3.2.1 配置系统的基本使用
.NET Core中的配置系统支持非常丰富的配置源,包括文件(JSON、XML、INI等)、注册表、环境变量、命令行、Azure Key Vault等,配置系统还支持自定义配置源。
用配置系统开发包Microsoft.Extensions.Configuration(这是.NetCore 中配置系统的一个基础开发包), 通过Microsoft.Extensions.Configuration.Json读取JSON配置文件:
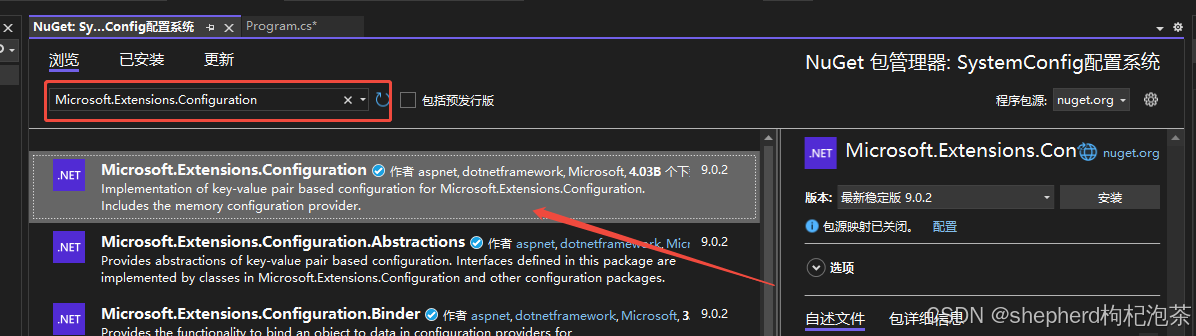
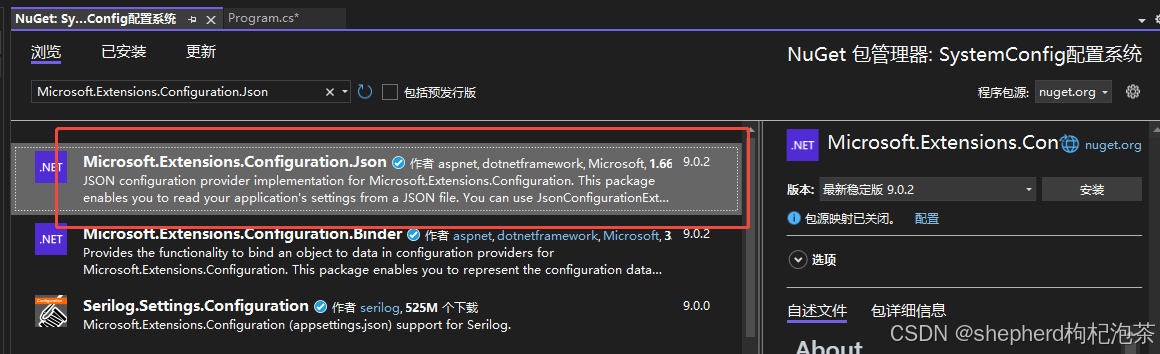
在项目中添加一个JSON文件:
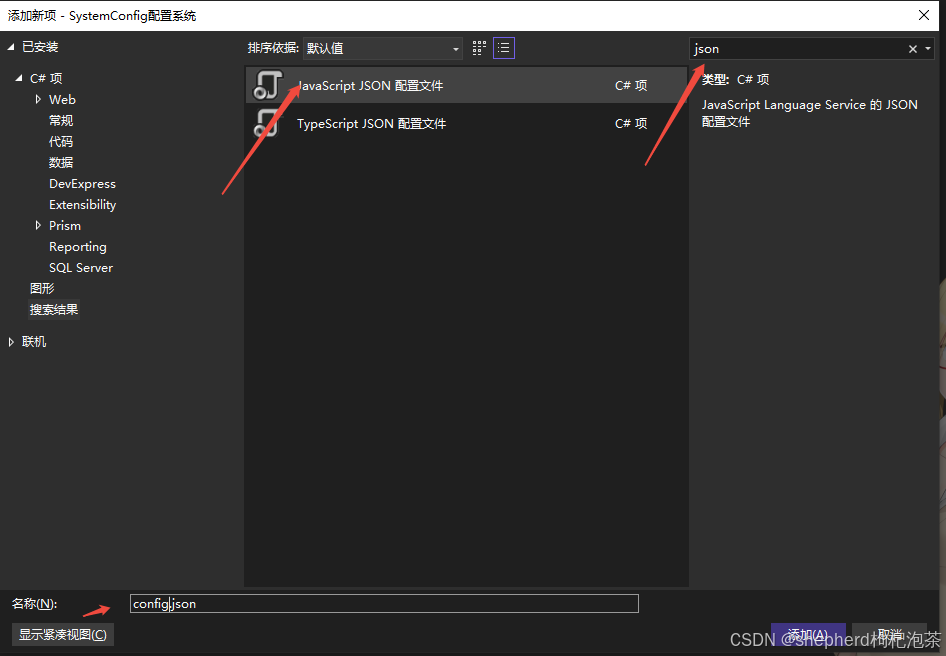
cs
{
"name": "枸杞泡茶",
"proxy": {
"address": "192.168.1.250",
"port": "5432"
}
}
设置文件属性:如果较新则复制
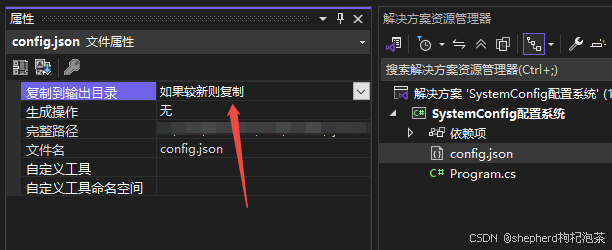
读取配置:
cs
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Configuration.Json;
using System.Runtime.InteropServices;
ConfigurationBuilder cfBuilder = new ConfigurationBuilder();
cfBuilder.AddJsonFile("config.json",optional:false,reloadOnChange:false);
IConfigurationRoot config = cfBuilder.Build();
string name = config["name"];
Console.WriteLine($"name={name}");
string proxyAddress = config.GetSection("proxy:address").Value;
Console.WriteLine($"proxyAddress={proxyAddress}");
Console.ReadKey();
调试结果:

