s
#include <iostream>
#include <cstring>
using namespace std;
class mystring
{
private:
char*p;
int len;
public:
mystring(const char* p = NULL);
~mystring();
void copy(const mystring& str);
void append(const mystring& str);
void show();
bool compare(const mystring& str);
void swap(mystring& str);
void operator=(const mystring& str);
mystring operator+(const mystring& str);
char& operator[](int i);
void operator+=(const mystring& str);
};
//默认参数构造函数
mystring::mystring(const char* p)
:p(NULL),len(0)
{
if(p != NULL)
{
this->len = strlen(p);
this->p = new char[len+1];
strcpy(this->p,p);
}
}
//析构函数
mystring::~mystring()
{
if(p != NULL)
{
delete[] p;
}
}
//成员方法
/*******************************************************************/
//拷贝功能
void mystring::copy(const mystring& str)
{
if(p != NULL)
{
delete[] p;
p = NULL;
}
len = str.len;
if(str.p == NULL)
{
p = NULL;
}
else
{
p = new char[len+1];
strcpy(p,str.p);
}
}
//追加功能
void mystring::append(const mystring& str)
{
if(str.p == NULL)
{
return;
}
char* temp = p;
len = len + str.len;
p = new char[len];
strcpy(p,temp);
strcat(p,str.p);
}
//打印功能
void mystring::show()
{
if(p == NULL)
{
return;
}
cout << p <<endl;
}
//比较功能
bool mystring::compare(const mystring& str)
{
return strcmp(p,str.p) == 0;
}
//交换功能
void mystring::swap(mystring& str)
{
char* temp = p;
int i = len;
p = str.p;
str.p = temp;
len = str.len;
str.len = i;
}
//运算符重载
/**********************************************************/
//重载"="
void mystring::operator=(const mystring& str)
{
if(p != NULL)
{
delete[] p;
p = NULL;
}
if(str.p == NULL)
{
p = NULL;
len = 0;
}
else
{
len = str.len;
p = new char[len+1];
strcpy(p,str.p);
}
}
//重载"+"
mystring mystring::operator+(const mystring& str)
{
mystring temp;
temp.len = len + str.len;
temp.p = new char[temp.len];
strcpy(temp.p,p);
strcat(temp.p,str.p);
return temp;
}
//重载"+="
void mystring::operator+=(const mystring& str)
{
if(str.p == NULL)
{
return;
}
char* temp = p;
len = len + str.len;
p = new char[len];
strcpy(p,temp);
strcat(p,str.p);
if(temp != NULL)
{
delete[] temp;
}
}
//重载"[]"
char& mystring::operator[](int i)
{
return p[i];
}
int main()
{
mystring str("0000");
mystring ptr("11111");
str = ptr + str;
str.show();
str[0] = 'A';
str.show();
str += ptr;
str.show();
return 0;
}
第二题
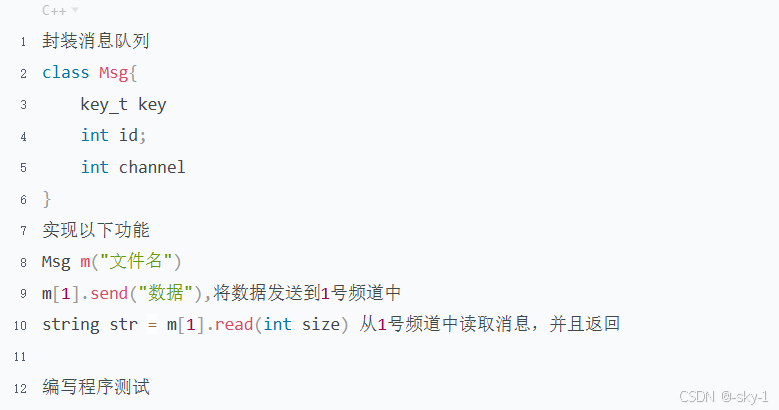
#include<iostream>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <cstring>
#include <unistd.h>
#include <pthread.h>
using namespace std;
struct msg_pack
{
