<configuration>
<!--指定文件路径-->
<basedir>${basedir}/src/main/resources</basedir>
<!--指定具体的文件名-->
<includes>
<include>**/version.properties</include>
</includes>
<replacements>
<replacement>
<!--替换的目标文本-->
<token>maven-build-timestamp</token>
<!--替换后的值-->
<value>${maven.build.timestamp}</value>
</replacement>
</replacements>
</configuration>
</plugin>
</plugins>
</build>
定义类 VersionController:
- 使用 @PropertySource 读取配置文件,@Value 读取配置文件的属性值。
- 接口方法 getVersion 中将 versionNumber 和 buildDate,使用
.
拼接后返回。
java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.PropertySource;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@PropertySource(value = {"classpath:version.properties"})
@RequestMapping("/version")
public class VersionController {
@Value("${version.number}")
private String versionNumber;
@Value("${version.build-date}")
private String buildDate;
@GetMapping
public String getVersion() {
return String.join(".", versionNumber, buildDate);
}
}
测试
项目执行 maven 打包命令
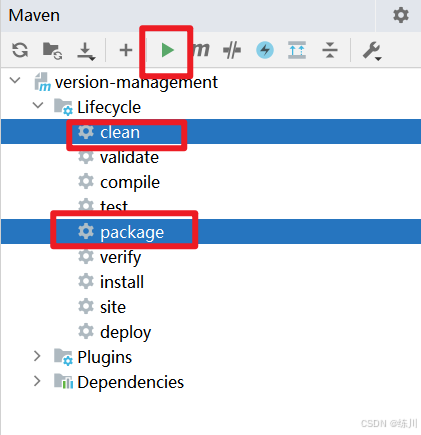
找到打包后的 jar 目录
目录中输入 cmd 进入命令行窗口,命令行执行java -jar jar包名
启动项目(jar包名 需替换为你自己的)
进行浏览器访问接口验证
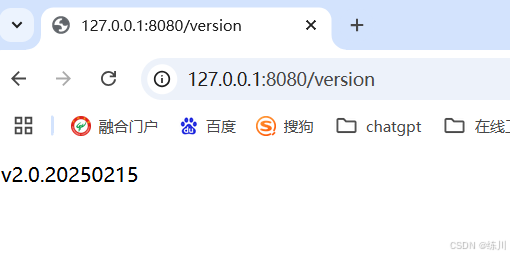
版本更新时的操作
在版本更新时,需要去修改配置文件version.properties
的 version.number
版本号数字,之后 git push 本次修改。
然后,使用 Jenkins 重新打包项目,版本号就会自动更新。
如果有帮助的话,可以点个赞支持一下嘛
🙏