3.2.2 使用选项方式读取配置
这是.NET Core中比较推荐的方式,因为他跟依赖注入机制结合的更好,且可实现配置修改后的自动刷新,所以更方便使用。在使用这种方式时需要安装两个包:
Microsoft.Extensions.Options
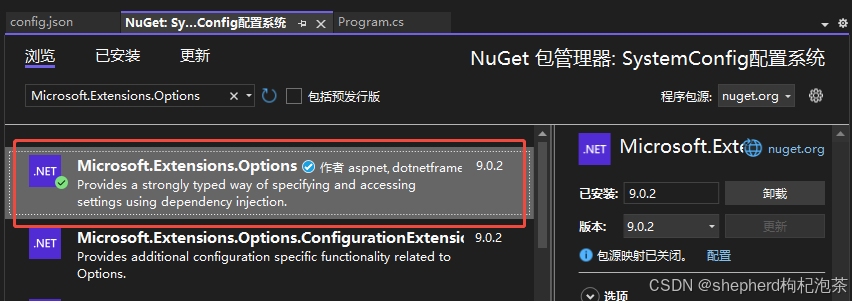
Microsoft.Extensions.Configuration.Binder
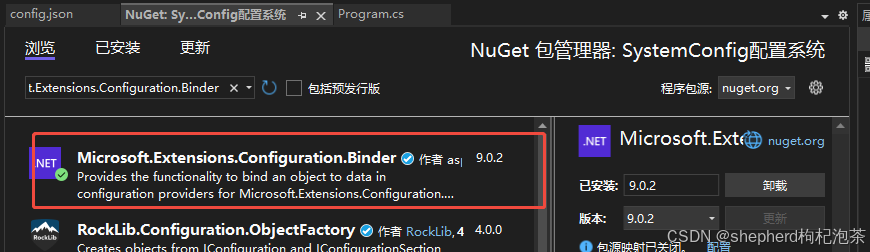
添加一个文件appsetting.json:
cs
{
"Logging": { "LogLevel": { "Default": "warning" } },
"DB": {
"DbType": "SQLServer",
"ConnectionString": "Data Source=.,Initial Catalog=DemoDB; Integrated Security=True"
},
"Smtp": {
"Server": "smtp.youzack.com",
"UserName": "zack",
"Password": "hello888",
"AllowedHosts": "*"
}
}
建立对应配置的模型:
cs
public class DbSettings
{
public string DbType { get; set; }
public string ConnectionString { get; set; }
}
public class SmtpSettings
{
public string Server { get; set; }
public string UserName { get; set; }
public string Password { get; set; }
}
选项方式读取配置时需要和依赖注入一起使用,因此创建一个类获取注入的选项值。
书中所讲,有三种泛型接口类型可使用:IOptions<T> 、IOptionsMonitor<T> 、IOptionsSnapshot<T> 。结合三者的特性:IOptions<T> 不能即时修改应用,IOptionsMonitor<T> 在范围内不保持一致,IOptionsSnapshot<T> 可在同一范围内保持一致。所以主要讲解了IOptionsSnapshot<T> 。