long channel;
char buf[64];
};
class Msg
{
private:
key_t key;
int id;
int channel;
public:
Msg(const char* file);
~Msg();
void send(const char* buf);
string read();
Msg& operator[](int num);
};
//构造函数定义
Msg::Msg(const char* file)
{
if(access(file,F_OK) == -1)
{
cout<<"文件不存在"<<endl;
return;
}
key = ftok(file,1);
id = msgget(key,IPC_CREAT|0664);
}
//析构函数定义
Msg::~Msg()
{
msgctl(id,IPC_RMID,NULL);
}
//成员方法:读取数据
string Msg::read()
{
msg_pack pack = {0};
pack.channel = channel;
msgrcv(id,&pack,sizeof(pack.buf),channel,0);
return pack.buf;
}
//成员方法:发送数据
void Msg::send(const char* buf)
{
msg_pack pack = {0};
pack.channel = channel;
strcpy(pack.buf,buf);
msgsnd(id,&pack,strlen(pack.buf),IPC_NOWAIT);
}
//重载"[]"运算符,将里面的数据写入消息频道
Msg& Msg::operator[](int num)
{
channel = num;
return *this;
}
void* pthread_main(void* arg)
{
Msg* g = (Msg*)arg;
while(1)
{
string s = g[2].read();
cout <<"\b\b\b\b\b\b\b"<<"收到的消息为:"<< s <<endl;
}
}
int main()
{
pthread_t id;
Msg m("1.txt");
pthread_create(&id,0,pthread_main,&m);
char brr[64] = "";
while(1)
{
memset(brr,0,sizeof(brr));
cout<<"请输入:";
cin>>brr;
m[1].send(brr);
}
return 0;
}
第三题
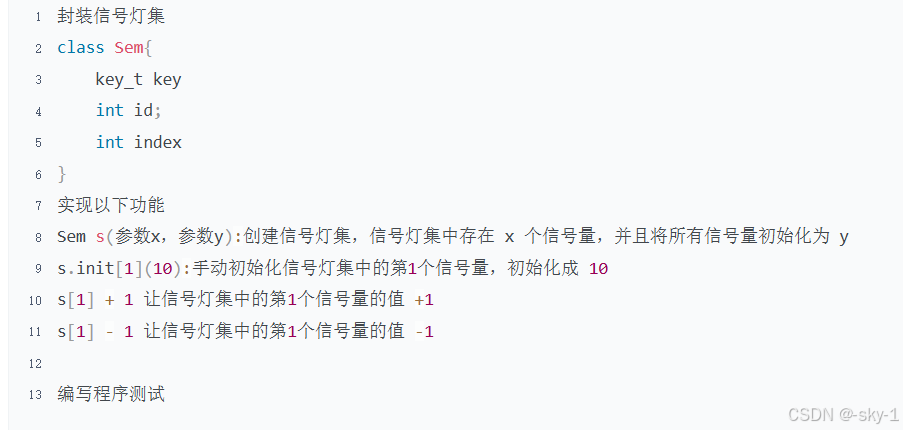
#include <iostream>
#include <sys/sem.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
using namespace std;
class Sem
{
private:
key_t key;
int id;
int index;
public:
Sem(const char* file,int x,int y);
~Sem();
void init(int num);
Sem& operator[](int a);
friend void operator+(const Sem& m,int i);
friend void operator-(const Sem& m,int i);
};
//构造函数
Sem::Sem(const char* file,int x,int y)
{
if(key = ftok(file,1) == -1)
{
cout <<"没有该文件"<<endl;
}
id = semget(key,x,IPC_CREAT|0666);
for(int i=0; i<x; i++)
{
semctl(id,i,SETVAL,y);
}
}
//析构函数
Sem::~Sem()
{
semctl(id,0,IPC_RMID);
}
//手动初始化
void Sem::init(int num)
{
semctl(id,index,SETVAL,num);
}
//内重载"[]"(写入下标)
Sem& Sem::operator[](int index)
{
this->index = index;
return *this;
}
//外重载"+"(解锁)
void operator+(const Sem& m,int i)
{
struct sembuf buf = {0};
buf.sem_num = m.index;
buf.sem_op = i;
buf.sem_flg = SEM_UNDO;
semop(m.id,&buf,1);
}
//外重载"-"(解锁)
void operator-(const Sem& m,int i)
{
struct sembuf buf = {0};
buf.sem_num = m.index;
buf.sem_op = (0-i);
buf.sem_flg = SEM_UNDO;
semop(m.id,&buf,1);
}
int main()
{
Sem s("2.txt",2,0);
s[1].init(1);
pid_t pid = fork();
if(pid>0)
{
while(1)
{
s[1] - 1;
cout << "父进程" <<endl;
sleep(1);
s[0] + 1;
}
}
if(pid == 0)
{
while(1)
{
s[0] - 1;
cout << "子进程" <<endl;
sleep(1);
s[1] + 1;
}
}
return 0;
}