这是读取配置的Demo类:
cs
class Demo
{
private readonly IOptionsSnapshot<DbSettings> optDbSettings;
private readonly IOptionsSnapshot<SmtpSettings> optSmtpSettings;
public Demo(IOptionsSnapshot<DbSettings> optDbSettings, IOptionsSnapshot<SmtpSettings> optSmtpSettings)
{
this.optDbSettings = optDbSettings;
this.optSmtpSettings = optSmtpSettings;
}
public void Test()
{
var db = optDbSettings.Value;
Console.WriteLine($"数据库:{db.DbType},{db.ConnectionString}");
var smtp = optSmtpSettings.Value;
Console.WriteLine($"Smtp:{smtp.Server},{smtp.UserName},{smtp.Password}");
}
}
编写注入服务到容器的代码:
cs
// Configure and read from appsettings.json
ConfigurationBuilder configBuilder = new ConfigurationBuilder();
configBuilder.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true);
IConfigurationRoot config = configBuilder.Build();
// Set up dependency injection
ServiceCollection services = new ServiceCollection();
services.AddOptions().Configure<DbSettings>(e => config.GetSection("DB").Bind(e)).Configure<SmtpSettings>(e => config.GetSection("smtp").Bind(e));
services.AddTransient<Demo>();
// Build service provider
using (var sp = services.BuildServiceProvider())
{
while (true)
{
using (var scope = sp.CreateScope())
{
var spScope = scope.ServiceProvider;
var demo = spScope.GetRequiredService<Demo>();
demo.Test();
// Access and print SMTP settings
var smtpSettings = spScope.GetRequiredService<IOptionsSnapshot<SmtpSettings>>().Value;
Console.WriteLine($"Smtp: {smtpSettings.Server}, {smtpSettings.UserName}, {smtpSettings.Password}");
}
Console.WriteLine("可以改配置啦");
Console.ReadKey();
}
}
启动调试,不退出程序修改配置文件并保存:
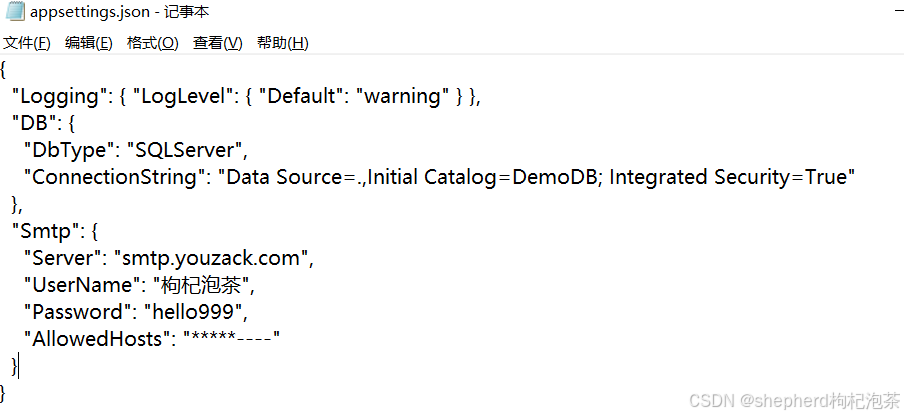
Enter健继续执行:程序内读取的参数已经发生变化:
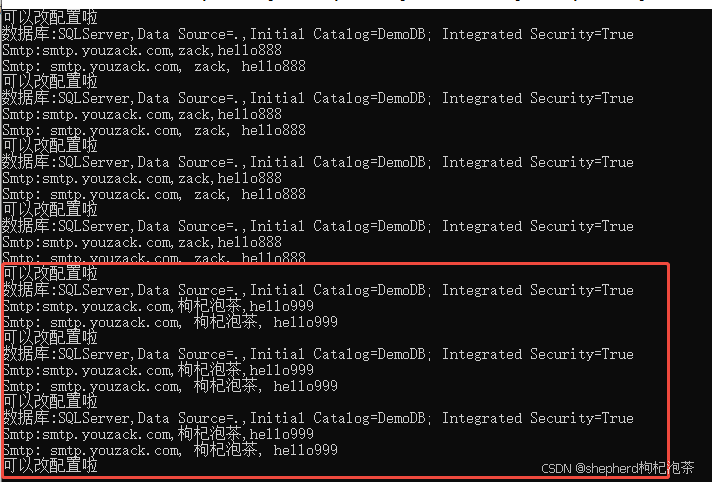
3.2.3 从命令行读取配置
通过NuGet安装:
Microsoft.Extensions.Configuration
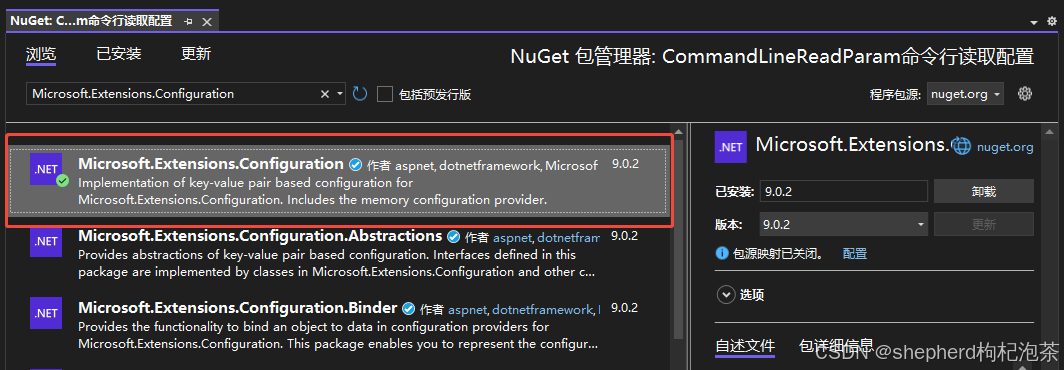
Microsoft.Extensions.Configuration.CommandLine
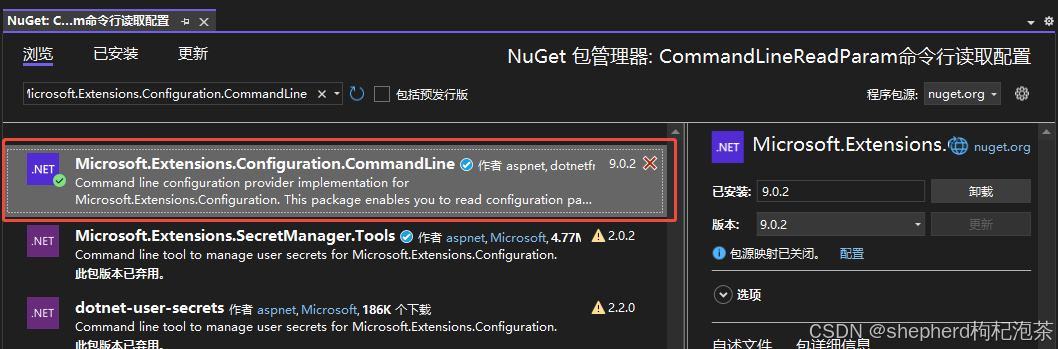
读取:
cs
using Microsoft.Extensions.Configuration.CommandLine;
using Microsoft.Extensions.Configuration;
ConfigurationBuilder configBuilder = new ConfigurationBuilder();
configBuilder.AddCommandLine(args);
IConfigurationRoot config = configBuilder.Build();
string name = config["Name"];
Console.WriteLine($"Name:{name}");
启动调试:
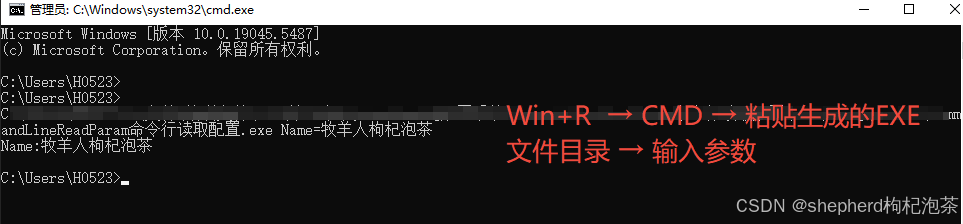
3.2.4 从环境变量读取配置
安装NuGet包 Microsoft.Extensions.Configuration.EnvironmentVariables
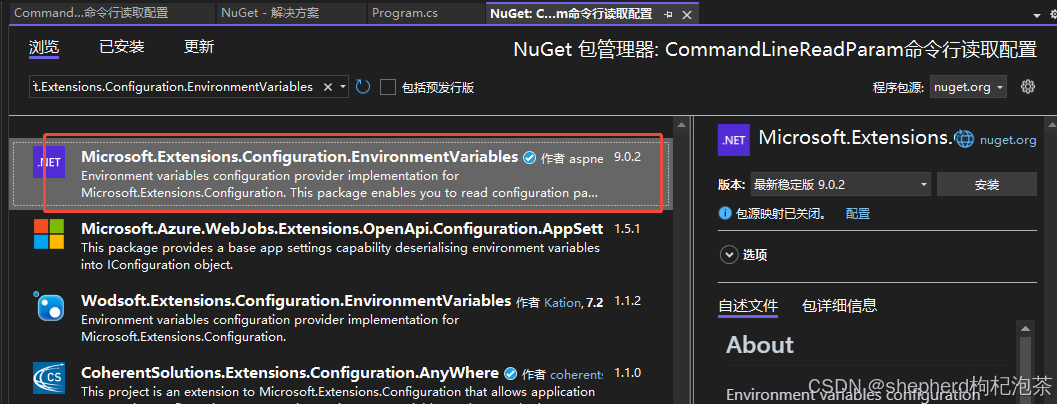
例如,有这样一个环境变量:
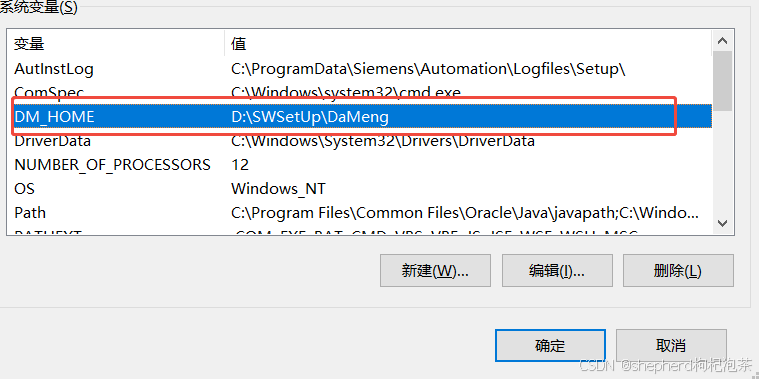
读取:
cs
using Microsoft.Extensions.Configuration;
ConfigurationBuilder configBuilder = new ConfigurationBuilder();
configBuilder.AddEnvironmentVariables("DM_");
IConfigurationRoot config = configBuilder.Build();
string home = config["HOME"];
//name = Environment.GetEnvironmentVariable("DM_HOME");
Console.WriteLine($"Home:{home}");
Console.ReadKey();
读取结果
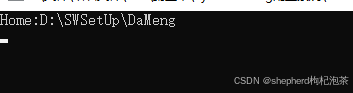
3.2.5 其他配置提供程序
讲了一个Apollo,可以在.NET Core 中连接Apollo读取配置
3.2.6 案例:开发自己的配置提供程序
这里讲了杨老师自己开发的一个配置一共程序:Zack.AnyDBConfigProvider。
3.2.7 多配置源问题
.NET Core 的配置系统中允许添加多个配置源,且遵循一个原则:后添加的配置提供程序中的配置覆盖之前的配置。